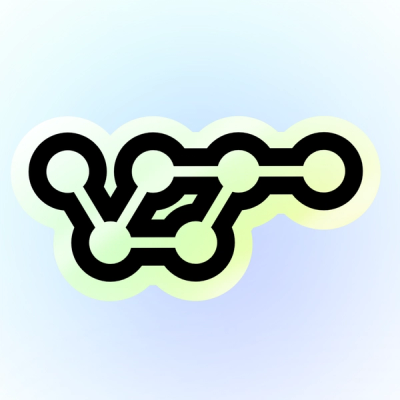
Security News
vlt Debuts New JavaScript Package Manager and Serverless Registry at NodeConf EU
vlt introduced its new package manager and a serverless registry this week, innovating in a space where npm has stagnated.
@cucumber/cucumber
Advanced tools
@cucumber/cucumber is a popular tool for Behavior-Driven Development (BDD). It allows you to write tests in a natural language that non-programmers can read, using Gherkin syntax. This makes it easier for teams to collaborate on defining the behavior of an application.
Defining Features with Gherkin
This feature allows you to define the behavior of your application in plain language using Gherkin syntax. The example defines a feature for user login with a scenario for successful login.
Feature: User login
Scenario: Successful login
Given the user is on the login page
When the user enters valid credentials
Then the user should be redirected to the dashboard
Step Definitions
Step definitions are the glue between Gherkin steps and the actual code that executes them. This example shows how to implement the steps defined in the Gherkin feature.
const { Given, When, Then } = require('@cucumber/cucumber');
Given('the user is on the login page', function () {
// code to navigate to login page
});
When('the user enters valid credentials', function () {
// code to enter credentials
});
Then('the user should be redirected to the dashboard', function () {
// code to check redirection
});
Running Tests
You can run your Cucumber tests using the `cucumber-js` command. This will execute all the feature files and their corresponding step definitions.
npx cucumber-js
jest-cucumber is a library that allows you to use Gherkin syntax with Jest, a popular JavaScript testing framework. It provides similar functionality to @cucumber/cucumber but is integrated with Jest, making it easier to use if you are already using Jest for your unit tests.
cypress-cucumber-preprocessor is a plugin for Cypress that allows you to write your Cypress tests in Gherkin syntax. It combines the power of Cypress for end-to-end testing with the readability of Cucumber.
CodeceptJS is an end-to-end testing framework that supports BDD-style tests using Gherkin syntax. It provides a high-level API for writing tests and supports multiple backends like WebDriver, Puppeteer, and TestCafe.
Automated tests in plain language, for Node.js
Cucumber is a tool for running automated tests written in plain language. Because they're written in plain language, they can be read by anyone on your team. Because they can be read by anyone, you can use them to help improve communication, collaboration and trust on your team.
This is the JavaScript implementation of Cucumber. It runs on maintained versions of Node.js. You can quickly try it via CodeSandbox, or read on to get started locally in a couple of minutes.
Looking to contribute? Read our code of conduct first, then check the contributing guide to get up and running.
Cucumber is available on npm:
$ npm install @cucumber/cucumber
Let's take this example of something to test:
class Greeter {
sayHello() {
return 'hello'
}
}
First, write your feature in features/greeting.feature
:
Feature: Greeting
Scenario: Say hello
When the greeter says hello
Then I should have heard "hello"
Next, implement your steps in features/support/steps.js
:
const assert = require('assert')
const { When, Then } = require('@cucumber/cucumber')
const { Greeter } = require('../../src')
When('the greeter says hello', function () {
this.whatIHeard = new Greeter().sayHello()
});
Then('I should have heard {string}', function (expectedResponse) {
assert.equal(this.whatIHeard, expectedResponse)
});
Finally, run Cucumber:
$ npx cucumber-js
And see the output:
If you learn best by example, we have a repo with several example projects, that might help you get going.
The following documentation is for main
, which might contain some unreleased features. See documentation for older versions if you need it.
Support is available from the community if you need it.
FAQs
The official JavaScript implementation of Cucumber.
The npm package @cucumber/cucumber receives a total of 1,208,477 weekly downloads. As such, @cucumber/cucumber popularity was classified as popular.
We found that @cucumber/cucumber demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt introduced its new package manager and a serverless registry this week, innovating in a space where npm has stagnated.
Security News
Research
The Socket Research Team uncovered a malicious Python package typosquatting the popular 'fabric' SSH library, silently exfiltrating AWS credentials from unsuspecting developers.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.