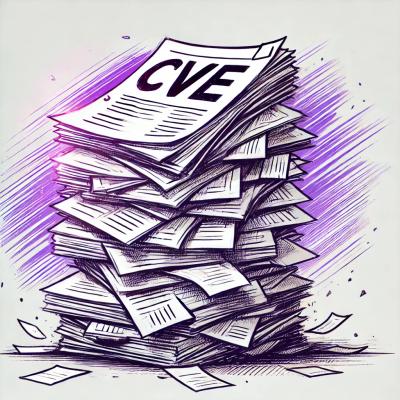
Security News
Node.js EOL Versions CVE Dubbed the "Worst CVE of the Year" by Security Experts
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
@corefunc/class-fillable-dto
Advanced tools
Class Fillable DTO with validation functionality. 🟩 NodeJS only.
class MyCoolDto extends FillableDto {
public shouldDisplayMessage: boolean;
}
const INCLUDE_KEYS: ReadonlyArray<keyof IMyCoolDto> = [
'shouldDisplayMessage',
] as const;
class MyCoolDto extends FillableDto implements IMyCoolDto {
public readonly shouldDisplayMessage: boolean;
public constructor(attributes: Partial<IMyCoolDto>) {
super(attributes, INCLUDE_KEYS);
}
}
const myCoolDto = new MyCoolDto({
shouldDisplayMessage: false,
thisPropertyWillBeStripped: true,
});
const DEFAULT_VALUES: Readonly<IMyCoolDto> = {
shouldDisplayMessage: false,
} as const;
class MyCoolDto extends FillableDto implements IMyCoolDto {
public readonly shouldDisplayMessage: boolean;
public constructor(attributes: Partial<IMyCoolDto>) {
super(attributes, undefined, DEFAULT_VALUES);
}
}
DO NOT SET DEFAULT VALUES IN CLASS PROPERTIES!!!
Attributes argument passed to the constructor will be overwritten with a class property default value.
const DEFAULT_VALUES: Readonly<IMyCoolDto> = {
shouldDisplayMessage: false,
} as const;
class MyCoolDto extends FillableDto implements IMyCoolDto {
public readonly isActive: boolean = false; // 🛑✋⚠️ No!!!
public readonly shouldDisplayMessage: boolean;
public constructor(attributes: Partial<IMyCoolDto>) {
super(attributes, undefined, DEFAULT_VALUES);
}
}
const myCoolDto = new MyCoolDto({
isActive: true,
shouldDisplayMessage: false,
});
// `false` as in class property default declaration
console.log(myCoolDto.isActive); // false
interface IMyCoolDto {
shouldDisplayMessage: boolean;
}
const INCLUDE_KEYS: ReadonlyArray<keyof IMyCoolDto> = [
'shouldDisplayMessage',
] as const;
const DEFAULT_VALUES: Readonly<IMyCoolDto> = {
shouldDisplayMessage: false,
} as const;
class MyCoolDto extends FillableDto implements IMyCoolDto {
public readonly shouldDisplayMessage: boolean;
public constructor(attributes: Partial<IMyCoolDto>) {
super(attributes, INCLUDE_KEYS, DEFAULT_VALUES);
}
}
import { IsBoolean } from 'class-validator';
class MyCoolDto extends FillableDto implements IMyCoolDto {
@IsBoolean()
public readonly shouldDisplayMessage: boolean;
public constructor(attributes: Partial<IMyCoolDto>) {
super(attributes, INCLUDE_KEYS, DEFAULT_VALUES);
}
}
const attributes = { shouldDisplayMessage: true };
const includeKeys = ["isActive", "shouldDisplayMessage"];
const defaults = { isActive: true };
const myCoolDto = new MyCoolDto(attributes, includeKeys, defaults);
// re-assing everithing
myCoolDto.assign(attributes, includeKeys, defaults);
const myCoolDtoFromJSON = MyCoolDto
.fromJSON(`{"shouldDisplayMessage":true}`);
const myCoolDtoFromObject = MyCoolDto
.fromPlain({ shouldDisplayMessage: true });
const isValid = myCoolDto.isValid(true); // silent
myCoolDto.isValid(false); // throws error
const error: null | string = myCoolDto.getError();
const errors: string[] = myCoolDto.getErrors();
myCoolDto.toJSON(); // creates plain object clone
myCoolDto.toObject(); // creates plain object clone
myCoolDto.toString(); // object packed in JSON string
myCoolDto.lock(); // prevents further modifications
import { validateInstance } from '@corefunc/class-fillable-dto';
// Empty array if there is no errors.
validateInstance(new ClassName());
// ['Provided value is not an object. Value is [null].']
validateInstance(null);
FAQs
Fillable DTO with validation
The npm package @corefunc/class-fillable-dto receives a total of 6 weekly downloads. As such, @corefunc/class-fillable-dto popularity was classified as not popular.
We found that @corefunc/class-fillable-dto demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
Security News
cURL and Go security teams are publicly rejecting CVSS as flawed for assessing vulnerabilities and are calling for more accurate, context-aware approaches.
Security News
Bun 1.2 enhances its JavaScript runtime with 90% Node.js compatibility, built-in S3 and Postgres support, HTML Imports, and faster, cloud-first performance.