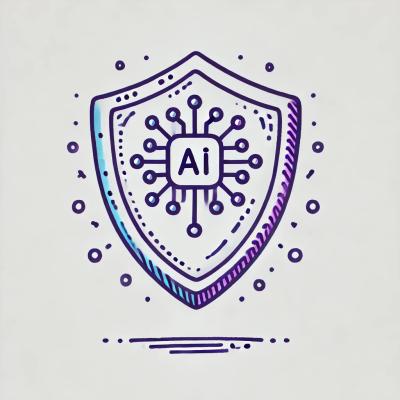
Security News
38% of CISOs Fear They’re Not Moving Fast Enough on AI
CISOs are racing to adopt AI for cybersecurity, but hurdles in budgets and governance may leave some falling behind in the fight against cyber threats.
@debitoor/cipher
Advanced tools
Encrypt/decrypt objects using aes-256-cbc algorithm
npm i -SE @debitoor/cipher
const cipher = require('@debitoor/cipher')('secret');
try {
const encrypted = cipher.encrypt({userId: '123456'});
console.log(encrypted); // { iv: 'eb0911c423161f0488337e5007887581', data: 'fd9612df14729ec373214f151b62fab74f8d7c5756082e4d057632dc5ea8d088' }
const decrypted = cipher.decrypt(encrypted);
console.log(decrypted); // { userId: '123456' }
} catch (e) {
// Handle error during encrypting/decrypting
}
{iv, data}
where iv - initialization vector, data - encrypted object. Throws error if json is invalid.{iv, data}
as argument where iv - initialization vector¹, data - encrypted object. Throws error if wrong secret key or incorrect data provided.¹ (https://nodejs.org/api/crypto.html#crypto_crypto_createcipheriv_algorithm_key_iv_options): Initialization vectors should be unpredictable and unique; ideally, they will be cryptographically random. They do not have to be secret: IVs are typically just added to ciphertext messages unencrypted. It may sound contradictory that something has to be unpredictable and unique, but does not have to be secret; it is important to remember that an attacker must not be able to predict ahead of time what a given IV will be.
FAQs
Encrypt/decrypt data
The npm package @debitoor/cipher receives a total of 18 weekly downloads. As such, @debitoor/cipher popularity was classified as not popular.
We found that @debitoor/cipher demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 41 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CISOs are racing to adopt AI for cybersecurity, but hurdles in budgets and governance may leave some falling behind in the fight against cyber threats.
Research
Security News
Socket researchers uncovered a backdoored typosquat of BoltDB in the Go ecosystem, exploiting Go Module Proxy caching to persist undetected for years.
Security News
Company News
Socket is joining TC54 to help develop standards for software supply chain security, contributing to the evolution of SBOMs, CycloneDX, and Package URL specifications.