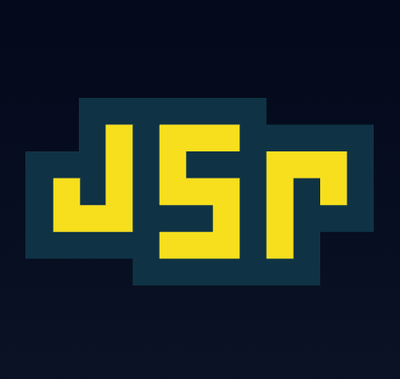
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
@chakra-ui/accordion
Advanced tools
@chakra-ui/accordion is a component library for creating accessible and customizable accordion components in React applications. It is part of the Chakra UI library, which provides a set of accessible, reusable, and composable React components.
Basic Accordion
This code demonstrates a basic accordion with two sections. Each section can be expanded or collapsed by clicking on the header.
import { Accordion, AccordionItem, AccordionButton, AccordionPanel, AccordionIcon } from '@chakra-ui/accordion';
function BasicAccordion() {
return (
<Accordion>
<AccordionItem>
<h2>
<AccordionButton>
<Box flex='1' textAlign='left'>
Section 1
</Box>
<AccordionIcon />
</AccordionButton>
</h2>
<AccordionPanel pb={4}>
Content for section 1
</AccordionPanel>
</AccordionItem>
<AccordionItem>
<h2>
<AccordionButton>
<Box flex='1' textAlign='left'>
Section 2
</Box>
<AccordionIcon />
</AccordionButton>
</h2>
<AccordionPanel pb={4}>
Content for section 2
</AccordionPanel>
</AccordionItem>
</Accordion>
);
}
Accordion with Multiple Expandable Sections
This code demonstrates an accordion where multiple sections can be expanded at the same time. The `allowMultiple` prop enables this functionality.
import { Accordion, AccordionItem, AccordionButton, AccordionPanel, AccordionIcon } from '@chakra-ui/accordion';
function MultipleExpandableAccordion() {
return (
<Accordion allowMultiple>
<AccordionItem>
<h2>
<AccordionButton>
<Box flex='1' textAlign='left'>
Section 1
</Box>
<AccordionIcon />
</AccordionButton>
</h2>
<AccordionPanel pb={4}>
Content for section 1
</AccordionPanel>
</AccordionItem>
<AccordionItem>
<h2>
<AccordionButton>
<Box flex='1' textAlign='left'>
Section 2
</Box>
<AccordionIcon />
</AccordionButton>
</h2>
<AccordionPanel pb={4}>
Content for section 2
</AccordionPanel>
</AccordionItem>
</Accordion>
);
}
Accordion with Controlled State
This code demonstrates an accordion with controlled state. The `index` state variable controls which sections are expanded, and the `onChange` handler updates this state.
import { useState } from 'react';
import { Accordion, AccordionItem, AccordionButton, AccordionPanel, AccordionIcon } from '@chakra-ui/accordion';
function ControlledAccordion() {
const [index, setIndex] = useState([0]);
return (
<Accordion index={index} onChange={setIndex} allowMultiple>
<AccordionItem>
<h2>
<AccordionButton>
<Box flex='1' textAlign='left'>
Section 1
</Box>
<AccordionIcon />
</AccordionButton>
</h2>
<AccordionPanel pb={4}>
Content for section 1
</AccordionPanel>
</AccordionItem>
<AccordionItem>
<h2>
<AccordionButton>
<Box flex='1' textAlign='left'>
Section 2
</Box>
<AccordionIcon />
</AccordionButton>
</h2>
<AccordionPanel pb={4}>
Content for section 2
</AccordionPanel>
</AccordionItem>
</Accordion>
);
}
react-accessible-accordion is a lightweight and accessible accordion component for React. It focuses on providing a simple API and ensuring accessibility. Compared to @chakra-ui/accordion, it is more lightweight but may lack some of the advanced customization options provided by Chakra UI.
react-bootstrap is a popular library that provides Bootstrap components for React, including an Accordion component. It offers a wide range of pre-styled components and is a good choice if you are already using Bootstrap in your project. However, it may not be as flexible or customizable as @chakra-ui/accordion.
material-ui is a comprehensive library of React components that implement Google's Material Design. It includes an Accordion component with extensive customization options. While it offers a rich set of features and design consistency, it may be more complex to use compared to @chakra-ui/accordion.
An accordion is a vertically stacked set of interactive headings that each contain a title or content snippet representing a section of content.
The headings function as controls that enable users to reveal or hide their associated sections of content.
yarn add @chakra-ui/accordion
# or
npm i @chakra-ui/accordion
import {
Accordion,
AccordionItem,
AccordionButton,
AccordionPanel,
} from "@chakra-ui/accordion"
Accordion
: manages the global state of all opened accordionitems via
context.AccordionItem
: manages the state for a single accordion item.AccordionButton
: the trigger to open/close an accordion item.AccordionPanel
: the main content area for the accordion item.By default, only one accordion can be visible at a time, and it can't be toggled.
Note 🚨: Each accordion button must be wrapped in an heading tag, that is appropriate for the information architecture of the page.
<Accordion>
<AccordionItem>
<h2>
<AccordionButton>Section 1 title</AccordionButton>
</h2>
<AccordionPanel>Panel 1</AccordionPanel>
</AccordionItem>
<AccordionItem>
<h2>
<AccordionButton>Section 2 title</AccordionButton>
</h2>
<AccordionPanel>Panel 2</AccordionPanel>
</AccordionItem>
</Accordion>
To make each accordion toggle (expand/collapse) on click, pass the allowToggle
prop.
<Accordion allowToggle>
<AccordionItem>
<AccordionButton>
<chakra.div flex="1" textAlign="left">
Section 1 title
</chakra.div>
<AccordionIcon />
</AccordionButton>
<AccordionPanel pb={4}>Panel 1</AccordionPanel>
</AccordionItem>
<AccordionItem>
<AccordionButton>
<chakra.div flex="1" textAlign="left">
Section 2 title
</chakra.div>
<AccordionIcon />
</AccordionButton>
<AccordionPanel pb={4}>Panel 2</AccordionPanel>
</AccordionItem>
</Accordion>
To allow multiple accordions to be visible at a time, pass the allowMultiple
prop.
<Accordion allowMultiple>
<AccordionItem>
<AccordionButton>
<chakra.div flex="1" textAlign="left">
Section 1 title
</chakra.div>
<AccordionIcon />
</AccordionButton>
<AccordionPanel pb={4}>Panel 1</AccordionPanel>
</AccordionItem>
<AccordionItem>
<AccordionButton>
<chakra.div flex="1" textAlign="left">
Section 2 title
</chakra.div>
<AccordionIcon />
</AccordionButton>
<AccordionPanel pb={4}>Panel 2</AccordionPanel>
</AccordionItem>
</Accordion>
https://www.w3.org/TR/wai-aria-practices/examples/accordion/accordion.html https://inclusive-components.design/collapsible-sections/ https://github.com/stereobooster/react-accessible-accordion https://jqueryui.com/accordion/
FAQs
A simple and accessible accordion component for React & Chakra UI
The npm package @chakra-ui/accordion receives a total of 460,273 weekly downloads. As such, @chakra-ui/accordion popularity was classified as popular.
We found that @chakra-ui/accordion demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.