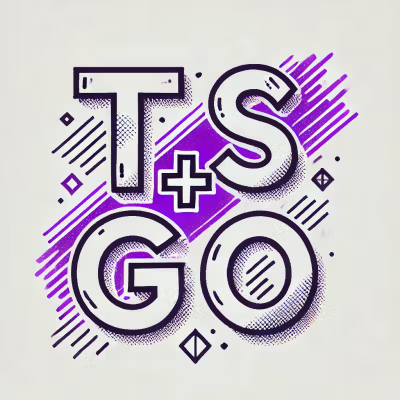
Security News
TypeScript is Porting Its Compiler to Go for 10x Faster Builds
TypeScript is porting its compiler to Go, delivering 10x faster builds, lower memory usage, and improved editor performance for a smoother developer experience.
@blnkfinance/blnk-typescript
Advanced tools
Ensure that you have the following installed on your machine:
To start, clone the Blnk repository from GitHub:
git clone https://github.com/blnkfinance/blnk && cd blnk
Install the Blnk TypeScript SDK in your project:
npm install @blnkfinance/blnk-typescript --save
In your cloned directory, create a configuration file named blnk.json
with the following content:
{
"project_name": "Blnk",
"data_source": {
"dns": "postgres://postgres:password@postgres:5432/blnk?sslmode=disable"
},
"redis": {
"dns": "redis:6379"
},
"server": {
"domain": "blnk.io",
"ssl": false,
"ssl_email": "jerryenebeli@gmail.com",
"port": "5001"
},
"notification": {
"slack": {
"webhook_url": "https://hooks.slack.com"
}
}
}
This configuration sets up connections to PostgreSQL and Redis, specifies your server details, and allows Slack notifications if needed.
With Docker Compose, launch the Blnk server:
docker compose up
Once running, your server will be accessible at http://localhost:5001.
The Blnk CLI offers quick access to manage ledgers, balances, and transactions. To verify the installation and view available commands, use:
blnk --help
In Blnk, ledgers are used to categorize balances for organized tracking. When you first install Blnk, an internal ledger called the General Ledger is created by default.
Using the SDK, create a ledger for user accounts:
import { BlnkInit } from '@blnkfinance/blnk-typescript';
const blnk = await BlnkInit('<secret_key_if_set>', { baseUrl: 'http://localhost:5001' });
const { Ledgers } = blnk;
const newLedger = await Ledgers.create({
name: "Customer Savings Account",
meta_data: {
project_owner: "YOUR_APP_NAME"
}
});
console.log("Ledger Created:", newLedger);
This creates a new ledger for storing customer balances.
Balances represent the store of value within a ledger, like a wallet or account. Each balance belongs to a ledger.
To create a balance, specify the ledger_id
and other details:
const { LedgerBalances } = blnk;
const newBalance = await LedgerBalances.create({
ledger_id: "ldg_073f7ffe-9dfd-42ce-aa50-d1dca1788adc",
currency: "USD",
meta_data: {
first_name: "Alice",
last_name: "Hart",
account_number: "1234567890"
}
});
console.log("Balance Created:", newBalance);
Transactions track financial activities within your application. Blnk ensures that each transaction is both immutable and idempotent.
To record a transaction, you’ll need the source
and destination
balance IDs:
const { Transactions } = blnk;
const newTransaction = await Transactions.create({
amount: 750,
reference: "ref_001adcfgf",
currency: "USD",
precision: 100,
source: "bln_28edb3e5-c168-4127-a1c4-16274e7a28d3",
destination: "bln_ebcd230f-6265-4d4a-a4ca-45974c47f746",
description: "Sent from app",
meta_data: {
sender_name: "John Doe",
sender_account: "00000000000"
}
});
console.log("Transaction Recorded:", newTransaction);
The Blnk CLI allows you to list all ledgers, balances, and transactions quickly:
blnk ledgers list
blnk balances list
blnk transactions list
For more examples and advanced use cases, please refer to the Examples Code.
If you encounter any issues, please report them on GitHub.
FAQs
Blnk Finance SDK in TypeScript
The npm package @blnkfinance/blnk-typescript receives a total of 1 weekly downloads. As such, @blnkfinance/blnk-typescript popularity was classified as not popular.
We found that @blnkfinance/blnk-typescript demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
TypeScript is porting its compiler to Go, delivering 10x faster builds, lower memory usage, and improved editor performance for a smoother developer experience.
Research
Security News
The Socket Research Team has discovered six new malicious npm packages linked to North Korea’s Lazarus Group, designed to steal credentials and deploy backdoors.
Security News
Socket CEO Feross Aboukhadijeh discusses the open web, open source security, and how Socket tackles software supply chain attacks on The Pair Program podcast.