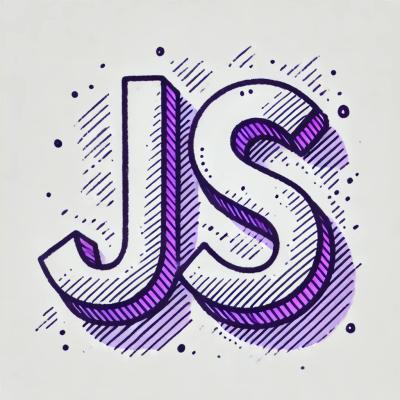
Security News
JavaScript Leaders Demand Oracle Release the JavaScript Trademark
In an open letter, JavaScript community leaders urge Oracle to give up the JavaScript trademark, arguing that it has been effectively abandoned through nonuse.
@choc-ui/chakra-autocomplete
Advanced tools
```bash npm i --save @choc-ui/chakra-autocomplete #or yarn add @choc-ui/chakra-autocomplete ```
npm i @choc-ui/chakra-autocomplete
npm i --save @choc-ui/chakra-autocomplete
#or
yarn add @choc-ui/chakra-autocomplete
import {
AutoComplete,
AutoCompleteInput,
AutoCompleteItem,
AutoCompleteList,
} from '@choc-ui/chakra-autocomplete';
export default () => {
const options = ['apple', 'appoint', 'zap', 'cap', 'japan'];
return (
<AutoComplete rollNavigation>
<AutoCompleteInput
variant="filled"
placeholder="Search..."
defaultValue="ap"
autoFocus
/>
<AutoCompleteList>
{options.map((option, oid) => (
<AutoCompleteItem
key={`option-${oid}`}
value={option}
textTransform="capitalize"
>
{option}
</AutoCompleteItem>
))}
</AutoCompleteList>
</AutoComplete>
);
};
You can create groups with the
AutoCompleteGroup
Component
import {
AutoComplete,
AutoCompleteGroup,
AutoCompleteInput,
AutoCompleteItem,
AutoCompleteList,
} from '@choc-ui/chakra-autocomplete';
export default () => {
const fruits = ['Apple', 'Grape', 'Pawpaw'];
const countries = ['Korea', 'Nigeria', 'India'];
return (
<AutoComplete rollNavigation>
<AutoCompleteInput
variant="filled"
placeholder="Search..."
pl="10"
defaultValue="ap"
autoFocus
/>
<AutoCompleteList>
<AutoCompleteGroup title="Fruits" showDivider>
{fruits.map((option, oid) => (
<AutoCompleteItem
key={`fruits-${oid}`}
value={option}
textTransform="capitalize"
>
{option}
</AutoCompleteItem>
))}
</AutoCompleteGroup>
<AutoCompleteGroup title="countries" showDivider>
{countries.map((option, oid) => (
<AutoCompleteItem
key={`countries-${oid}`}
value={option}
textTransform="capitalize"
>
{option}
</AutoCompleteItem>
))}
</AutoCompleteGroup>
</AutoCompleteList>
</AutoComplete>
);
};
To access the internal state of the
AutoComplete
, use a function as children (commonly known as a render prop). You'll get access to the internal stateisOpen
and methodonClose
.
import {
AutoComplete,
AutoCompleteInput,
AutoCompleteItem,
AutoCompleteList,
} from '@choc-ui/chakra-autocomplete';
import { ChevronDownIcon, ChevronRightIcon } from '@chakra-ui/icons';
import { Icon, InputGroup, InputRightElement } from '@chakra-ui/react';
export default () => {
const options = ['apple', 'appoint', 'zap', 'cap', 'japan'];
return (
<AutoComplete rollNavigation>
{({ isOpen }) => (
<>
<InputGroup>
<AutoCompleteInput variant="filled" placeholder="Search..." />
<InputRightElement
children={
<Icon as={isOpen ? ChevronRightIcon : ChevronDownIcon} />
}
/>
</InputGroup>
<AutoCompleteList>
{options.map((option, oid) => (
<AutoCompleteItem
key={`optio-${oid}`}
value={option}
textTransform="capitalize"
align="center"
>
{option}
</AutoCompleteItem>
))}
</AutoCompleteList>
</>
)}
</AutoComplete>
);
};
Watch the Icon on the right.
You can Render whatever you want. The
AutoComplete
Items are regularChakra
Boxes.
import {
AutoComplete,
AutoCompleteInput,
AutoCompleteItem,
AutoCompleteList,
} from '@choc-ui/chakra-autocomplete';
import { Avatar, Box, Text } from '@chakra-ui/react';
export default () => {
const people = [
{ name: 'Dan Abramov', image: 'https://bit.ly/dan-abramov' },
{ name: 'Kent Dodds', image: 'https://bit.ly/kent-c-dodds' },
{ name: 'Segun Adebayo', image: 'https://bit.ly/sage-adebayo' },
{ name: 'Prosper Otemuyiwa', image: 'https://bit.ly/prosper-baba' },
{ name: 'Ryan Florence', image: 'https://bit.ly/ryan-florence' },
];
return (
<AutoComplete rollNavigation>
<AutoCompleteInput
variant="filled"
placeholder="Search..."
pl="10"
defaultValue="ap"
autoFocus
/>
<AutoCompleteList>
{people.map((person, oid) => (
<AutoCompleteItem
key={`option-${oid}`}
value={person.name}
textTransform="capitalize"
align="center"
>
<Avatar size="sm" name={person.name} src={person.image} />
<Text ml="4">{person.name}</Text>
</AutoCompleteItem>
))}
</AutoCompleteList>
</AutoComplete>
);
};
NB: Feel free to request any additional Prop
in Issues.
Wrapper and Provider for AutoCompleteInput
and AutoCompleteList
AutoComplete composes Box so you can pass all Box props to change its style.
Prop | Type | Description | Required | Default |
---|---|---|---|---|
children |
|
Children can be a function which is provided with the | No | ——— |
onChange |
|
Callback for when the autocomplete value changes, and when input changes if in | No | ——— |
emptyState |
| Component to display when no options are found. set to true to use default. | No | No Options Found |
rollNavigation | boolean | set to true to allow keyboard navigation to restart on both ends. | No | false |
focusInputOnSelect | boolean | Determines if Input should be focused after an option is selected. | No | false |
freeSolo | boolean | Set freeSolo to true so the textbox can contain any arbitrary value. | No | false |
creatable | boolean | Allows creation of new Items, set to true to show `Add {newInput}` It can also be a function that returns a `ReactNode` and is provided with the `newInput` and an `Emphasize` component.
e.g.
When using | No | false |
selectOnFocus | boolean | select the text in input when input is focused | No | false |
openOnFocus | boolean | Open the suggestions once input is focused. | No | false |
emphasize |
| The parts of the option string that match the `AutoCompleteInput` value are emphasized. Pass boolean to bolden it, or a Chakra `CSSObject` for custom styling. | No | false |
defaultIsOpen | boolean | If true, the suggestions menu is open by default | No | false |
onSelectOption |
| Will be called every time suggestion is selected via mouse or keyboard. It returns the selectedValue, the selectionMethod and a boolean specifying if the input is a new one; useFul when combined with creatable mode. | No | ——— |
suggestWhenEmpty | boolean | Return false to prevent selecting the option. | No | false |
closeOnBlur | boolean | If true, the menu will close when the AutoComplete Component loses focus. | No | true |
closeOnselect | boolean | If true, the menu will close when an item is selected, by mouse or keyboard. | No | true |
maxSuggestions | number | Limit the maximum number of suggestions that is shown. | No | ——— |
shouldRenderSuggestions |
|
By default, suggestions are rendered when the input isn't blank. Feel free to override this behaviour. This function gets the current value of the input e.g. The following function is to show the suggestions only if the input has more than two characters.
| No | ——— |
Input for AutoComplete
value.
AutoCompleteInput composes Input so you can pass all Input props to change its style.
Prop | Type | Description | Required | Default |
---|---|---|---|---|
initialFilter | boolean | determines if the options are filtered by default, when a `defaultValue` is provided to the input. | No | true |
Wrapper for AutoCompleteGroup
and AutoCompleteItem
AutoCompleteList composes Box so you can pass all Box props to change its style.
Wrapper for collections of AutoCompleteItem
s
AutoCompleteGroup composes Box so you can pass all Box props to change its style.
Prop | Type | Description | Required | Default |
---|---|---|---|---|
title | string | The group title | No | ——— |
titleStyles |
| Styles for title decoration, if present | No |
|
showDivider | boolean | If true, a divider is shown | No | false |
dividerColor | string | Color for divider, if present | No | inherit |
This Composes your suggestions
AutoCompleteItem composes Flex so you can pass all Flex props to change its style.
Prop | Type | Description | Required | Default |
---|---|---|---|---|
value | string | The value of the Option | yes | ——— |
_focus |
| Styles for focused Item | No |
|
This is an item that is not filtered with the other options, but syncs with the highlight and active states.
AutoCompleteFixedItem composes Flex so you can pass all Flex props to change its style.
AutoCompleteFixedItem composes AutoCompleteItem so you can pass all AutoCompleteItem props to change its style.
Prop | Type | Description | Required | Default |
---|---|---|---|---|
onItemSelect |
| Callback to run when Item is selected my mouse or keyboard. Provides the select method | No | ——— |
Thanks goes to these wonderful people (emoji key):
Abraham 💻 | Sam Margalit 📖 | gepd 💻 |
This project follows the all-contributors specification. Contributions of any kind welcome!
FAQs
Unknown package
The npm package @choc-ui/chakra-autocomplete receives a total of 10,009 weekly downloads. As such, @choc-ui/chakra-autocomplete popularity was classified as popular.
We found that @choc-ui/chakra-autocomplete demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
In an open letter, JavaScript community leaders urge Oracle to give up the JavaScript trademark, arguing that it has been effectively abandoned through nonuse.
Security News
The initial version of the Socket Python SDK is now on PyPI, enabling developers to more easily interact with the Socket REST API in Python projects.
Security News
Floating dependency ranges in npm can introduce instability and security risks into your project by allowing unverified or incompatible versions to be installed automatically, leading to unpredictable behavior and potential conflicts.