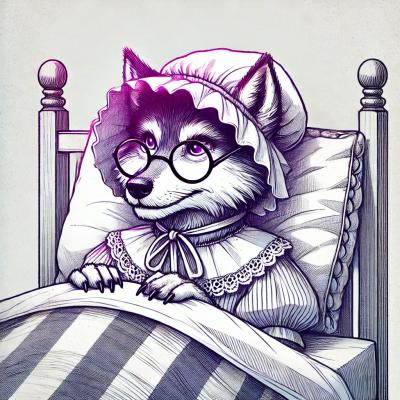
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
@capacitor/haptics
Advanced tools
The Haptics API provides physical feedback to the user through touch or vibration.
@capacitor/haptics is a Capacitor plugin that provides haptic feedback functionality for mobile applications. It allows developers to trigger various types of haptic feedback, such as vibrations and impact feedback, to enhance the user experience.
Impact Feedback
This feature allows you to trigger an impact feedback with different styles such as light, medium, and heavy. The code sample demonstrates how to trigger a heavy impact feedback.
import { Haptics, ImpactStyle } from '@capacitor/haptics';
async function triggerImpact() {
await Haptics.impact({ style: ImpactStyle.Heavy });
}
triggerImpact();
Notification Feedback
This feature allows you to trigger notification feedback with different types such as success, warning, and error. The code sample demonstrates how to trigger a success notification feedback.
import { Haptics, NotificationType } from '@capacitor/haptics';
async function triggerNotification() {
await Haptics.notification({ type: NotificationType.SUCCESS });
}
triggerNotification();
Vibration
This feature allows you to trigger a simple vibration. The code sample demonstrates how to trigger a basic vibration feedback.
import { Haptics } from '@capacitor/haptics';
async function triggerVibration() {
await Haptics.vibrate();
}
triggerVibration();
Selection Feedback
This feature allows you to provide feedback for selection changes. The code sample demonstrates how to trigger selection start, change, and end feedback.
import { Haptics } from '@capacitor/haptics';
async function triggerSelection() {
await Haptics.selectionStart();
// Perform some selection action
await Haptics.selectionChanged();
// Perform some selection action
await Haptics.selectionEnd();
}
triggerSelection();
react-native-haptic-feedback is a React Native library that provides haptic feedback functionality similar to @capacitor/haptics. It allows developers to trigger different types of haptic feedback on iOS and Android devices. Compared to @capacitor/haptics, it is specifically designed for React Native applications.
cordova-plugin-haptic is a Cordova plugin that provides haptic feedback functionality. It allows developers to trigger various types of haptic feedback on mobile devices. Compared to @capacitor/haptics, it is designed for Cordova-based applications.
expo-haptics is an Expo module that provides haptic feedback functionality for React Native applications. It allows developers to trigger different types of haptic feedback on iOS and Android devices. Compared to @capacitor/haptics, it is part of the Expo ecosystem and is designed for use with Expo-managed workflows.
The Haptics API provides physical feedback to the user through touch or vibration.
On devices that don't have Taptic Engine or Vibrator, the API calls will resolve without performing any action.
npm install @capacitor/haptics
npx cap sync
import { Haptics, ImpactStyle } from '@capacitor/haptics';
const hapticsImpactMedium = async () => {
await Haptics.impact({ style: ImpactStyle.Medium });
};
const hapticsImpactLight = async () => {
await Haptics.impact({ style: ImpactStyle.Light });
};
const hapticsVibrate = async () => {
await Haptics.vibrate();
};
const hapticsSelectionStart = async () => {
await Haptics.selectionStart();
};
const hapticsSelectionChanged = async () => {
await Haptics.selectionChanged();
};
const hapticsSelectionEnd = async () => {
await Haptics.selectionEnd();
};
impact(...)
notification(...)
vibrate(...)
selectionStart()
selectionChanged()
selectionEnd()
impact(options?: ImpactOptions | undefined) => Promise<void>
Trigger a haptics "impact" feedback
Param | Type |
---|---|
options | ImpactOptions |
Since: 1.0.0
notification(options?: NotificationOptions | undefined) => Promise<void>
Trigger a haptics "notification" feedback
Param | Type |
---|---|
options | NotificationOptions |
Since: 1.0.0
vibrate(options?: VibrateOptions | undefined) => Promise<void>
Vibrate the device
Param | Type |
---|---|
options | VibrateOptions |
Since: 1.0.0
selectionStart() => Promise<void>
Trigger a selection started haptic hint
Since: 1.0.0
selectionChanged() => Promise<void>
Trigger a selection changed haptic hint. If a selection was started already, this will cause the device to provide haptic feedback
Since: 1.0.0
selectionEnd() => Promise<void>
If selectionStart() was called, selectionEnd() ends the selection. For example, call this when a user has lifted their finger from a control
Since: 1.0.0
Prop | Type | Description | Default | Since |
---|---|---|---|---|
style | ImpactStyle | Impact Feedback Style The mass of the objects in the collision simulated by a UIImpactFeedbackGenerator object. | ImpactStyle.Heavy | 1.0.0 |
Prop | Type | Description | Default | Since |
---|---|---|---|---|
type | NotificationType | Notification Feedback Type The type of notification feedback generated by a UINotificationFeedbackGenerator object. | NotificationType.SUCCESS | 1.0.0 |
Prop | Type | Description | Default | Since |
---|---|---|---|---|
duration | number | Duration of the vibration in milliseconds. | 300 | 1.0.0 |
Members | Value | Description | Since |
---|---|---|---|
Heavy | 'HEAVY' | A collision between large, heavy user interface elements | 1.0.0 |
Medium | 'MEDIUM' | A collision between moderately sized user interface elements | 1.0.0 |
Light | 'LIGHT' | A collision between small, light user interface elements | 1.0.0 |
Members | Value | Description | Since |
---|---|---|---|
Success | 'SUCCESS' | A notification feedback type indicating that a task has completed successfully | 1.0.0 |
Warning | 'WARNING' | A notification feedback type indicating that a task has produced a warning | 1.0.0 |
Error | 'ERROR' | A notification feedback type indicating that a task has failed | 1.0.0 |
FAQs
The Haptics API provides physical feedback to the user through touch or vibration.
The npm package @capacitor/haptics receives a total of 51,546 weekly downloads. As such, @capacitor/haptics popularity was classified as popular.
We found that @capacitor/haptics demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 7 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.