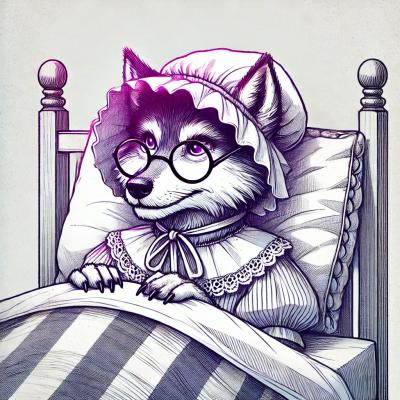
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
@capacitor/device
Advanced tools
The Device API exposes internal information about the device, such as the model and operating system version, along with user information such as unique ids.
@capacitor/device is a Capacitor plugin that provides access to device information such as the device model, operating system, and unique identifiers. It allows developers to retrieve various details about the device on which their app is running.
Get Device Info
This feature allows you to retrieve general information about the device, such as the model, operating system, and manufacturer.
const { Device } = require('@capacitor/device');
async function getDeviceInfo() {
const info = await Device.getInfo();
console.log(info);
}
getDeviceInfo();
Get Battery Info
This feature allows you to get information about the device's battery status, including the battery level and whether the device is charging.
const { Device } = require('@capacitor/device');
async function getBatteryInfo() {
const info = await Device.getBatteryInfo();
console.log(info);
}
getBatteryInfo();
Get Language Code
This feature allows you to retrieve the device's current language code, which can be useful for localization purposes.
const { Device } = require('@capacitor/device');
async function getLanguageCode() {
const info = await Device.getLanguageCode();
console.log(info);
}
getLanguageCode();
Get Unique ID
This feature allows you to get a unique identifier for the device, which can be used for tracking or analytics purposes.
const { Device } = require('@capacitor/device');
async function getUniqueId() {
const info = await Device.getId();
console.log(info);
}
getUniqueId();
react-native-device-info is a React Native library that provides device information such as device model, system version, and unique identifiers. It offers similar functionalities to @capacitor/device but is specifically designed for React Native applications.
cordova-plugin-device is a Cordova plugin that provides information about the device's hardware and software. It offers functionalities similar to @capacitor/device but is intended for use with Cordova-based projects.
expo-device is an Expo module that provides information about the device, such as the device model, operating system, and unique identifiers. It is similar to @capacitor/device but is designed for use with Expo and React Native projects.
The Device API exposes internal information about the device, such as the model and operating system version, along with user information such as unique ids.
npm install @capacitor/device
npx cap sync
Apple mandates that app developers now specify approved reasons for API usage to enhance user privacy. By May 1st, 2024, it's required to include these reasons when submitting apps to the App Store Connect.
When using this specific plugin in your app, you must create a PrivacyInfo.xcprivacy
file in /ios/App
or use the VS Code Extension to generate it, specifying the usage reasons.
For detailed steps on how to do this, please see the Capacitor Docs.
For this plugin, the required dictionary key is NSPrivacyAccessedAPICategoryDiskSpace and the recommended reason is 85F4.1.
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE plist PUBLIC "-//Apple//DTD PLIST 1.0//EN" "http://www.apple.com/DTDs/PropertyList-1.0.dtd">
<plist version="1.0">
<dict>
<key>NSPrivacyAccessedAPITypes</key>
<array>
<!-- Add this dict entry to the array if the PrivacyInfo file already exists -->
<dict>
<key>NSPrivacyAccessedAPIType</key>
<string>NSPrivacyAccessedAPICategoryDiskSpace</string>
<key>NSPrivacyAccessedAPITypeReasons</key>
<array>
<string>85F4.1</string>
</array>
</dict>
</array>
</dict>
</plist>
import { Device } from '@capacitor/device';
const logDeviceInfo = async () => {
const info = await Device.getInfo();
console.log(info);
};
const logBatteryInfo = async () => {
const info = await Device.getBatteryInfo();
console.log(info);
};
getId() => Promise<DeviceId>
Return an unique identifier for the device.
Returns: Promise<DeviceId>
Since: 1.0.0
getInfo() => Promise<DeviceInfo>
Return information about the underlying device/os/platform.
Returns: Promise<DeviceInfo>
Since: 1.0.0
getBatteryInfo() => Promise<BatteryInfo>
Return information about the battery.
Returns: Promise<BatteryInfo>
Since: 1.0.0
getLanguageCode() => Promise<GetLanguageCodeResult>
Get the device's current language locale code.
Returns: Promise<GetLanguageCodeResult>
Since: 1.0.0
getLanguageTag() => Promise<LanguageTag>
Get the device's current language locale tag.
Returns: Promise<LanguageTag>
Since: 4.0.0
Prop | Type | Description | Since |
---|---|---|---|
identifier | string | The identifier of the device as available to the app. This identifier may change on modern mobile platforms that only allow per-app install ids. On iOS, the identifier is a UUID that uniquely identifies a device to the app’s vendor (read more). on Android 8+, the identifier is a 64-bit number (expressed as a hexadecimal string), unique to each combination of app-signing key, user, and device (read more). On web, a random identifier is generated and stored on localStorage for subsequent calls. If localStorage is not available a new random identifier will be generated on every call. | 1.0.0 |
Prop | Type | Description | Since |
---|---|---|---|
name | string | The name of the device. For example, "John's iPhone". This is only supported on iOS and Android 7.1 or above. On iOS 16+ this will return a generic device name without the appropriate entitlements. | 1.0.0 |
model | string | The device model. For example, "iPhone13,4". | 1.0.0 |
platform | 'ios' | 'android' | 'web' | The device platform (lowercase). | 1.0.0 |
operatingSystem | OperatingSystem | The operating system of the device. | 1.0.0 |
osVersion | string | The version of the device OS. | 1.0.0 |
iOSVersion | number | The iOS version number. Only available on iOS. Multi-part version numbers are crushed down into an integer padded to two-digits, ex: "16.3.1" -> 160301 | 5.0.0 |
androidSDKVersion | number | The Android SDK version number. Only available on Android. | 5.0.0 |
manufacturer | string | The manufacturer of the device. | 1.0.0 |
isVirtual | boolean | Whether the app is running in a simulator/emulator. | 1.0.0 |
memUsed | number | Approximate memory used by the current app, in bytes. Divide by 1048576 to get the number of MBs used. | 1.0.0 |
diskFree | number | How much free disk space is available on the normal data storage path for the os, in bytes. On Android it returns the free disk space on the "system" partition holding the core Android OS. On iOS this value is not accurate. | 1.0.0 |
diskTotal | number | The total size of the normal data storage path for the OS, in bytes. On Android it returns the disk space on the "system" partition holding the core Android OS. | 1.0.0 |
realDiskFree | number | How much free disk space is available on the normal data storage, in bytes. | 1.1.0 |
realDiskTotal | number | The total size of the normal data storage path, in bytes. | 1.1.0 |
webViewVersion | string | The web view browser version | 1.0.0 |
Prop | Type | Description | Since |
---|---|---|---|
batteryLevel | number | A percentage (0 to 1) indicating how much the battery is charged. | 1.0.0 |
isCharging | boolean | Whether the device is charging. | 1.0.0 |
Prop | Type | Description | Since |
---|---|---|---|
value | string | Two character language code. | 1.0.0 |
Prop | Type | Description | Since |
---|---|---|---|
value | string | Returns a well-formed IETF BCP 47 language tag. | 4.0.0 |
'ios' | 'android' | 'windows' | 'mac' | 'unknown'
FAQs
The Device API exposes internal information about the device, such as the model and operating system version, along with user information such as unique ids.
The npm package @capacitor/device receives a total of 78,072 weekly downloads. As such, @capacitor/device popularity was classified as popular.
We found that @capacitor/device demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.