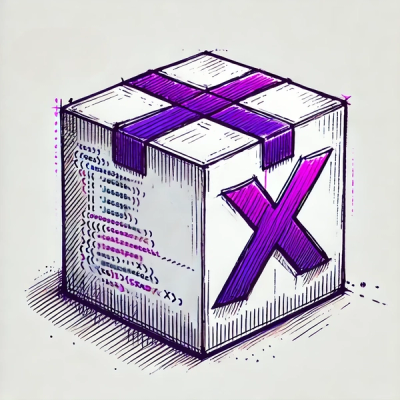
Security News
pnpm 10.0.0 Blocks Lifecycle Scripts by Default
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.
@condenast/cna-st-codec
Advanced tools
Standalone library to service all cna.st encoding requests
@condenast/cna-st-codec
Standalone library to service all cna.st encoding requests. Below are the services provided by the library:
npm install @condenast/cna-st-codec
Documentation: Click here
Query Parameter | Type | Required | Example | Description |
---|---|---|---|---|
origin | string | yes | 60d5a8e87a19ee043b25fb5b | Origin of this link (ex: Copilot page id, newsletter id, etc) |
locale | string | no | UK | Used for localised links. |
brand | string | yes | 4gKgcF5Myw5MXdcFAvDQL5L1iAGH | Id of the source’s brand |
channel | string | no | newsletter, website, instagram | The channel where the click originated |
campaign | string | no | summersale | Custom Campaign parameter |
product | string | no | 30d5a8eKa919ee043b25fP9IK | ID of product |
component | string | no | PLP-Carousel_4 | Name to identify which component is generating revenue (including the position) |
bucket | string | no | Br1639018827824exx | A+B test cell bucket ID |
actionId | string | no | 123456 | Unique ID to reference a click back to a conversion with GA |
offerUrl | string | yes | Retailer product/offer URL | |
referrer | string | no | Origin URL where click happened | |
source | string | no | verso | source name eg: verso, amp |
const cnaStCodec = require('@condenast/cna-st-codec');
const params = {
brand: "4gKgcEs3YBmxYhgNxdHg8VnbgTnc",
offerUrl: "http://www.amazon.com/",
origin: "60d5a8e87a19ee043b25fb5b"
};
let encodedText = cnaStCodec.encodeAffiliateParams(params);
console.log(encodedText);
// Output: 4jjCSCMwBgMHssUvJ6jQbjEfYZMoqQmMUyrkXyfPygqXC4jXjS8nfaa7CwiuVicTz35jocCjuWUSzdvRi6RpqSkKB7ybSrg4KShpeGTbBdLesvvhbgo
const cnaStCodec = require('@condenast/cna-st-codec');
let encodedText = "4jjCSCMwBgMHssUvJ6jQbjEfYZMoqQmMUyrkXyfPygqXC4jXjS8nfaa7CwiuVicTz35jocCjuWUSzdvRi6RpqSkKB7ybSrg4KShpeGTbBdLesvvhbgo";
const params = {
offerUrl: "http://go.skimlinks.com/",
campaign: "test"
};
encodedText = cnaStCodec.encodeAffiliateParams(encodedText, params);
console.log(encodedText);
// Output: RTBwSQLjveX2mJoa9QBGwjuqBsCuZwtP3mdimfraBSaLWCnYkwWyZL85Nvj1ML5qYfokBdnhojbDXLW4mWjBPvotMHwFYNCg4YLYAaSRCYB4eaT79vayNPto
const cnaStCodec = require('@condenast/cna-st-codec');
let encodedText = "4jjCSCMwBgMHssUvJ6jQbjEfYZMoqQmMUyrkXyfPygqXC4jXjS8nfaa7CwiuVicTz35jocCjuWUSzdvRi6RpqSkKB7ybSrg4KShpeGTbBdLesvvhbgo";
let decoded = cnaStCodec.decodeAffiliateParams(encodedText);
console.log(decoded);
// Output: {
// referrer: '',
// actionId: '',
// bucket: '',
// component: '',
// campaign: 'test',
// channel: '',
// locale: '',
// origin: '60d5a8e87a19ee043b25fb5b',
// product: '',
// offerUrl: 'https://go.skimlinks.com',
// brand: '4gKgcEs3YBmxYhgNxdHg8VnbgTnc'
// }
FAQs
Standalone library to service all cna.st encoding requests
The npm package @condenast/cna-st-codec receives a total of 366 weekly downloads. As such, @condenast/cna-st-codec popularity was classified as not popular.
We found that @condenast/cna-st-codec demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 377 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.
Product
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
Research
Security News
Socket researchers have discovered multiple malicious npm packages targeting Solana private keys, abusing Gmail to exfiltrate the data and drain Solana wallets.