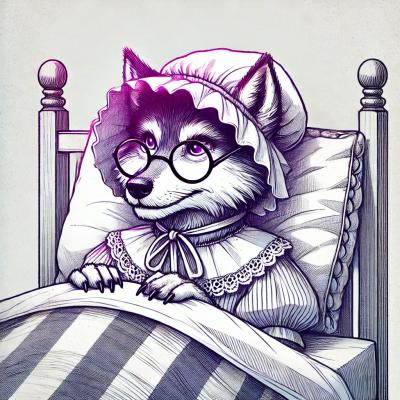
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
@cuvva/postcodesio-client
Advanced tools
Abstracts access to the postcodes.io
API.
var PostcodesIO = require('postcodesio-client');
var postcodes = new PostcodesIO();
postcodes.lookup('EC1V 9LB').then(postcode => {
console.log(postcode);
// {
// "postcode": "EC1V 9LB",
// "admin_district": "Islington",
// "longitude": -0.091247681768113,
// "latitude": 51.5278436902703,
// "region": "London",
// ...
// }
});
$ npm install postcodesio-client
Create an instance of the client, providing options if you wish:
var PostcodesIO = require('postcodesio-client');
var postcodes = new PostcodesIO('https://api.postcodes.io', {
headers: { 'User-Agent': 'MyAwesomeApp/1.0.0' } // default {} - extra headers
});
Make requests using Promises:
postcodes
.lookup('EC1V 9LB')
.then(function (postcode) {
console.log(postcode);
}, function (error) {
console.log('oh no, it failed ;_;');
});
Not found (404) responses from the API are not considered errors. Instead, the
output will be null
.
postcodes.lookup('F4K3AA').then(postcode => {
console.log(postcode);
// null
});
Only Promises (Promises/A+) are supported - callbacks are not. The following two sections specify exactly how you can apply Promises to the reference below.
postcodes
.method(parameters...)
.then(function (outputs...) {
}, function (error) {
});
method
- the method you want to callparameters
- any arguments specific to that method (see Methods)error
- instance of Error
outputs
- any outputs for that method (see Methods)Both parameters
and outputs
can mean zero, one, or many arguments. These are
specified for each method.
Get lots of data for a postcode or outcode.
postcodes.lookup(code)
Parameters:
code
- string - the postcode or outcode to retrieveOutputs:
This may also be called explicitly as postcodes.lookupPostcode(postcode)
or postcodes.lookupOutcode(outcode)
.
Find postcodes closest to a coordinate.
postcodes.near(latitude, longitude)
This may also be called explicitly as postcodes.nearCoordinate(latitude, longitude, options)
.
Parameters:
latitude
- number - the latitude of the coordinatelongitude
- number - the longitude of the coordinateoptions
- object - optional, only available with an explicit callOutputs:
postcodes
- array of Postcode - the nearby postcodesFind the postcodes closest to another postcode.
Warning: results may include the original postcode.
postcodes.near(postcode)
This may also be called explicitly as postcodes.nearPostcode(postcode)
.
Parameters:
postcode
- string - the postcode to search aroundOutputs:
postcodes
- array of Postcode - the nearby postcodesFind the single closest postcode to a coordinate.
postcodes.reverseGeocode(latitude, longitude)
Parameters:
latitude
- number - the latitude of the coordinatelongitude
- number - the longitude of the coordinateOutputs:
postcode
- Postcode - the nearby postcodeValidates that the postcode exists. (Means it is in the official Royal Mail Postcode Address File).
postcodes.validate(postcode)
Parameters:
postcode
- string - the postcode to retrieveOutputs:
exists
- boolean - indicates existence of postcodeRetrieve a random postcode. Not really sure why you'd want to do this, but here it is...
postcodes.random()
Outputs:
postcode
- Postcode - a random postcodeExample:
{
"postcode": "EC1V 9LB",
"quality": 1,
"eastings": 532506,
"northings": 182719,
"country": "England",
"nhs_ha": "London",
"admin_county": null,
"admin_district": "Islington",
"admin_ward": "Bunhill",
"longitude": -0.091247681768113,
"latitude": 51.5278436902703,
"parliamentary_constituency": "Islington South and Finsbury",
"european_electoral_region": "London",
"primary_care_trust": "Islington",
"region": "London",
"parish": null,
"lsoa": "Islington 023A",
"msoa": "Islington 023",
"nuts": null,
"incode": "9LB",
"outcode": "EC1V",
"ccg": "NHS Islington"
}
Example:
{
"outcode": "EC1V",
"longitude": -0.0981811622126924,
"latitude": 51.5266761246198,
"northings": 182576,
"eastings": 532028,
"admin_district": [
"Hackney",
"Islington"
],
"parish": [
"Hackney, unparished area",
"Islington, unparished area"
],
"admin_county": [],
"admin_ward": [
"Bunhill",
"Hoxton West",
"Clerkenwell",
"Hoxton East & Shoreditch",
"St Peter's"
],
"country": [
"England"
]
}
Install the development dependencies first:
$ npm install
Then run the tests:
$ npm test
Please open an issue on this repository.
MIT licensed - see LICENSE file
FAQs
Abstracts access to the Postcodes.io API
We found that @cuvva/postcodesio-client demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.