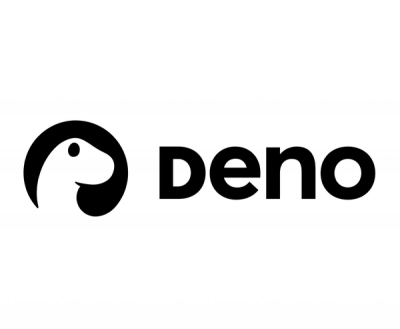
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
@caasi/hooks
Advanced tools
Some heretical React Hooks.
npm install @caasi/hooks
useConcat
Yields one value or another.
const x = useConcat(0, 1); // yields 0 and 1
useArray
Yields every values from a given array.
const xs = useMemo(() => [0, 1, 2], []);
const x = useArray(xs); // yields 0, 1 and 2
useGenerator
Treats a generator as a stream and collects values from it.
function* gen() {
yield 0;
return 1;
}
const iter = useMemo(() => gen(), []);
const [value, done] = useGenerator(iter);
useDefined
Filters out undefined values.
function* gen() {
yield 0;
yield undefined;
return 1;
}
const iter = useMemo(() => gen(), []);
const [value, done] = useGenerator(iter);
const x = useDefined(value); // yields 0 and 1
useFold
Folds over a hook value.
function* gen() {
yield 1;
yield 2;
return 3;
}
const iter = useMemo(() => gen(), []);
const [value, done] = useGenerator(iter);
const x = useFold(value, (a, b) => a + b, 0); // yields 0, 1, 3 and 6
useFilter
Filters out unwanted values.
const mod2 = useCallback(x => x % 2 === 0);
const as = useMemo(() => [0, 1, 2], []);
const x = useArray(as);
const y = useFilter(x, mod2); // yields 0 and 2
usePromise
Resolves a Promise
and returns its status.
const p = useMemo(() => api.get('https://example.com'), []);
const [value, error, isPending] = usePromise(p);
useMaybe
/useOptional
Chains optional values into another optional value.
const ab = useMaybe(
[a, b],
(a, b) => a * a + b * b,
);
useTime
Gives a DOMHighResTimeStamp
diff from the time it was called first time.
const t = useTime();
useRange
Gives a number between start
and end
. It's an application of useTime
and it's useful in simple animations.
const theta = useRange(0, 2 * Math.PI);
const x = Math.cos(theta);
const y = Math.sin(theta);
const pt = { x, y };
useImageData
Reads an image from a URL and gives you an ImageData
.
const imageData = useImageData('https://example.com/lena.png');
useBlob
/useFile
Reads a Blob
as an ArrayBuffer
or a string. Defaults to an ArrayBuffer
.
enum ResultType {
ARRAY_BUFFER = 'arraybuffer',
BINARY_STRING = 'binarystring',
DATA_URL = 'dataurl',
TEXT = 'text',
}
const dataurl = useBlob(file, useBlob.ResultType.DATA_URL);
useObjectURL
Creates an object URL from anything. It's useful with an image blob.
const imageData = useImageData(useObjectURL(file));
useImageFile
Gives a ImageData
from an image blob. It's an alias of file => useImageData(useObjectURL(file))
.
useWebSocket
Opens a web socket and streams messages.
const [socket, messages = []] = useWebSocket('wss://echo.websocket.org');
const msgs = messages.filter(x => x).reverse();
useProp
Binds a state and a state handler to a React element.
const [val, elem] = useProp(element, value, 'value', 'onChange', e => e.target.value);
useInput
A shortcut to bind a value to an input element.
const [r, rRange] = useInput(
<input type="range" min="0.0" max="1.0" step="0.01" />,
'1.0',
)
useSpace
Stores state histories.
It's named as useSpace
instead of useHistory
because it flattens a value in time to space(a list).
const [state, setState] = useState(0);
const history = useSpace(state);
useEffect(() => {
setState(s => s + 1);
console.log(history);
}, [history]);
useDefined
to keep the previous defined value.github
useFold
useFilter
undefined
as a reset signal in useSpace
useConcat
useImageData
useImageFile
useProp
useInput
useObjectURL
useTime
useRange
useWebSocket
FAQs
Some useful React Hooks
We found that @caasi/hooks demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.