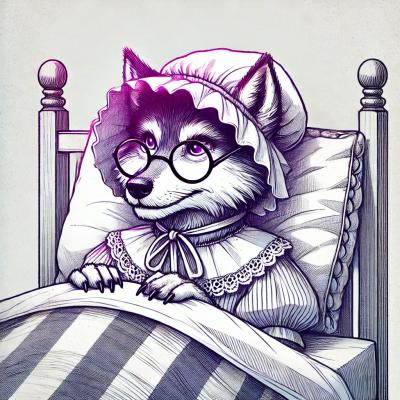
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
@chialab/dna
Advanced tools
DNA • Progressive Web Components
DNA is a view library with first class support for reactive and functional Web Components. No polyfills are required: DNA uses its template engine to handle Custom Elements life cycle, resulting more efficient, reliable and light.
DNA does not introduce any custom pattern for Component definitions, since it is based on the standard Custom Elements specifications, so the life cycle is almost the same, with some helper methods.
In order to be fast, predictive and easier to install, DNA uses a custom template engine. Components automatically re-render when the state change and only the necessary patches are applied to the DOM tree thanks to an in-place diffing algorithm.
If you are familiar with JSX, you can write your templates using the React syntax, but if you prefer to use standard JavaScript you can also use template strings to avoid the build step in your workflow.
DNA comes with a lot of features in a very small package. You can use <slot>
elements like in Shadow DOM contexts, observe properties changes and delegate events. It can also resolve Promise
s and pipe Observable
s directly in the template.
Usage via unpkg.com as ES6 module:
import { Component, customElements, html, ... } from 'https://unpkg.com/@chialab/dna?module';
Install via NPM:
$ npm i @chialab/dna
$ yarn add @chialab/dna
import { Component, customElements, html, ... } from '@chialab/dna';
This is an example of a Component defined via DNA. Please refer to the documentation for more examples and cases of use.
Define the component (TypeScript)
import { Component, customElement, property, listen } from '@chialab/dna';
@customElement('hello-world')
class HelloWorld extends Component {
// define an observed property
@property() name: string = '';
render() {
return <>
<input name="firstName" value={this.name} />
<h1>Hello {this.name || 'World'}!</h1>
</>;
}
// delegate an event
@listen('change', 'input[name="firstName"]')
private onChange(event: Event, target: HTMLInputElement) {
this.name = target.value;
}
}
Define the component (JavaScript)
import { Component, customElements, html, property, listen } from '@chialab/dna';
class HelloWorld extends Component {
static get properties() {
return {
// define an observed property
name: {
type: String,
defaultValue: '',
},
};
}
static get listeners() {
return {
// delegate an event
'change input[name="firstName"]': function(event, target) {
this.name = target.value;
}
};
}
render() {
return html`
<input name="firstName" value="${this.name}" />
<h1>Hello ${this.name || 'World'}!</h1>
`;
}
}
customElements.define('hello-world', HelloWorld);
Then use the element in your HTML:
<hello-world></hello-world>
Tests are run against all ever green browsers, Internet Explorer and old Safari versions. DNA itself does not require any polyfill and it is distribute as ES6 module (with untranspiled classes and async
/await
statements), but some Babel helpers if you want to use decorators need support for Symbol
, Object.assign
and Array.prototype.find
. Also, a polyfill for Promise
is required in IE11 if you are using async methods or the registry's whenDefined
method.
Install the dependencies and run the build
script:
$ yarn install
$ yarn build
This will generate the the ESM bundles in the dist
folder, as well as the declaration files.
Run the test
script:
$ yarn test
DNA is released under the MIT license.
FAQs
Progressive Web Components
The npm package @chialab/dna receives a total of 147 weekly downloads. As such, @chialab/dna popularity was classified as not popular.
We found that @chialab/dna demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.