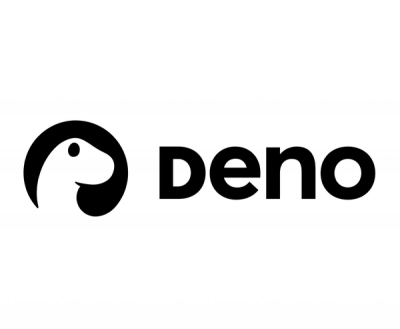
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
@casbin/hapi-authz
Advanced tools
This is a authorization middleware for Hapi js, and it is based on Node-Casbin.
npm i casbin @casbin/hapi-authz --save
const { newEnforcer } = require('casbin');
const hapiauthz = require('@casbin/hapi-authz');
...
const init = async () => {
...
const enforcer = await newEnforcer('model.conf', 'policy.csv') // replace with your model and policy file location
await server.register({
plugin: hapiauthz.Hapiauthz,
options: {
newEnforcer: enforcer
}
...
})
}
This package provides BasicAuthorizer
, which checks the Authorization header for the username.
If you want to use another authentication method like OAuth, you needs to extends BasicAuthorizer
as below:
class MyAuthorizer extends hapiauthz.BasicAuthorizer {
constructor(request, enforcer) {
super(request, enforcer);
}
getUserName () {
const { username } = this.request.credentials.username
return username
}
}
const init = async () => {
...
const enforcer = await newEnforcer('model.conf', 'policy.csv') // replace with your model and policy file location
await server.register({
plugin: hapiauthz.Hapiauthz,
options: {
newEnforcer: enforcer,
authorizer: (request, option) => new MyAuthorizer(request, option)
}
...
})
}
The authorization determines a request based on {subject, object, action}
, which means what subject
can perform what action
on what object
. In this plugin, the meanings are:
subject
: the logged-on user nameobject
: the URL path for the web resource like "dataset1/item1"action
: HTTP method like GET, POST, PUT, DELETE, or the high-level actions you defined like "read-file", "write-blog"For how to write authorization policy and other details, please refer to the Casbin's documentation.
FAQs
Basic authorization middleware using casbin
The npm package @casbin/hapi-authz receives a total of 4 weekly downloads. As such, @casbin/hapi-authz popularity was classified as not popular.
We found that @casbin/hapi-authz demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.