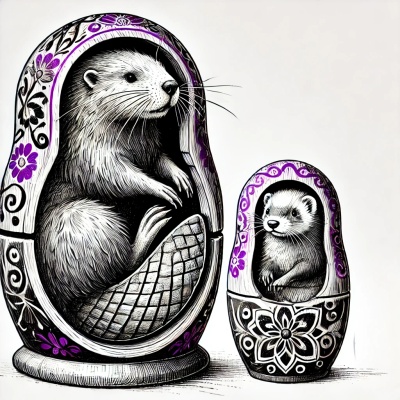
Research
Another Wave: North Korean Contagious Interview Campaign Drops 35 New Malicious npm Packages
North Korean threat actors linked to the Contagious Interview campaign return with 35 new malicious npm packages using a stealthy multi-stage malware loader.