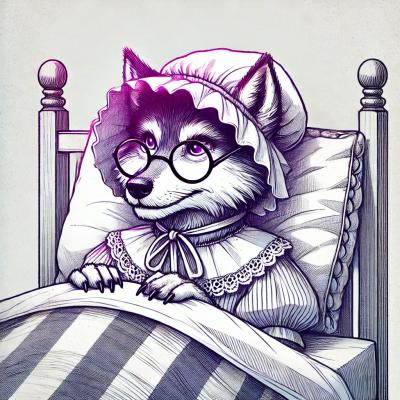
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
@codemirror/legacy-modes
Advanced tools
Collection of ported legacy language modes for the CodeMirror code editor
@codemirror/legacy-modes is a package that provides support for legacy modes in CodeMirror 6. It allows users to utilize syntax highlighting and other features from older versions of CodeMirror in the newer version.
Syntax Highlighting
This code sample demonstrates how to use the @codemirror/legacy-modes package to add Python syntax highlighting to a CodeMirror 6 editor instance.
import { legacyMode } from '@codemirror/legacy-modes';
import { StreamLanguage } from '@codemirror/language';
import { python } from '@codemirror/legacy-modes/mode/python';
const pythonLanguage = StreamLanguage.define(python);
const editor = new EditorView({
state: EditorState.create({
doc: 'print("Hello, World!")',
extensions: [pythonLanguage]
}),
parent: document.body
});
Legacy Mode Integration
This code sample shows how to integrate JavaScript syntax highlighting from legacy CodeMirror modes into a CodeMirror 6 editor.
import { legacyMode } from '@codemirror/legacy-modes';
import { StreamLanguage } from '@codemirror/language';
import { javascript } from '@codemirror/legacy-modes/mode/javascript';
const jsLanguage = StreamLanguage.define(javascript);
const editor = new EditorView({
state: EditorState.create({
doc: 'console.log("Hello, World!");',
extensions: [jsLanguage]
}),
parent: document.body
});
The 'codemirror' package is the original CodeMirror library, which includes a wide range of language modes and features for creating code editors. It is the predecessor to CodeMirror 6 and includes built-in support for many languages, but it does not have the modular architecture of CodeMirror 6.
Prism is a lightweight, extensible syntax highlighter. It is designed to be easy to extend and customize, and it supports a wide range of languages. Unlike @codemirror/legacy-modes, Prism is not tied to a specific editor and can be used in various contexts.
Highlight.js is a syntax highlighter written in JavaScript. It works in the browser as well as on the server. It is similar to @codemirror/legacy-modes in that it provides syntax highlighting for many languages, but it is not specifically designed for integration with CodeMirror.
[ WEBSITE | ISSUES | FORUM | CHANGELOG ]
This package implements a collection of ported stream
language modes for
the CodeMirror code editor. Each mode is
available as a separate script file, under
"@codemirror/legacy-modes/mode/[name]"
, and exports the values
listed below.
The project page has more information, a number of examples and the documentation.
This code is released under an MIT license.
We aim to be an inclusive, welcoming community. To make that explicit, we have a code of conduct that applies to communication around the project.
Using modes from this package works like this:
Install this package and the
@codemirror/language
package.
Find the StreamParser
instance you need in the reference below.
Add StreamLanguage.define(theParser)
to your editor's
configuration.
For example, to load the Lua mode, you'd do something like...
import {StreamLanguage} from "@codemirror/language"
import {lua} from "@codemirror/legacy-modes/mode/lua"
import {EditorView, basicSetup} from "codemirror"
let view = new EditorView({
extensions: [basicSetup, StreamLanguage.define(lua)]
})
apl: StreamParser<unknown>
asciiArmor: StreamParser<unknown>
asn1(conf: {keywords?: Object<any>, cmipVerbs?: Object<any>, compareTypes?: Object<any>, status?: Object<any>, tags?: Object<any>, storage?: Object<any>, modifier?: Object<any>, accessTypes?: Object<any>, multiLineStrings?: boolean}) → StreamParser<unknown>
asterisk: StreamParser<unknown>
brainfuck: StreamParser<unknown>
clike(conf: {name: string, statementIndentUnit?: number, dontAlignCalls?: boolean, keywords?: Object<any>, types?: Object<any>, builtin?: Object<any>, blockKeywords?: Object<any>, atoms?: Object<any>, hooks?: Object<any>, multiLineStrings?: boolean, indentStatements?: boolean, indentSwitch?: boolean, namespaceSeparator?: string, isPunctuationChar?: RegExp, numberStart?: RegExp, number?: RegExp, isOperatorChar?: RegExp, isIdentifierChar?: RegExp, isReservedIdentifier?: fn(id: string) → boolean}) → StreamParser<unknown>
c: StreamParser<unknown>
cpp: StreamParser<unknown>
java: StreamParser<unknown>
csharp: StreamParser<unknown>
scala: StreamParser<unknown>
kotlin: StreamParser<unknown>
shader: StreamParser<unknown>
nesC: StreamParser<unknown>
objectiveC: StreamParser<unknown>
objectiveCpp: StreamParser<unknown>
squirrel: StreamParser<unknown>
ceylon: StreamParser<unknown>
dart: StreamParser<unknown>
clojure: StreamParser<unknown>
cmake: StreamParser<unknown>
cobol: StreamParser<unknown>
coffeeScript: StreamParser<unknown>
commonLisp: StreamParser<unknown>
crystal: StreamParser<unknown>
css: StreamParser<unknown>
sCSS: StreamParser<unknown>
less: StreamParser<unknown>
gss: StreamParser<unknown>
cypher: StreamParser<unknown>
d: StreamParser<unknown>
diff: StreamParser<unknown>
dockerFile: StreamParser<unknown>
dtd: StreamParser<unknown>
dylan: StreamParser<unknown>
ebnf: StreamParser<unknown>
ecl: StreamParser<unknown>
eiffel: StreamParser<unknown>
elm: StreamParser<unknown>
erlang: StreamParser<unknown>
factor: StreamParser<unknown>
fcl: StreamParser<unknown>
forth: StreamParser<unknown>
fortran: StreamParser<unknown>
gas: StreamParser<unknown>
gasArm: StreamParser<unknown>
gherkin: StreamParser<unknown>
go: StreamParser<unknown>
groovy: StreamParser<unknown>
haskell: StreamParser<unknown>
haxe: StreamParser<unknown>
hxml: StreamParser<unknown>
http: StreamParser<unknown>
idl: StreamParser<unknown>
javascript: StreamParser<unknown>
json: StreamParser<unknown>
jsonld: StreamParser<unknown>
typescript: StreamParser<unknown>
jinja2: StreamParser<unknown>
julia: StreamParser<unknown>
liveScript: StreamParser<unknown>
lua: StreamParser<unknown>
mathematica: StreamParser<unknown>
mbox: StreamParser<unknown>
mirc: StreamParser<unknown>
oCaml: StreamParser<unknown>
fSharp: StreamParser<unknown>
sml: StreamParser<unknown>
modelica: StreamParser<unknown>
mscgen: StreamParser<unknown>
msgenny: StreamParser<unknown>
xu: StreamParser<unknown>
mumps: StreamParser<unknown>
nginx: StreamParser<unknown>
nsis: StreamParser<unknown>
ntriples: StreamParser<unknown>
octave: StreamParser<unknown>
oz: StreamParser<unknown>
pascal: StreamParser<unknown>
pegjs: StreamParser<unknown>
perl: StreamParser<unknown>
pig: StreamParser<unknown>
powerShell: StreamParser<unknown>
properties: StreamParser<unknown>
protobuf: StreamParser<unknown>
pug: StreamParser<unknown>
puppet: StreamParser<unknown>
python: StreamParser<unknown>
cython: StreamParser<unknown>
q: StreamParser<unknown>
r: StreamParser<unknown>
rpmChanges: StreamParser<unknown>
rpmSpec: StreamParser<unknown>
ruby: StreamParser<unknown>
rust: StreamParser<unknown>
sas: StreamParser<unknown>
sass: StreamParser<unknown>
scheme: StreamParser<unknown>
shell: StreamParser<unknown>
sieve: StreamParser<unknown>
interface
RulesimpleMode<K extends string>(states: {[P in K]: P extends "languageData" ? Object<any> : Rule[]} & {start: Rule[]}) → StreamParser<unknown>
smalltalk: StreamParser<unknown>
solr: StreamParser<unknown>
sparql: StreamParser<unknown>
spreadsheet: StreamParser<unknown>
sql(conf: {client?: Object<any>, atoms?: Object<any>, builtin?: Object<any>, keywords?: Object<any>, operatorChars?: RegExp, support?: Object<any>, hooks?: Object<any>, dateSQL?: Object<any>, backslashStringEscapes?: boolean, brackets?: RegExp, punctuation?: RegExp}) → StreamParser<unknown>
standardSQL: StreamParser<unknown>
msSQL: StreamParser<unknown>
mySQL: StreamParser<unknown>
mariaDB: StreamParser<unknown>
sqlite: StreamParser<unknown>
cassandra: StreamParser<unknown>
plSQL: StreamParser<unknown>
hive: StreamParser<unknown>
pgSQL: StreamParser<unknown>
gql: StreamParser<unknown>
gpSQL: StreamParser<unknown>
sparkSQL: StreamParser<unknown>
esper: StreamParser<unknown>
stex: StreamParser<unknown>
stexMath: StreamParser<unknown>
stylus: StreamParser<unknown>
swift: StreamParser<unknown>
tcl: StreamParser<unknown>
textile: StreamParser<unknown>
tiddlyWiki: StreamParser<unknown>
tiki: StreamParser<unknown>
toml: StreamParser<unknown>
troff: StreamParser<unknown>
ttcnCfg: StreamParser<unknown>
ttcn: StreamParser<unknown>
turtle: StreamParser<unknown>
vb: StreamParser<unknown>
vbScript: StreamParser<unknown>
vbScriptASP: StreamParser<unknown>
velocity: StreamParser<unknown>
verilog: StreamParser<unknown>
tlv: StreamParser<unknown>
vhdl: StreamParser<unknown>
wast: StreamParser<unknown>
webIDL: StreamParser<unknown>
xml: StreamParser<unknown>
html: StreamParser<unknown>
xQuery: StreamParser<unknown>
yacas: StreamParser<unknown>
yaml: StreamParser<unknown>
z80: StreamParser<unknown>
ez80: StreamParser<unknown>
6.4.0 (2024-04-05)
Only match Solr operator words when they are upper-case.
Fix an infinite loop when tokenizing heredoc strings in the Crystal mode.
Add the old Pug mode.
FAQs
Collection of ported legacy language modes for the CodeMirror code editor
The npm package @codemirror/legacy-modes receives a total of 0 weekly downloads. As such, @codemirror/legacy-modes popularity was classified as not popular.
We found that @codemirror/legacy-modes demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.