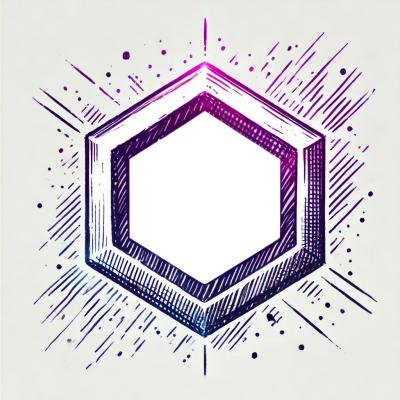
Security News
Maven Central Adds Sigstore Signature Validation
Maven Central now validates Sigstore signatures, making it easier for developers to verify the provenance of Java packages.
@bnk/backend-websocket-manager
Advanced tools
@bnk/backend-websocket-manager is a modular, extensible, and strongly-typed WebSocket manager for Bun-based servers, designed to handle a variety of real-time use cases with minimal overhead. This package leverages Bun’s native ServerWebSocket
, offering a customizable, pluggable architecture to manage application state and broadcast changes to connected clients.
BaseMessage
, MessageHandler
) to ensure type safety for state and message handling.getState
and setState
functions, allowing you to store and retrieve state from a DB, in-memory object, or any other storage.broadcastState()
.debug: true
in the configuration to enable verbose logging.getState
, setState
, or individual message handlers within Bun’s test suite.# Using Bun
bun add @bnk/backend-websocket-manager
# Or, if you are mixing with npm/yarn, you can also do:
npm install @bnk/backend-websocket-manager
# yarn add @bnk/backend-websocket-manager
Below is a minimal example of how to use @bnk/backend-websocket-manager. This example sets up an in-memory state and a single message handler for demonstration.
// my-app-state.ts
export interface MyAppState {
counter: number;
}
// my-message-types.ts
import { BaseMessage } from "@bnk/backend-websocket-manager";
export interface IncrementMessage extends BaseMessage {
type: "increment";
amount: number;
}
// Optionally combine multiple messages into a union
export type MyAppMessage = IncrementMessage;
// my-message-handlers.ts
import { MessageHandler } from "@bnk/backend-websocket-manager";
import { MyAppState, MyAppMessage } from "./my-message-types";
// A simple handler to increment a counter in the state
export const incrementHandler: MessageHandler<MyAppState, MyAppMessage> = {
type: "increment",
async handle(ws, message, getState, setState) {
const state = await getState();
state.counter += message.amount;
await setState(state);
},
};
export const myHandlers = [incrementHandler];
// backend-websocket-manager-setup.ts
import { WebSocketManager } from "@bnk/backend-websocket-manager";
import { MyAppState, MyAppMessage } from "./my-message-types";
import { myHandlers } from "./my-message-handlers";
// In-memory example state
let currentState: MyAppState = { counter: 0 };
const getState = async (): Promise<MyAppState> => {
// Return a clone to simulate immutable reads
return structuredClone(currentState);
};
const setState = async (newState: MyAppState): Promise<void> => {
// Simulate saving newState to a DB or in-memory store
currentState = structuredClone(newState);
};
// Create the manager
export const myWebSocketManager = new WebSocketManager<MyAppState, MyAppMessage>({
getState,
setState,
messageHandlers: myHandlers,
debug: true, // optional
});
// bun-server.ts
import { serve } from "bun";
import { myWebSocketManager } from "./backend-websocket-manager-setup";
serve({
port: 3000,
fetch(req: Request) {
return new Response("Hello from Bun server!", { status: 200 });
},
websocket: {
open(ws) {
myWebSocketManager.handleOpen(ws);
},
close(ws) {
myWebSocketManager.handleClose(ws);
},
async message(ws, msg) {
// Handle the incoming message
await myWebSocketManager.handleMessage(ws, msg.toString());
// Optionally broadcast updated state to all clients
await myWebSocketManager.broadcastState();
},
}
});
console.log("Server running at http://localhost:3000");
From the browser (or another WebSocket client), connect and send an increment
message:
const ws = new WebSocket("ws://localhost:3000");
ws.onopen = () => {
ws.send(JSON.stringify({ type: "increment", amount: 5 }));
};
ws.onmessage = (event) => {
console.log("Server says:", event.data);
};
You can define multiple handlers to deal with different message subtypes. Each handler is matched by its type
field.
In more complex applications, create separate modules for different domains (e.g., user chat, project management, etc.) and then combine all handlers into one array:
const allHandlers = [
...chatHandlers,
...projectHandlers,
// etc...
];
const manager = new WebSocketManager({
getState,
setState,
messageHandlers: allHandlers,
});
Whenever your state changes, you can call manager.broadcastState()
to push the updated state to all connected clients. Internally, this calls getState()
, serializes it, and sends it to each active WebSocket connection.
Set debug: true
in the manager config to see detailed logs of connections, closures, and message parsing. This is helpful for troubleshooting and development.
If you’re building a high-availability or production-grade system, you may also implement a heartbeat or ping/pong mechanism. This can help detect stale connections. See the test suite for an example of using a heartbeat interval.
@bnk/backend-websocket-manager is built for straightforward testing with Bun. Key points:
getState
and setState
for unit tests.getState
, setState
, and a mocked WebSocket.A sample test file is included in src/generic-backend-websocket-manager.test.ts
, showcasing how to verify:
To run tests:
bun test src/
Feel free to open issues or pull requests to improve @bnk/backend-websocket-manager. Whether it’s a feature request, bug fix, or documentation enhancement, all contributions are welcome.
This project is licensed under the MIT License.
@bnk/backend-websocket-manager aims to give you a solid foundation for real-time WebSocket applications using Bun, with minimal friction and maximum flexibility. If you find this library helpful, consider sharing feedback and improvements!
FAQs
Unknown package
The npm package @bnk/backend-websocket-manager receives a total of 0 weekly downloads. As such, @bnk/backend-websocket-manager popularity was classified as not popular.
We found that @bnk/backend-websocket-manager demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Maven Central now validates Sigstore signatures, making it easier for developers to verify the provenance of Java packages.
Security News
CISOs are racing to adopt AI for cybersecurity, but hurdles in budgets and governance may leave some falling behind in the fight against cyber threats.
Research
Security News
Socket researchers uncovered a backdoored typosquat of BoltDB in the Go ecosystem, exploiting Go Module Proxy caching to persist undetected for years.