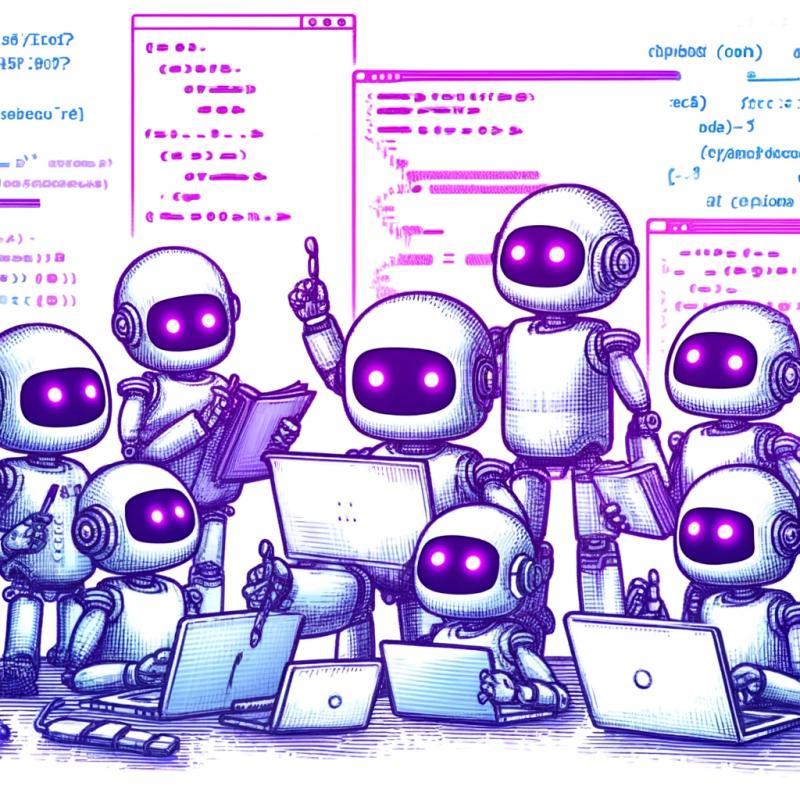
Security News
New Research Shows Teams of LLM Agents Can Autonomously Exploit Zero-Day Vulnerabilities with 53% Success Rate
Researchers have demonstrated that teams of LLM agents can exploit zero-day vulnerabilities with a 53% success rate, and the costs of using AI to do so are rapidly becoming more affordable than hiring a human penetration tester.