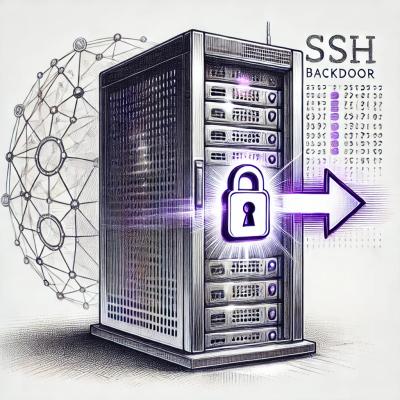
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
@burstjs/core
Advanced tools
Burst-related functions and models for building Burstcoin applications.
Burst-related functions and models for building Burstcoin applications.
burstJS can be used with NodeJS or Web. Two formats are available
Install using npm:
npm install @burstjs/core
or using yarn:
yarn add @burstjs/core
import {composeApi, ApiSettings} from '@burstjs/core'
import {convertNQTStringToNumber} from '@burstjs/util'
const apiSettings = new ApiSettings('http://at-testnet.burst-alliance.org:6876', 'burst');
const api = composeApi(apiSettings);
// this self-executing file makes turns this file into a starting point of your app
(async () => {
try{
const {balanceNQT} = await api.account.getAccountBalance('13036514135565182944')
console.log(`Account Balance: ${convertNQTStringToNumber(balanceNQT)} BURST`)
}
catch(e){ // e is of type HttpError (as part of @burstjs/http)
console.error(`Whooops, something went wrong: ${e.message}`)
}
})()
<script>
Each package is available as bundled standalone library using IIFE.
This way burstJS can be used also within <script>
-Tags.
This might be useful for Wordpress and/or other PHP applications.
Just import the package using the HTML <script>
tag.
<script src='https://cdn.jsdelivr.net/npm/@burstjs/core/dist/burstjs.min.js'></script>
(function(){
const api = b$.composeApi({
nodeHost: "http://at-testnet.burst-alliance.org:6876",
apiRootUrl: "/burst"
});
api.network.getBlockchainStatus().then(console.log).catch(console.error);
})()
See more here:
@burstjs/core Online Documentation
Settings for API used in [[composeApi]]
Helper class for contracts
A contract owns additional data, which is splitted in 8 byte blocks. The content is encoded in hexadecimal representation and big endianness. This helper class facilitates access to these data
Generic BRS Web Service class.
Composes the API, i.e. setup the environment and mounts the API structure with its functions.
const api = composeApi({
nodeHost: 'https://wallet1.burst-team.us:2083', // one of the mainnet nodes
})
Note, that this method mounts the entire API, i.e. all available methods. One may also customize the API composition using [[ApiComposer]].
Creates BRS Http send parameters for a transaction from attachment data
Copyright (c) 2019 Burst Apps Team
Kind: inner class of api
The API composer mounts the API for given service and selected methods
Usually you would use [[composeApi]], which gives you all available API methods. Unfortunately, this will import almost all dependencies, even if you need only a fraction of the methods. To take advantage of tree-shaking (dead code elimination) you can compose your own API with the methods you need. This can reduce your final bundle significantly.
Usage:
const burstService = new BurstService({ nodeHost: 'https://testnet.burst.fun', })
const api = apiComposer .create(burstService) .withMessageApi({ sendTextMessage }) .withAccountApi({ getAccountTransactions, getUnconfirmedAccountTransactions, getAccountBalance, generateSendTransactionQRCode, generateSendTransactionQRCodeAddress, }) .compose();
The with<section>Api
uses factory methods from the api.core.factories package
Kind: inner class of api
Adds the [[BlockApi]] to be composed
Kind: instance method of ApiComposer
Param | Description |
---|---|
creatorMap | A map of creator/factory functions for the endpoints |
Adds the [[AccountApi]] to be composed
Kind: instance method of ApiComposer
Param | Description |
---|---|
creatorMap | A map of creator/factory functions for the endpoints |
Adds the [[NetworkApi]] to be composed
Kind: instance method of ApiComposer
Param | Description |
---|---|
creatorMap | A map of creator/factory functions for the endpoints |
Adds the [[MessageApi]] to be composed
Kind: instance method of ApiComposer
Param | Description |
---|---|
creatorMap | A map of creator/factory functions for the endpoints |
Adds the [[TransactionApi]] to be composed
Kind: instance method of ApiComposer
Param | Description |
---|---|
creatorMap | A map of creator/factory functions for the endpoints |
Adds the [[AliasApi]] to be composed
Kind: instance method of ApiComposer
Param | Description |
---|---|
creatorMap | A map of creator/factory functions for the endpoints |
Adds the [[AssetApi]] to be composed
Kind: instance method of ApiComposer
Param | Description |
---|---|
creatorMap | A map of creator/factory functions for the endpoints |
Adds the [[ContractApi]] to be composed
Kind: instance method of ApiComposer
Param | Description |
---|---|
creatorMap | A map of creator/factory functions for the endpoints |
Composes the API Note: As of being a builder pattern, this need to call this method as last
Kind: instance method of ApiComposer
Creates the composer instance
Kind: static method of ApiComposer
Returns:
the composer instance
Param |
---|
service |
The default deadline (in minutes) for Transactions
Kind: static property of core
The default endpoint for [[ApiSettings]]
Kind: static property of core
Message class
The Message class is used to model a plain message attached to a transaction.
Kind: static property of core
EncryptedMessage class
The EncryptedMessage class is a model for a encrypted message attached to a transaction.
Kind: static property of core
Account class
The account class serves as a model for a Burstcoin account. It's meant to model the response from BRS API, except publicKey has been moved into the keys object.
Kind: inner class of core
Attachment class
The attachment class is used to appended to transaction where appropriate. It is a super class for Message and EncryptedMessage.
Kind: inner class of core
Original work Copyright (c) 2018 PoC-Consortium Modified work Copyright (c) 2019 Burst Apps Team
Kind: inner class of core
Constants for arbitrary subtypes
Kind: inner property of core
Constants for asset subtypes
Kind: inner property of core
Constants for escrow subtypes
Kind: inner property of core
Constants for leasing subtypes
Kind: inner property of core
Constants for marketplace subtypes
Kind: inner property of core
Constants for payment subtypes
Kind: inner property of core
Constants for reward recipient subtypes (Pool Operation)
Kind: inner property of core
Constants for smart contract (aka AT) subtypes
Kind: inner property of core
Constants for transaction types
The transaction type is part of every [[Transaction]] object and used to distinguish block data. Additionally, to the transaction type a subtype is sent, that specifies the kind of transaction more detailly.
Kind: inner property of core
The smallest possible fee
Kind: inner constant of core
Get the transaction attachment version identifier
Attachment types are identified by a field version.
Kind: inner method of core
Returns:
return Identifier, if exists, otherwise undefined
Param | Description |
---|---|
transaction | The transaction to be checked |
Checks if a transaction attachment is of specific version
Kind: inner method of core
Returns:
true, if version string matches
Param | Description |
---|---|
transaction | The transaction to be checked |
versionIdentifier | The version string, i.e. MultiOutCreation |
Extracts a variables value as hexadecimal string from a contract's machine data
This is a generic function to extract arbitrary data from a contract. I's recommended to use the [[ContractHelper]] class instead
Kind: inner method of core
Returns:
The value as hexadecimal string (already considering endianness)
Param | Default | Description |
---|---|---|
position | The variables position | |
length | 16 | The length of data to be extracted |
Deprecated
Kind: inner method of core
Returns:
HttpParams
Param | Description |
---|---|
transaction | The transaction with the attachment |
params | Some HttpParams |
Signs and broadcasts a transaction
Kind: inner method of core
Returns:
The transaction Id
Param | Description |
---|---|
unsignedTransaction | The unsigned transaction context |
service | The service used for |
Tries to extract recipients and its amounts for multi out payments (different and same amount)
Kind: inner method of core
Returns:
A list of recipients and their payed amount (in NQT)
An exception in case of wrong transaction types
Param | Description |
---|---|
transaction | The transaction |
Gets the amount from a transaction, considering ordinary and multi out transactions (with same and different payments)
Kind: inner method of core
Returns:
the amount in BURST (not NQT)
Param | Description |
---|---|
recipientId | The numeric id of the recipient |
transaction | The payment transaction |
Checks if a transaction is a multi out transaction with same amounts for each recipient
Kind: inner method of core
Returns:
true, if is a multi out transaction
Param | Description |
---|---|
transaction | Transaction to be checked |
Checks if a transaction is a multi out transaction (with different amounts)
Kind: inner method of core
Returns:
true, if is a multi out transaction
Param | Description |
---|---|
transaction | Transaction to be checked |
The default deadline (in minutes) for Transactions
Kind: static property of core
The default endpoint for [[ApiSettings]]
Kind: static property of core
Message class
The Message class is used to model a plain message attached to a transaction.
Kind: static property of core
EncryptedMessage class
The EncryptedMessage class is a model for a encrypted message attached to a transaction.
Kind: static property of core
Account class
The account class serves as a model for a Burstcoin account. It's meant to model the response from BRS API, except publicKey has been moved into the keys object.
Kind: inner class of core
Attachment class
The attachment class is used to appended to transaction where appropriate. It is a super class for Message and EncryptedMessage.
Kind: inner class of core
Original work Copyright (c) 2018 PoC-Consortium Modified work Copyright (c) 2019 Burst Apps Team
Kind: inner class of core
Constants for arbitrary subtypes
Kind: inner property of core
Constants for asset subtypes
Kind: inner property of core
Constants for escrow subtypes
Kind: inner property of core
Constants for leasing subtypes
Kind: inner property of core
Constants for marketplace subtypes
Kind: inner property of core
Constants for payment subtypes
Kind: inner property of core
Constants for reward recipient subtypes (Pool Operation)
Kind: inner property of core
Constants for smart contract (aka AT) subtypes
Kind: inner property of core
Constants for transaction types
The transaction type is part of every [[Transaction]] object and used to distinguish block data. Additionally, to the transaction type a subtype is sent, that specifies the kind of transaction more detailly.
Kind: inner property of core
The smallest possible fee
Kind: inner constant of core
Get the transaction attachment version identifier
Attachment types are identified by a field version.
Kind: inner method of core
Returns:
return Identifier, if exists, otherwise undefined
Param | Description |
---|---|
transaction | The transaction to be checked |
Checks if a transaction attachment is of specific version
Kind: inner method of core
Returns:
true, if version string matches
Param | Description |
---|---|
transaction | The transaction to be checked |
versionIdentifier | The version string, i.e. MultiOutCreation |
Extracts a variables value as hexadecimal string from a contract's machine data
This is a generic function to extract arbitrary data from a contract. I's recommended to use the [[ContractHelper]] class instead
Kind: inner method of core
Returns:
The value as hexadecimal string (already considering endianness)
Param | Default | Description |
---|---|---|
position | The variables position | |
length | 16 | The length of data to be extracted |
Deprecated
Kind: inner method of core
Returns:
HttpParams
Param | Description |
---|---|
transaction | The transaction with the attachment |
params | Some HttpParams |
Signs and broadcasts a transaction
Kind: inner method of core
Returns:
The transaction Id
Param | Description |
---|---|
unsignedTransaction | The unsigned transaction context |
service | The service used for |
Tries to extract recipients and its amounts for multi out payments (different and same amount)
Kind: inner method of core
Returns:
A list of recipients and their payed amount (in NQT)
An exception in case of wrong transaction types
Param | Description |
---|---|
transaction | The transaction |
Gets the amount from a transaction, considering ordinary and multi out transactions (with same and different payments)
Kind: inner method of core
Returns:
the amount in BURST (not NQT)
Param | Description |
---|---|
recipientId | The numeric id of the recipient |
transaction | The payment transaction |
Checks if a transaction is a multi out transaction with same amounts for each recipient
Kind: inner method of core
Returns:
true, if is a multi out transaction
Param | Description |
---|---|
transaction | Transaction to be checked |
Checks if a transaction is a multi out transaction (with different amounts)
Kind: inner method of core
Returns:
true, if is a multi out transaction
Param | Description |
---|---|
transaction | Transaction to be checked |
The default deadline (in minutes) for Transactions
Kind: static property of core
The default endpoint for [[ApiSettings]]
Kind: static property of core
Message class
The Message class is used to model a plain message attached to a transaction.
Kind: static property of core
EncryptedMessage class
The EncryptedMessage class is a model for a encrypted message attached to a transaction.
Kind: static property of core
Account class
The account class serves as a model for a Burstcoin account. It's meant to model the response from BRS API, except publicKey has been moved into the keys object.
Kind: inner class of core
Attachment class
The attachment class is used to appended to transaction where appropriate. It is a super class for Message and EncryptedMessage.
Kind: inner class of core
Original work Copyright (c) 2018 PoC-Consortium Modified work Copyright (c) 2019 Burst Apps Team
Kind: inner class of core
Constants for arbitrary subtypes
Kind: inner property of core
Constants for asset subtypes
Kind: inner property of core
Constants for escrow subtypes
Kind: inner property of core
Constants for leasing subtypes
Kind: inner property of core
Constants for marketplace subtypes
Kind: inner property of core
Constants for payment subtypes
Kind: inner property of core
Constants for reward recipient subtypes (Pool Operation)
Kind: inner property of core
Constants for smart contract (aka AT) subtypes
Kind: inner property of core
Constants for transaction types
The transaction type is part of every [[Transaction]] object and used to distinguish block data. Additionally, to the transaction type a subtype is sent, that specifies the kind of transaction more detailly.
Kind: inner property of core
The smallest possible fee
Kind: inner constant of core
Get the transaction attachment version identifier
Attachment types are identified by a field version.
Kind: inner method of core
Returns:
return Identifier, if exists, otherwise undefined
Param | Description |
---|---|
transaction | The transaction to be checked |
Checks if a transaction attachment is of specific version
Kind: inner method of core
Returns:
true, if version string matches
Param | Description |
---|---|
transaction | The transaction to be checked |
versionIdentifier | The version string, i.e. MultiOutCreation |
Extracts a variables value as hexadecimal string from a contract's machine data
This is a generic function to extract arbitrary data from a contract. I's recommended to use the [[ContractHelper]] class instead
Kind: inner method of core
Returns:
The value as hexadecimal string (already considering endianness)
Param | Default | Description |
---|---|---|
position | The variables position | |
length | 16 | The length of data to be extracted |
Deprecated
Kind: inner method of core
Returns:
HttpParams
Param | Description |
---|---|
transaction | The transaction with the attachment |
params | Some HttpParams |
Signs and broadcasts a transaction
Kind: inner method of core
Returns:
The transaction Id
Param | Description |
---|---|
unsignedTransaction | The unsigned transaction context |
service | The service used for |
Tries to extract recipients and its amounts for multi out payments (different and same amount)
Kind: inner method of core
Returns:
A list of recipients and their payed amount (in NQT)
An exception in case of wrong transaction types
Param | Description |
---|---|
transaction | The transaction |
Gets the amount from a transaction, considering ordinary and multi out transactions (with same and different payments)
Kind: inner method of core
Returns:
the amount in BURST (not NQT)
Param | Description |
---|---|
recipientId | The numeric id of the recipient |
transaction | The payment transaction |
Checks if a transaction is a multi out transaction with same amounts for each recipient
Kind: inner method of core
Returns:
true, if is a multi out transaction
Param | Description |
---|---|
transaction | Transaction to be checked |
Checks if a transaction is a multi out transaction (with different amounts)
Kind: inner method of core
Returns:
true, if is a multi out transaction
Param | Description |
---|---|
transaction | Transaction to be checked |
The default deadline (in minutes) for Transactions
Kind: static property of core
The default endpoint for [[ApiSettings]]
Kind: static property of core
Message class
The Message class is used to model a plain message attached to a transaction.
Kind: static property of core
EncryptedMessage class
The EncryptedMessage class is a model for a encrypted message attached to a transaction.
Kind: static property of core
Account class
The account class serves as a model for a Burstcoin account. It's meant to model the response from BRS API, except publicKey has been moved into the keys object.
Kind: inner class of core
Attachment class
The attachment class is used to appended to transaction where appropriate. It is a super class for Message and EncryptedMessage.
Kind: inner class of core
Original work Copyright (c) 2018 PoC-Consortium Modified work Copyright (c) 2019 Burst Apps Team
Kind: inner class of core
Constants for arbitrary subtypes
Kind: inner property of core
Constants for asset subtypes
Kind: inner property of core
Constants for escrow subtypes
Kind: inner property of core
Constants for leasing subtypes
Kind: inner property of core
Constants for marketplace subtypes
Kind: inner property of core
Constants for payment subtypes
Kind: inner property of core
Constants for reward recipient subtypes (Pool Operation)
Kind: inner property of core
Constants for smart contract (aka AT) subtypes
Kind: inner property of core
Constants for transaction types
The transaction type is part of every [[Transaction]] object and used to distinguish block data. Additionally, to the transaction type a subtype is sent, that specifies the kind of transaction more detailly.
Kind: inner property of core
The smallest possible fee
Kind: inner constant of core
Get the transaction attachment version identifier
Attachment types are identified by a field version.
Kind: inner method of core
Returns:
return Identifier, if exists, otherwise undefined
Param | Description |
---|---|
transaction | The transaction to be checked |
Checks if a transaction attachment is of specific version
Kind: inner method of core
Returns:
true, if version string matches
Param | Description |
---|---|
transaction | The transaction to be checked |
versionIdentifier | The version string, i.e. MultiOutCreation |
Extracts a variables value as hexadecimal string from a contract's machine data
This is a generic function to extract arbitrary data from a contract. I's recommended to use the [[ContractHelper]] class instead
Kind: inner method of core
Returns:
The value as hexadecimal string (already considering endianness)
Param | Default | Description |
---|---|---|
position | The variables position | |
length | 16 | The length of data to be extracted |
Deprecated
Kind: inner method of core
Returns:
HttpParams
Param | Description |
---|---|
transaction | The transaction with the attachment |
params | Some HttpParams |
Signs and broadcasts a transaction
Kind: inner method of core
Returns:
The transaction Id
Param | Description |
---|---|
unsignedTransaction | The unsigned transaction context |
service | The service used for |
Tries to extract recipients and its amounts for multi out payments (different and same amount)
Kind: inner method of core
Returns:
A list of recipients and their payed amount (in NQT)
An exception in case of wrong transaction types
Param | Description |
---|---|
transaction | The transaction |
Gets the amount from a transaction, considering ordinary and multi out transactions (with same and different payments)
Kind: inner method of core
Returns:
the amount in BURST (not NQT)
Param | Description |
---|---|
recipientId | The numeric id of the recipient |
transaction | The payment transaction |
Checks if a transaction is a multi out transaction with same amounts for each recipient
Kind: inner method of core
Returns:
true, if is a multi out transaction
Param | Description |
---|---|
transaction | Transaction to be checked |
Checks if a transaction is a multi out transaction (with different amounts)
Kind: inner method of core
Returns:
true, if is a multi out transaction
Param | Description |
---|---|
transaction | Transaction to be checked |
The default deadline (in minutes) for Transactions
Kind: static property of core
The default endpoint for [[ApiSettings]]
Kind: static property of core
Message class
The Message class is used to model a plain message attached to a transaction.
Kind: static property of core
EncryptedMessage class
The EncryptedMessage class is a model for a encrypted message attached to a transaction.
Kind: static property of core
Account class
The account class serves as a model for a Burstcoin account. It's meant to model the response from BRS API, except publicKey has been moved into the keys object.
Kind: inner class of core
Attachment class
The attachment class is used to appended to transaction where appropriate. It is a super class for Message and EncryptedMessage.
Kind: inner class of core
Original work Copyright (c) 2018 PoC-Consortium Modified work Copyright (c) 2019 Burst Apps Team
Kind: inner class of core
Constants for arbitrary subtypes
Kind: inner property of core
Constants for asset subtypes
Kind: inner property of core
Constants for escrow subtypes
Kind: inner property of core
Constants for leasing subtypes
Kind: inner property of core
Constants for marketplace subtypes
Kind: inner property of core
Constants for payment subtypes
Kind: inner property of core
Constants for reward recipient subtypes (Pool Operation)
Kind: inner property of core
Constants for smart contract (aka AT) subtypes
Kind: inner property of core
Constants for transaction types
The transaction type is part of every [[Transaction]] object and used to distinguish block data. Additionally, to the transaction type a subtype is sent, that specifies the kind of transaction more detailly.
Kind: inner property of core
The smallest possible fee
Kind: inner constant of core
Get the transaction attachment version identifier
Attachment types are identified by a field version.
Kind: inner method of core
Returns:
return Identifier, if exists, otherwise undefined
Param | Description |
---|---|
transaction | The transaction to be checked |
Checks if a transaction attachment is of specific version
Kind: inner method of core
Returns:
true, if version string matches
Param | Description |
---|---|
transaction | The transaction to be checked |
versionIdentifier | The version string, i.e. MultiOutCreation |
Extracts a variables value as hexadecimal string from a contract's machine data
This is a generic function to extract arbitrary data from a contract. I's recommended to use the [[ContractHelper]] class instead
Kind: inner method of core
Returns:
The value as hexadecimal string (already considering endianness)
Param | Default | Description |
---|---|---|
position | The variables position | |
length | 16 | The length of data to be extracted |
Deprecated
Kind: inner method of core
Returns:
HttpParams
Param | Description |
---|---|
transaction | The transaction with the attachment |
params | Some HttpParams |
Signs and broadcasts a transaction
Kind: inner method of core
Returns:
The transaction Id
Param | Description |
---|---|
unsignedTransaction | The unsigned transaction context |
service | The service used for |
Tries to extract recipients and its amounts for multi out payments (different and same amount)
Kind: inner method of core
Returns:
A list of recipients and their payed amount (in NQT)
An exception in case of wrong transaction types
Param | Description |
---|---|
transaction | The transaction |
Gets the amount from a transaction, considering ordinary and multi out transactions (with same and different payments)
Kind: inner method of core
Returns:
the amount in BURST (not NQT)
Param | Description |
---|---|
recipientId | The numeric id of the recipient |
transaction | The payment transaction |
Checks if a transaction is a multi out transaction with same amounts for each recipient
Kind: inner method of core
Returns:
true, if is a multi out transaction
Param | Description |
---|---|
transaction | Transaction to be checked |
Checks if a transaction is a multi out transaction (with different amounts)
Kind: inner method of core
Returns:
true, if is a multi out transaction
Param | Description |
---|---|
transaction | Transaction to be checked |
The default deadline (in minutes) for Transactions
Kind: static property of core
The default endpoint for [[ApiSettings]]
Kind: static property of core
Message class
The Message class is used to model a plain message attached to a transaction.
Kind: static property of core
EncryptedMessage class
The EncryptedMessage class is a model for a encrypted message attached to a transaction.
Kind: static property of core
Account class
The account class serves as a model for a Burstcoin account. It's meant to model the response from BRS API, except publicKey has been moved into the keys object.
Kind: inner class of core
Attachment class
The attachment class is used to appended to transaction where appropriate. It is a super class for Message and EncryptedMessage.
Kind: inner class of core
Original work Copyright (c) 2018 PoC-Consortium Modified work Copyright (c) 2019 Burst Apps Team
Kind: inner class of core
Constants for arbitrary subtypes
Kind: inner property of core
Constants for asset subtypes
Kind: inner property of core
Constants for escrow subtypes
Kind: inner property of core
Constants for leasing subtypes
Kind: inner property of core
Constants for marketplace subtypes
Kind: inner property of core
Constants for payment subtypes
Kind: inner property of core
Constants for reward recipient subtypes (Pool Operation)
Kind: inner property of core
Constants for smart contract (aka AT) subtypes
Kind: inner property of core
Constants for transaction types
The transaction type is part of every [[Transaction]] object and used to distinguish block data. Additionally, to the transaction type a subtype is sent, that specifies the kind of transaction more detailly.
Kind: inner property of core
The smallest possible fee
Kind: inner constant of core
Get the transaction attachment version identifier
Attachment types are identified by a field version.
Kind: inner method of core
Returns:
return Identifier, if exists, otherwise undefined
Param | Description |
---|---|
transaction | The transaction to be checked |
Checks if a transaction attachment is of specific version
Kind: inner method of core
Returns:
true, if version string matches
Param | Description |
---|---|
transaction | The transaction to be checked |
versionIdentifier | The version string, i.e. MultiOutCreation |
Extracts a variables value as hexadecimal string from a contract's machine data
This is a generic function to extract arbitrary data from a contract. I's recommended to use the [[ContractHelper]] class instead
Kind: inner method of core
Returns:
The value as hexadecimal string (already considering endianness)
Param | Default | Description |
---|---|---|
position | The variables position | |
length | 16 | The length of data to be extracted |
Deprecated
Kind: inner method of core
Returns:
HttpParams
Param | Description |
---|---|
transaction | The transaction with the attachment |
params | Some HttpParams |
Signs and broadcasts a transaction
Kind: inner method of core
Returns:
The transaction Id
Param | Description |
---|---|
unsignedTransaction | The unsigned transaction context |
service | The service used for |
Tries to extract recipients and its amounts for multi out payments (different and same amount)
Kind: inner method of core
Returns:
A list of recipients and their payed amount (in NQT)
An exception in case of wrong transaction types
Param | Description |
---|---|
transaction | The transaction |
Gets the amount from a transaction, considering ordinary and multi out transactions (with same and different payments)
Kind: inner method of core
Returns:
the amount in BURST (not NQT)
Param | Description |
---|---|
recipientId | The numeric id of the recipient |
transaction | The payment transaction |
Checks if a transaction is a multi out transaction with same amounts for each recipient
Kind: inner method of core
Returns:
true, if is a multi out transaction
Param | Description |
---|---|
transaction | Transaction to be checked |
Checks if a transaction is a multi out transaction (with different amounts)
Kind: inner method of core
Returns:
true, if is a multi out transaction
Param | Description |
---|---|
transaction | Transaction to be checked |
The default deadline (in minutes) for Transactions
Kind: static property of core
The default endpoint for [[ApiSettings]]
Kind: static property of core
Message class
The Message class is used to model a plain message attached to a transaction.
Kind: static property of core
EncryptedMessage class
The EncryptedMessage class is a model for a encrypted message attached to a transaction.
Kind: static property of core
Account class
The account class serves as a model for a Burstcoin account. It's meant to model the response from BRS API, except publicKey has been moved into the keys object.
Kind: inner class of core
Attachment class
The attachment class is used to appended to transaction where appropriate. It is a super class for Message and EncryptedMessage.
Kind: inner class of core
Original work Copyright (c) 2018 PoC-Consortium Modified work Copyright (c) 2019 Burst Apps Team
Kind: inner class of core
Constants for arbitrary subtypes
Kind: inner property of core
Constants for asset subtypes
Kind: inner property of core
Constants for escrow subtypes
Kind: inner property of core
Constants for leasing subtypes
Kind: inner property of core
Constants for marketplace subtypes
Kind: inner property of core
Constants for payment subtypes
Kind: inner property of core
Constants for reward recipient subtypes (Pool Operation)
Kind: inner property of core
Constants for smart contract (aka AT) subtypes
Kind: inner property of core
Constants for transaction types
The transaction type is part of every [[Transaction]] object and used to distinguish block data. Additionally, to the transaction type a subtype is sent, that specifies the kind of transaction more detailly.
Kind: inner property of core
The smallest possible fee
Kind: inner constant of core
Get the transaction attachment version identifier
Attachment types are identified by a field version.
Kind: inner method of core
Returns:
return Identifier, if exists, otherwise undefined
Param | Description |
---|---|
transaction | The transaction to be checked |
Checks if a transaction attachment is of specific version
Kind: inner method of core
Returns:
true, if version string matches
Param | Description |
---|---|
transaction | The transaction to be checked |
versionIdentifier | The version string, i.e. MultiOutCreation |
Extracts a variables value as hexadecimal string from a contract's machine data
This is a generic function to extract arbitrary data from a contract. I's recommended to use the [[ContractHelper]] class instead
Kind: inner method of core
Returns:
The value as hexadecimal string (already considering endianness)
Param | Default | Description |
---|---|---|
position | The variables position | |
length | 16 | The length of data to be extracted |
Deprecated
Kind: inner method of core
Returns:
HttpParams
Param | Description |
---|---|
transaction | The transaction with the attachment |
params | Some HttpParams |
Signs and broadcasts a transaction
Kind: inner method of core
Returns:
The transaction Id
Param | Description |
---|---|
unsignedTransaction | The unsigned transaction context |
service | The service used for |
Tries to extract recipients and its amounts for multi out payments (different and same amount)
Kind: inner method of core
Returns:
A list of recipients and their payed amount (in NQT)
An exception in case of wrong transaction types
Param | Description |
---|---|
transaction | The transaction |
Gets the amount from a transaction, considering ordinary and multi out transactions (with same and different payments)
Kind: inner method of core
Returns:
the amount in BURST (not NQT)
Param | Description |
---|---|
recipientId | The numeric id of the recipient |
transaction | The payment transaction |
Checks if a transaction is a multi out transaction with same amounts for each recipient
Kind: inner method of core
Returns:
true, if is a multi out transaction
Param | Description |
---|---|
transaction | Transaction to be checked |
Checks if a transaction is a multi out transaction (with different amounts)
Kind: inner method of core
Returns:
true, if is a multi out transaction
Param | Description |
---|---|
transaction | Transaction to be checked |
The default deadline (in minutes) for Transactions
Kind: static property of core
The default endpoint for [[ApiSettings]]
Kind: static property of core
Message class
The Message class is used to model a plain message attached to a transaction.
Kind: static property of core
EncryptedMessage class
The EncryptedMessage class is a model for a encrypted message attached to a transaction.
Kind: static property of core
Account class
The account class serves as a model for a Burstcoin account. It's meant to model the response from BRS API, except publicKey has been moved into the keys object.
Kind: inner class of core
Attachment class
The attachment class is used to appended to transaction where appropriate. It is a super class for Message and EncryptedMessage.
Kind: inner class of core
Original work Copyright (c) 2018 PoC-Consortium Modified work Copyright (c) 2019 Burst Apps Team
Kind: inner class of core
Constants for arbitrary subtypes
Kind: inner property of core
Constants for asset subtypes
Kind: inner property of core
Constants for escrow subtypes
Kind: inner property of core
Constants for leasing subtypes
Kind: inner property of core
Constants for marketplace subtypes
Kind: inner property of core
Constants for payment subtypes
Kind: inner property of core
Constants for reward recipient subtypes (Pool Operation)
Kind: inner property of core
Constants for smart contract (aka AT) subtypes
Kind: inner property of core
Constants for transaction types
The transaction type is part of every [[Transaction]] object and used to distinguish block data. Additionally, to the transaction type a subtype is sent, that specifies the kind of transaction more detailly.
Kind: inner property of core
The smallest possible fee
Kind: inner constant of core
Get the transaction attachment version identifier
Attachment types are identified by a field version.
Kind: inner method of core
Returns:
return Identifier, if exists, otherwise undefined
Param | Description |
---|---|
transaction | The transaction to be checked |
Checks if a transaction attachment is of specific version
Kind: inner method of core
Returns:
true, if version string matches
Param | Description |
---|---|
transaction | The transaction to be checked |
versionIdentifier | The version string, i.e. MultiOutCreation |
Extracts a variables value as hexadecimal string from a contract's machine data
This is a generic function to extract arbitrary data from a contract. I's recommended to use the [[ContractHelper]] class instead
Kind: inner method of core
Returns:
The value as hexadecimal string (already considering endianness)
Param | Default | Description |
---|---|---|
position | The variables position | |
length | 16 | The length of data to be extracted |
Deprecated
Kind: inner method of core
Returns:
HttpParams
Param | Description |
---|---|
transaction | The transaction with the attachment |
params | Some HttpParams |
Signs and broadcasts a transaction
Kind: inner method of core
Returns:
The transaction Id
Param | Description |
---|---|
unsignedTransaction | The unsigned transaction context |
service | The service used for |
Tries to extract recipients and its amounts for multi out payments (different and same amount)
Kind: inner method of core
Returns:
A list of recipients and their payed amount (in NQT)
An exception in case of wrong transaction types
Param | Description |
---|---|
transaction | The transaction |
Gets the amount from a transaction, considering ordinary and multi out transactions (with same and different payments)
Kind: inner method of core
Returns:
the amount in BURST (not NQT)
Param | Description |
---|---|
recipientId | The numeric id of the recipient |
transaction | The payment transaction |
Checks if a transaction is a multi out transaction with same amounts for each recipient
Kind: inner method of core
Returns:
true, if is a multi out transaction
Param | Description |
---|---|
transaction | Transaction to be checked |
Checks if a transaction is a multi out transaction (with different amounts)
Kind: inner method of core
Returns:
true, if is a multi out transaction
Param | Description |
---|---|
transaction | Transaction to be checked |
The default deadline (in minutes) for Transactions
Kind: static property of core
The default endpoint for [[ApiSettings]]
Kind: static property of core
Message class
The Message class is used to model a plain message attached to a transaction.
Kind: static property of core
EncryptedMessage class
The EncryptedMessage class is a model for a encrypted message attached to a transaction.
Kind: static property of core
Account class
The account class serves as a model for a Burstcoin account. It's meant to model the response from BRS API, except publicKey has been moved into the keys object.
Kind: inner class of core
Attachment class
The attachment class is used to appended to transaction where appropriate. It is a super class for Message and EncryptedMessage.
Kind: inner class of core
Original work Copyright (c) 2018 PoC-Consortium Modified work Copyright (c) 2019 Burst Apps Team
Kind: inner class of core
Constants for arbitrary subtypes
Kind: inner property of core
Constants for asset subtypes
Kind: inner property of core
Constants for escrow subtypes
Kind: inner property of core
Constants for leasing subtypes
Kind: inner property of core
Constants for marketplace subtypes
Kind: inner property of core
Constants for payment subtypes
Kind: inner property of core
Constants for reward recipient subtypes (Pool Operation)
Kind: inner property of core
Constants for smart contract (aka AT) subtypes
Kind: inner property of core
Constants for transaction types
The transaction type is part of every [[Transaction]] object and used to distinguish block data. Additionally, to the transaction type a subtype is sent, that specifies the kind of transaction more detailly.
Kind: inner property of core
The smallest possible fee
Kind: inner constant of core
Get the transaction attachment version identifier
Attachment types are identified by a field version.
Kind: inner method of core
Returns:
return Identifier, if exists, otherwise undefined
Param | Description |
---|---|
transaction | The transaction to be checked |
Checks if a transaction attachment is of specific version
Kind: inner method of core
Returns:
true, if version string matches
Param | Description |
---|---|
transaction | The transaction to be checked |
versionIdentifier | The version string, i.e. MultiOutCreation |
Extracts a variables value as hexadecimal string from a contract's machine data
This is a generic function to extract arbitrary data from a contract. I's recommended to use the [[ContractHelper]] class instead
Kind: inner method of core
Returns:
The value as hexadecimal string (already considering endianness)
Param | Default | Description |
---|---|---|
position | The variables position | |
length | 16 | The length of data to be extracted |
Deprecated
Kind: inner method of core
Returns:
HttpParams
Param | Description |
---|---|
transaction | The transaction with the attachment |
params | Some HttpParams |
Signs and broadcasts a transaction
Kind: inner method of core
Returns:
The transaction Id
Param | Description |
---|---|
unsignedTransaction | The unsigned transaction context |
service | The service used for |
Tries to extract recipients and its amounts for multi out payments (different and same amount)
Kind: inner method of core
Returns:
A list of recipients and their payed amount (in NQT)
An exception in case of wrong transaction types
Param | Description |
---|---|
transaction | The transaction |
Gets the amount from a transaction, considering ordinary and multi out transactions (with same and different payments)
Kind: inner method of core
Returns:
the amount in BURST (not NQT)
Param | Description |
---|---|
recipientId | The numeric id of the recipient |
transaction | The payment transaction |
Checks if a transaction is a multi out transaction with same amounts for each recipient
Kind: inner method of core
Returns:
true, if is a multi out transaction
Param | Description |
---|---|
transaction | Transaction to be checked |
Checks if a transaction is a multi out transaction (with different amounts)
Kind: inner method of core
Returns:
true, if is a multi out transaction
Param | Description |
---|---|
transaction | Transaction to be checked |
The default deadline (in minutes) for Transactions
Kind: static property of core
The default endpoint for [[ApiSettings]]
Kind: static property of core
Message class
The Message class is used to model a plain message attached to a transaction.
Kind: static property of core
EncryptedMessage class
The EncryptedMessage class is a model for a encrypted message attached to a transaction.
Kind: static property of core
Account class
The account class serves as a model for a Burstcoin account. It's meant to model the response from BRS API, except publicKey has been moved into the keys object.
Kind: inner class of core
Attachment class
The attachment class is used to appended to transaction where appropriate. It is a super class for Message and EncryptedMessage.
Kind: inner class of core
Original work Copyright (c) 2018 PoC-Consortium Modified work Copyright (c) 2019 Burst Apps Team
Kind: inner class of core
Constants for arbitrary subtypes
Kind: inner property of core
Constants for asset subtypes
Kind: inner property of core
Constants for escrow subtypes
Kind: inner property of core
Constants for leasing subtypes
Kind: inner property of core
Constants for marketplace subtypes
Kind: inner property of core
Constants for payment subtypes
Kind: inner property of core
Constants for reward recipient subtypes (Pool Operation)
Kind: inner property of core
Constants for smart contract (aka AT) subtypes
Kind: inner property of core
Constants for transaction types
The transaction type is part of every [[Transaction]] object and used to distinguish block data. Additionally, to the transaction type a subtype is sent, that specifies the kind of transaction more detailly.
Kind: inner property of core
The smallest possible fee
Kind: inner constant of core
Get the transaction attachment version identifier
Attachment types are identified by a field version.
Kind: inner method of core
Returns:
return Identifier, if exists, otherwise undefined
Param | Description |
---|---|
transaction | The transaction to be checked |
Checks if a transaction attachment is of specific version
Kind: inner method of core
Returns:
true, if version string matches
Param | Description |
---|---|
transaction | The transaction to be checked |
versionIdentifier | The version string, i.e. MultiOutCreation |
Extracts a variables value as hexadecimal string from a contract's machine data
This is a generic function to extract arbitrary data from a contract. I's recommended to use the [[ContractHelper]] class instead
Kind: inner method of core
Returns:
The value as hexadecimal string (already considering endianness)
Param | Default | Description |
---|---|---|
position | The variables position | |
length | 16 | The length of data to be extracted |
Deprecated
Kind: inner method of core
Returns:
HttpParams
Param | Description |
---|---|
transaction | The transaction with the attachment |
params | Some HttpParams |
Signs and broadcasts a transaction
Kind: inner method of core
Returns:
The transaction Id
Param | Description |
---|---|
unsignedTransaction | The unsigned transaction context |
service | The service used for |
Tries to extract recipients and its amounts for multi out payments (different and same amount)
Kind: inner method of core
Returns:
A list of recipients and their payed amount (in NQT)
An exception in case of wrong transaction types
Param | Description |
---|---|
transaction | The transaction |
Gets the amount from a transaction, considering ordinary and multi out transactions (with same and different payments)
Kind: inner method of core
Returns:
the amount in BURST (not NQT)
Param | Description |
---|---|
recipientId | The numeric id of the recipient |
transaction | The payment transaction |
Checks if a transaction is a multi out transaction with same amounts for each recipient
Kind: inner method of core
Returns:
true, if is a multi out transaction
Param | Description |
---|---|
transaction | Transaction to be checked |
Checks if a transaction is a multi out transaction (with different amounts)
Kind: inner method of core
Returns:
true, if is a multi out transaction
Param | Description |
---|---|
transaction | Transaction to be checked |
The default deadline (in minutes) for Transactions
Kind: static property of core
The default endpoint for [[ApiSettings]]
Kind: static property of core
Message class
The Message class is used to model a plain message attached to a transaction.
Kind: static property of core
EncryptedMessage class
The EncryptedMessage class is a model for a encrypted message attached to a transaction.
Kind: static property of core
Account class
The account class serves as a model for a Burstcoin account. It's meant to model the response from BRS API, except publicKey has been moved into the keys object.
Kind: inner class of core
Attachment class
The attachment class is used to appended to transaction where appropriate. It is a super class for Message and EncryptedMessage.
Kind: inner class of core
Original work Copyright (c) 2018 PoC-Consortium Modified work Copyright (c) 2019 Burst Apps Team
Kind: inner class of core
Constants for arbitrary subtypes
Kind: inner property of core
Constants for asset subtypes
Kind: inner property of core
Constants for escrow subtypes
Kind: inner property of core
Constants for leasing subtypes
Kind: inner property of core
Constants for marketplace subtypes
Kind: inner property of core
Constants for payment subtypes
Kind: inner property of core
Constants for reward recipient subtypes (Pool Operation)
Kind: inner property of core
Constants for smart contract (aka AT) subtypes
Kind: inner property of core
Constants for transaction types
The transaction type is part of every [[Transaction]] object and used to distinguish block data. Additionally, to the transaction type a subtype is sent, that specifies the kind of transaction more detailly.
Kind: inner property of core
The smallest possible fee
Kind: inner constant of core
Get the transaction attachment version identifier
Attachment types are identified by a field version.
Kind: inner method of core
Returns:
return Identifier, if exists, otherwise undefined
Param | Description |
---|---|
transaction | The transaction to be checked |
Checks if a transaction attachment is of specific version
Kind: inner method of core
Returns:
true, if version string matches
Param | Description |
---|---|
transaction | The transaction to be checked |
versionIdentifier | The version string, i.e. MultiOutCreation |
Extracts a variables value as hexadecimal string from a contract's machine data
This is a generic function to extract arbitrary data from a contract. I's recommended to use the [[ContractHelper]] class instead
Kind: inner method of core
Returns:
The value as hexadecimal string (already considering endianness)
Param | Default | Description |
---|---|---|
position | The variables position | |
length | 16 | The length of data to be extracted |
Deprecated
Kind: inner method of core
Returns:
HttpParams
Param | Description |
---|---|
transaction | The transaction with the attachment |
params | Some HttpParams |
Signs and broadcasts a transaction
Kind: inner method of core
Returns:
The transaction Id
Param | Description |
---|---|
unsignedTransaction | The unsigned transaction context |
service | The service used for |
Tries to extract recipients and its amounts for multi out payments (different and same amount)
Kind: inner method of core
Returns:
A list of recipients and their payed amount (in NQT)
An exception in case of wrong transaction types
Param | Description |
---|---|
transaction | The transaction |
Gets the amount from a transaction, considering ordinary and multi out transactions (with same and different payments)
Kind: inner method of core
Returns:
the amount in BURST (not NQT)
Param | Description |
---|---|
recipientId | The numeric id of the recipient |
transaction | The payment transaction |
Checks if a transaction is a multi out transaction with same amounts for each recipient
Kind: inner method of core
Returns:
true, if is a multi out transaction
Param | Description |
---|---|
transaction | Transaction to be checked |
Checks if a transaction is a multi out transaction (with different amounts)
Kind: inner method of core
Returns:
true, if is a multi out transaction
Param | Description |
---|---|
transaction | Transaction to be checked |
The default deadline (in minutes) for Transactions
Kind: static property of core
The default endpoint for [[ApiSettings]]
Kind: static property of core
Message class
The Message class is used to model a plain message attached to a transaction.
Kind: static property of core
EncryptedMessage class
The EncryptedMessage class is a model for a encrypted message attached to a transaction.
Kind: static property of core
Account class
The account class serves as a model for a Burstcoin account. It's meant to model the response from BRS API, except publicKey has been moved into the keys object.
Kind: inner class of core
Attachment class
The attachment class is used to appended to transaction where appropriate. It is a super class for Message and EncryptedMessage.
Kind: inner class of core
Original work Copyright (c) 2018 PoC-Consortium Modified work Copyright (c) 2019 Burst Apps Team
Kind: inner class of core
Constants for arbitrary subtypes
Kind: inner property of core
Constants for asset subtypes
Kind: inner property of core
Constants for escrow subtypes
Kind: inner property of core
Constants for leasing subtypes
Kind: inner property of core
Constants for marketplace subtypes
Kind: inner property of core
Constants for payment subtypes
Kind: inner property of core
Constants for reward recipient subtypes (Pool Operation)
Kind: inner property of core
Constants for smart contract (aka AT) subtypes
Kind: inner property of core
Constants for transaction types
The transaction type is part of every [[Transaction]] object and used to distinguish block data. Additionally, to the transaction type a subtype is sent, that specifies the kind of transaction more detailly.
Kind: inner property of core
The smallest possible fee
Kind: inner constant of core
Get the transaction attachment version identifier
Attachment types are identified by a field version.
Kind: inner method of core
Returns:
return Identifier, if exists, otherwise undefined
Param | Description |
---|---|
transaction | The transaction to be checked |
Checks if a transaction attachment is of specific version
Kind: inner method of core
Returns:
true, if version string matches
Param | Description |
---|---|
transaction | The transaction to be checked |
versionIdentifier | The version string, i.e. MultiOutCreation |
Extracts a variables value as hexadecimal string from a contract's machine data
This is a generic function to extract arbitrary data from a contract. I's recommended to use the [[ContractHelper]] class instead
Kind: inner method of core
Returns:
The value as hexadecimal string (already considering endianness)
Param | Default | Description |
---|---|---|
position | The variables position | |
length | 16 | The length of data to be extracted |
Deprecated
Kind: inner method of core
Returns:
HttpParams
Param | Description |
---|---|
transaction | The transaction with the attachment |
params | Some HttpParams |
Signs and broadcasts a transaction
Kind: inner method of core
Returns:
The transaction Id
Param | Description |
---|---|
unsignedTransaction | The unsigned transaction context |
service | The service used for |
Tries to extract recipients and its amounts for multi out payments (different and same amount)
Kind: inner method of core
Returns:
A list of recipients and their payed amount (in NQT)
An exception in case of wrong transaction types
Param | Description |
---|---|
transaction | The transaction |
Gets the amount from a transaction, considering ordinary and multi out transactions (with same and different payments)
Kind: inner method of core
Returns:
the amount in BURST (not NQT)
Param | Description |
---|---|
recipientId | The numeric id of the recipient |
transaction | The payment transaction |
Checks if a transaction is a multi out transaction with same amounts for each recipient
Kind: inner method of core
Returns:
true, if is a multi out transaction
Param | Description |
---|---|
transaction | Transaction to be checked |
Checks if a transaction is a multi out transaction (with different amounts)
Kind: inner method of core
Returns:
true, if is a multi out transaction
Param | Description |
---|---|
transaction | Transaction to be checked |
The default deadline (in minutes) for Transactions
Kind: static property of core
The default endpoint for [[ApiSettings]]
Kind: static property of core
Message class
The Message class is used to model a plain message attached to a transaction.
Kind: static property of core
EncryptedMessage class
The EncryptedMessage class is a model for a encrypted message attached to a transaction.
Kind: static property of core
Account class
The account class serves as a model for a Burstcoin account. It's meant to model the response from BRS API, except publicKey has been moved into the keys object.
Kind: inner class of core
Attachment class
The attachment class is used to appended to transaction where appropriate. It is a super class for Message and EncryptedMessage.
Kind: inner class of core
Original work Copyright (c) 2018 PoC-Consortium Modified work Copyright (c) 2019 Burst Apps Team
Kind: inner class of core
Constants for arbitrary subtypes
Kind: inner property of core
Constants for asset subtypes
Kind: inner property of core
Constants for escrow subtypes
Kind: inner property of core
Constants for leasing subtypes
Kind: inner property of core
Constants for marketplace subtypes
Kind: inner property of core
Constants for payment subtypes
Kind: inner property of core
Constants for reward recipient subtypes (Pool Operation)
Kind: inner property of core
Constants for smart contract (aka AT) subtypes
Kind: inner property of core
Constants for transaction types
The transaction type is part of every [[Transaction]] object and used to distinguish block data. Additionally, to the transaction type a subtype is sent, that specifies the kind of transaction more detailly.
Kind: inner property of core
The smallest possible fee
Kind: inner constant of core
Get the transaction attachment version identifier
Attachment types are identified by a field version.
Kind: inner method of core
Returns:
return Identifier, if exists, otherwise undefined
Param | Description |
---|---|
transaction | The transaction to be checked |
Checks if a transaction attachment is of specific version
Kind: inner method of core
Returns:
true, if version string matches
Param | Description |
---|---|
transaction | The transaction to be checked |
versionIdentifier | The version string, i.e. MultiOutCreation |
Extracts a variables value as hexadecimal string from a contract's machine data
This is a generic function to extract arbitrary data from a contract. I's recommended to use the [[ContractHelper]] class instead
Kind: inner method of core
Returns:
The value as hexadecimal string (already considering endianness)
Param | Default | Description |
---|---|---|
position | The variables position | |
length | 16 | The length of data to be extracted |
Deprecated
Kind: inner method of core
Returns:
HttpParams
Param | Description |
---|---|
transaction | The transaction with the attachment |
params | Some HttpParams |
Signs and broadcasts a transaction
Kind: inner method of core
Returns:
The transaction Id
Param | Description |
---|---|
unsignedTransaction | The unsigned transaction context |
service | The service used for |
Tries to extract recipients and its amounts for multi out payments (different and same amount)
Kind: inner method of core
Returns:
A list of recipients and their payed amount (in NQT)
An exception in case of wrong transaction types
Param | Description |
---|---|
transaction | The transaction |
Gets the amount from a transaction, considering ordinary and multi out transactions (with same and different payments)
Kind: inner method of core
Returns:
the amount in BURST (not NQT)
Param | Description |
---|---|
recipientId | The numeric id of the recipient |
transaction | The payment transaction |
Checks if a transaction is a multi out transaction with same amounts for each recipient
Kind: inner method of core
Returns:
true, if is a multi out transaction
Param | Description |
---|---|
transaction | Transaction to be checked |
Checks if a transaction is a multi out transaction (with different amounts)
Kind: inner method of core
Returns:
true, if is a multi out transaction
Param | Description |
---|---|
transaction | Transaction to be checked |
The default deadline (in minutes) for Transactions
Kind: static property of core
The default endpoint for [[ApiSettings]]
Kind: static property of core
Message class
The Message class is used to model a plain message attached to a transaction.
Kind: static property of core
EncryptedMessage class
The EncryptedMessage class is a model for a encrypted message attached to a transaction.
Kind: static property of core
Account class
The account class serves as a model for a Burstcoin account. It's meant to model the response from BRS API, except publicKey has been moved into the keys object.
Kind: inner class of core
Attachment class
The attachment class is used to appended to transaction where appropriate. It is a super class for Message and EncryptedMessage.
Kind: inner class of core
Original work Copyright (c) 2018 PoC-Consortium Modified work Copyright (c) 2019 Burst Apps Team
Kind: inner class of core
Constants for arbitrary subtypes
Kind: inner property of core
Constants for asset subtypes
Kind: inner property of core
Constants for escrow subtypes
Kind: inner property of core
Constants for leasing subtypes
Kind: inner property of core
Constants for marketplace subtypes
Kind: inner property of core
Constants for payment subtypes
Kind: inner property of core
Constants for reward recipient subtypes (Pool Operation)
Kind: inner property of core
Constants for smart contract (aka AT) subtypes
Kind: inner property of core
Constants for transaction types
The transaction type is part of every [[Transaction]] object and used to distinguish block data. Additionally, to the transaction type a subtype is sent, that specifies the kind of transaction more detailly.
Kind: inner property of core
The smallest possible fee
Kind: inner constant of core
Get the transaction attachment version identifier
Attachment types are identified by a field version.
Kind: inner method of core
Returns:
return Identifier, if exists, otherwise undefined
Param | Description |
---|---|
transaction | The transaction to be checked |
Checks if a transaction attachment is of specific version
Kind: inner method of core
Returns:
true, if version string matches
Param | Description |
---|---|
transaction | The transaction to be checked |
versionIdentifier | The version string, i.e. MultiOutCreation |
Extracts a variables value as hexadecimal string from a contract's machine data
This is a generic function to extract arbitrary data from a contract. I's recommended to use the [[ContractHelper]] class instead
Kind: inner method of core
Returns:
The value as hexadecimal string (already considering endianness)
Param | Default | Description |
---|---|---|
position | The variables position | |
length | 16 | The length of data to be extracted |
Deprecated
Kind: inner method of core
Returns:
HttpParams
Param | Description |
---|---|
transaction | The transaction with the attachment |
params | Some HttpParams |
Signs and broadcasts a transaction
Kind: inner method of core
Returns:
The transaction Id
Param | Description |
---|---|
unsignedTransaction | The unsigned transaction context |
service | The service used for |
Tries to extract recipients and its amounts for multi out payments (different and same amount)
Kind: inner method of core
Returns:
A list of recipients and their payed amount (in NQT)
An exception in case of wrong transaction types
Param | Description |
---|---|
transaction | The transaction |
Gets the amount from a transaction, considering ordinary and multi out transactions (with same and different payments)
Kind: inner method of core
Returns:
the amount in BURST (not NQT)
Param | Description |
---|---|
recipientId | The numeric id of the recipient |
transaction | The payment transaction |
Checks if a transaction is a multi out transaction with same amounts for each recipient
Kind: inner method of core
Returns:
true, if is a multi out transaction
Param | Description |
---|---|
transaction | Transaction to be checked |
Checks if a transaction is a multi out transaction (with different amounts)
Kind: inner method of core
Returns:
true, if is a multi out transaction
Param | Description |
---|---|
transaction | Transaction to be checked |
The default deadline (in minutes) for Transactions
Kind: static property of core
The default endpoint for [[ApiSettings]]
Kind: static property of core
Message class
The Message class is used to model a plain message attached to a transaction.
Kind: static property of core
EncryptedMessage class
The EncryptedMessage class is a model for a encrypted message attached to a transaction.
Kind: static property of core
Account class
The account class serves as a model for a Burstcoin account. It's meant to model the response from BRS API, except publicKey has been moved into the keys object.
Kind: inner class of core
Attachment class
The attachment class is used to appended to transaction where appropriate. It is a super class for Message and EncryptedMessage.
Kind: inner class of core
Original work Copyright (c) 2018 PoC-Consortium Modified work Copyright (c) 2019 Burst Apps Team
Kind: inner class of core
Constants for arbitrary subtypes
Kind: inner property of core
Constants for asset subtypes
Kind: inner property of core
Constants for escrow subtypes
Kind: inner property of core
Constants for leasing subtypes
Kind: inner property of core
Constants for marketplace subtypes
Kind: inner property of core
Constants for payment subtypes
Kind: inner property of core
Constants for reward recipient subtypes (Pool Operation)
Kind: inner property of core
Constants for smart contract (aka AT) subtypes
Kind: inner property of core
Constants for transaction types
The transaction type is part of every [[Transaction]] object and used to distinguish block data. Additionally, to the transaction type a subtype is sent, that specifies the kind of transaction more detailly.
Kind: inner property of core
The smallest possible fee
Kind: inner constant of core
Get the transaction attachment version identifier
Attachment types are identified by a field version.
Kind: inner method of core
Returns:
return Identifier, if exists, otherwise undefined
Param | Description |
---|---|
transaction | The transaction to be checked |
Checks if a transaction attachment is of specific version
Kind: inner method of core
Returns:
true, if version string matches
Param | Description |
---|---|
transaction | The transaction to be checked |
versionIdentifier | The version string, i.e. MultiOutCreation |
Extracts a variables value as hexadecimal string from a contract's machine data
This is a generic function to extract arbitrary data from a contract. I's recommended to use the [[ContractHelper]] class instead
Kind: inner method of core
Returns:
The value as hexadecimal string (already considering endianness)
Param | Default | Description |
---|---|---|
position | The variables position | |
length | 16 | The length of data to be extracted |
Deprecated
Kind: inner method of core
Returns:
HttpParams
Param | Description |
---|---|
transaction | The transaction with the attachment |
params | Some HttpParams |
Signs and broadcasts a transaction
Kind: inner method of core
Returns:
The transaction Id
Param | Description |
---|---|
unsignedTransaction | The unsigned transaction context |
service | The service used for |
Tries to extract recipients and its amounts for multi out payments (different and same amount)
Kind: inner method of core
Returns:
A list of recipients and their payed amount (in NQT)
An exception in case of wrong transaction types
Param | Description |
---|---|
transaction | The transaction |
Gets the amount from a transaction, considering ordinary and multi out transactions (with same and different payments)
Kind: inner method of core
Returns:
the amount in BURST (not NQT)
Param | Description |
---|---|
recipientId | The numeric id of the recipient |
transaction | The payment transaction |
Checks if a transaction is a multi out transaction with same amounts for each recipient
Kind: inner method of core
Returns:
true, if is a multi out transaction
Param | Description |
---|---|
transaction | Transaction to be checked |
Checks if a transaction is a multi out transaction (with different amounts)
Kind: inner method of core
Returns:
true, if is a multi out transaction
Param | Description |
---|---|
transaction | Transaction to be checked |
The default deadline (in minutes) for Transactions
Kind: static property of core
The default endpoint for [[ApiSettings]]
Kind: static property of core
Message class
The Message class is used to model a plain message attached to a transaction.
Kind: static property of core
EncryptedMessage class
The EncryptedMessage class is a model for a encrypted message attached to a transaction.
Kind: static property of core
Account class
The account class serves as a model for a Burstcoin account. It's meant to model the response from BRS API, except publicKey has been moved into the keys object.
Kind: inner class of core
Attachment class
The attachment class is used to appended to transaction where appropriate. It is a super class for Message and EncryptedMessage.
Kind: inner class of core
Original work Copyright (c) 2018 PoC-Consortium Modified work Copyright (c) 2019 Burst Apps Team
Kind: inner class of core
Constants for arbitrary subtypes
Kind: inner property of core
Constants for asset subtypes
Kind: inner property of core
Constants for escrow subtypes
Kind: inner property of core
Constants for leasing subtypes
Kind: inner property of core
Constants for marketplace subtypes
Kind: inner property of core
Constants for payment subtypes
Kind: inner property of core
Constants for reward recipient subtypes (Pool Operation)
Kind: inner property of core
Constants for smart contract (aka AT) subtypes
Kind: inner property of core
Constants for transaction types
The transaction type is part of every [[Transaction]] object and used to distinguish block data. Additionally, to the transaction type a subtype is sent, that specifies the kind of transaction more detailly.
Kind: inner property of core
The smallest possible fee
Kind: inner constant of core
Get the transaction attachment version identifier
Attachment types are identified by a field version.
Kind: inner method of core
Returns:
return Identifier, if exists, otherwise undefined
Param | Description |
---|---|
transaction | The transaction to be checked |
Checks if a transaction attachment is of specific version
Kind: inner method of core
Returns:
true, if version string matches
Param | Description |
---|---|
transaction | The transaction to be checked |
versionIdentifier | The version string, i.e. MultiOutCreation |
Extracts a variables value as hexadecimal string from a contract's machine data
This is a generic function to extract arbitrary data from a contract. I's recommended to use the [[ContractHelper]] class instead
Kind: inner method of core
Returns:
The value as hexadecimal string (already considering endianness)
Param | Default | Description |
---|---|---|
position | The variables position | |
length | 16 | The length of data to be extracted |
Deprecated
Kind: inner method of core
Returns:
HttpParams
Param | Description |
---|---|
transaction | The transaction with the attachment |
params | Some HttpParams |
Signs and broadcasts a transaction
Kind: inner method of core
Returns:
The transaction Id
Param | Description |
---|---|
unsignedTransaction | The unsigned transaction context |
service | The service used for |
Tries to extract recipients and its amounts for multi out payments (different and same amount)
Kind: inner method of core
Returns:
A list of recipients and their payed amount (in NQT)
An exception in case of wrong transaction types
Param | Description |
---|---|
transaction | The transaction |
Gets the amount from a transaction, considering ordinary and multi out transactions (with same and different payments)
Kind: inner method of core
Returns:
the amount in BURST (not NQT)
Param | Description |
---|---|
recipientId | The numeric id of the recipient |
transaction | The payment transaction |
Checks if a transaction is a multi out transaction with same amounts for each recipient
Kind: inner method of core
Returns:
true, if is a multi out transaction
Param | Description |
---|---|
transaction | Transaction to be checked |
Checks if a transaction is a multi out transaction (with different amounts)
Kind: inner method of core
Returns:
true, if is a multi out transaction
Param | Description |
---|---|
transaction | Transaction to be checked |
The default deadline (in minutes) for Transactions
Kind: static property of core
The default endpoint for [[ApiSettings]]
Kind: static property of core
Message class
The Message class is used to model a plain message attached to a transaction.
Kind: static property of core
EncryptedMessage class
The EncryptedMessage class is a model for a encrypted message attached to a transaction.
Kind: static property of core
Account class
The account class serves as a model for a Burstcoin account. It's meant to model the response from BRS API, except publicKey has been moved into the keys object.
Kind: inner class of core
Attachment class
The attachment class is used to appended to transaction where appropriate. It is a super class for Message and EncryptedMessage.
Kind: inner class of core
Original work Copyright (c) 2018 PoC-Consortium Modified work Copyright (c) 2019 Burst Apps Team
Kind: inner class of core
Constants for arbitrary subtypes
Kind: inner property of core
Constants for asset subtypes
Kind: inner property of core
Constants for escrow subtypes
Kind: inner property of core
Constants for leasing subtypes
Kind: inner property of core
Constants for marketplace subtypes
Kind: inner property of core
Constants for payment subtypes
Kind: inner property of core
Constants for reward recipient subtypes (Pool Operation)
Kind: inner property of core
Constants for smart contract (aka AT) subtypes
Kind: inner property of core
Constants for transaction types
The transaction type is part of every [[Transaction]] object and used to distinguish block data. Additionally, to the transaction type a subtype is sent, that specifies the kind of transaction more detailly.
Kind: inner property of core
The smallest possible fee
Kind: inner constant of core
Get the transaction attachment version identifier
Attachment types are identified by a field version.
Kind: inner method of core
Returns:
return Identifier, if exists, otherwise undefined
Param | Description |
---|---|
transaction | The transaction to be checked |
Checks if a transaction attachment is of specific version
Kind: inner method of core
Returns:
true, if version string matches
Param | Description |
---|---|
transaction | The transaction to be checked |
versionIdentifier | The version string, i.e. MultiOutCreation |
Extracts a variables value as hexadecimal string from a contract's machine data
This is a generic function to extract arbitrary data from a contract. I's recommended to use the [[ContractHelper]] class instead
Kind: inner method of core
Returns:
The value as hexadecimal string (already considering endianness)
Param | Default | Description |
---|---|---|
position | The variables position | |
length | 16 | The length of data to be extracted |
Deprecated
Kind: inner method of core
Returns:
HttpParams
Param | Description |
---|---|
transaction | The transaction with the attachment |
params | Some HttpParams |
Signs and broadcasts a transaction
Kind: inner method of core
Returns:
The transaction Id
Param | Description |
---|---|
unsignedTransaction | The unsigned transaction context |
service | The service used for |
Tries to extract recipients and its amounts for multi out payments (different and same amount)
Kind: inner method of core
Returns:
A list of recipients and their payed amount (in NQT)
An exception in case of wrong transaction types
Param | Description |
---|---|
transaction | The transaction |
Gets the amount from a transaction, considering ordinary and multi out transactions (with same and different payments)
Kind: inner method of core
Returns:
the amount in BURST (not NQT)
Param | Description |
---|---|
recipientId | The numeric id of the recipient |
transaction | The payment transaction |
Checks if a transaction is a multi out transaction with same amounts for each recipient
Kind: inner method of core
Returns:
true, if is a multi out transaction
Param | Description |
---|---|
transaction | Transaction to be checked |
Checks if a transaction is a multi out transaction (with different amounts)
Kind: inner method of core
Returns:
true, if is a multi out transaction
Param | Description |
---|---|
transaction | Transaction to be checked |
The default deadline (in minutes) for Transactions
Kind: static property of core
The default endpoint for [[ApiSettings]]
Kind: static property of core
Message class
The Message class is used to model a plain message attached to a transaction.
Kind: static property of core
EncryptedMessage class
The EncryptedMessage class is a model for a encrypted message attached to a transaction.
Kind: static property of core
Account class
The account class serves as a model for a Burstcoin account. It's meant to model the response from BRS API, except publicKey has been moved into the keys object.
Kind: inner class of core
Attachment class
The attachment class is used to appended to transaction where appropriate. It is a super class for Message and EncryptedMessage.
Kind: inner class of core
Original work Copyright (c) 2018 PoC-Consortium Modified work Copyright (c) 2019 Burst Apps Team
Kind: inner class of core
Constants for arbitrary subtypes
Kind: inner property of core
Constants for asset subtypes
Kind: inner property of core
Constants for escrow subtypes
Kind: inner property of core
Constants for leasing subtypes
Kind: inner property of core
Constants for marketplace subtypes
Kind: inner property of core
Constants for payment subtypes
Kind: inner property of core
Constants for reward recipient subtypes (Pool Operation)
Kind: inner property of core
Constants for smart contract (aka AT) subtypes
Kind: inner property of core
Constants for transaction types
The transaction type is part of every [[Transaction]] object and used to distinguish block data. Additionally, to the transaction type a subtype is sent, that specifies the kind of transaction more detailly.
Kind: inner property of core
The smallest possible fee
Kind: inner constant of core
Get the transaction attachment version identifier
Attachment types are identified by a field version.
Kind: inner method of core
Returns:
return Identifier, if exists, otherwise undefined
Param | Description |
---|---|
transaction | The transaction to be checked |
Checks if a transaction attachment is of specific version
Kind: inner method of core
Returns:
true, if version string matches
Param | Description |
---|---|
transaction | The transaction to be checked |
versionIdentifier | The version string, i.e. MultiOutCreation |
Extracts a variables value as hexadecimal string from a contract's machine data
This is a generic function to extract arbitrary data from a contract. I's recommended to use the [[ContractHelper]] class instead
Kind: inner method of core
Returns:
The value as hexadecimal string (already considering endianness)
Param | Default | Description |
---|---|---|
position | The variables position | |
length | 16 | The length of data to be extracted |
Deprecated
Kind: inner method of core
Returns:
HttpParams
Param | Description |
---|---|
transaction | The transaction with the attachment |
params | Some HttpParams |
Signs and broadcasts a transaction
Kind: inner method of core
Returns:
The transaction Id
Param | Description |
---|---|
unsignedTransaction | The unsigned transaction context |
service | The service used for |
Tries to extract recipients and its amounts for multi out payments (different and same amount)
Kind: inner method of core
Returns:
A list of recipients and their payed amount (in NQT)
An exception in case of wrong transaction types
Param | Description |
---|---|
transaction | The transaction |
Gets the amount from a transaction, considering ordinary and multi out transactions (with same and different payments)
Kind: inner method of core
Returns:
the amount in BURST (not NQT)
Param | Description |
---|---|
recipientId | The numeric id of the recipient |
transaction | The payment transaction |
Checks if a transaction is a multi out transaction with same amounts for each recipient
Kind: inner method of core
Returns:
true, if is a multi out transaction
Param | Description |
---|---|
transaction | Transaction to be checked |
Checks if a transaction is a multi out transaction (with different amounts)
Kind: inner method of core
Returns:
true, if is a multi out transaction
Param | Description |
---|---|
transaction | Transaction to be checked |
The default deadline (in minutes) for Transactions
Kind: static property of core
The default endpoint for [[ApiSettings]]
Kind: static property of core
Message class
The Message class is used to model a plain message attached to a transaction.
Kind: static property of core
EncryptedMessage class
The EncryptedMessage class is a model for a encrypted message attached to a transaction.
Kind: static property of core
Account class
The account class serves as a model for a Burstcoin account. It's meant to model the response from BRS API, except publicKey has been moved into the keys object.
Kind: inner class of core
Attachment class
The attachment class is used to appended to transaction where appropriate. It is a super class for Message and EncryptedMessage.
Kind: inner class of core
Original work Copyright (c) 2018 PoC-Consortium Modified work Copyright (c) 2019 Burst Apps Team
Kind: inner class of core
Constants for arbitrary subtypes
Kind: inner property of core
Constants for asset subtypes
Kind: inner property of core
Constants for escrow subtypes
Kind: inner property of core
Constants for leasing subtypes
Kind: inner property of core
Constants for marketplace subtypes
Kind: inner property of core
Constants for payment subtypes
Kind: inner property of core
Constants for reward recipient subtypes (Pool Operation)
Kind: inner property of core
Constants for smart contract (aka AT) subtypes
Kind: inner property of core
Constants for transaction types
The transaction type is part of every [[Transaction]] object and used to distinguish block data. Additionally, to the transaction type a subtype is sent, that specifies the kind of transaction more detailly.
Kind: inner property of core
The smallest possible fee
Kind: inner constant of core
Get the transaction attachment version identifier
Attachment types are identified by a field version.
Kind: inner method of core
Returns:
return Identifier, if exists, otherwise undefined
Param | Description |
---|---|
transaction | The transaction to be checked |
Checks if a transaction attachment is of specific version
Kind: inner method of core
Returns:
true, if version string matches
Param | Description |
---|---|
transaction | The transaction to be checked |
versionIdentifier | The version string, i.e. MultiOutCreation |
Extracts a variables value as hexadecimal string from a contract's machine data
This is a generic function to extract arbitrary data from a contract. I's recommended to use the [[ContractHelper]] class instead
Kind: inner method of core
Returns:
The value as hexadecimal string (already considering endianness)
Param | Default | Description |
---|---|---|
position | The variables position | |
length | 16 | The length of data to be extracted |
Deprecated
Kind: inner method of core
Returns:
HttpParams
Param | Description |
---|---|
transaction | The transaction with the attachment |
params | Some HttpParams |
Signs and broadcasts a transaction
Kind: inner method of core
Returns:
The transaction Id
Param | Description |
---|---|
unsignedTransaction | The unsigned transaction context |
service | The service used for |
Tries to extract recipients and its amounts for multi out payments (different and same amount)
Kind: inner method of core
Returns:
A list of recipients and their payed amount (in NQT)
An exception in case of wrong transaction types
Param | Description |
---|---|
transaction | The transaction |
Gets the amount from a transaction, considering ordinary and multi out transactions (with same and different payments)
Kind: inner method of core
Returns:
the amount in BURST (not NQT)
Param | Description |
---|---|
recipientId | The numeric id of the recipient |
transaction | The payment transaction |
Checks if a transaction is a multi out transaction with same amounts for each recipient
Kind: inner method of core
Returns:
true, if is a multi out transaction
Param | Description |
---|---|
transaction | Transaction to be checked |
Checks if a transaction is a multi out transaction (with different amounts)
Kind: inner method of core
Returns:
true, if is a multi out transaction
Param | Description |
---|---|
transaction | Transaction to be checked |
The default deadline (in minutes) for Transactions
Kind: static property of core
The default endpoint for [[ApiSettings]]
Kind: static property of core
Message class
The Message class is used to model a plain message attached to a transaction.
Kind: static property of core
EncryptedMessage class
The EncryptedMessage class is a model for a encrypted message attached to a transaction.
Kind: static property of core
Account class
The account class serves as a model for a Burstcoin account. It's meant to model the response from BRS API, except publicKey has been moved into the keys object.
Kind: inner class of core
Attachment class
The attachment class is used to appended to transaction where appropriate. It is a super class for Message and EncryptedMessage.
Kind: inner class of core
Original work Copyright (c) 2018 PoC-Consortium Modified work Copyright (c) 2019 Burst Apps Team
Kind: inner class of core
Constants for arbitrary subtypes
Kind: inner property of core
Constants for asset subtypes
Kind: inner property of core
Constants for escrow subtypes
Kind: inner property of core
Constants for leasing subtypes
Kind: inner property of core
Constants for marketplace subtypes
Kind: inner property of core
Constants for payment subtypes
Kind: inner property of core
Constants for reward recipient subtypes (Pool Operation)
Kind: inner property of core
Constants for smart contract (aka AT) subtypes
Kind: inner property of core
Constants for transaction types
The transaction type is part of every [[Transaction]] object and used to distinguish block data. Additionally, to the transaction type a subtype is sent, that specifies the kind of transaction more detailly.
Kind: inner property of core
The smallest possible fee
Kind: inner constant of core
Get the transaction attachment version identifier
Attachment types are identified by a field version.
Kind: inner method of core
Returns:
return Identifier, if exists, otherwise undefined
Param | Description |
---|---|
transaction | The transaction to be checked |
Checks if a transaction attachment is of specific version
Kind: inner method of core
Returns:
true, if version string matches
Param | Description |
---|---|
transaction | The transaction to be checked |
versionIdentifier | The version string, i.e. MultiOutCreation |
Extracts a variables value as hexadecimal string from a contract's machine data
This is a generic function to extract arbitrary data from a contract. I's recommended to use the [[ContractHelper]] class instead
Kind: inner method of core
Returns:
The value as hexadecimal string (already considering endianness)
Param | Default | Description |
---|---|---|
position | The variables position | |
length | 16 | The length of data to be extracted |
Deprecated
Kind: inner method of core
Returns:
HttpParams
Param | Description |
---|---|
transaction | The transaction with the attachment |
params | Some HttpParams |
Signs and broadcasts a transaction
Kind: inner method of core
Returns:
The transaction Id
Param | Description |
---|---|
unsignedTransaction | The unsigned transaction context |
service | The service used for |
Tries to extract recipients and its amounts for multi out payments (different and same amount)
Kind: inner method of core
Returns:
A list of recipients and their payed amount (in NQT)
An exception in case of wrong transaction types
Param | Description |
---|---|
transaction | The transaction |
Gets the amount from a transaction, considering ordinary and multi out transactions (with same and different payments)
Kind: inner method of core
Returns:
the amount in BURST (not NQT)
Param | Description |
---|---|
recipientId | The numeric id of the recipient |
transaction | The payment transaction |
Checks if a transaction is a multi out transaction with same amounts for each recipient
Kind: inner method of core
Returns:
true, if is a multi out transaction
Param | Description |
---|---|
transaction | Transaction to be checked |
Checks if a transaction is a multi out transaction (with different amounts)
Kind: inner method of core
Returns:
true, if is a multi out transaction
Param | Description |
---|---|
transaction | Transaction to be checked |
The default deadline (in minutes) for Transactions
Kind: static property of core
The default endpoint for [[ApiSettings]]
Kind: static property of core
Message class
The Message class is used to model a plain message attached to a transaction.
Kind: static property of core
EncryptedMessage class
The EncryptedMessage class is a model for a encrypted message attached to a transaction.
Kind: static property of core
Account class
The account class serves as a model for a Burstcoin account. It's meant to model the response from BRS API, except publicKey has been moved into the keys object.
Kind: inner class of core
Attachment class
The attachment class is used to appended to transaction where appropriate. It is a super class for Message and EncryptedMessage.
Kind: inner class of core
Original work Copyright (c) 2018 PoC-Consortium Modified work Copyright (c) 2019 Burst Apps Team
Kind: inner class of core
Constants for arbitrary subtypes
Kind: inner property of core
Constants for asset subtypes
Kind: inner property of core
Constants for escrow subtypes
Kind: inner property of core
Constants for leasing subtypes
Kind: inner property of core
Constants for marketplace subtypes
Kind: inner property of core
Constants for payment subtypes
Kind: inner property of core
Constants for reward recipient subtypes (Pool Operation)
Kind: inner property of core
Constants for smart contract (aka AT) subtypes
Kind: inner property of core
Constants for transaction types
The transaction type is part of every [[Transaction]] object and used to distinguish block data. Additionally, to the transaction type a subtype is sent, that specifies the kind of transaction more detailly.
Kind: inner property of core
The smallest possible fee
Kind: inner constant of core
Get the transaction attachment version identifier
Attachment types are identified by a field version.
Kind: inner method of core
Returns:
return Identifier, if exists, otherwise undefined
Param | Description |
---|---|
transaction | The transaction to be checked |
Checks if a transaction attachment is of specific version
Kind: inner method of core
Returns:
true, if version string matches
Param | Description |
---|---|
transaction | The transaction to be checked |
versionIdentifier | The version string, i.e. MultiOutCreation |
Extracts a variables value as hexadecimal string from a contract's machine data
This is a generic function to extract arbitrary data from a contract. I's recommended to use the [[ContractHelper]] class instead
Kind: inner method of core
Returns:
The value as hexadecimal string (already considering endianness)
Param | Default | Description |
---|---|---|
position | The variables position | |
length | 16 | The length of data to be extracted |
Deprecated
Kind: inner method of core
Returns:
HttpParams
Param | Description |
---|---|
transaction | The transaction with the attachment |
params | Some HttpParams |
Signs and broadcasts a transaction
Kind: inner method of core
Returns:
The transaction Id
Param | Description |
---|---|
unsignedTransaction | The unsigned transaction context |
service | The service used for |
Tries to extract recipients and its amounts for multi out payments (different and same amount)
Kind: inner method of core
Returns:
A list of recipients and their payed amount (in NQT)
An exception in case of wrong transaction types
Param | Description |
---|---|
transaction | The transaction |
Gets the amount from a transaction, considering ordinary and multi out transactions (with same and different payments)
Kind: inner method of core
Returns:
the amount in BURST (not NQT)
Param | Description |
---|---|
recipientId | The numeric id of the recipient |
transaction | The payment transaction |
Checks if a transaction is a multi out transaction with same amounts for each recipient
Kind: inner method of core
Returns:
true, if is a multi out transaction
Param | Description |
---|---|
transaction | Transaction to be checked |
Checks if a transaction is a multi out transaction (with different amounts)
Kind: inner method of core
Returns:
true, if is a multi out transaction
Param | Description |
---|---|
transaction | Transaction to be checked |
The default deadline (in minutes) for Transactions
Kind: static property of core
The default endpoint for [[ApiSettings]]
Kind: static property of core
Message class
The Message class is used to model a plain message attached to a transaction.
Kind: static property of core
EncryptedMessage class
The EncryptedMessage class is a model for a encrypted message attached to a transaction.
Kind: static property of core
Account class
The account class serves as a model for a Burstcoin account. It's meant to model the response from BRS API, except publicKey has been moved into the keys object.
Kind: inner class of core
Attachment class
The attachment class is used to appended to transaction where appropriate. It is a super class for Message and EncryptedMessage.
Kind: inner class of core
Original work Copyright (c) 2018 PoC-Consortium Modified work Copyright (c) 2019 Burst Apps Team
Kind: inner class of core
Constants for arbitrary subtypes
Kind: inner property of core
Constants for asset subtypes
Kind: inner property of core
Constants for escrow subtypes
Kind: inner property of core
Constants for leasing subtypes
Kind: inner property of core
Constants for marketplace subtypes
Kind: inner property of core
Constants for payment subtypes
Kind: inner property of core
Constants for reward recipient subtypes (Pool Operation)
Kind: inner property of core
Constants for smart contract (aka AT) subtypes
Kind: inner property of core
Constants for transaction types
The transaction type is part of every [[Transaction]] object and used to distinguish block data. Additionally, to the transaction type a subtype is sent, that specifies the kind of transaction more detailly.
Kind: inner property of core
The smallest possible fee
Kind: inner constant of core
Get the transaction attachment version identifier
Attachment types are identified by a field version.
Kind: inner method of core
Returns:
return Identifier, if exists, otherwise undefined
Param | Description |
---|---|
transaction | The transaction to be checked |
Checks if a transaction attachment is of specific version
Kind: inner method of core
Returns:
true, if version string matches
Param | Description |
---|---|
transaction | The transaction to be checked |
versionIdentifier | The version string, i.e. MultiOutCreation |
Extracts a variables value as hexadecimal string from a contract's machine data
This is a generic function to extract arbitrary data from a contract. I's recommended to use the [[ContractHelper]] class instead
Kind: inner method of core
Returns:
The value as hexadecimal string (already considering endianness)
Param | Default | Description |
---|---|---|
position | The variables position | |
length | 16 | The length of data to be extracted |
Deprecated
Kind: inner method of core
Returns:
HttpParams
Param | Description |
---|---|
transaction | The transaction with the attachment |
params | Some HttpParams |
Signs and broadcasts a transaction
Kind: inner method of core
Returns:
The transaction Id
Param | Description |
---|---|
unsignedTransaction | The unsigned transaction context |
service | The service used for |
Tries to extract recipients and its amounts for multi out payments (different and same amount)
Kind: inner method of core
Returns:
A list of recipients and their payed amount (in NQT)
An exception in case of wrong transaction types
Param | Description |
---|---|
transaction | The transaction |
Gets the amount from a transaction, considering ordinary and multi out transactions (with same and different payments)
Kind: inner method of core
Returns:
the amount in BURST (not NQT)
Param | Description |
---|---|
recipientId | The numeric id of the recipient |
transaction | The payment transaction |
Checks if a transaction is a multi out transaction with same amounts for each recipient
Kind: inner method of core
Returns:
true, if is a multi out transaction
Param | Description |
---|---|
transaction | Transaction to be checked |
Checks if a transaction is a multi out transaction (with different amounts)
Kind: inner method of core
Returns:
true, if is a multi out transaction
Param | Description |
---|---|
transaction | Transaction to be checked |
The default deadline (in minutes) for Transactions
Kind: static property of core
The default endpoint for [[ApiSettings]]
Kind: static property of core
Message class
The Message class is used to model a plain message attached to a transaction.
Kind: static property of core
EncryptedMessage class
The EncryptedMessage class is a model for a encrypted message attached to a transaction.
Kind: static property of core
Account class
The account class serves as a model for a Burstcoin account. It's meant to model the response from BRS API, except publicKey has been moved into the keys object.
Kind: inner class of core
Attachment class
The attachment class is used to appended to transaction where appropriate. It is a super class for Message and EncryptedMessage.
Kind: inner class of core
Original work Copyright (c) 2018 PoC-Consortium Modified work Copyright (c) 2019 Burst Apps Team
Kind: inner class of core
Constants for arbitrary subtypes
Kind: inner property of core
Constants for asset subtypes
Kind: inner property of core
Constants for escrow subtypes
Kind: inner property of core
Constants for leasing subtypes
Kind: inner property of core
Constants for marketplace subtypes
Kind: inner property of core
Constants for payment subtypes
Kind: inner property of core
Constants for reward recipient subtypes (Pool Operation)
Kind: inner property of core
Constants for smart contract (aka AT) subtypes
Kind: inner property of core
Constants for transaction types
The transaction type is part of every [[Transaction]] object and used to distinguish block data. Additionally, to the transaction type a subtype is sent, that specifies the kind of transaction more detailly.
Kind: inner property of core
The smallest possible fee
Kind: inner constant of core
Get the transaction attachment version identifier
Attachment types are identified by a field version.
Kind: inner method of core
Returns:
return Identifier, if exists, otherwise undefined
Param | Description |
---|---|
transaction | The transaction to be checked |
Checks if a transaction attachment is of specific version
Kind: inner method of core
Returns:
true, if version string matches
Param | Description |
---|---|
transaction | The transaction to be checked |
versionIdentifier | The version string, i.e. MultiOutCreation |
Extracts a variables value as hexadecimal string from a contract's machine data
This is a generic function to extract arbitrary data from a contract. I's recommended to use the [[ContractHelper]] class instead
Kind: inner method of core
Returns:
The value as hexadecimal string (already considering endianness)
Param | Default | Description |
---|---|---|
position | The variables position | |
length | 16 | The length of data to be extracted |
Deprecated
Kind: inner method of core
Returns:
HttpParams
Param | Description |
---|---|
transaction | The transaction with the attachment |
params | Some HttpParams |
Signs and broadcasts a transaction
Kind: inner method of core
Returns:
The transaction Id
Param | Description |
---|---|
unsignedTransaction | The unsigned transaction context |
service | The service used for |
Tries to extract recipients and its amounts for multi out payments (different and same amount)
Kind: inner method of core
Returns:
A list of recipients and their payed amount (in NQT)
An exception in case of wrong transaction types
Param | Description |
---|---|
transaction | The transaction |
Gets the amount from a transaction, considering ordinary and multi out transactions (with same and different payments)
Kind: inner method of core
Returns:
the amount in BURST (not NQT)
Param | Description |
---|---|
recipientId | The numeric id of the recipient |
transaction | The payment transaction |
Checks if a transaction is a multi out transaction with same amounts for each recipient
Kind: inner method of core
Returns:
true, if is a multi out transaction
Param | Description |
---|---|
transaction | Transaction to be checked |
Checks if a transaction is a multi out transaction (with different amounts)
Kind: inner method of core
Returns:
true, if is a multi out transaction
Param | Description |
---|---|
transaction | Transaction to be checked |
Settings for API used in [[composeApi]]
Param | Type | Description |
---|---|---|
nodeHost | string | The url of the Burst peer |
apiVersion | ApiVersion | For future usage. |
httpOptions | any | AxiosRequestSettings | Optional http options, like additional header. The default implementation uses axios. In case of a custom client pass your own options. see Axios Configuration |
Helper class for contracts
A contract owns additional data, which is splitted in 8 byte blocks. The content is encoded in hexadecimal representation and big endianness. This helper class facilitates access to these data
Kind: global class
Kind: instance method of ContractHelper
Returns:
Get the contract
Get a variable as string
Kind: instance method of ContractHelper
Returns:
The data as string (Utf-8)
Param | Description |
---|---|
index | The index of variable (starting at 0) |
Get multiple data blocks as string
Kind: instance method of ContractHelper
Returns:
The data as string (Utf-8)
Param | Description |
---|---|
index | The index of variable (starting at 0) |
count | Number of blocks |
Get a variable as decimal (string)
Kind: instance method of ContractHelper
Returns:
The data as a decimal string sequence
Param | Description |
---|---|
index | The index of variable (starting at 0) |
Get a variable at given position/index
Kind: instance method of ContractHelper
Returns:
The data as hexadecimal string (in little endianness)
Param | Description |
---|---|
index | The index of variable (starting at 0) |
Get a hexadecimal data block of arbitrary length at given position/index
Kind: instance method of ContractHelper
Returns:
The data as hexadecimal string (in little endianness)
Param | Description |
---|---|
index | The index of variable (starting at 0) |
length | The length of the data block (must be a multiple of 2) |
Generic BRS Web Service class.
Kind: global class
string
Promise.<T>
Promise.<T>
Creates Service instance
Param | Description |
---|---|
settings | The settings for the service |
string
Mounts a BRS conform API (V1) endpoint of format <host>?requestType=getBlock&height=123
Kind: instance method of BurstService
Returns: string
-
The mounted url (without host)
Param | Type | Description |
---|---|---|
method | string | The method name for |
data | any | A JSON object which will be mapped to url params |
Promise.<T>
Requests a query to BRS
Kind: instance method of BurstService
Returns: Promise.<T>
-
The response data of success
HttpError in case of failure
Param | Type | Description |
---|---|---|
method | string | The BRS method according https://burstwiki.org/wiki/The_Burst_API |
args | any | A JSON object which will be mapped to url params |
options | any | AxiosRequestConfig | The optional request configuration for the passed Http client |
Promise.<T>
Send data to BRS
Kind: instance method of BurstService
Returns: Promise.<T>
-
The response data of success
HttpError in case of failure
Param | Type | Description |
---|---|---|
method | string | The BRS method according https://burstwiki.org/wiki/The_Burst_API. Note that there are only a few POST methods |
args | any | A JSON object which will be mapped to url params |
body | any | An object with key value pairs to submit as post body |
options | any | AxiosRequestConfig | The optional request configuration for the passed Http client |
Composes the API, i.e. setup the environment and mounts the API structure with its functions.
const api = composeApi({
nodeHost: 'https://wallet1.burst-team.us:2083', // one of the mainnet nodes
})
Note, that this method mounts the entire API, i.e. all available methods. One may also customize the API composition using [[ApiComposer]].
Kind: global variable
Returns:
The complete API
Param | Description |
---|---|
settings | necessary execution context |
Param | Type | Description |
---|---|---|
nodeHost | string | The url of the Burst peer |
apiVersion | ApiVersion | For future usage. |
httpOptions | any | AxiosRequestSettings | Optional http options, like additional header. The default implementation uses axios. In case of a custom client pass your own options. see Axios Configuration |
Creates BRS Http send parameters for a transaction from attachment data
Kind: global function
Returns:
HttpParams
Param | Description |
---|---|
attachment | The attachment |
params | Any object |
FAQs
Burst-related functions and models for building Burstcoin applications.
The npm package @burstjs/core receives a total of 7 weekly downloads. As such, @burstjs/core popularity was classified as not popular.
We found that @burstjs/core demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.