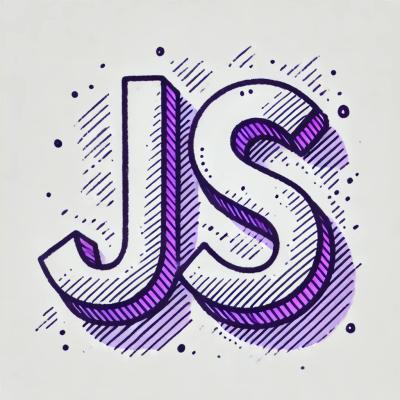
Security News
JavaScript Leaders Demand Oracle Release the JavaScript Trademark
In an open letter, JavaScript community leaders urge Oracle to give up the JavaScript trademark, arguing that it has been effectively abandoned through nonuse.
@choc-ui/chakra-autocomplete
Advanced tools
```bash npm i --save @choc-ui/chakra-autocomplete #or yarn add @choc-ui/chakra-autocomplete ```
npm i @choc-ui/chakra-autocomplete
npm i --save @choc-ui/chakra-autocomplete
#or
yarn add @choc-ui/chakra-autocomplete
import {
AutoComplete,
AutoCompleteInput,
AutoCompleteItem,
AutoCompleteList,
} from '@choc-ui/chakra-autocomplete';
export default () => {
const options = ['apple', 'appoint', 'zap', 'cap', 'japan'];
return (
<AutoComplete>
<AutoCompleteInput
variant="filled"
placeholder="Search..."
defaultValue="ap"
autoFocus
/>
<AutoCompleteList rollNavigation>
{options.map((option, oid) => (
<AutoCompleteItem
key={`option-${oid}`}
value={option}
textTransform="capitalize"
>
{option}
</AutoCompleteItem>
))}
</AutoCompleteList>
</AutoComplete>
);
};
You can create groups with the
AutoCompleteGroup
Component
import {
AutoComplete,
AutoCompleteGroup,
AutoCompleteInput,
AutoCompleteItem,
AutoCompleteList,
} from '@choc-ui/chakra-autocomplete';
export default () => {
const fruits = ['Apple', 'Grape', 'Pawpaw'];
const countries = ['Korea', 'Nigeria', 'India'];
return (
<AutoComplete>
<AutoCompleteInput
variant="filled"
placeholder="Search..."
pl="10"
defaultValue="ap"
autoFocus
/>
<AutoCompleteList rollNavigation>
<AutoCompleteGroup title="Fruits" showDivider>
{fruits.map((option, oid) => (
<AutoCompleteItem
key={`fruits-${oid}`}
value={option}
textTransform="capitalize"
>
{option}
</AutoCompleteItem>
))}
</AutoCompleteGroup>
<AutoCompleteGroup title="countries" showDivider>
{countries.map((option, oid) => (
<AutoCompleteItem
key={`countries-${oid}`}
value={option}
textTransform="capitalize"
>
{option}
</AutoCompleteItem>
))}
</AutoCompleteGroup>
</AutoCompleteList>
</AutoComplete>
);
};
You can Render whatever you want. The
AutoComplete
Items are regularChakra
Boxes.
import {
AutoComplete,
AutoCompleteInput,
AutoCompleteItem,
AutoCompleteList,
} from '@choc-ui/chakra-autocomplete';
import { Avatar, Box, Text } from '@chakra-ui/react';
export default () => {
const people = [
{ name: 'Dan Abramov', image: 'https://bit.ly/dan-abramov' },
{ name: 'Kent Dodds', image: 'https://bit.ly/kent-c-dodds' },
{ name: 'Segun Adebayo', image: 'https://bit.ly/sage-adebayo' },
{ name: 'Prosper Otemuyiwa', image: 'https://bit.ly/prosper-baba' },
{ name: 'Ryan Florence', image: 'https://bit.ly/ryan-florence' },
];
return (
<AutoComplete>
<AutoCompleteInput
variant="filled"
placeholder="Search..."
pl="10"
defaultValue="ap"
autoFocus
/>
<AutoCompleteList rollNavigation>
{people.map((person, oid) => (
<AutoCompleteItem
key={`option-${oid}`}
value={person.name}
textTransform="capitalize"
align="center"
>
<Avatar size="sm" name={person.name} src={person.image} />
<Text ml="4">{person.name}</Text>
</AutoCompleteItem>
))}
</AutoCompleteList>
</AutoComplete>
);
};
NB: Feel free to request any additional Prop
in Issues.
Wrapper and Provider for AutoCompleteInput
and AutoCompleteList
AutoComplete composes Box so you can pass all Box props to change its style.
NB: If you have more than one AutoComplete
Component on a page, they must be assigned unique id
s
onChange
Description
Function that provides current Input value and is called anytime, suggestion is selected- useful for uncontrolled Input, but wants to use Value
Type
(value: string) => void
Default
null
Required
No
onSelectOption
Description
Will be called every time suggestion is selected via mouse or keyboard.
Type
(optionValue: string, selectMethod: 'click'|'keyboard') => void;
Default
null
Required
No
onOptionHighlight
Description
Will be called every time the highlighted option changes.
Type
(optionValue: string) => void
Default
null
Required
No
emphasize
Description
The parts of the option string that match the
AutoCompleteInput
value are emphasized. Pass boolean to bolden it, or a ChakraCSSObject
for custom styling. e.g.
<AutoComplete emphasize>
...
</AutoComplete>
<!--Or-->
<AutoComplete emphasize={{ color: 'blue.400', fontWeight: 'bold' }}>
...
</AutoComplete>
Type
boolean | CSSObject;
Default
const emphasizeStyles = {
fontWeight: 'extrabold',
};
Required
No
shouldRenderSuggestions
Description
By default, suggestions are rendered when the input isn't blank. Feel free to override this behaviour. This function gets the current value of the input
e.g. The following function is to show the suggestions only if the input has more than two characters.
function shouldRenderSuggestions(value) {
return value.trim().length > 2;
}
Type
(value: string) => void
Default
null
Required
No
focusInputOnSelect
Description
Determines if Input should be focused after Select
Type
boolean;
Default
true
Required
No
closeOnSelect
Description
If true, the menu will close when an item is selected, by mouse or keyboard.
Type
boolean;
Default
true
Required
No
closeOnBlur
Description
If true, the menu will close when the
AutoComplete
Component loses focus.
Type
boolean;
Default
true
Required
No
suggestWhenEmpty
Description
If the suggestions shoud show when the input is Empty. - It is used when the input is focused.
Type
boolean;
Default
false
Required
No
suggestWhenEmpty
Description
Component to render when no match is found. Pass null, to just close the menu.
Type
ReactNode;
Default
null
Required
No
Input for AutoComplete
value.
AutoComplete composes Input so you can pass all Input props to change its style.
onChange
Description
When you want the AutoComplete in controlled mode, it's a function that returns, the change event anytime the input value changes.
Type
(e: ChangeEVent) => void
Default
undefined
Required
No
value
Description
The value of the
AutoComplete
input in controlled mode. You should store this in state. And it must bes used with theonChange
prop.
Type
string;
Default
undefined
Required
No
Wrapper for AutoCompleteGroup
and AutoCompleteItem
AutoCompleteList composes Box so you can pass all Box props to change its style.
rollNavigation
Description
Determines if keyboard navigation should roll over after getting to either ends.
Type
boolean;
Default
false
Required
No
Wrapper for collections of AutoCompleteItem
s
AutoComplete composes Box so you can pass all Box props to change its style.
showDivider
Description
Determines if a divider should be rendered above the group. The divider will automatically be unrendered when that group is first in that suggestions.
Type
boolean;
Default
null
Required
No
dividerColor
Description
Color for the group divider, when
showDivider
istrue
Type
string;
Default
inherit
Required
No
titleStyles
Description
Styles to be applied to group's
title
. It Composes Text so you can pass all Text props to change its style.
Type
TextProps;
Default
const textStyles: TextProps = {
fontSize: 'xs',
textTransform: 'uppercase',
fontWeight: 'extrabold',
letterSpacing: 'wider',
ml: '5',
};
Required
No
This Composes your suggestions
AutoComplete composes Flex so you can pass all Flex props to change its style.
_focus
Description
Like the default pseudo
_focus
, but this determines the style applied when thatItem
highlighted by mouse or keyboard,
Type
CSSObject;
Default
const _focus = {
bg: useColorModeValue('gray.200', 'whiteAlpha.100'),
};
Required
No
FAQs
Unknown package
The npm package @choc-ui/chakra-autocomplete receives a total of 10,009 weekly downloads. As such, @choc-ui/chakra-autocomplete popularity was classified as popular.
We found that @choc-ui/chakra-autocomplete demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
In an open letter, JavaScript community leaders urge Oracle to give up the JavaScript trademark, arguing that it has been effectively abandoned through nonuse.
Security News
The initial version of the Socket Python SDK is now on PyPI, enabling developers to more easily interact with the Socket REST API in Python projects.
Security News
Floating dependency ranges in npm can introduce instability and security risks into your project by allowing unverified or incompatible versions to be installed automatically, leading to unpredictable behavior and potential conflicts.