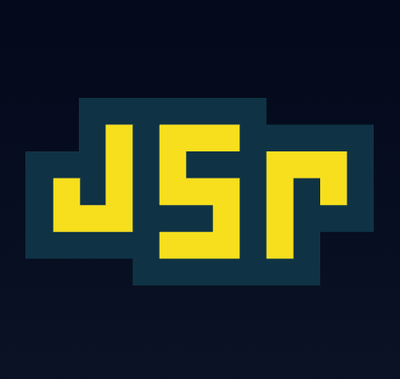
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
@cypress/browserify-preprocessor
Advanced tools
Cypress preprocessor for bundling JavaScript via browserify
@cypress/browserify-preprocessor is a Cypress plugin that allows you to use Browserify to bundle your test files. This is particularly useful for transforming and bundling JavaScript files using various Browserify plugins and transforms before they are executed in the Cypress test runner.
Custom Browserify Options
This feature allows you to customize the Browserify options, such as enabling Babel transformations. The code sample demonstrates how to modify the default options to enable Babel transformations by setting `babelrc` to true.
const browserify = require('@cypress/browserify-preprocessor');
module.exports = (on) => {
const options = browserify.defaultOptions;
options.browserifyOptions.transform[1][1].babelrc = true;
on('file:preprocessor', browserify(options));
};
Adding Custom Transforms
This feature allows you to add custom transforms to the Browserify bundling process. The code sample shows how to add the `envify` transform to the Browserify options.
const browserify = require('@cypress/browserify-preprocessor');
module.exports = (on) => {
const options = browserify.defaultOptions;
options.browserifyOptions.transform.push(['envify', { global: true }]);
on('file:preprocessor', browserify(options));
};
Using TypeScript with Browserify
This feature allows you to use TypeScript with Browserify by adding the `tsify` plugin and including `.ts` file extensions. The code sample demonstrates how to configure Browserify to handle TypeScript files.
const browserify = require('@cypress/browserify-preprocessor');
module.exports = (on) => {
const options = browserify.defaultOptions;
options.browserifyOptions.extensions.push('.ts');
options.browserifyOptions.plugin.unshift(['tsify']);
on('file:preprocessor', browserify(options));
};
The `cypress-rollup-preprocessor` package enables you to use Rollup to bundle your Cypress test files. Like `@cypress/browserify-preprocessor`, it allows for custom transformations and plugins, but it uses Rollup as the bundler. Rollup is known for its efficient tree-shaking capabilities, which can result in smaller bundle sizes.
The `cypress-esbuild-preprocessor` package allows you to use esbuild to bundle your Cypress test files. Esbuild is known for its speed and efficiency, making it a good alternative to Browserify for faster build times. This package provides similar functionality but leverages the performance benefits of esbuild.
Cypress preprocessor for bundling JavaScript via browserify.
This is the default preprocessor that comes packaged with Cypress. You'd typically need to install this if you want to modify the default options we've configured.
Modifying the default options allows you to add support for things like:
Requires Node version 6.5.0 or above.
npm install --save-dev @cypress/browserify-preprocessor
In your project's plugins file:
const browserify = require('@cypress/browserify-preprocessor')
module.exports = (on) => {
on('file:preprocessor', browserify())
}
Pass in options as the second argument to browserify
:
module.exports = (on) => {
const options = {
// options here
}
on('file:preprocessor', browserify(options))
}
Object of options passed to browserify.
// example
browserify({
browserifyOptions: {
extensions: ['.js', '.ts'],
plugin: [
['tsify']
]
}
})
If you pass one of the top-level options in (extensions
, transform
, etc), it will override the default. In the above example, browserify will process .js
and .ts
files, but not .jsx
or .coffee
. If you wish to add to or modify existing options, read about modifying the default options.
watchify is automatically configured as a plugin (as needed), so it's not necessary to manually specify it.
Source maps are always enabled unless explicitly disabled by specifying debug: false
.
Default:
{
extensions: ['.js', '.jsx', '.coffee'],
transform: [
[
'coffeeify',
{}
],
[
'babelify',
{
ast: false,
babelrc: false,
plugins: [
'babel-plugin-add-module-exports',
'@babel/plugin-proposal-class-properties',
'@babel/plugin-proposal-object-rest-spread',
'@babel/plugin-transform-runtime',
],
presets: [
'@babel/preset-env',
'@babel/preset-react',
]
},
]
],
debug: true,
plugin: [],
cache: {},
packageCache: {}
}
Note: cache
and packageCache
are always set to {}
and cannot be overridden. Otherwise, file watching would not function correctly.
Object of options passed to watchify
// example
browserify({
watchifyOptions: {
delay: 500
}
})
Default:
{
ignoreWatch: [
'**/.git/**',
'**/.nyc_output/**',
'**/.sass-cache/**',
'**/bower_components/**',
'**/coverage/**',
'**/node_modules/**'
],
}
A function that is called with the browserify bundle. This allows you to specify external files and plugins. See the browserify docs for methods available.
// example
browserify({
onBundle (bundle) {
bundle.external('react')
bundle.plugin('some-plugin')
}
})
Default: undefined
The default options are provided as browserify.defaultOptions
so they can be more easily modified.
If, for example, you want to update the options for the babelify
transform to turn on babelrc
loading, you could do the following:
const browserify = require('@cypress/browserify-preprocessor')
module.exports = (on) => {
const options = browserify.defaultOptions
options.browserifyOptions.transform[1][1].babelrc = true
on('file:preprocessor', browserify(options))
}
Run all tests once:
npm test
Run tests in watch mode:
npm run test-watch
This project is licensed under the terms of the MIT license.
FAQs
Cypress preprocessor for bundling JavaScript via browserify
The npm package @cypress/browserify-preprocessor receives a total of 343,866 weekly downloads. As such, @cypress/browserify-preprocessor popularity was classified as popular.
We found that @cypress/browserify-preprocessor demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.