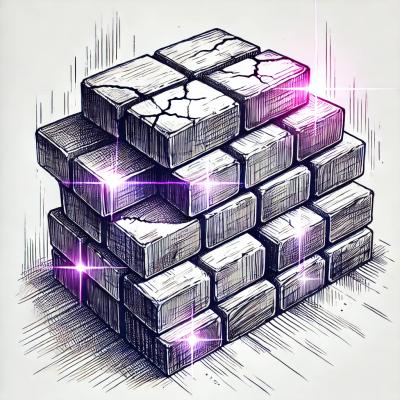
Security News
Input Validation Vulnerabilities Dominate MITRE's 2024 CWE Top 25 List
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
@devseed-ui/theme-provider
Advanced tools
import {
// Theme
DevseedUiThemeProvider,
GlobalStyles,
createUITheme,
theme,
themeVal,
createColorPalette,
// Stylizing function
stylizeFunction,
// Conversions and math
unitify,
rem,
px,
val2px,
val2rem,
multiply,
divide,
add,
subtract,
min,
max,
// Global spacing and RGBA
glsp,
rgba,
// Media queries
media,
// Style helpers
antialiased,
visuallyHidden,
listReset,
truncated,
visuallyDisabled,
disabled,
unscrollableY,
unscrollableX
} from '@devseed-ui/theme-provider';
See the API reference for a description of each export.
The components of the devseed-ui library require settings to be defined through a theme using specific variables.
The theme-provider
contains the base provider with the ui-library's default theme. This can be used directly, or overridden by the user.
import { DevseedUiThemeProvider } from '@devseed-ui/theme-provider';
The DevseedUiThemeProvider
should wrap the whole application so that all components can get the correct variables from the theme.
This will apply the default ui-library theme and some global styles to normalize presentation across browsers.
...
render (
<DevseedUiThemeProvider>
{components...}
<DevseedUiThemeProvider
)
It is also possible to provide a custom theme to DevseedUiThemeProvider
using the theme
prop.
It is important that the custom theme contains a value for all variables in the theme.
const myCustomTheme = {
...
};
...
render (
<DevseedUiThemeProvider theme={myCustomTheme}>
{components...}
<DevseedUiThemeProvider
)
The best way to provide a new theme is to use the createUITheme
helper and override the base theme variables, while also being able to add new variables.
This helper will ensure that defaults are set when no custom values are provided. Check (./src/theme.d.ts)[theme.d.ts] for a list of all theme properties.
const myCustomTheme = createUITheme({
color: {
base: '#F00',
// This is a custom color.
infographicColor: '#FF0'
}
});
...
render (
<DevseedUiThemeProvider theme={myCustomTheme}>
{components...}
<DevseedUiThemeProvider
)
Check theme.d.ts for the default ui-library theme values.
Utilities directly related with the theme.
DevseedUiThemeProvider
[React Component]
theme
prop. See How theming works with the ui-library.GlobalStyles
[React Component]
DevseedUiThemeProvider
, so this is not used often.createUITheme(definition)
[function]
theme
[object]
themeVal(path)
[function]
const Msg = styled.p`
color: ${themeVal('color.primary')};
`;
createColorPalette(name, baseColor)
[function]
stylizeFunction(function)
[function]
tint
function provided by Polished has the following signature: tint(percentage: (number | string), color: string): string
const Msg = styled.p`
color: ${({ theme }) => tint('50%', theme.color.primary)};
`;
themeVal
const _tint = stylizeFunction(tint)
const Msg = styled.p`
color: ${tint('50%', themeVal('color.primary'))};
`;
Utilities to be used with styled-components to do conversions and math operations.
All the functions can be used directly with styled-components and themeVal
, for example:
const Msg = styled.p`
padding: ${multiply(themeVal('layout.border'), 3)}; // 3px
`;
unitify(unit)
[function]
unit
to the value. const percent = unitify('%');
percent(10) // -> 10%
rem(value)
[function]
rem
to the give value.px(value)
[function]
rem
to the give value.val2px(value)
[function]
theme.type.base.root
).val2rem(value)
[function]
theme.type.base.root
).multiply
[function]
2rem * 2 = 4rem | 2 * 2rem = 4
divide
[function]
2rem / 2 = 1rem | 2 / 2rem = 1
add
[function]
2rem + 2 = 4rem | 2 + 2rem = 4
subtract
[function]
4rem - 2 = 2rem | 4 - 2rem = 2
min
[function]
10px, 15 = 10 | 4rem, 5px = 4
max
[function]
10px, 15 = 15 | 4rem, 5px = 5
glsp(...args)
[function]
layout.space
. This allows all the components to gracefully scale based on a single setting. padding: ${glsp(2, 1 / 2)}; // 2rem 0.5rem
padding: ${glsp(2, 0.5)}; // 2rem 0.5rem
padding: ${glsp()}; // 1rem
1
.rgba(color, value)
[function]
rgba
exported by the polished
module, but modified to work with styled-components. See Stylizing function. const Msg = styled.p`
color: ${rgba(themeVal('color.primary'), 0.5)};
`;
The media queries will be available through the media
object as Up
, Only
, and Down
variations of each range defined on the theme.
For example, with the range (medium: [768, 991]
):
mediumUp
will be triggered from 768px;mediumOnly
will stay active between 768px and 991px;mediumDown
while the viewport is below or at 991px.All available options are:
media.xsmallOnly
media.xsmallDown
media.smallUp
media.smallOnly
media.smallDown
media.mediumUp
media.mediumOnly
media.mediumDown
media.largeUp
media.largeOnly
media.largeDown
media.xlargeUp
media.xlargeOnly
These can be used directly on styled-components using template literals:
const Msg = styled.p`
color: red;
${media.mediumUp`
color: blue;
`}
${media.largeUp`
color: green;
`}
`;
The helpers are to be used within a styled-component and return useful snippets of code.
antialiased
[function]
visuallyHidden
[function]
listReset
[function]
ul
and ol
) styling. Say goodbye to default padding, margin, and bullets/numbers.truncated
[function]
disabled
[function]
visuallyDisabled
[function]
disabled
, but the pointer events remain active. This is useful when, for example, paired with a tooltip that needs the hover
event to fire.unscrollableY
[function]
unscrollableX
[function]
Use directly in a styled-component:
const Msg = styled.p`
${antialiased()}
`;
FAQs
devseed UI Kit Theme
The npm package @devseed-ui/theme-provider receives a total of 121 weekly downloads. As such, @devseed-ui/theme-provider popularity was classified as not popular.
We found that @devseed-ui/theme-provider demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.