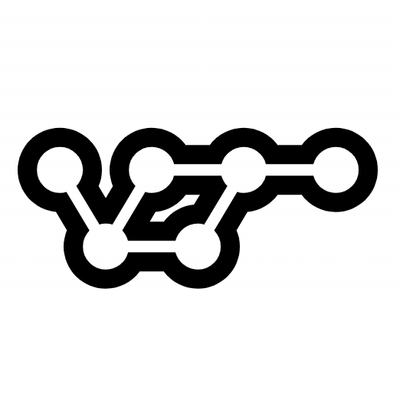
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
@chelmsfordbeer/pdf-creator-node
Advanced tools
Step 1 - install the pdf creator package using the following command
$ npm i @chelmsfordbeer/pdf-creator-node --save
--save flag adds package name to package.json file.
Step 2 - Add required packages and read HTML template
//Required package
const pdf = require("@chelmsfordbeer/pdf-creator-node");
const fs = require("fs");
// Read HTML Template
const html = fs.readFileSync("template.html", "utf8");
Step 3 - Create your HTML Template
<!DOCTYPE html>
<html>
<head>
<mate charest="utf-8" />
<title>Hello world!</title>
</head>
<body>
<h1>User List</h1>
<ul>
{{#each users}}
<li>Name: {{this.name}}</li>
<li>Age: {{this.age}}</li>
<br />
{{/each}}
</ul>
</body>
</html>
Step 4 - Provide format and orientation as per your need
"height": "10.5in", // allowed units: mm, cm, in, px
"width": "8in", // allowed units: mm, cm, in, px
or -
"format": "Letter", // allowed units: A3, A4, A5, Legal, Letter, Tabloid
"orientation": "portrait", // portrait or landscape
const options = {
format: "A3",
orientation: "portrait",
border: "10mm",
header: {
height: "45mm",
contents: '<div style="text-align: center;">Author: Shyam Hajare</div>'
},
footer: {
height: "28mm",
contents: {
first: 'Cover page',
2: 'Second page', // Any page number is working. 1-based index
default: '<span style="color: #444;">{{page}}</span>/<span>{{pages}}</span>', // fallback value
last: 'Last Page'
}
}
};
Step 5 - Provide HTML, user data and PDF path for output
const users = [
{
name: "Shyam",
age: "26",
},
{
name: "Navjot",
age: "26",
},
{
name: "Vitthal",
age: "26",
},
];
const document = {
html: html,
data: {
users: users,
},
path: "./output.pdf", // Output a file in the current directory
type: "" // Remove path and set type to "buffer" or "stream" if needed.
};
Step 6 - After setting all parameters, just pass document and options to pdf.create
method.
pdf.create(document, options)
.then((res) => {
console.log(res);
})
.catch((error) => {
console.error(error);
});
Please refer to the following if you want to use conditions in your HTML template:
pdf-creator-node is MIT licensed.
FAQs
node pdf creator
We found that @chelmsfordbeer/pdf-creator-node demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.