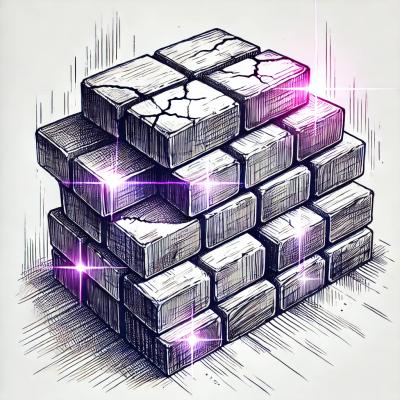
Security News
Input Validation Vulnerabilities Dominate MITRE's 2024 CWE Top 25 List
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
@corentinth/chisels
Advanced tools
Collection of utilities for JavaScript and TypeScript, lightweight and tree-shakable.
Opinionated collection of reusable JS/TS utilities and types.
# Using npm
npm install @corentinth/chisels
# Using yarn
yarn add @corentinth/chisels
# Using pnpm
pnpm add @corentinth/chisels
// Using ES6 import
import { safely } from '@corentinth/chisels';
// Using CommonJS require
const { safely } = require('@corentinth/chisels');
const [result, error] = safely(mayThrowError);
console.log({ result, error });
safelySync
Function - See source
Safely executes a function and return a tuple with the result and an error if any.
const [result, error] = safelySync(myFunction);
if (error) {
console.error(error);
}
console.log(result);
safely
Function - See source
Safely executes an async function or promise and return a tuple with the result and an error if any.
const [result, error] = await safely(myFunction);
if (error) {
console.error(error);
}
console.log(result);
formatBytes
Function - See source
Formats a number of bytes into a human-readable string.
const formatted = formatBytes({ bytes: 4194304 });
console.log(formatted); // 4 MiB
castError
Function - See source
Casts an unknown value to an Error.
try {
// ...
} catch (rawError) {
const error = castError(rawError);
// Do something with a proper Error instance
}
joinUrlPaths
Function - See source
Join URL parts and trim slashes.
const url = joinUrlPaths('/part1/', '/part2/', 'part3', 'part4/');
console.log(url); // 'part1/part2/part3/part4'
buildUrl
Function - See source
Functional wrapper around URL constructor to build an URL string from a base URL and optional path, query params and hash.
const url = buildUrl({ baseUrl: 'https://example.com', path: 'foo', queryParams: { a: '1', b: '2' }, hash: 'hash' });
console.log(url); // 'https://example.com/foo?a=1&b=2#hash'
injectArguments
Function - See source
Injects arguments into a set of functions. Useful for DI of repositories, services, etc.
const functions = {
getUser: ({ userId, db }) => db.users.find({ id: userId }),
removeUser: ({ userId, db }) => db.users.remove({ id: userId }),
};
const { getUser, removeUser } = injectArguments(functions, { db });
getUser({ userId: 1 });
removeUser({ userId: 1 });
PartialBy
Type alias - See source
Make some properties of T optional
type User = {
id: number;
name: string;
email: string;
};
type PartialUser = PartialBy<User, 'email' | 'name'>;
const user: PartialUser = { id: 1 };
Expand
Type alias - See source
Flatten an object type for better IDE support
Dictionary
Type alias - See source
Record<string, T> alias
const dictionary: Dictionary<number> = {
a: 1,
b: 2,
};
DeepPartial
Type alias - See source
Make all properties of T optional recursively
Subtract
Type alias - See source
Exclude properties of T that are in U
type User = {
id: number;
name: string;
email: string;
};
type WithId = {
id: number;
}
type UserWithoutId = Subtract<User, WithId>;
This project is licensed under the MIT License. See the LICENSE file for more information.
This project is crafted with ❤️ by Corentin Thomasset.
FAQs
Collection of utilities for JavaScript and TypeScript, lightweight and tree-shakable.
The npm package @corentinth/chisels receives a total of 20 weekly downloads. As such, @corentinth/chisels popularity was classified as not popular.
We found that @corentinth/chisels demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.