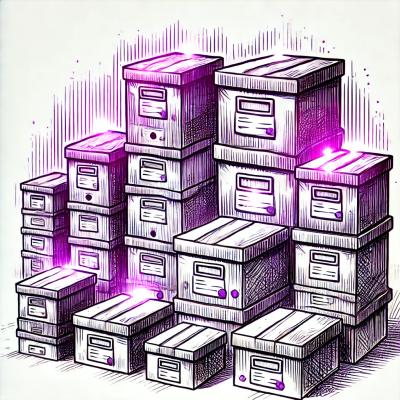
Security News
PyPI’s New Archival Feature Closes a Major Security Gap
PyPI now allows maintainers to archive projects, improving security and helping users make informed decisions about their dependencies.
@bolttech/atoms-breadcrumbs
Advanced tools
A React component to display a list of previous visited links.
A React component to display a list of previous visited links.
Use the package manager npm or yarn to install the component.
npm install @bolttech/atoms-breadcrumbs
or
yarn add @bolttech/atoms-breadcrumbs
The Breadcrumbs
component accepts the following properties:
Prop | Type | Description |
---|---|---|
id | string | The id of the breadcrumb container. |
dataTestId | string or element | The data-testid of the breadcrumbs container. |
item | BreadcrumbItem | The list of items of the breadcrumbs. |
The breadcrumb
component has the following behaviours:
import React from 'react';
import { Breadcrumbs } from '@bolttech/atoms-breadcrumbs';
import { bolttechTheme, BolttechThemeProvider } from '@bolttech/frontend-foundations';
const ExampleComponent = () => {
const items = [
{ label: 'Home', href: '/' },
{ label: 'About', href: '/about' },
{ label: 'Contact', href: '/contact' },
];
return (
<BolttechThemeProvider theme={bolttechTheme}>
<Breadcrumbs id="breadcrumbs" dataTestId="breadcrumbs" items={items} />
</BolttechThemeProvider>
);
};
export default ExampleComponent;
FAQs
A React component to display a list of previous visited links.
We found that @bolttech/atoms-breadcrumbs demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PyPI now allows maintainers to archive projects, improving security and helping users make informed decisions about their dependencies.
Research
Security News
Malicious npm package postcss-optimizer delivers BeaverTail malware, targeting developer systems; similarities to past campaigns suggest a North Korean connection.
Security News
CISA's KEV data is now on GitHub, offering easier access, API integration, commit history tracking, and automated updates for security teams and researchers.