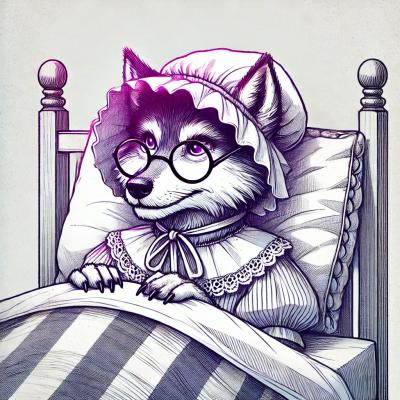
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
@cnhis-frontend/unplugin-i18n-helper
Advanced tools
node version: >=16.0.0
npm i @cnhis-frontend/unplugin-i18n-helper -D
# or
yarn add @cnhis-frontend/unplugin-i18n-helper -D
# or
pnpm install @cnhis-frontend/unplugin-i18n-helper -D
import i18nHelperPlugin from '@cnhis-frontend/unplugin-i18n-helper'
import path from 'path'
export default () => {
return {
plugins: [
i18nHelperPlugin.vite({
includes: ["src/**"],
exclude: ["node_modules/*", "src/i18n.js"],
customI18n: "i18nHelper",
customI18nUrl: "/src/i18n",
dictJson: path.resolve(__dirname, "./src/dict.json"),
raw: true,
output: false,
transforms: ["V3Template"], // vue3 模板编译静态提升优化导致部分内容非响应式 可以增加 V3Template 解决
skipCallExpression: /^fn$/ // 默认值 /^console\.[a-zA-Z]+$/ 不处理console.log 等 console 调用
}),
],
}
}
// 原始代码
const fn = (val) => "(" + val + ")";
const name1 = "一二三"; // 普通字符串
const name2 = `一二三${name1}`; // 模板字符串
const name3 = `${`一二三${name1}`}一二三${fn(name1)}`; // 复杂模板字符串
const name4 = "三" + "2" + "一二三"; // 表达式不参与 只针对字符串和模板字符串
const name5 = "i18n!:一二三"; // i18n!: 开头的内容不参与编译
const name6 = " 一二三 "; // 首尾空格不参与编译 可设置ignorePrefix和ignoreSuffix自定义规则
fn('一二三'); // skipCallExpression 配置 跳过 fn 的入参处理
console.log('一二三')
// 处理后结果
import {i18nHelper} from "/src/i18n.js"
const fn = (val) => "(" + val + ")";
const name1 = i18nHelper("一二三");
const name2 = i18nHelper("一二三{0}",[name1]);
const name3 = i18nHelper("{0}一二三{1}",[i18nHelper("一二三{0}",[name1]),fn(name1)]);
const name4 = i18nHelper("三") + "2" + i18nHelper("一二三");
const name5 = "一二三";
const name6 = ` ${i18nHelper("一二三")} `;
fn('一二三'); // skipCallExpression 配置 跳过 fn 的入参处理
console.log(i18nHelper("一二三"));
json 内容
{
"一二三": "123",
"一二三{0}": "123{0}",
"{0}一二三{1}": "{0}123{1}"
}
// 处理后结果
import {i18nHelper} from "/src/i18n.js"
const fn = val => '(' + val + ')';
const name1 = i18nHelper("123",null,"一二三");
const name2 = i18nHelper("123{0}",[name1],"一二三{0}");
const name3 = i18nHelper("{0}123{1}",[i18nHelper("123{0}",[name1],"一二三{0}"),fn(name1)],"{0}一二三{1}");
const name4 = "三" + "2" + i18nHelper("123",null,"一二三");
const name5 = "一二三";
const name6 = ` ${i18nHelper("123",null,"一二三")} `;
参数 | 类型 | 默认值 | 必填 | 说明 |
---|---|---|---|---|
customI18n | string | - | 是 | 自定义 i18n 方法 |
customI18nUrl | string | - | 是 | 自定义i8n 方法导入地址 |
dictJson | string[] | - | 否 | 匹配字典 |
includes | Array<string|RegExp>|string|RegExp | - | 否 | 匹配文件规则 |
exclude | Array<string|RegExp>|string|RegExp | - | 否 | 忽略文件规则 |
ignoreMark | string | i18n!: | 否 | 忽略以该标识开头的内容 |
ignorePrefix | RegExp | /^\s+/ | 否 | 忽略正则匹配的前缀内容 (默认首尾空格会忽略) |
ignoreSuffix | RegExp | /\s+$/ | 否 | 忽略正则匹配的后缀内容 (默认首尾空格会忽略) |
skipCallExpression | Array<RegExp>|RegExp | /^console.[a-zA-Z]+$/ | 否 | 跳过调用表达式的入参处理(默认不处理console.*()) |
transforms | Array<string> | 否 | 参见 内置 transfrom | |
raw | boolean | - | 否 | 是否保留 dictJson 匹配前的 原始值 (是 将作为customI18n 第三个参数传入) |
output | boolean | - | 否 | 是否输出字符串处理的结果 |
jsx | boolean | - | 否 | 针对jsx的处理 |
// vue.config.js
chainWebpack: config => {
// 将国际化字典内容生成hash
const hash = i18nHelperPlugin.createFileHash(path.resolve(__dirname, './src/dict.json'));
config.module
.rule("vue")
.use("vue-loader")
.tap(options => {
// 找到 vue-loader 的配置 并 设置 cacheIdentifier 加入国际化字典配置的hash
options.cacheIdentifier += hash;
return options;
});
}
// webpack5 的 buildDependencies 里面加入 配置
configureWebpack: {
cache: {
buildDependencies: {
config: [path.resolve(__dirname, './src/dict.json')],
},
}
}
/** 例如在 vue-cli脚手架项目中 */
// 源码
const name = `${1}一二三${2}`;
//预期结果
const name = i18nHelper("{0}123{1}",[1,2],"{0}一二三{1}")
//实际结果
var name = "".concat(1, i18nHelper("123",null,"一二三"), 2);
/**
* 方案一
* 因为 babel-loader 对ES6语法做了转义, 而插件执行顺序是在 babel后
* 所以需要将 babel 处理时机置后 改为enforce="post"
* 注意js 文件中有 jsx 的代码则要启用配置选项jsx避免报错
*/
// vue.config.js
chainWebpack: config => {
config.module
.rule("js")
.post()
.end();
}
/**
* 方案二
* 若不考虑兼容性问题,可以 exclude 选项 关掉 babel 的 模板字符串 处理插件
*/
// babel.config.js
module.exports = {
presets: [
[
"@vue/cli-plugin-babel/preset",
{
exclude: ["transform-template-literals"],
},
],
],
};
FAQs
自动查找 包含中文 的字符串和模板字符串,并替换为自定义的国际化方法
We found that @cnhis-frontend/unplugin-i18n-helper demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.