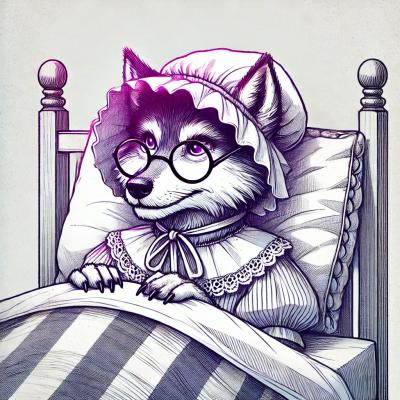
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
@cypress/webpack-preprocessor
Advanced tools
The @cypress/webpack-preprocessor package is a plugin for Cypress that allows you to use webpack to preprocess your JavaScript files before they are loaded into Cypress. This enables you to use webpack features such as ES6 syntax, importing CSS or images, and bundling your test files.
Preprocessing test files with webpack
This feature allows you to preprocess your test files using webpack. You can specify your webpack configuration and watch options. This is useful for using ES6/ESNext features, importing CSS, images, or other assets in your test files.
const webpackPreprocessor = require('@cypress/webpack-preprocessor');
module.exports = (on) => {
const options = {
webpackOptions: require('./webpack.config'),
watchOptions: {}
};
on('file:preprocessor', webpackPreprocessor(options));
};
Customizing webpack configuration
This feature allows you to customize the webpack configuration used for preprocessing. In this example, TypeScript files are being handled by adding a rule for `.ts` files and using the `ts-loader`.
const webpackPreprocessor = require('@cypress/webpack-preprocessor');
const webpackOptions = {
resolve: {
extensions: ['.ts', '.js']
},
module: {
rules: [
{
test: /\.ts$/,
exclude: /node_modules/,
use: [{ loader: 'ts-loader' }]
}
]
}
};
const options = {
webpackOptions,
watchOptions: {}
};
module.exports = (on) => {
on('file:preprocessor', webpackPreprocessor(options));
};
While not a Cypress plugin, karma-webpack offers similar functionality for the Karma test runner. It preprocesses and bundles test files using webpack, similar to what @cypress/webpack-preprocessor does for Cypress.
Cypress preprocessor for bundling JavaScript via webpack
npm install --save-dev @cypress/webpack-preprocessor
This package relies on the following peer dependencies:
It is likely you already have these installed either directly or as a transient dependency, but if not, you will need to install them.
npm install --save-dev @babel/core @babel/preset-env babel-loader webpack
This version is only compatible with webpack 4.x+ and Babel 7.x+.
@cypress/webpack-preprocessor
1.x@cypress/webpack-preprocessor
<= 2.xThis plugin (and all Cypress plugins) run in Cypress's own version of Node. If you require npm packages or your own code into the pluginsFile, they needs to be compatible with the version of Node that Cypress uses.
In your project's plugins file:
const webpack = require('@cypress/webpack-preprocessor')
module.exports = (on) => {
on('file:preprocessor', webpack())
}
Pass in options as the second argument to webpack
:
const webpack = require('@cypress/webpack-preprocessor')
module.exports = (on) => {
const options = {
// send in the options from your webpack.config.js, so it works the same
// as your app's code
webpackOptions: require('../../webpack.config'),
watchOptions: {},
}
on('file:preprocessor', webpack(options))
}
Object of webpack options. Just require
in the options from your webpack.config.js
to use the same options as your app.
Default:
{
module: {
rules: [
{
test: /\.jsx?$/,
exclude: [/node_modules/],
use: [{
loader: 'babel-loader',
options: {
presets: ['@babel/preset-env'],
},
}],
},
],
},
}
Source maps are always enabled unless explicitly disabled by specifying devtool: false
.
Object of options for watching. See webpack's docs.
Default: {}
An array of file path strings for additional entries to be included in the bundle.
By necessity, this preprocessor sets the entry point for webpack as the spec file or support file. The additionalEntries
option allows you to specify more entry points in order to utilize webpack's multi-main entry. This allows runtime dependency resolution.
Default: []
Example:
const webpack = require('@cypress/webpack-preprocessor')
module.exports = (on) => {
const options = {
webpackOptions: require('../../webpack.config'),
additionalEntries: ['./app/some-module.js'],
}
on('file:preprocessor', webpack(options))
}
The default options are provided as webpack.defaultOptions
so they can be more easily modified.
If, for example, you want to update the options for the babel-loader
to add the stage-3 preset, you could do the following:
const webpack = require('@cypress/webpack-preprocessor')
module.exports = (on) => {
const options = webpack.defaultOptions
options.webpackOptions.module.rules[0].use[0].options.presets.push('babel-preset-stage-3')
on('file:preprocessor', webpack(options))
}
Use the version of Node that matches Cypress.
Run all tests once:
npm test
Run tests in watch mode:
npm run test-watch
This project is licensed under the terms of the MIT license.
FAQs
Cypress preprocessor for bundling JavaScript via webpack
The npm package @cypress/webpack-preprocessor receives a total of 430,454 weekly downloads. As such, @cypress/webpack-preprocessor popularity was classified as popular.
We found that @cypress/webpack-preprocessor demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.