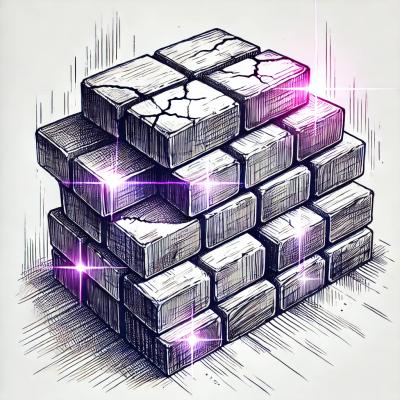
Security News
Input Validation Vulnerabilities Dominate MITRE's 2024 CWE Top 25 List
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
@biopassid/face-sdk-react-native
Advanced tools
Key Features • Customizations • Quick Start Guide • FaceConfig • FaceEvent • Changelog • License • Support
Increase the security of your applications without harming your user experience.
All customization available:
Enable or disable components:
Change all colors:
Check out our official documentation for more in depth information on BioPass ID.
Android | iOS | |
---|---|---|
Support | SDK 21+ | iOS 13+ |
- A device with a camera
- License key
- Internet connection is required to verify the license
npm install @biopassid/face-sdk-react-native
Change the minimum Android sdk version to 21 (or higher) in your android/app/build.gradle
file.
minSdkVersion 21
Change the compile Android sdk version to 31 (or higher) in your android/app/build.gradle
file.
compileSdkVersion 31
Requires iOS 13.0 or higher.
Add two rows to the ios/Info.plist
:
Privacy - Camera Usage Description
and a usage description.Privacy - Photo Library Usage Description
and a usage description.If editing Info.plist as text, add:
<key>NSCameraUsageDescription</key>
<string>Your camera usage description</string>
<key>NSPhotoLibraryUsageDescription</key>
<string>Your library usage description</string>
Then go into your project's ios folder and run pod install.
# Go into ios folder
$ cd ios
# Install dependencies
$ pod install
To call Face Capture in your React Native project is as easy as follow:
import React from "react";
import { StyleSheet, View, Button } from "react-native";
import {
FaceConfigPreset,
FaceConfigType,
FaceEvent,
buildCameraView,
FaceCameraLensDirection,
} from "@biopassid/face-sdk-react-native";
export function App() {
const config = FaceConfigPreset.getConfig(
FaceConfigType.FACE_CAPTURE
)
.setLicenseKey("your-license-key")
.setDefaultCameraPosition(FaceCameraLensDirection.FRONT)
.setShowFlipCameraButton(true)
.setStringsScreenTitle("Capturando Face")
.setStylesOverlayColor("#CC000000");
function callback(event: FaceEvent) {
// handle Face return
console.log('Photo: ', event.photo.substring(0, 20));
}
function handleButton() {
buildCameraView(config, callback);
};
return (
<View style={styles.container}>
<Button onPress={handleButton} title="Capture Face" />
</View>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
alignItems: "center",
justifyContent: "center",
backgroundColor: '#FFFFFF',
},
});
For this example we used the Enroll from the Multibiometrics plan.
Here, you will need an API key to be able to make requests to the BioPass ID API. To get your API key contact us through our website BioPass ID.
import React from 'react';
import {StyleSheet, View, Button} from 'react-native';
import {
FaceConfigPreset,
FaceConfigType,
FaceEvent,
buildCameraView,
} from '@biopassid/face-sdk-react-native';
export default function App() {
// Instantiate Face config by passing your license key
const config = FaceConfigPreset.getConfig(
FaceConfigType.FACE_CAPTURE,
).setLicenseKey('your-license-key');
// Handle Face callback
async function callback(event: FaceEvent) {
// Create headers passing your api key
const headers = {
'Content-Type': 'application/json',
'Ocp-Apim-Subscription-Key': 'your-api-key',
};
// Create json body
const body = JSON.stringify({
Person: {
CustomID: 'your-customID',
Face: [{'Face-1': event.photo}],
},
});
// Execute request to BioPass ID API
const response = await fetch(
'https://api.biopassid.com/multibiometrics/enroll',
{
method: 'POST',
headers,
body,
},
);
const data = await response.json();
// Handle API response
console.log('Response status: ', response.status);
console.log('Response body: ', data);
}
// Build Face camera view
function handleButton() {
buildCameraView(config, callback);
}
return (
<View style={styles.container}>
<Button onPress={handleButton} title="Capture Face" />
</View>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
alignItems: 'center',
justifyContent: 'center',
backgroundColor: '#FFFFFF',
},
});
You can pass a function callback to receive the captured image. Images are returned in Base64 string format. You can write you own callback following this example:
function callback(event: FaceEvent) {
console.log('Photo: ', event.photo.substring(0, 20));
doSomething(event);
}
To use Face Capture you need a license key. To set the license key needed is simple as setting another attribute. Simply doing:
const config = FaceConfigPreset.getConfig(FaceConfigType.FACE_CAPTURE)
.setLicenseKey('your-license-key');
For automatic capture to work, the autoCapture needs to be enabled. By default, autoCapture is already enabled. If autoCapture is disabled, automatic capture will be disabled and the responsibility for capturing will be the user's responsibility through a button. For continuous capture, autoCapture will be ignored and automatic capture will always occur. To set the autoCapture is simple as setting another attribute. Simply doing:
const config = FaceConfigPreset.getConfig(FaceConfigType.FACE_CAPTURE)
.setLicenseKey('your-license-key')
.setAutoCapture(true);
To use the autoCaptureTimeout, the autoCapture needs to be on and a time in milliseconds needs to be passed to autoCaptureTimeout. By default, the autoCaptureTimeout value is 3000ms for single capture and 1000ms for continuous capture. If the autoCaptureTimeout is equal to zero, the capture will be instantaneous. To set the autoCaptureTimeout is simple as setting another attribute. Simply doing:
const config = FaceConfigPreset.getConfig(FaceConfigType.FACE_CAPTURE)
.setLicenseKey('your-license-key')
.setAutoCapture(true)
.setAutoCaptureTimeout(3000);
You can use the maxNumberFrames to set a maximum number of frames to be captured during continuous capture. For the single capture, the maxNumberFrames will always be ignored. If the maxNumberFrames is less than one or null, the capture will continue until the user presses the back button. Setting maxNumberFrames is as simple as setting another attribute. Simply by doing:
const config = FaceConfigPreset.getConfig(FaceConfigType.CONTINUOUS_CAPTURE)
.setLicenseKey('your-license-key')
.setMaxNumberFrames(5);
You can also use pre-build configurations on your application, so you can automatically start using multiples services and features that better suit your application. You can instantiate each one and use it's default properties, or if you prefer you can change every config available. Here are the types that are supported right now:
Variable name | Type | Default value |
---|---|---|
licenseKey | string | "" |
cameraPreset | FaceCameraPreset | FaceCameraPreset.MEDIUM |
defaultCameraPosition | FaceCameraLensDirection | FaceCameraLensDirection.FRONT |
outputFormat | FaceOutputFormat | FaceOutputFormat.JPEG |
captureButtonType | FaceCaptureButtonType | FaceCaptureButtonType.DEFAULT |
showFlashButton | boolean | false |
showFlipCameraButton | boolean | true |
flashEnabledByDefault | boolean | false |
showHelpText | boolean | true |
showScreenTitle | boolean | true |
autoCapture | boolean | true |
autoCaptureTimeout | number | 3000 // time in milliseconds |
maxNumberFrames | ?number | null |
strings | FaceStrings | |
styles | FaceStyles |
Variable name | Type | Default value |
---|---|---|
licenseKey | string | "" |
cameraPreset | FaceCameraPreset | FaceCameraPreset.MEDIUM |
defaultCameraPosition | FaceCameraLensDirection | FaceCameraLensDirection.FRONT |
outputFormat | FaceOutputFormat | FaceOutputFormat.JPEG |
captureButtonType | FaceCaptureButtonType | FaceCaptureButtonType.DEFAULT |
showFlashButton | boolean | false |
showFlipCameraButton | boolean | false |
flashEnabledByDefault | boolean | false |
showHelpText | boolean | true |
showScreenTitle | boolean | true |
autoCapture | boolean | true |
autoCaptureTimeout | number | 1000 // time in milliseconds |
maxNumberFrames | ?number | null |
strings | FaceStrings | |
styles | FaceStyles |
Variable name | Type | Default value |
---|---|---|
screenTitle | string | "Capturando Face" |
helpText | string | "Encaixe seu rosto no formato acima e aguarde o sinal verde" |
loading | string | "Processando..." |
customCaptureButtonText | string | "Capture Face" |
noFaceDetectedMessage | string | "Nenhuma face detectada" |
multipleFacesDetectedMessage | string | "Múltiplas faces detectadas" |
detectedFaceIsCenteredMessage | string | "Mantenha o celular parado" |
detectedFaceIsCloseMessage | string | "Afaste o rosto da câmera" |
detectedFaceIsDistantMessage | string | "Aproxime o rosto da câmera" |
detectedFaceIsOnTheLeftMessage | string | "Mova o celular para a direita" |
detectedFaceIsOnTheRightMessage | string | "Mova o celular para a esquerda" |
detectedFaceIsTooUpMessage | string | "Mova o celular para baixo" |
detectedFaceIsTooDownMessage | string | "Mova o celular para cima" |
Variable name | Type | Default value |
---|---|---|
faceShape | FaceScreenShape | FaceScreenShape.CUSTOM |
faceColor | string | "#FFFFFF" |
faceEnabledColor | string | "#16AC81" |
faceDisabledColor | string | "#E25353" |
overlayColor | string | "#CC000000" |
backButtonIcon | ?string | null |
backButtonColor | string | "#FFFFFF" |
backButtonSize | FaceSize | FaceSize(24, 24) |
flashButtonIcon | ?string | null |
flashButtonSize | FaceSize | FaceSize(24, 24) |
flashButtonEnabledColor | string | "#FFCC01" |
flashButtonDisabledColor | string | "#FFFFFF" |
flipCameraButtonIcon | ?string | null |
flipCameraButtonColor | string | "#FFFFFF" |
flipCameraButtonSize | FaceSize | FaceSize(65, 43) |
textColor | string | "#FFFFFF" |
textSize | number | 20 |
customFont | ?string | null |
captureButtonIcon | ?string | null |
captureButtonColor | string | "#D9D9D9" |
captureButtonSize | FaceSize | FaceSize(76, 76) |
customCaptureButtonBackgroundColor | string | "#D6A262" |
customCaptureButtonSize | FaceSize | FaceSize(300, 45) |
customCaptureButtonTextColor | string | "#FFFFFF" |
customCaptureButtonTextSize | number | 20 |
You can use the default font family or set a font you prefer. To set a font, create a folder font under res directory in your android/app/src/main/res
. Download the font which ever you want and paste it inside font folder. All font file names must be only: lowercase a-z, 0-9, or underscore. The structure should be some thing like below.
To add the font files to your Xcode project:
Then, add the "Fonts provided by application" key to your app’s Info.plist file. For the key’s value, provide an array of strings containing the relative paths to any added font files.
In the following example, the font file is inside the fonts directory, so you use fonts/roboto_mono_bold_italic.ttf as the string value in the Info.plist file.
Finally, just set the font passing the name of the font file when instantiating FaceConfig in your React Native app.
const config = FaceConfigPreset.getConfig(FaceConfigType.FACE_CAPTURE)
.setStylesFontFamily("roboto_mono_bold_italic");
You can use the default icon or set a icon you prefer. To set a icon, download the icon which ever you want and paste it inside drawable folder in your android/app/src/main/res
. All icon file names must be only: lowercase a-z, 0-9, or underscore. The structure should be some thing like below.
To add icon files to your Xcode project:
Finally, just set the icon passing the name of the icon file when instantiating FaceConfig in your React Native app.
const config = FaceConfigPreset.getConfig(FaceConfigType.FACE_CAPTURE)
// changing back button icon
.setStylesBackButtonIcon("ic_baseline_camera")
// changing flash button icon
.setStylesFlashButtonIcon("ic_baseline_camera")
// changing flip camera button icon
.setStylesFlipCameraButtonIcon("ic_baseline_camera")
// changing capture button icon
.setStylesCaptureButtonIcon("ic_baseline_camera");
Variable name | Type |
---|---|
width | number |
height | number |
Name | Resolution |
---|---|
FaceCameraPreset.LOW | 240p (352x288 on iOS, 320x240 on Android) |
FaceCameraPreset.MEDIUM | 480p (640x480 on iOS, 720x480 on Android) |
FaceCameraPreset.HIGH | 720p (1280x720) |
FaceCameraPreset.VERYHIGH | 1080p (1920x1080) |
FaceCameraPreset.ULTRAHIGH | 2160p (3840x2160) |
FaceCameraPreset.MAX | The highest resolution available |
Name |
---|
FaceCameraLensDirection.FRONT |
FaceCameraLensDirection.BACK |
Name |
---|
FaceCaptureFormat.JPEG |
FaceCaptureFormat.PNG |
Name |
---|
FaceCaptureButtonType.CUSTOM |
FaceCaptureButtonType.ICON |
Name |
---|
FaceScreenShape.SQUARE |
FaceScreenShape.ELLIPSE |
FaceScreenShape.CUSTOM |
const config = FaceConfigPreset.getConfig(FaceConfigType.FACE_CAPTURE)
.setCaptureButtonType(FaceCaptureButtonType.CUSTOM)
.setStylesCustomButtonBackgroundColor("#FFFF00")
.setStylesCustomButtonTextColor("#FF00FF")
.setStringsCustomButtonText("Capture");
const config = FaceConfigPreset.getConfig(FaceConfigType.FACE_CAPTURE)
.setCaptureButtonType(FaceCaptureButtonType.ICON)
.setStylesCaptureButtonIcon("ic_baseline_camera")
.setStylesCaptureButtonColor("#FF0000")
.setStylesCaptureButtonSize({width: 76, height: 76});
To instantiate it's easy as to do one function call (as we have seen previously on the example). You only need to specify which type of config you want using a ENUM FaceConfigType. Every type however can have different features implemented, here are the supported types:
Config Type | Enum | feature |
---|---|---|
Face Capture | FaceConfigType.FACE_CAPTURE | Capture still image and detects face |
Continuous Capture | FaceConfigType.CONTINUOUS_CAPTURE | Capture every frame per second |
On your Face callback function, you receive a FaceEvent.
Name | Type |
---|---|
photo | string // Base64 string |
This project is proprietary software. See the LICENSE for more information.
FAQs
BioPass ID Face React Native module.
The npm package @biopassid/face-sdk-react-native receives a total of 460 weekly downloads. As such, @biopassid/face-sdk-react-native popularity was classified as not popular.
We found that @biopassid/face-sdk-react-native demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.