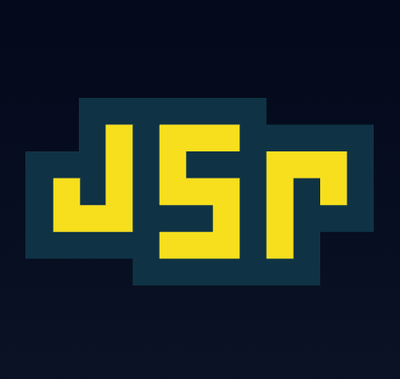
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
@chakra-ui/modal
Advanced tools
An accessible dialog (modal) component for React & Chakra UI
@chakra-ui/modal is a component library for creating accessible and customizable modal dialogs in React applications. It provides a set of components and hooks to easily manage modal states and styles.
Basic Modal
This code demonstrates how to create a basic modal using @chakra-ui/modal. It includes a button to open the modal, and the modal itself contains a header, body, footer, and a close button.
```jsx
import { Modal, ModalOverlay, ModalContent, ModalHeader, ModalFooter, ModalBody, ModalCloseButton, Button, useDisclosure } from '@chakra-ui/react';
function BasicModal() {
const { isOpen, onOpen, onClose } = useDisclosure();
return (
<>
<Button onClick={onOpen}>Open Modal</Button>
<Modal isOpen={isOpen} onClose={onClose}>
<ModalOverlay />
<ModalContent>
<ModalHeader>Modal Title</ModalHeader>
<ModalCloseButton />
<ModalBody>
This is the modal body.
</ModalBody>
<ModalFooter>
<Button colorScheme="blue" mr={3} onClick={onClose}>
Close
</Button>
</ModalFooter>
</ModalContent>
</Modal>
</>
);
}
```
Centered Modal
This code demonstrates how to create a centered modal using @chakra-ui/modal. The `isCentered` prop ensures that the modal is vertically and horizontally centered on the screen.
```jsx
import { Modal, ModalOverlay, ModalContent, ModalHeader, ModalFooter, ModalBody, ModalCloseButton, Button, useDisclosure } from '@chakra-ui/react';
function CenteredModal() {
const { isOpen, onOpen, onClose } = useDisclosure();
return (
<>
<Button onClick={onOpen}>Open Centered Modal</Button>
<Modal isOpen={isOpen} onClose={onClose} isCentered>
<ModalOverlay />
<ModalContent>
<ModalHeader>Centered Modal</ModalHeader>
<ModalCloseButton />
<ModalBody>
This modal is centered.
</ModalBody>
<ModalFooter>
<Button colorScheme="blue" mr={3} onClick={onClose}>
Close
</Button>
</ModalFooter>
</ModalContent>
</Modal>
</>
);
}
```
Scrollable Modal
This code demonstrates how to create a scrollable modal using @chakra-ui/modal. The `scrollBehavior` prop set to `inside` ensures that the modal content is scrollable if it exceeds the viewport height.
```jsx
import { Modal, ModalOverlay, ModalContent, ModalHeader, ModalFooter, ModalBody, ModalCloseButton, Button, useDisclosure } from '@chakra-ui/react';
function ScrollableModal() {
const { isOpen, onOpen, onClose } = useDisclosure();
return (
<>
<Button onClick={onOpen}>Open Scrollable Modal</Button>
<Modal isOpen={isOpen} onClose={onClose} scrollBehavior="inside">
<ModalOverlay />
<ModalContent>
<ModalHeader>Scrollable Modal</ModalHeader>
<ModalCloseButton />
<ModalBody>
<p>Content that is long enough to require scrolling...</p>
<p>More content...</p>
<p>Even more content...</p>
</ModalBody>
<ModalFooter>
<Button colorScheme="blue" mr={3} onClick={onClose}>
Close
</Button>
</ModalFooter>
</ModalContent>
</Modal>
</>
);
}
```
react-modal is a widely-used library for creating accessible modal dialogs in React. It provides a simple API and is highly customizable. Compared to @chakra-ui/modal, react-modal is more lightweight but may require more manual styling and state management.
react-bootstrap is a popular library that provides Bootstrap components for React, including modals. It offers a comprehensive set of pre-styled components and is easy to integrate with Bootstrap themes. Compared to @chakra-ui/modal, react-bootstrap is more opinionated in terms of styling and may be heavier due to its reliance on Bootstrap.
material-ui is a comprehensive library of React components that implement Google's Material Design. It includes a Modal component with extensive customization options. Compared to @chakra-ui/modal, material-ui offers a broader range of components and design consistency but can be more complex to configure.
A modal is a window overlaid on either the primary window or another dialog window. Contents behind a modal dialog are inert meaning that users cannot interact with content behind the dialog.
yarn add @chakra-ui/modal
# or
npm i @chakra-ui/modal
import {
Modal,
ModalOverlay,
ModalContent,
ModalHeader,
ModalFooter,
ModalBody,
ModalCloseButton,
} from "@chakra-ui/react"
When the modal opens, focus is sent into the modal and set to the first tabbable
element. If there are no tabbed element, focus is set on the ModalContent
.
function BasicUsage() {
const { isOpen, onOpen, onClose } = useDisclosure()
return (
<>
<Button onClick={onOpen}>Open Modal</Button>
<Modal isOpen={isOpen} onClose={onClose}>
<ModalOverlay>
<ModalContent>
<ModalHeader>Modal Title</ModalHeader>
<ModalCloseButton />
<ModalBody>
<Lorem count={2} />
</ModalBody>
<ModalFooter>
<Button colorScheme="blue" mr={3} onClick={onClose}>
Close
</Button>
<Button variant="ghost">Secondary Action</Button>
</ModalFooter>
</ModalContent>
</ModalOverlay>
</Modal>
</>
)
}
When the dialog closes, it returns focus to the element that triggered. Set
finalFocusRef
to element that should receive focus when the modal opens.
function ReturnFocus() {
const { isOpen, onOpen, onClose } = useDisclosure()
const finalRef = React.useRef()
return (
<>
<Box ref={finalRef} tabIndex={-1} aria-label="Focus moved to this box">
Some other content that'll receive focus on close.
</Box>
<Button mt={4} onClick={onOpen}>
Open Modal
</Button>
<Modal finalFocusRef={finalRef} isOpen={isOpen} onClose={onClose}>
<ModalOverlay>
<ModalContent>
<ModalHeader>Modal Title</ModalHeader>
<ModalCloseButton />
<ModalBody>
<Lorem count={2} />
</ModalBody>
<ModalFooter>
<Button colorScheme="blue" mr={3} onClick={onClose}>
Close
</Button>
<Button variant="ghost">Secondary Action</Button>
</ModalFooter>
</ModalContent>
</ModalOverlay>
</Modal>
</>
)
}
AlertDialog component is used interrupt the user with a mandatory confirmation or action.
yarn add @chakra-ui/alert-dialog
# or
npm i @chakra-ui/alert-dialog
import {
AlertDialog,
AlertDialogBody,
AlertDialogFooter,
AlertDialogHeader,
AlertDialogContent,
AlertDialogOverlay,
} from "@chakra-ui/react"
AlertDialog requires that you provide the leastDestructiveRef
prop.
Based on WAI-ARIA specifications, focus should be placed on the least destructive element when the dialog opens, to prevent users from accidentally confirming the destructive action.
function AlertDialogExample() {
const [isOpen, setIsOpen] = React.useState()
const onClose = () => setIsOpen(false)
const cancelRef = React.useRef()
return (
<>
<Button colorScheme="red" onClick={() => setIsOpen(true)}>
Delete Customer
</Button>
<AlertDialog
isOpen={isOpen}
leastDestructiveRef={cancelRef}
onClose={onClose}
>
<AlertDialogOverlay>
<AlertDialogContent>
<AlertDialogHeader fontSize="lg" fontWeight="bold">
Delete Customer
</AlertDialogHeader>
<AlertDialogBody>
Are you sure? You can't undo this action afterwards.
</AlertDialogBody>
<AlertDialogFooter>
<Button ref={cancelRef} onClick={onClose}>
Cancel
</Button>
<Button colorScheme="red" onClick={onClose} ml={3}>
Delete
</Button>
</AlertDialogFooter>
</AlertDialogContent>
</AlertDialogOverlay>
</AlertDialog>
</>
)
}
Using the above example will throw a typescript error. You'll want to modify the
type of the useRef
hook to the HTML element you're placing the focus on and
default the value to null.
const cancelRef = React.useRef<HTMLButtonElement>(null)
The Drawer component is a panel that slides out from the edge of the screen. It can be useful when you need users to complete a task or view some details without leaving the current page.
yarn add @chakra-ui/modal
# or
npm i @chakra-ui/modal
import {
Drawer,
DrawerOverlay,
DrawerContent,
DrawerHeader,
DrawerFooter,
DrawerBody,
DrawerCloseButton,
} from "@chakra-ui/react"
By default, focus is placed on DrawerCloseButton
when the drawer opens.
function DrawerExample() {
const { isOpen, onOpen, onClose } = useDisclosure()
const btnRef = React.useRef()
return (
<>
<Button ref={btnRef} colorScheme="teal" onClick={onOpen}>
Open
</Button>
<Drawer
isOpen={isOpen}
placement="right"
onClose={onClose}
finalFocusRef={btnRef}
>
<DrawerOverlay />
<DrawerContent>
<DrawerCloseButton />
<DrawerHeader>Create your account</DrawerHeader>
<DrawerBody>
<Input placeholder="Type here..." />
</DrawerBody>
<DrawerFooter>
<Button variant="outline" mr={3} onClick={onClose}>
Cancel
</Button>
<Button color="blue">Save</Button>
</DrawerFooter>
</DrawerContent>
</Drawer>
</>
)
}
FAQs
An accessible dialog (modal) component for React & Chakra UI
The npm package @chakra-ui/modal receives a total of 460,730 weekly downloads. As such, @chakra-ui/modal popularity was classified as popular.
We found that @chakra-ui/modal demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.