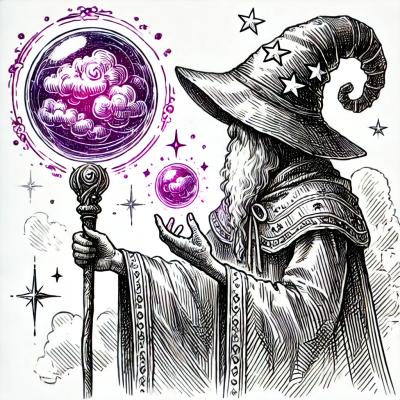
Security News
Cloudflare Adds Security.txt Setup Wizard
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
@ckeditor/ckeditor5-angular
Advanced tools
Official CKEditor 5 Angular 2+ component.
Note: This is development preview. There might be some small bugs and the API might change a little bit.
CKEditor 5 consists of a ready to use builds and a CKEditor 5 Framework upon which the builds are based.
Currently, the CKEditor 5 component for Angular supports integrating CKEditor 5 only via builds. Integrating CKEditor 5 from source is not yet possible due to the lack of ability to adjust webpack configuration in angular-cli
.
In your existing Angular project, install the CKEditor component:
npm install --save-dev @ckeditor/ckeditor5-angular
Install one of the official editor builds:
or create a custom one (e.g. if you want to install more plugins or customize any other thing which cannot be controlled via editor configuration).
Let's pick the @ckeditor/ckeditor5-build-classic
:
npm install --save-dev @ckeditor/ckeditor5-build-classic
Note: You may need to allow external JS in your project's tsconfig.json
for the builds to work properly:
"compilerOptions": {
"allowJs": true
}
Include the CKEditor module:
import { CKEditorModule } from '@ckeditor/ckeditor5-angular';
@NgModule( {
imports: [
...
CKEditorModule,
...
],
...
} )
Import the editor build in your Angular component and assign it to a public
property so it becomes accessible in the template:
import * as ClassicEditor from '@ckeditor/ckeditor5-build-classic';
@Component( {
...
} )
export class MyComponent {
public Editor = ClassicEditor;
...
}
You can import as many editor builds as you want.
Use the <ckeditor>
tag in the template to run the editor
<ckeditor [editor]="Editor" data="<p>Hello world!</p>"></ckeditor>
If you use the Document editor, you need to add the toolbar to the DOM manually.
import * as DecoupledEditor from '@ckeditor/ckeditor5-build-decoupled-document';
@Component( {
...
} )
export class MyComponent {
public Editor = DecoupledEditor;
public onReady( editor ) {
editor.ui.view.editable.element.parentElement.insertBefore(
editor.ui.view.toolbar.element,
editor.ui.view.editable.element
);
}
}
<ckeditor [editor]="Editor" data="<p>Hello world!</p>" (ready)="onReady($event)"></ckeditor>
ngModel
The component implements the ControlValueAccessor
interface and works with the ngModel
.
Create some model in your component to share with the editor:
@Component( {
...
} )
export class MyComponent {
public model = {
editorData: '<p>Hello world!</p>'
};
...
}
Use the model in the template to enable a 2–way data binding:
<ckeditor [(ngModel)]="model.editorData" [editor]="Editor"></ckeditor>
@Inputs
editor
(required)The Editor which provides the static create()
method to create an instance of the editor:
<ckeditor [editor]="Editor"></ckeditor>
config
The configuration of the editor:
<ckeditor [config]="{ toolbar: [ 'heading', '|', 'bold', 'italic' ] }" ...></ckeditor>
data
The initial data of the editor. It can be a static value:
<ckeditor data="<p>Hello world!</p>" ...></ckeditor>
or a shared parent component's property
@Component( {
...
} )
export class MyComponent {
public editorData = '<p>Hello world!</p>';
...
}
<ckeditor [data]="editorData" ...></ckeditor>
tagName
Specifies the tag name of the HTML element on which the editor will be created.
The default tag is div
.
<ckeditor tagName="textarea" ...></ckeditor>
disabled
Controls the editor's read–only state:
@Component( {
...
} )
export class MyComponent {
public isDisabled = false;
...
toggleDisabled() {
this.isDisabled = !this.isDisabled
}
}
<ckeditor [disabled]="isDisabled" ...></ckeditor>
<button (click)="toggleDisabled()">
{{ isDisabled ? 'Enable editor' : 'Disable editor' }}
</button>
@Outputs
ready
Fires when the editor is ready. It corresponds with the editor#ready
event. Fires with the editor instance.
change
Fires when the content of the editor has changed. It corresponds with the editor.model.document#change
event.
Fires with an object containing the editor and the CKEditor5 change event.
blur
Fires when the editing view of the editor is blurred. It corresponds with the editor.editing.view.document#blur
event.
Fires with an object containing the editor and the CKEditor5 blur event.
focus
Fires when the editing view of the editor is focused. It corresponds with the editor.editing.view.document#focus
event.
Fires with an object containing the editor and the CKEditor5 focus event.
Having cloned this repository, install necessary dependencies:
npm install
This repository contains the following code:
./src/ckeditor
contains the CKEditor component,./src/app
a demo application using the component.Note: The npm package contains a packaged component only.
To open a demo application using the component, run:
npm run start
To test it in the production, use:
npm run start -- --prod
To run unit tests, use:
npm run test
To run e2e tests run:
npm run e2e
To run coverage tests run:
npm run coverage
Play with the application and make sure the component works properly.
This project uses the ng-packagr to create a package meeting the Angular Package Format specification. Calling
npm run build-package
creates a package in the ./dist
directory, which can be then published in npm.
To publish the new package in the npm registry, run:
npm run publish
Having generated a package, create a symlink to the ckeditor5-angular/dist
package directory to test it in another (3rd–party) Angular project:
ln -s /path/to/ckeditor5-angular/dist node_modules/\@ckeditor/ckeditor5-angular
You may also need the following config in angular.json
to include the symlinked component package without errors:
{
"project-name": {
"architect": {
"build": {
"options": {
"preserveSymlinks": true
}
}
}
}
}
Licensed under the terms of GNU General Public License Version 2 or later. For full details about the license, please check the LICENSE.md file.
FAQs
Official Angular component for CKEditor 5 – the best browser-based rich text editor.
We found that @ckeditor/ckeditor5-angular demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
Security News
The Socket Research team breaks down a malicious npm package targeting the legitimate DOMPurify library. It uses obfuscated code to hide that it is exfiltrating browser and crypto wallet data.
Security News
ENISA’s 2024 report highlights the EU’s top cybersecurity threats, including rising DDoS attacks, ransomware, supply chain vulnerabilities, and weaponized AI.