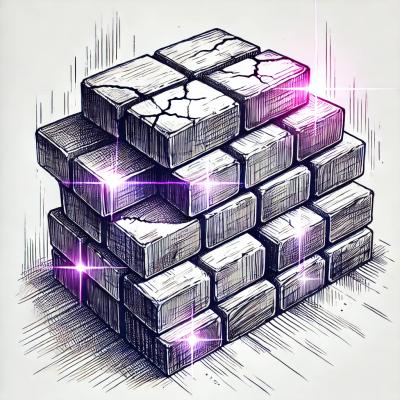
Security News
Input Validation Vulnerabilities Dominate MITRE's 2024 CWE Top 25 List
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
@burstjs/core
Advanced tools
Burst-related functions and models for building Burstcoin applications.
Burst-related functions and models for building Burstcoin applications.
burstJS can be used with NodeJS or Web. Two formats are available
Install using npm:
npm install @burstjs/core
or using yarn:
yarn add @burstjs/core
import {composeApi, ApiSettings} from '@burstjs/core'
import {BurstValue} from '@burstjs/util'
const apiSettings = new ApiSettings('https://testnet.burstcoin.network:6876');
const api = composeApi(apiSettings);
// this self-executing file makes turns this file into a starting point of your app
(async () => {
try{
const {balanceNQT} = await api.account.getAccountBalance('13036514135565182944')
console.log(`Account Balance: ${BurstValue.fromPlanck(balanceNQT).toString()}`)
}
catch(e){ // e is of type HttpError (as part of @burstjs/http)
console.error(`Whooops, something went wrong: ${e.message}`)
}
})()
<script>
Each package is available as bundled standalone library using IIFE.
This way burstJS can be used also within <script>
-Tags.
This might be useful for Wordpress and/or other PHP applications.
Just import the package using the HTML <script>
tag.
<script src='https://cdn.jsdelivr.net/npm/@burstjs/core/dist/burstjs.min.js'></script>
(function(){
const api = b$.composeApi({nodeHost: "https://testnet.burstcoin.network:6876"});
api.network.getBlockchainStatus().then(console.log).catch(console.error);
})()
See more here:
@burstjs/core Online Documentation
The API composer mounts the API for given service and selected methods
Usually you would use [[composeApi]], which gives you all available API methods. Unfortunately, this will import almost all dependencies, even if you need only a fraction of the methods. To take advantage of tree-shaking (dead code elimination) you can compose your own API with the methods you need. This can reduce your final bundle significantly.
Usage:
const burstService = new BurstService({ nodeHost: 'https://testnet.burst.fun', })
const api = apiComposer .create(burstService) .withMessageApi({ sendTextMessage }) .withAccountApi({ getAccountTransactions, getUnconfirmedAccountTransactions, getAccountBalance, generateSendTransactionQRCode, generateSendTransactionQRCodeAddress, }) .compose();
The with<section>Api
uses factory methods from the api.core.factories package
Settings for API used in [[composeApi]]
Composes the API, i.e. setup the environment and mounts the API structure with its functions.
const api = composeApi(new ApiSettings('https://wallet1.burst-team.us:2083')), // one of the mainnet nodes
Note, that this method mounts the entire API, i.e. all available methods. One may also customize the API composition using [[ApiComposer]].
Get the transaction attachment version identifier
Attachment types are identified by a field version.
Checks if a transaction attachment is of specific version
The default deadline (in minutes) for Transactions
The default endpoint for [[ApiSettings]]
Constants for arbitrary subtypes
Constants for asset subtypes
Constants for escrow subtypes
Constants for leasing subtypes
Constants for marketplace subtypes
Constants for payment subtypes
Constants for reward recipient subtypes (Pool Operation)
Constants for smart contract (aka AT) subtypes
Constants for transaction types
The transaction type is part of every [[Transaction]] object and used to distinguish block data. Additionally, to the transaction type a subtype is sent, that specifies the kind of transaction more detailly.
Constructs an Attachment
Creates BRS Http send parameters for a transaction from attachment data
Generic BRS Web Service class.
Tries to extract recipients and its amounts for multi out payments (different and same amount)
Gets the amount from a transaction, considering ordinary and multi out transactions (with same and different payments)
Checks if a transaction is a multi out transaction with same amounts for each recipient
Checks if a transaction is a multi out transaction (with different amounts)
Account class
The account class serves as a model for a Burstcoin account. It's meant to model the response from BRS API, except publicKey has been moved into the keys object.
Attachment class
The attachment class is used to appended to transaction where appropriate. It is a super class for Message and EncryptedMessage.
Message class
The Message class is used to model a plain message attached to a transaction.
EncryptedMessage class
The EncryptedMessage class is a model for a encrypted message attached to a transaction.
Copyright (c) 2019 Burst Apps Team
The API composer mounts the API for given service and selected methods
Usually you would use [[composeApi]], which gives you all available API methods. Unfortunately, this will import almost all dependencies, even if you need only a fraction of the methods. To take advantage of tree-shaking (dead code elimination) you can compose your own API with the methods you need. This can reduce your final bundle significantly.
Usage:
const burstService = new BurstService({ nodeHost: 'https://testnet.burst.fun', })
const api = apiComposer .create(burstService) .withMessageApi({ sendTextMessage }) .withAccountApi({ getAccountTransactions, getUnconfirmedAccountTransactions, getAccountBalance, generateSendTransactionQRCode, generateSendTransactionQRCodeAddress, }) .compose();
The with<section>Api
uses factory methods from the api.core.factories package
Kind: inner class of api
Param | Type | Description |
---|---|---|
nodeHost | string | The url of the Burst peer |
apiVersion | ApiVersion | For future usage. |
httpClientOptions | any | AxiosRequestSettings | Optional http options, like additional header. The default implementation uses axios. In case of a custom client pass your own options. see Axios Configuration |
Settings for API used in [[composeApi]]
Kind: inner class of api
Param | Type | Description |
---|---|---|
nodeHost | string | The url of the Burst peer |
apiVersion | ApiVersion | For future usage. |
httpClientOptions | any | AxiosRequestSettings | Optional http options, like additional header. The default implementation uses axios. In case of a custom client pass your own options. see Axios Configuration |
Composes the API, i.e. setup the environment and mounts the API structure with its functions.
const api = composeApi(new ApiSettings('https://wallet1.burst-team.us:2083')), // one of the mainnet nodes
Note, that this method mounts the entire API, i.e. all available methods. One may also customize the API composition using [[ApiComposer]].
Returns:
The complete API
Param | Description |
---|---|
settings | necessary execution context |
Kind: inner class of api
Param | Type | Description |
---|---|---|
nodeHost | string | The url of the Burst peer |
apiVersion | ApiVersion | For future usage. |
httpClientOptions | any | AxiosRequestSettings | Optional http options, like additional header. The default implementation uses axios. In case of a custom client pass your own options. see Axios Configuration |
Get the transaction attachment version identifier
Attachment types are identified by a field version.
Returns:
return Identifier, if exists, otherwise undefined
Param | Description |
---|---|
transaction | The transaction to be checked |
string
Promise.<T>
Promise.<T>
Kind: inner class of core
string
Promise.<T>
Promise.<T>
Creates Service instance
Param | Description |
---|---|
settings | The settings for the service |
string
Mounts a BRS conform API (V1) endpoint of format <host>?requestType=getBlock&height=123
Kind: instance method of BurstService
Returns: string
-
The mounted url (without host)
Param | Type | Description |
---|---|---|
method | string | The method name for |
data | any | A JSON object which will be mapped to url params |
Promise.<T>
Requests a query to BRS
Kind: instance method of BurstService
Returns: Promise.<T>
-
The response data of success
HttpError in case of failure
Param | Type | Description |
---|---|---|
method | string | The BRS method according https://burstwiki.org/wiki/The_Burst_API |
args | any | A JSON object which will be mapped to url params |
options | any | AxiosRequestConfig | The optional request configuration for the passed Http client |
Promise.<T>
Send data to BRS
Kind: instance method of BurstService
Returns: Promise.<T>
-
The response data of success
HttpError in case of failure
Param | Type | Description |
---|---|---|
method | string | The BRS method according https://burstwiki.org/wiki/The_Burst_API. Note that there are only a few POST methods |
args | any | A JSON object which will be mapped to url params |
body | any | An object with key value pairs to submit as post body |
options | any | AxiosRequestConfig | The optional request configuration for the passed Http client |
Checks if a transaction attachment is of specific version
Returns:
true, if version string matches
Param | Description |
---|---|
transaction | The transaction to be checked |
versionIdentifier | The version string, i.e. MultiOutCreation |
string
Promise.<T>
Promise.<T>
Kind: inner class of core
string
Promise.<T>
Promise.<T>
Creates Service instance
Param | Description |
---|---|
settings | The settings for the service |
string
Mounts a BRS conform API (V1) endpoint of format <host>?requestType=getBlock&height=123
Kind: instance method of BurstService
Returns: string
-
The mounted url (without host)
Param | Type | Description |
---|---|---|
method | string | The method name for |
data | any | A JSON object which will be mapped to url params |
Promise.<T>
Requests a query to BRS
Kind: instance method of BurstService
Returns: Promise.<T>
-
The response data of success
HttpError in case of failure
Param | Type | Description |
---|---|---|
method | string | The BRS method according https://burstwiki.org/wiki/The_Burst_API |
args | any | A JSON object which will be mapped to url params |
options | any | AxiosRequestConfig | The optional request configuration for the passed Http client |
Promise.<T>
Send data to BRS
Kind: instance method of BurstService
Returns: Promise.<T>
-
The response data of success
HttpError in case of failure
Param | Type | Description |
---|---|---|
method | string | The BRS method according https://burstwiki.org/wiki/The_Burst_API. Note that there are only a few POST methods |
args | any | A JSON object which will be mapped to url params |
body | any | An object with key value pairs to submit as post body |
options | any | AxiosRequestConfig | The optional request configuration for the passed Http client |
string
Promise.<T>
Promise.<T>
Kind: inner class of core
string
Promise.<T>
Promise.<T>
Creates Service instance
Param | Description |
---|---|
settings | The settings for the service |
string
Mounts a BRS conform API (V1) endpoint of format <host>?requestType=getBlock&height=123
Kind: instance method of BurstService
Returns: string
-
The mounted url (without host)
Param | Type | Description |
---|---|---|
method | string | The method name for |
data | any | A JSON object which will be mapped to url params |
Promise.<T>
Requests a query to BRS
Kind: instance method of BurstService
Returns: Promise.<T>
-
The response data of success
HttpError in case of failure
Param | Type | Description |
---|---|---|
method | string | The BRS method according https://burstwiki.org/wiki/The_Burst_API |
args | any | A JSON object which will be mapped to url params |
options | any | AxiosRequestConfig | The optional request configuration for the passed Http client |
Promise.<T>
Send data to BRS
Kind: instance method of BurstService
Returns: Promise.<T>
-
The response data of success
HttpError in case of failure
Param | Type | Description |
---|---|---|
method | string | The BRS method according https://burstwiki.org/wiki/The_Burst_API. Note that there are only a few POST methods |
args | any | A JSON object which will be mapped to url params |
body | any | An object with key value pairs to submit as post body |
options | any | AxiosRequestConfig | The optional request configuration for the passed Http client |
The default deadline (in minutes) for Transactions
string
Promise.<T>
Promise.<T>
Kind: inner class of core
string
Promise.<T>
Promise.<T>
Creates Service instance
Param | Description |
---|---|
settings | The settings for the service |
string
Mounts a BRS conform API (V1) endpoint of format <host>?requestType=getBlock&height=123
Kind: instance method of BurstService
Returns: string
-
The mounted url (without host)
Param | Type | Description |
---|---|---|
method | string | The method name for |
data | any | A JSON object which will be mapped to url params |
Promise.<T>
Requests a query to BRS
Kind: instance method of BurstService
Returns: Promise.<T>
-
The response data of success
HttpError in case of failure
Param | Type | Description |
---|---|---|
method | string | The BRS method according https://burstwiki.org/wiki/The_Burst_API |
args | any | A JSON object which will be mapped to url params |
options | any | AxiosRequestConfig | The optional request configuration for the passed Http client |
Promise.<T>
Send data to BRS
Kind: instance method of BurstService
Returns: Promise.<T>
-
The response data of success
HttpError in case of failure
Param | Type | Description |
---|---|---|
method | string | The BRS method according https://burstwiki.org/wiki/The_Burst_API. Note that there are only a few POST methods |
args | any | A JSON object which will be mapped to url params |
body | any | An object with key value pairs to submit as post body |
options | any | AxiosRequestConfig | The optional request configuration for the passed Http client |
The default endpoint for [[ApiSettings]]
string
Promise.<T>
Promise.<T>
Kind: inner class of core
string
Promise.<T>
Promise.<T>
Creates Service instance
Param | Description |
---|---|
settings | The settings for the service |
string
Mounts a BRS conform API (V1) endpoint of format <host>?requestType=getBlock&height=123
Kind: instance method of BurstService
Returns: string
-
The mounted url (without host)
Param | Type | Description |
---|---|---|
method | string | The method name for |
data | any | A JSON object which will be mapped to url params |
Promise.<T>
Requests a query to BRS
Kind: instance method of BurstService
Returns: Promise.<T>
-
The response data of success
HttpError in case of failure
Param | Type | Description |
---|---|---|
method | string | The BRS method according https://burstwiki.org/wiki/The_Burst_API |
args | any | A JSON object which will be mapped to url params |
options | any | AxiosRequestConfig | The optional request configuration for the passed Http client |
Promise.<T>
Send data to BRS
Kind: instance method of BurstService
Returns: Promise.<T>
-
The response data of success
HttpError in case of failure
Param | Type | Description |
---|---|---|
method | string | The BRS method according https://burstwiki.org/wiki/The_Burst_API. Note that there are only a few POST methods |
args | any | A JSON object which will be mapped to url params |
body | any | An object with key value pairs to submit as post body |
options | any | AxiosRequestConfig | The optional request configuration for the passed Http client |
Constants for arbitrary subtypes
string
Promise.<T>
Promise.<T>
Kind: inner class of core
string
Promise.<T>
Promise.<T>
Creates Service instance
Param | Description |
---|---|
settings | The settings for the service |
string
Mounts a BRS conform API (V1) endpoint of format <host>?requestType=getBlock&height=123
Kind: instance method of BurstService
Returns: string
-
The mounted url (without host)
Param | Type | Description |
---|---|---|
method | string | The method name for |
data | any | A JSON object which will be mapped to url params |
Promise.<T>
Requests a query to BRS
Kind: instance method of BurstService
Returns: Promise.<T>
-
The response data of success
HttpError in case of failure
Param | Type | Description |
---|---|---|
method | string | The BRS method according https://burstwiki.org/wiki/The_Burst_API |
args | any | A JSON object which will be mapped to url params |
options | any | AxiosRequestConfig | The optional request configuration for the passed Http client |
Promise.<T>
Send data to BRS
Kind: instance method of BurstService
Returns: Promise.<T>
-
The response data of success
HttpError in case of failure
Param | Type | Description |
---|---|---|
method | string | The BRS method according https://burstwiki.org/wiki/The_Burst_API. Note that there are only a few POST methods |
args | any | A JSON object which will be mapped to url params |
body | any | An object with key value pairs to submit as post body |
options | any | AxiosRequestConfig | The optional request configuration for the passed Http client |
Constants for asset subtypes
string
Promise.<T>
Promise.<T>
Kind: inner class of core
string
Promise.<T>
Promise.<T>
Creates Service instance
Param | Description |
---|---|
settings | The settings for the service |
string
Mounts a BRS conform API (V1) endpoint of format <host>?requestType=getBlock&height=123
Kind: instance method of BurstService
Returns: string
-
The mounted url (without host)
Param | Type | Description |
---|---|---|
method | string | The method name for |
data | any | A JSON object which will be mapped to url params |
Promise.<T>
Requests a query to BRS
Kind: instance method of BurstService
Returns: Promise.<T>
-
The response data of success
HttpError in case of failure
Param | Type | Description |
---|---|---|
method | string | The BRS method according https://burstwiki.org/wiki/The_Burst_API |
args | any | A JSON object which will be mapped to url params |
options | any | AxiosRequestConfig | The optional request configuration for the passed Http client |
Promise.<T>
Send data to BRS
Kind: instance method of BurstService
Returns: Promise.<T>
-
The response data of success
HttpError in case of failure
Param | Type | Description |
---|---|---|
method | string | The BRS method according https://burstwiki.org/wiki/The_Burst_API. Note that there are only a few POST methods |
args | any | A JSON object which will be mapped to url params |
body | any | An object with key value pairs to submit as post body |
options | any | AxiosRequestConfig | The optional request configuration for the passed Http client |
Constants for escrow subtypes
string
Promise.<T>
Promise.<T>
Kind: inner class of core
string
Promise.<T>
Promise.<T>
Creates Service instance
Param | Description |
---|---|
settings | The settings for the service |
string
Mounts a BRS conform API (V1) endpoint of format <host>?requestType=getBlock&height=123
Kind: instance method of BurstService
Returns: string
-
The mounted url (without host)
Param | Type | Description |
---|---|---|
method | string | The method name for |
data | any | A JSON object which will be mapped to url params |
Promise.<T>
Requests a query to BRS
Kind: instance method of BurstService
Returns: Promise.<T>
-
The response data of success
HttpError in case of failure
Param | Type | Description |
---|---|---|
method | string | The BRS method according https://burstwiki.org/wiki/The_Burst_API |
args | any | A JSON object which will be mapped to url params |
options | any | AxiosRequestConfig | The optional request configuration for the passed Http client |
Promise.<T>
Send data to BRS
Kind: instance method of BurstService
Returns: Promise.<T>
-
The response data of success
HttpError in case of failure
Param | Type | Description |
---|---|---|
method | string | The BRS method according https://burstwiki.org/wiki/The_Burst_API. Note that there are only a few POST methods |
args | any | A JSON object which will be mapped to url params |
body | any | An object with key value pairs to submit as post body |
options | any | AxiosRequestConfig | The optional request configuration for the passed Http client |
Constants for leasing subtypes
string
Promise.<T>
Promise.<T>
Kind: inner class of core
string
Promise.<T>
Promise.<T>
Creates Service instance
Param | Description |
---|---|
settings | The settings for the service |
string
Mounts a BRS conform API (V1) endpoint of format <host>?requestType=getBlock&height=123
Kind: instance method of BurstService
Returns: string
-
The mounted url (without host)
Param | Type | Description |
---|---|---|
method | string | The method name for |
data | any | A JSON object which will be mapped to url params |
Promise.<T>
Requests a query to BRS
Kind: instance method of BurstService
Returns: Promise.<T>
-
The response data of success
HttpError in case of failure
Param | Type | Description |
---|---|---|
method | string | The BRS method according https://burstwiki.org/wiki/The_Burst_API |
args | any | A JSON object which will be mapped to url params |
options | any | AxiosRequestConfig | The optional request configuration for the passed Http client |
Promise.<T>
Send data to BRS
Kind: instance method of BurstService
Returns: Promise.<T>
-
The response data of success
HttpError in case of failure
Param | Type | Description |
---|---|---|
method | string | The BRS method according https://burstwiki.org/wiki/The_Burst_API. Note that there are only a few POST methods |
args | any | A JSON object which will be mapped to url params |
body | any | An object with key value pairs to submit as post body |
options | any | AxiosRequestConfig | The optional request configuration for the passed Http client |
Constants for marketplace subtypes
string
Promise.<T>
Promise.<T>
Kind: inner class of core
string
Promise.<T>
Promise.<T>
Creates Service instance
Param | Description |
---|---|
settings | The settings for the service |
string
Mounts a BRS conform API (V1) endpoint of format <host>?requestType=getBlock&height=123
Kind: instance method of BurstService
Returns: string
-
The mounted url (without host)
Param | Type | Description |
---|---|---|
method | string | The method name for |
data | any | A JSON object which will be mapped to url params |
Promise.<T>
Requests a query to BRS
Kind: instance method of BurstService
Returns: Promise.<T>
-
The response data of success
HttpError in case of failure
Param | Type | Description |
---|---|---|
method | string | The BRS method according https://burstwiki.org/wiki/The_Burst_API |
args | any | A JSON object which will be mapped to url params |
options | any | AxiosRequestConfig | The optional request configuration for the passed Http client |
Promise.<T>
Send data to BRS
Kind: instance method of BurstService
Returns: Promise.<T>
-
The response data of success
HttpError in case of failure
Param | Type | Description |
---|---|---|
method | string | The BRS method according https://burstwiki.org/wiki/The_Burst_API. Note that there are only a few POST methods |
args | any | A JSON object which will be mapped to url params |
body | any | An object with key value pairs to submit as post body |
options | any | AxiosRequestConfig | The optional request configuration for the passed Http client |
Constants for payment subtypes
string
Promise.<T>
Promise.<T>
Kind: inner class of core
string
Promise.<T>
Promise.<T>
Creates Service instance
Param | Description |
---|---|
settings | The settings for the service |
string
Mounts a BRS conform API (V1) endpoint of format <host>?requestType=getBlock&height=123
Kind: instance method of BurstService
Returns: string
-
The mounted url (without host)
Param | Type | Description |
---|---|---|
method | string | The method name for |
data | any | A JSON object which will be mapped to url params |
Promise.<T>
Requests a query to BRS
Kind: instance method of BurstService
Returns: Promise.<T>
-
The response data of success
HttpError in case of failure
Param | Type | Description |
---|---|---|
method | string | The BRS method according https://burstwiki.org/wiki/The_Burst_API |
args | any | A JSON object which will be mapped to url params |
options | any | AxiosRequestConfig | The optional request configuration for the passed Http client |
Promise.<T>
Send data to BRS
Kind: instance method of BurstService
Returns: Promise.<T>
-
The response data of success
HttpError in case of failure
Param | Type | Description |
---|---|---|
method | string | The BRS method according https://burstwiki.org/wiki/The_Burst_API. Note that there are only a few POST methods |
args | any | A JSON object which will be mapped to url params |
body | any | An object with key value pairs to submit as post body |
options | any | AxiosRequestConfig | The optional request configuration for the passed Http client |
Constants for reward recipient subtypes (Pool Operation)
string
Promise.<T>
Promise.<T>
Kind: inner class of core
string
Promise.<T>
Promise.<T>
Creates Service instance
Param | Description |
---|---|
settings | The settings for the service |
string
Mounts a BRS conform API (V1) endpoint of format <host>?requestType=getBlock&height=123
Kind: instance method of BurstService
Returns: string
-
The mounted url (without host)
Param | Type | Description |
---|---|---|
method | string | The method name for |
data | any | A JSON object which will be mapped to url params |
Promise.<T>
Requests a query to BRS
Kind: instance method of BurstService
Returns: Promise.<T>
-
The response data of success
HttpError in case of failure
Param | Type | Description |
---|---|---|
method | string | The BRS method according https://burstwiki.org/wiki/The_Burst_API |
args | any | A JSON object which will be mapped to url params |
options | any | AxiosRequestConfig | The optional request configuration for the passed Http client |
Promise.<T>
Send data to BRS
Kind: instance method of BurstService
Returns: Promise.<T>
-
The response data of success
HttpError in case of failure
Param | Type | Description |
---|---|---|
method | string | The BRS method according https://burstwiki.org/wiki/The_Burst_API. Note that there are only a few POST methods |
args | any | A JSON object which will be mapped to url params |
body | any | An object with key value pairs to submit as post body |
options | any | AxiosRequestConfig | The optional request configuration for the passed Http client |
Constants for smart contract (aka AT) subtypes
string
Promise.<T>
Promise.<T>
Kind: inner class of core
string
Promise.<T>
Promise.<T>
Creates Service instance
Param | Description |
---|---|
settings | The settings for the service |
string
Mounts a BRS conform API (V1) endpoint of format <host>?requestType=getBlock&height=123
Kind: instance method of BurstService
Returns: string
-
The mounted url (without host)
Param | Type | Description |
---|---|---|
method | string | The method name for |
data | any | A JSON object which will be mapped to url params |
Promise.<T>
Requests a query to BRS
Kind: instance method of BurstService
Returns: Promise.<T>
-
The response data of success
HttpError in case of failure
Param | Type | Description |
---|---|---|
method | string | The BRS method according https://burstwiki.org/wiki/The_Burst_API |
args | any | A JSON object which will be mapped to url params |
options | any | AxiosRequestConfig | The optional request configuration for the passed Http client |
Promise.<T>
Send data to BRS
Kind: instance method of BurstService
Returns: Promise.<T>
-
The response data of success
HttpError in case of failure
Param | Type | Description |
---|---|---|
method | string | The BRS method according https://burstwiki.org/wiki/The_Burst_API. Note that there are only a few POST methods |
args | any | A JSON object which will be mapped to url params |
body | any | An object with key value pairs to submit as post body |
options | any | AxiosRequestConfig | The optional request configuration for the passed Http client |
Constants for transaction types
The transaction type is part of every [[Transaction]] object and used to distinguish block data. Additionally, to the transaction type a subtype is sent, that specifies the kind of transaction more detailly.
string
Promise.<T>
Promise.<T>
Kind: inner class of core
string
Promise.<T>
Promise.<T>
Creates Service instance
Param | Description |
---|---|
settings | The settings for the service |
string
Mounts a BRS conform API (V1) endpoint of format <host>?requestType=getBlock&height=123
Kind: instance method of BurstService
Returns: string
-
The mounted url (without host)
Param | Type | Description |
---|---|---|
method | string | The method name for |
data | any | A JSON object which will be mapped to url params |
Promise.<T>
Requests a query to BRS
Kind: instance method of BurstService
Returns: Promise.<T>
-
The response data of success
HttpError in case of failure
Param | Type | Description |
---|---|---|
method | string | The BRS method according https://burstwiki.org/wiki/The_Burst_API |
args | any | A JSON object which will be mapped to url params |
options | any | AxiosRequestConfig | The optional request configuration for the passed Http client |
Promise.<T>
Send data to BRS
Kind: instance method of BurstService
Returns: Promise.<T>
-
The response data of success
HttpError in case of failure
Param | Type | Description |
---|---|---|
method | string | The BRS method according https://burstwiki.org/wiki/The_Burst_API. Note that there are only a few POST methods |
args | any | A JSON object which will be mapped to url params |
body | any | An object with key value pairs to submit as post body |
options | any | AxiosRequestConfig | The optional request configuration for the passed Http client |
Deprecated
Constructs an Attachment
Returns:
HttpParams
Param | Description |
---|---|
transaction | The transaction with the attachment |
params | Some HttpParams |
string
Promise.<T>
Promise.<T>
Kind: inner class of core
string
Promise.<T>
Promise.<T>
Creates Service instance
Param | Description |
---|---|
settings | The settings for the service |
string
Mounts a BRS conform API (V1) endpoint of format <host>?requestType=getBlock&height=123
Kind: instance method of BurstService
Returns: string
-
The mounted url (without host)
Param | Type | Description |
---|---|---|
method | string | The method name for |
data | any | A JSON object which will be mapped to url params |
Promise.<T>
Requests a query to BRS
Kind: instance method of BurstService
Returns: Promise.<T>
-
The response data of success
HttpError in case of failure
Param | Type | Description |
---|---|---|
method | string | The BRS method according https://burstwiki.org/wiki/The_Burst_API |
args | any | A JSON object which will be mapped to url params |
options | any | AxiosRequestConfig | The optional request configuration for the passed Http client |
Promise.<T>
Send data to BRS
Kind: instance method of BurstService
Returns: Promise.<T>
-
The response data of success
HttpError in case of failure
Param | Type | Description |
---|---|---|
method | string | The BRS method according https://burstwiki.org/wiki/The_Burst_API. Note that there are only a few POST methods |
args | any | A JSON object which will be mapped to url params |
body | any | An object with key value pairs to submit as post body |
options | any | AxiosRequestConfig | The optional request configuration for the passed Http client |
Creates BRS Http send parameters for a transaction from attachment data
Returns:
HttpParams
Param | Description |
---|---|
attachment | The attachment |
params | Any object |
string
Promise.<T>
Promise.<T>
Kind: inner class of core
string
Promise.<T>
Promise.<T>
Creates Service instance
Param | Description |
---|---|
settings | The settings for the service |
string
Mounts a BRS conform API (V1) endpoint of format <host>?requestType=getBlock&height=123
Kind: instance method of BurstService
Returns: string
-
The mounted url (without host)
Param | Type | Description |
---|---|---|
method | string | The method name for |
data | any | A JSON object which will be mapped to url params |
Promise.<T>
Requests a query to BRS
Kind: instance method of BurstService
Returns: Promise.<T>
-
The response data of success
HttpError in case of failure
Param | Type | Description |
---|---|---|
method | string | The BRS method according https://burstwiki.org/wiki/The_Burst_API |
args | any | A JSON object which will be mapped to url params |
options | any | AxiosRequestConfig | The optional request configuration for the passed Http client |
Promise.<T>
Send data to BRS
Kind: instance method of BurstService
Returns: Promise.<T>
-
The response data of success
HttpError in case of failure
Param | Type | Description |
---|---|---|
method | string | The BRS method according https://burstwiki.org/wiki/The_Burst_API. Note that there are only a few POST methods |
args | any | A JSON object which will be mapped to url params |
body | any | An object with key value pairs to submit as post body |
options | any | AxiosRequestConfig | The optional request configuration for the passed Http client |
Generic BRS Web Service class.
string
Promise.<T>
Promise.<T>
Kind: inner class of core
string
Promise.<T>
Promise.<T>
Creates Service instance
Param | Description |
---|---|
settings | The settings for the service |
string
Mounts a BRS conform API (V1) endpoint of format <host>?requestType=getBlock&height=123
Kind: instance method of BurstService
Returns: string
-
The mounted url (without host)
Param | Type | Description |
---|---|---|
method | string | The method name for |
data | any | A JSON object which will be mapped to url params |
Promise.<T>
Requests a query to BRS
Kind: instance method of BurstService
Returns: Promise.<T>
-
The response data of success
HttpError in case of failure
Param | Type | Description |
---|---|---|
method | string | The BRS method according https://burstwiki.org/wiki/The_Burst_API |
args | any | A JSON object which will be mapped to url params |
options | any | AxiosRequestConfig | The optional request configuration for the passed Http client |
Promise.<T>
Send data to BRS
Kind: instance method of BurstService
Returns: Promise.<T>
-
The response data of success
HttpError in case of failure
Param | Type | Description |
---|---|---|
method | string | The BRS method according https://burstwiki.org/wiki/The_Burst_API. Note that there are only a few POST methods |
args | any | A JSON object which will be mapped to url params |
body | any | An object with key value pairs to submit as post body |
options | any | AxiosRequestConfig | The optional request configuration for the passed Http client |
Tries to extract recipients and its amounts for multi out payments (different and same amount)
Returns:
A list of recipients and their payed amount (in NQT)
An exception in case of wrong transaction types
Param | Description |
---|---|
transaction | The transaction |
string
Promise.<T>
Promise.<T>
Kind: inner class of core
string
Promise.<T>
Promise.<T>
Creates Service instance
Param | Description |
---|---|
settings | The settings for the service |
string
Mounts a BRS conform API (V1) endpoint of format <host>?requestType=getBlock&height=123
Kind: instance method of BurstService
Returns: string
-
The mounted url (without host)
Param | Type | Description |
---|---|---|
method | string | The method name for |
data | any | A JSON object which will be mapped to url params |
Promise.<T>
Requests a query to BRS
Kind: instance method of BurstService
Returns: Promise.<T>
-
The response data of success
HttpError in case of failure
Param | Type | Description |
---|---|---|
method | string | The BRS method according https://burstwiki.org/wiki/The_Burst_API |
args | any | A JSON object which will be mapped to url params |
options | any | AxiosRequestConfig | The optional request configuration for the passed Http client |
Promise.<T>
Send data to BRS
Kind: instance method of BurstService
Returns: Promise.<T>
-
The response data of success
HttpError in case of failure
Param | Type | Description |
---|---|---|
method | string | The BRS method according https://burstwiki.org/wiki/The_Burst_API. Note that there are only a few POST methods |
args | any | A JSON object which will be mapped to url params |
body | any | An object with key value pairs to submit as post body |
options | any | AxiosRequestConfig | The optional request configuration for the passed Http client |
Gets the amount from a transaction, considering ordinary and multi out transactions (with same and different payments)
Returns:
the amount in BURST (not NQT)
Param | Description |
---|---|
recipientId | The numeric id of the recipient |
transaction | The payment transaction |
string
Promise.<T>
Promise.<T>
Kind: inner class of core
string
Promise.<T>
Promise.<T>
Creates Service instance
Param | Description |
---|---|
settings | The settings for the service |
string
Mounts a BRS conform API (V1) endpoint of format <host>?requestType=getBlock&height=123
Kind: instance method of BurstService
Returns: string
-
The mounted url (without host)
Param | Type | Description |
---|---|---|
method | string | The method name for |
data | any | A JSON object which will be mapped to url params |
Promise.<T>
Requests a query to BRS
Kind: instance method of BurstService
Returns: Promise.<T>
-
The response data of success
HttpError in case of failure
Param | Type | Description |
---|---|---|
method | string | The BRS method according https://burstwiki.org/wiki/The_Burst_API |
args | any | A JSON object which will be mapped to url params |
options | any | AxiosRequestConfig | The optional request configuration for the passed Http client |
Promise.<T>
Send data to BRS
Kind: instance method of BurstService
Returns: Promise.<T>
-
The response data of success
HttpError in case of failure
Param | Type | Description |
---|---|---|
method | string | The BRS method according https://burstwiki.org/wiki/The_Burst_API. Note that there are only a few POST methods |
args | any | A JSON object which will be mapped to url params |
body | any | An object with key value pairs to submit as post body |
options | any | AxiosRequestConfig | The optional request configuration for the passed Http client |
Checks if a transaction is a multi out transaction with same amounts for each recipient
Returns:
true, if is a multi out transaction
Param | Description |
---|---|
transaction | Transaction to be checked |
string
Promise.<T>
Promise.<T>
Kind: inner class of core
string
Promise.<T>
Promise.<T>
Creates Service instance
Param | Description |
---|---|
settings | The settings for the service |
string
Mounts a BRS conform API (V1) endpoint of format <host>?requestType=getBlock&height=123
Kind: instance method of BurstService
Returns: string
-
The mounted url (without host)
Param | Type | Description |
---|---|---|
method | string | The method name for |
data | any | A JSON object which will be mapped to url params |
Promise.<T>
Requests a query to BRS
Kind: instance method of BurstService
Returns: Promise.<T>
-
The response data of success
HttpError in case of failure
Param | Type | Description |
---|---|---|
method | string | The BRS method according https://burstwiki.org/wiki/The_Burst_API |
args | any | A JSON object which will be mapped to url params |
options | any | AxiosRequestConfig | The optional request configuration for the passed Http client |
Promise.<T>
Send data to BRS
Kind: instance method of BurstService
Returns: Promise.<T>
-
The response data of success
HttpError in case of failure
Param | Type | Description |
---|---|---|
method | string | The BRS method according https://burstwiki.org/wiki/The_Burst_API. Note that there are only a few POST methods |
args | any | A JSON object which will be mapped to url params |
body | any | An object with key value pairs to submit as post body |
options | any | AxiosRequestConfig | The optional request configuration for the passed Http client |
Checks if a transaction is a multi out transaction (with different amounts)
Returns:
true, if is a multi out transaction
Param | Description |
---|---|
transaction | Transaction to be checked |
string
Promise.<T>
Promise.<T>
Kind: inner class of core
string
Promise.<T>
Promise.<T>
Creates Service instance
Param | Description |
---|---|
settings | The settings for the service |
string
Mounts a BRS conform API (V1) endpoint of format <host>?requestType=getBlock&height=123
Kind: instance method of BurstService
Returns: string
-
The mounted url (without host)
Param | Type | Description |
---|---|---|
method | string | The method name for |
data | any | A JSON object which will be mapped to url params |
Promise.<T>
Requests a query to BRS
Kind: instance method of BurstService
Returns: Promise.<T>
-
The response data of success
HttpError in case of failure
Param | Type | Description |
---|---|---|
method | string | The BRS method according https://burstwiki.org/wiki/The_Burst_API |
args | any | A JSON object which will be mapped to url params |
options | any | AxiosRequestConfig | The optional request configuration for the passed Http client |
Promise.<T>
Send data to BRS
Kind: instance method of BurstService
Returns: Promise.<T>
-
The response data of success
HttpError in case of failure
Param | Type | Description |
---|---|---|
method | string | The BRS method according https://burstwiki.org/wiki/The_Burst_API. Note that there are only a few POST methods |
args | any | A JSON object which will be mapped to url params |
body | any | An object with key value pairs to submit as post body |
options | any | AxiosRequestConfig | The optional request configuration for the passed Http client |
Account class
The account class serves as a model for a Burstcoin account. It's meant to model the response from BRS API, except publicKey has been moved into the keys object.
string
Promise.<T>
Promise.<T>
Kind: inner class of core
string
Promise.<T>
Promise.<T>
Creates Service instance
Param | Description |
---|---|
settings | The settings for the service |
string
Mounts a BRS conform API (V1) endpoint of format <host>?requestType=getBlock&height=123
Kind: instance method of BurstService
Returns: string
-
The mounted url (without host)
Param | Type | Description |
---|---|---|
method | string | The method name for |
data | any | A JSON object which will be mapped to url params |
Promise.<T>
Requests a query to BRS
Kind: instance method of BurstService
Returns: Promise.<T>
-
The response data of success
HttpError in case of failure
Param | Type | Description |
---|---|---|
method | string | The BRS method according https://burstwiki.org/wiki/The_Burst_API |
args | any | A JSON object which will be mapped to url params |
options | any | AxiosRequestConfig | The optional request configuration for the passed Http client |
Promise.<T>
Send data to BRS
Kind: instance method of BurstService
Returns: Promise.<T>
-
The response data of success
HttpError in case of failure
Param | Type | Description |
---|---|---|
method | string | The BRS method according https://burstwiki.org/wiki/The_Burst_API. Note that there are only a few POST methods |
args | any | A JSON object which will be mapped to url params |
body | any | An object with key value pairs to submit as post body |
options | any | AxiosRequestConfig | The optional request configuration for the passed Http client |
Attachment class
The attachment class is used to appended to transaction where appropriate. It is a super class for Message and EncryptedMessage.
string
Promise.<T>
Promise.<T>
Kind: inner class of core
string
Promise.<T>
Promise.<T>
Creates Service instance
Param | Description |
---|---|
settings | The settings for the service |
string
Mounts a BRS conform API (V1) endpoint of format <host>?requestType=getBlock&height=123
Kind: instance method of BurstService
Returns: string
-
The mounted url (without host)
Param | Type | Description |
---|---|---|
method | string | The method name for |
data | any | A JSON object which will be mapped to url params |
Promise.<T>
Requests a query to BRS
Kind: instance method of BurstService
Returns: Promise.<T>
-
The response data of success
HttpError in case of failure
Param | Type | Description |
---|---|---|
method | string | The BRS method according https://burstwiki.org/wiki/The_Burst_API |
args | any | A JSON object which will be mapped to url params |
options | any | AxiosRequestConfig | The optional request configuration for the passed Http client |
Promise.<T>
Send data to BRS
Kind: instance method of BurstService
Returns: Promise.<T>
-
The response data of success
HttpError in case of failure
Param | Type | Description |
---|---|---|
method | string | The BRS method according https://burstwiki.org/wiki/The_Burst_API. Note that there are only a few POST methods |
args | any | A JSON object which will be mapped to url params |
body | any | An object with key value pairs to submit as post body |
options | any | AxiosRequestConfig | The optional request configuration for the passed Http client |
Message class
The Message class is used to model a plain message attached to a transaction.
string
Promise.<T>
Promise.<T>
Kind: inner class of core
string
Promise.<T>
Promise.<T>
Creates Service instance
Param | Description |
---|---|
settings | The settings for the service |
string
Mounts a BRS conform API (V1) endpoint of format <host>?requestType=getBlock&height=123
Kind: instance method of BurstService
Returns: string
-
The mounted url (without host)
Param | Type | Description |
---|---|---|
method | string | The method name for |
data | any | A JSON object which will be mapped to url params |
Promise.<T>
Requests a query to BRS
Kind: instance method of BurstService
Returns: Promise.<T>
-
The response data of success
HttpError in case of failure
Param | Type | Description |
---|---|---|
method | string | The BRS method according https://burstwiki.org/wiki/The_Burst_API |
args | any | A JSON object which will be mapped to url params |
options | any | AxiosRequestConfig | The optional request configuration for the passed Http client |
Promise.<T>
Send data to BRS
Kind: instance method of BurstService
Returns: Promise.<T>
-
The response data of success
HttpError in case of failure
Param | Type | Description |
---|---|---|
method | string | The BRS method according https://burstwiki.org/wiki/The_Burst_API. Note that there are only a few POST methods |
args | any | A JSON object which will be mapped to url params |
body | any | An object with key value pairs to submit as post body |
options | any | AxiosRequestConfig | The optional request configuration for the passed Http client |
EncryptedMessage class
The EncryptedMessage class is a model for a encrypted message attached to a transaction.
string
Promise.<T>
Promise.<T>
Kind: inner class of core
string
Promise.<T>
Promise.<T>
Creates Service instance
Param | Description |
---|---|
settings | The settings for the service |
string
Mounts a BRS conform API (V1) endpoint of format <host>?requestType=getBlock&height=123
Kind: instance method of BurstService
Returns: string
-
The mounted url (without host)
Param | Type | Description |
---|---|---|
method | string | The method name for |
data | any | A JSON object which will be mapped to url params |
Promise.<T>
Requests a query to BRS
Kind: instance method of BurstService
Returns: Promise.<T>
-
The response data of success
HttpError in case of failure
Param | Type | Description |
---|---|---|
method | string | The BRS method according https://burstwiki.org/wiki/The_Burst_API |
args | any | A JSON object which will be mapped to url params |
options | any | AxiosRequestConfig | The optional request configuration for the passed Http client |
Promise.<T>
Send data to BRS
Kind: instance method of BurstService
Returns: Promise.<T>
-
The response data of success
HttpError in case of failure
Param | Type | Description |
---|---|---|
method | string | The BRS method according https://burstwiki.org/wiki/The_Burst_API. Note that there are only a few POST methods |
args | any | A JSON object which will be mapped to url params |
body | any | An object with key value pairs to submit as post body |
options | any | AxiosRequestConfig | The optional request configuration for the passed Http client |
Copyright (c) 2019 Burst Apps Team
Kind: global class
FAQs
Burst-related functions and models for building Burstcoin applications.
The npm package @burstjs/core receives a total of 7 weekly downloads. As such, @burstjs/core popularity was classified as not popular.
We found that @burstjs/core demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.