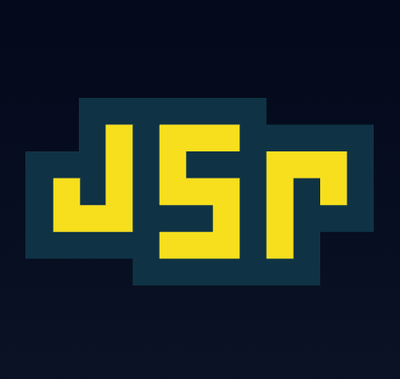
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
@choc-ui/chakra-autocomplete
Advanced tools
```bash npm i --save @choc-ui/chakra-autocomplete #or yarn add @choc-ui/chakra-autocomplete ```
npm i @choc-ui/chakra-autocomplete
npm i --save @choc-ui/chakra-autocomplete
#or
yarn add @choc-ui/chakra-autocomplete
import { Flex, FormControl, FormHelperText, FormLabel } from "@chakra-ui/react";
import * as React from "react";
import {
AutoComplete,
AutoCompleteInput,
AutoCompleteItem,
AutoCompleteList,
} from "@choc-ui/chakra-autocomplete";
function App() {
const countries = [
"nigeria",
"japan",
"india",
"united states",
"south korea",
];
return (
<Flex pt="48" justify="center" align="center" w="full">
<FormControl w="60">
<FormLabel>Olympics Soccer Winner</FormLabel>
<AutoComplete openOnFocus>
<AutoCompleteInput variant="filled" />
<AutoCompleteList>
{countries.map((country, cid) => (
<AutoCompleteItem
key={`option-${cid}`}
value={country}
textTransform="capitalize"
>
{country}
</AutoCompleteItem>
))}
</AutoCompleteList>
</AutoComplete>
<FormHelperText>Who do you support.</FormHelperText>
</FormControl>
</Flex>
);
}
export default App;
You can create groups with the AutoCompleteGroup
Component, and add a title with the AutoCompleteGroupTitle
component.
import { Flex, FormControl, FormHelperText, FormLabel } from "@chakra-ui/react";
import * as React from "react";
import {
AutoComplete,
AutoCompleteGroup,
AutoCompleteGroupTitle,
AutoCompleteInput,
AutoCompleteItem,
AutoCompleteList,
} from "@choc-ui/chakra-autocomplete";
function App() {
const continents = {
africa: ["nigeria", "south africa"],
asia: ["japan", "south korea"],
europe: ["united kingdom", "russia"],
};
return (
<Flex pt="48" justify="center" align="center" w="full">
<FormControl w="60">
<FormLabel>Olympics Soccer Winner</FormLabel>
<AutoComplete openOnFocus>
<AutoCompleteInput variant="filled" />
<AutoCompleteList>
{Object.entries(continents).map(([continent, countries], co_id) => (
<AutoCompleteGroup key={co_id} showDivider>
<AutoCompleteGroupTitle textTransform="capitalize">
{continent}
</AutoCompleteGroupTitle>
{countries.map((country, c_id) => (
<AutoCompleteItem
key={c_id}
value={country}
textTransform="capitalize"
>
{country}
</AutoCompleteItem>
))}
</AutoCompleteGroup>
))}
</AutoCompleteList>
</AutoComplete>
<FormHelperText>Who do you support.</FormHelperText>
</FormControl>
</Flex>
);
}
export default App;
To access the internal state of the AutoComplete
, use a function as children (commonly known as a render prop). You'll get access to the internal state isOpen
, with the onOpen
and onClose
methods.
import {
Flex,
FormControl,
FormHelperText,
FormLabel,
Icon,
InputGroup,
InputRightElement,
} from "@chakra-ui/react";
import * as React from "react";
import {
AutoComplete,
AutoCompleteInput,
AutoCompleteItem,
AutoCompleteList,
} from "@choc-ui/chakra-autocomplete";
import { FiChevronRight, FiChevronDown } from "react-icons/fi";
function App() {
const countries = [
"nigeria",
"japan",
"india",
"united states",
"south korea",
];
return (
<Flex pt="48" justify="center" align="center" w="full">
<FormControl w="60">
<FormLabel>Olympics Soccer Winner</FormLabel>
<AutoComplete openOnFocus>
{({ isOpen }) => (
<>
<InputGroup>
<AutoCompleteInput variant="filled" placeholder="Search..." />
<InputRightElement
children={
<Icon as={isOpen ? FiChevronRight : FiChevronDown} />
}
/>
</InputGroup>
<AutoCompleteList>
{countries.map((country, cid) => (
<AutoCompleteItem
key={`option-${cid}`}
value={country}
textTransform="capitalize"
>
{country}
</AutoCompleteItem>
))}
</AutoCompleteList>
</>
)}
</AutoComplete>
<FormHelperText>Who do you support.</FormHelperText>
</FormControl>
</Flex>
);
}
export default App;
You can Render whatever you want. The AutoComplete
Items are regular Chakra
Boxes.
import {
Avatar,
Flex,
FormControl,
FormHelperText,
FormLabel,
Text,
} from "@chakra-ui/react";
import * as React from "react";
import {
AutoComplete,
AutoCompleteInput,
AutoCompleteItem,
AutoCompleteList,
} from "@choc-ui/chakra-autocomplete";
function App() {
const people = [
{ name: "Dan Abramov", image: "https://bit.ly/dan-abramov" },
{ name: "Kent Dodds", image: "https://bit.ly/kent-c-dodds" },
{ name: "Segun Adebayo", image: "https://bit.ly/sage-adebayo" },
{ name: "Prosper Otemuyiwa", image: "https://bit.ly/prosper-baba" },
{ name: "Ryan Florence", image: "https://bit.ly/ryan-florence" },
];
return (
<Flex pt="48" justify="center" align="center" w="full" direction="column">
<FormControl id="email" w="60">
<FormLabel>Olympics Soccer Winner</FormLabel>
<AutoComplete openOnFocus>
<AutoCompleteInput variant="filled" />
<AutoCompleteList>
{people.map((person, oid) => (
<AutoCompleteItem
key={`option-${oid}`}
value={person.name}
textTransform="capitalize"
align="center"
>
<Avatar size="sm" name={person.name} src={person.image} />
<Text ml="4">{person.name}</Text>
</AutoCompleteItem>
))}
</AutoCompleteList>
</AutoComplete>
<FormHelperText>Who do you support.</FormHelperText>
</FormControl>
</Flex>
);
}
export default App;
Add the multiple
prop to AutoComplete
component, the AutoCompleteInput
will now expose the tags in it's children function.
The onChange
prop now returns an array of the chosen values
Now you can map the tags with the AutoCompleteTag
component or any other component of your choice. The label
and the onRemove
method are now exposed.
import { Flex, FormControl, FormHelperText, FormLabel } from "@chakra-ui/react";
import * as React from "react";
import {
AutoComplete,
AutoCompleteInput,
AutoCompleteItem,
AutoCompleteList,
AutoCompleteTag,
} from "@choc-ui/chakra-autocomplete";
function App() {
const countries = [
"nigeria",
"japan",
"india",
"united states",
"south korea",
];
return (
<Flex pt="48" justify="center" align="center" w="full" direction="column">
<FormControl id="email" w="60">
<FormLabel>Olympics Soccer Winner</FormLabel>
<AutoComplete openOnFocus multiple onChange={vals => console.log(vals)}>
<AutoCompleteInput variant="filled">
{({ tags }) =>
tags.map((tag, tid) => (
<AutoCompleteTag
key={tid}
label={tag.label}
onRemove={tag.onRemove}
/>
))
}
</AutoCompleteInput>
<AutoCompleteList>
{countries.map((country, cid) => (
<AutoCompleteItem
key={`option-${cid}`}
value={country}
textTransform="capitalize"
_selected={{ bg: "whiteAlpha.50" }}
_focus={{ bg: "whiteAlpha.100" }}
>
{country}
</AutoCompleteItem>
))}
</AutoCompleteList>
</AutoComplete>
<FormHelperText>Who do you support.</FormHelperText>
</FormControl>
</Flex>
);
}
export default App;
I know that title hardly expresses the point, but yeah, naming is tough. You might want your users to be able to add extra items when their options are not available in the provided options. e.g. adding a new tag to your Polywork profile.
First add the creatable
prop to the AutoComplete
component.
Then add the AutoCompleteCreatable
component to the bottom of the list. Refer to the references for more info on this component.
NB: Feel free to request any additional Prop
in Issues.
Wrapper and Provider for AutoCompleteInput
and AutoCompleteList
AutoComplete composes Box so you can pass all Box props to change its style.
NB: None of the props passed to it are required.
export type UseAutoCompleteProps = Partial<{
closeOnBlur: boolean; //close suggestions when input is blurred - true
closeOnSelect: boolean; //close suggestions when a suggestions is selected - true
creatable: boolean; //Allow addition of arbitrary values not present in suggestions - false
defaultIsOpen: boolean; //Suggestions list is open by default -- false
emphasize: boolean | CSSObject; //Highlight matching characters in suggestions, you can pass the styles - false
emptyState: boolean | MaybeRenderProp<{ value: Item["value"] }>; //render message when no suggestions match query
filter: (query: string, itemValue: Item["value"]) => boolean; //custom filter function
focusInputOnSelect: boolean; //focus input after a suggestion is selected - true
freeSolo: boolean; //allow entering of any values
maxSuggestions: number; //limit number of suggestions in list
multiple: boolean; //allow tags multi selection - false
onChange: (value: string | Item["value"][]) => void; //function to run whenever autocomplete value(s) changes
onSelectOption: (params: {
optionValue: string;
selectMethod: "mouse" | "keyboard" | null;
isNewInput: boolean;
}) => boolean | void; //method to call whenever a suggestion is selected
onOptionFocus: (params: {
optionValue: string;
selectMethod: "mouse" | "keyboard" | null;
isNewInput: boolean;
}) => boolean | void;//method to call whenever a suggestion is focused
onTagRemoved: (removedTag: Item["value"], tags: Item["value"][]) => void; //method to call whenever a tag is removed
openOnFocus: boolean; //open suggestions when input is focuses -false
rollNavigation: boolean; //allow keyboard navigation to switch to alternate ends when one end is reached
selectOnFocus: boolean; //select the text in input when it's focused. - false
shouldRenderSuggestions: (value: string) => boolean; //function to decide if suggestions should render, e.g. show suggestions only if there are at least two characters in the query value
suggestWhenEmpty: boolean; //show suggestions when input value is empty - false
}>;
}
Tags for multiple mode
AutoCompleteTag composes Tag so you can pass all Tag props to change its style.
Prop | Type | Description | Required | Default |
---|---|---|---|---|
label |
| Label that is displayed on the tag | Yes | ——— |
onRemove |
| Method to remove the tag from selected values | Yes | ——— |
Input for AutoComplete
value.
AutoCompleteInput composes Input so you can pass all Input props to change its style.
Prop | Type | Description | Required | Default |
---|---|---|---|---|
children |
|
callback that returns
| No | ——— |
Wrapper for AutoCompleteGroup
and AutoCompleteItem
AutoCompleteList composes Box so you can pass all Box props to change its style.
Wrapper for collections of AutoCompleteItem
s
AutoCompleteGroup composes Box so you can pass all Box props to change its style.
Prop | Type | Description | Required | Default |
---|---|---|---|---|
showDivider | boolean | If true, a divider is shown | No | false |
dividerColor | string | Color for divider, if present | No | inherit |
This Composes your suggestions
AutoCompleteItem composes Flex so you can pass all Flex props to change its style.
Prop | Type | Description | Required | Default |
---|---|---|---|---|
value | string | The value of the Option | yes | ——— |
fixed |
| Make an item visible at all times, regardless of filtering | No | ——— |
_fixed |
| Styles for fixed Itemm | No |
|
value | string | The value of the Option | yes | ——— |
disabled |
| Make an item disabled, so it cannot be selected | No | ——— |
_disabled |
| Styles for disabled Item(s) | No |
|
_selected |
| Styles for selected Item(s) | No |
|
_focus |
| Styles for focused Item | No |
|
Used with the AutoComplete
component's creatable
prop, to allow users enter arbitrary values, not available in the provided options.
AutoCompleteCreatable composes Flex so you can pass all Flex props to change its style.
It also accepts a function as its children
prop which is provided with the current inputValue
.
Prop | Type | Description | Required | Default |
---|---|---|---|---|
children |
|
| No | ——— |
Thanks goes to these wonderful people (emoji key):
Abraham 💻 | Sam Margalit 📖 | gepd 💻 |
This project follows the all-contributors specification. Contributions of any kind welcome!
FAQs
Unknown package
We found that @choc-ui/chakra-autocomplete demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.