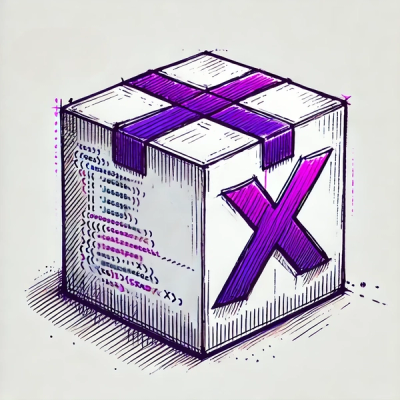
Security News
pnpm 10.0.0 Blocks Lifecycle Scripts by Default
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.
@botpress/client
Advanced tools
Official Botpress HTTP client for TypeScript. Queries the Botpress API.
npm install --save @botpress/client # for npm
yarn add @botpress/client # for yarn
pnpm add @botpress/client # for pnpm
import { Client } from '@botpress/client'
// 0. Type definitions for each operation's IO
type GetBotInput = ClientInputs['getBot']
type GetBotOutput = ClientOutputs['getBot']
const main = async () => {
const token = 'your-token'
const workspaceId = 'your-workspace-id'
const botId = 'your-bot-id'
const client = new Client({ token, workspaceId, botId })
// 1. plain operations
const { bot } = await client.getBot({ id: botId })
console.log('### bot', bot)
// 2. list utils with `.collect()` function
const [latestConversation] = await client.list
.conversations({ sortField: 'createdAt', sortDirection: 'desc', integrationName: 'telegram' })
.collect({ limit: 1 })
console.log('### latestConversation', latestConversation)
// 3. list utils with async generator and `for await` syntax
for await (const message of client.list.messages({ conversationId: latestConversation.id })) {
console.log(`### [${message.userId}]`, message.payload)
}
}
void main()
FAQs
Botpress Client
The npm package @botpress/client receives a total of 1,035 weekly downloads. As such, @botpress/client popularity was classified as popular.
We found that @botpress/client demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.
Product
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
Research
Security News
Socket researchers have discovered multiple malicious npm packages targeting Solana private keys, abusing Gmail to exfiltrate the data and drain Solana wallets.