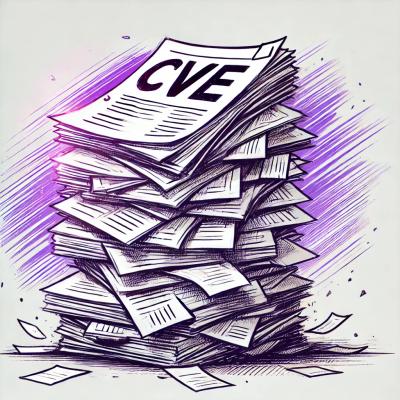
Security News
Node.js EOL Versions CVE Dubbed the "Worst CVE of the Year" by Security Experts
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
@danielsan/node-lazy-loader
Advanced tools
A Package for NPM Packages! Avoid unnecessary IO and save memory by allowing your users to load only the code they want
If you maintain a node package that has multiple functionalities that are not necessarily interdependent you are probably exporting the functionalities on your main js file like this:
module.exports = {
feature1: require('./lib/feature1'),
feature2: require('./lib/feature2'),
feature3: require('./lib/feature3')
...
featureN: require('./lib/featureN')
}
Some developers might install your node packages just because of a single one of those features, and most of them are loading your features like this:
const { feature1 } = require('your-package')
...
That forces their application to waste: processing time by performing the IO operations to load files from 1...N processing time by parsing those files and their respective require calls memory allocation since require will cache all loaded files
Some developers can inspect your code and conclude that they can load the expected dependency like this:
const feature1 = require('your-package/lib/feature1')
...
That works, but not just it is a minority of the users that do that, but they can run into problems if for some reason you decide to change a file name. For example:
module.exports = {
feature1: require('./lib/feature1-optimized'),
...
featureN: require('./lib/featureN')
}
const lazy = require('@danielsan/node-lazy-loader')(module)
module.exports = {
get feature1 () { lazy(this, 'feature1', './lib/feature1') },
get feature2 () { lazy(this, 'feature2', './lib/feature2') },
get feature3 () { lazy(this, 'feature3', './lib/x', 'someExportedPropertyFromX') },
...
get featureN () { lazy(this, 'featureN', './lib/featureN') }
}
By using getters the request will only happen when the property is read and this very package will rewrite the getter into a property with the expected value avoiding a function call the next time it is read
For example:
// your-module index.js
const lazy = require('@danielsan/node-lazy-loader')(module)
module.exports = {
get feature1 () {
console.log('reading feature1')
lazy(this, 'feature1', './lib/feature1')
}
}
// app index.js
const yourMod = require('your-module')
// the following line when executed will print 'reading feature1' then return the value
const a = yourMod.feature1
// the following line will only return the value since it is not longer a getter but a property
const b = yourMod.feature1
If you are not comfortable with passing module
to a 3rd-party function you can use it as follows:
const lazyCall = require('@danielsan/node-lazy-loader')({ require: module.require })
FAQs
A Package for NPM Packages! Avoid unnecessary IO and save memory by allowing your users to load only the code they want
We found that @danielsan/node-lazy-loader demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
Security News
cURL and Go security teams are publicly rejecting CVSS as flawed for assessing vulnerabilities and are calling for more accurate, context-aware approaches.
Security News
Bun 1.2 enhances its JavaScript runtime with 90% Node.js compatibility, built-in S3 and Postgres support, HTML Imports, and faster, cloud-first performance.