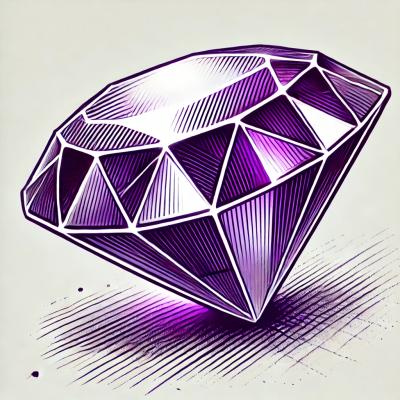
Security News
RubyGems.org Adds New Maintainer Role
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
@cincel.digital/react
Advanced tools
React wrapper for the pdf viewer providing seamless integration with React components.
yarn add @cincel.digital/react
# or
npm i @cincel.digital/react
import * as React from "react"
import {
PDFViewer,
PdfViewer,
PdfViewerCloseButton,
PdfViewerContent,
PdfViewerRenderer,
PdfViewerToolbar,
} from "@cincel.digital/react"
const pv = new PDFViewer({ mode: "read" })
pv.debug = true
function IntegrationTest() {
const [file, setFile] = React.useState<File | null>(null)
const handleFileUpload = async (
event: React.ChangeEvent<HTMLInputElement>,
) => {
const file = event.target.files?.[0]
if (!file) return
setFile(file)
}
return (
<PdfViewer
pdfViewer={pv}
file={file}
url="https://dev.api.cincel.digital/v3/convert-to-pdf"
onClose={() => setFile(null)}
renderEmptyState={(state) => {
if (state.loading) {
return <pre>Converting to PDF...</pre>
} else if (state.empty) {
return (
<div>
<pre>Select a document to convert to PDF.</pre>
<input
type="file"
title="Select a file"
onChange={handleFileUpload}
/>
{state.error && <pre>{JSON.stringify(state.error.message)}</pre>}
</div>
)
}
}}
>
<PdfViewerContent>
<PdfViewerCloseButton />
<PdfViewerToolbar />
<PdfViewerRenderer />
</PdfViewerContent>
</PdfViewer>
)
}
The React integration is built upon an anatomy, which allows for composing the layout in any desired way. Custom styles can be passed to the Reactish, or grouped into multiple elements to achieve the desired effect/layout. For instance, to customize the toolbar, a render prop is provided, enabling the replacement of the render without complications.
<div className="flex space-x-4">
<PdfViewerToolbar>
{({ toolbar }) => (
<div>
<button onClick={toolbar.fullscreen}>fullscreen</button>
<button onClick={toolbar.zoomIn}>Zoom in</button>
<button onClick={toolbar.zoomOut}>Zoom out</button>
</div>
)}
</PdfViewerToolbar>
<PdfViewerToolbar>
{({ toolbar }) => (
<div>
<button onClick={toolbar.text}>Free text</button>
<button onClick={() => toolbar.signature("LJGXQo")}>
Add signature
</button>
</div>
)}
</PdfViewerToolbar>
</div>
This is the value for the Toolbar:
Property | Description |
---|---|
text | Callback function for the "text" action. Invoked when the "Free text" button is clicked. |
date | Callback function for the "date" action. Invoked when the "Add date" button is clicked. |
signature(signerId) | Callback function for the "signature" action. Invoked when the "Add signature" button is clicked. The signerId parameter represents the ID of the signer. |
zoomIn | Function to zoom in the PDF Viewer. |
zoomOut | Function to zoom out the PDF Viewer. |
fullscreen | Function to toggle fullscreen mode for the PDF Viewer. |
Please note that the annotations(text, date & signature) will only be present if
the Viewer was created in edit
mode.
const pv = new PDFViewer({ mode: "edit" })
const signers: Signer[] = [
{ id: "01", color: "#F77036", name: "Alice" },
{ id: "02", color: "#1EF7DA", name: "Bob" },
]
<PdfViewer
...
signers={signers}
>
..
<PdfViewerToolbar>
{({ toolbar, signers }) => (
<div>
<button onClick={toolbar.text}>Free text</button>
<button onClick={() => toolbar.signature(signers[0].id)}>Add signature</button>
</div>
)}
</PdfViewerToolbar>
..
</PdfViewer>
To utilize the date annotation feature, a two-way data binding needs to be
established. For this purpose, it is essential to pass the onBeforeDateChange
property to the toolbar component. This function will be triggered when users
perform a double-click/tap on a date-type annotation. During this event, the
annotation to be modified must be stored in a React state for later use in the
toolbar.date
method.
const [isOpen, actions] = useBoolean()
const [dateToChange, setDateToChange] = React.useState<Annotation<string> | null>(null)
return (<PdfViewer
.....
>
<PdfViewerToolbar
onBeforeDateChange={(annotationToChange) => {
setDateToChange(annotationToChange)
actions.setTrue()
}}
>
{({ toolbar }) => {
return (
<Popover
isOpen={isOpen}
positions={["bottom"]}
onClickOutside={() => actions.setFalse()}
content={() => (
<div
style={{
display: "flex",
flexDirection: "column",
padding: "8px",
background: "white",
}}
>
<input
type="date"
onChange={(e: React.ChangeEvent<HTMLInputElement>) => {
// commit the change
toolbar.date?.({
value: e.target.value,
id: dateToChange?.id,
})
setDateToChange(null)
actions.setFalse()
}}
/>
</div>
)}
>
<button onClick={actions.toggle}>Date</button>
</Popover>
)
}}
</PdfViewerToolbar>
</PdfViewer>)
FAQs
React wrapper for the pdf viewer providing seamless integration with React components.
We found that @cincel.digital/react demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.
Security News
Research
Socket's threat research team has detected five malicious npm packages targeting Roblox developers, deploying malware to steal credentials and personal data.