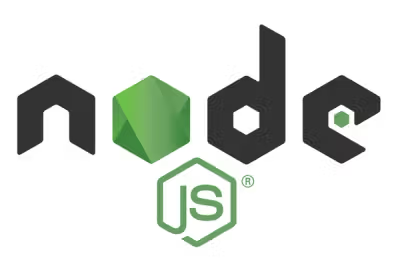
Security News
require(esm) Backported to Node.js 20, Paving the Way for ESM-Only Packages
require(esm) backported to Node.js 20, easing the transition to ESM-only packages and reducing complexity for developers as Node 18 nears end-of-life.
@diesdasdigital/js-canvas-library
Advanced tools
This is a JS library to create canvas based applications using the Elm Architecture.
This is a JS library to create canvas based applications using the Elm Architecture.
To install all dependencies run:
yarn add @diesdasdigital/js-canvas-library
Here is a basic example of rendering a rectangle using the library. Its color will change as soon as the user clicks the button.
An example highlighting subscriptions and fetching data can be found in example.js
.
import { canvas } from "@diesdasdigital/js-canvas-library";
function init(flags, meta) {
const model = {
color: "red",
viewport: meta.viewport,
};
const cmds = [];
return [model, cmds];
}
const msg = {
userClickedOnButton: () => ({
type: "userClickedOnButton",
}),
};
function update(_msg, model) {
switch (_msg.type) {
case "userClickedOnButton":
return [{ ...model, color: "green" }, [scheduleCustomSideEffect()]];
default:
return [model, []];
}
}
function view(context, model) {
const SIZE = 20;
const HALF = 0.5;
context.fillStyle = model.color;
context.fillRect(
model.viewport.width * HALF - SIZE * HALF,
model.viewport.height * HALF - SIZE * HALF,
SIZE,
SIZE
);
}
function subscriptions() {
return [];
}
const send = canvas(
document.querySelector(".canvas"),
{} // <- flags
)({
init,
view,
update,
subscriptions,
});
document.querySelector(".button").addEventListener("click", () => {
send(msg.userClickedOnButton());
});
Commands can be returned as the second item in the array retured in each update case, eg
return [model, [command1, command2…]]
request
The request
command is a minimal wrapper of the browser’s fetch
function. The first two
arguments are the same as in fetch: url and request options. The additional third and fourth
arguments are message which are dispatched when the request succeeds or fails. request
will
throw an error if not all 4 arguments are passed to make sure success and error case are handled.
import { request } from "@diesdasdigital/js-canvas-library";
request(
"https://example.com/",
options,
successMessage,
errorMessage
),
In case one needs to send a custom message inside update
it is possible to do so by calling the
message function like a command:
return [model, [msg.doSomething]];
This is handy in case message should be dispatched after state has been updated or based on a state change without a user interaction.
It can also be used to handle side effects, for example to send a message which then is handled in update
and only performs a side effect without changing the model. Please talk to Max, if you need more than the request
side effect. There is a good chance it can be added to the library.
Built-in subscriptions for some events can be imported from the library.
import { events } from "@diesdasdigital/js-canvas-library";
onMouseMove
The message passed to onMouseMove
will receive an object { x, y }
with the
mouse coordinates relative to the canvas element. x = 0; y = 0
is equivalent to
having the mouse on the top left corner of the canvas. The function receiving this
object in the example below is msg.updateMouse
.
function subscriptions() {
return [events.onMouseMove(msg.updateMouse)];
}
onAnimationFrame
The message passed to onAnimationFrame
will receive the timestamp of the animation
frame as an argument. (in the example code below that function is msg.tick
)
function subscriptions() {
return [events.onAnimationFrame(msg.tick)];
}
Note: all of the following commands should be run in the project’s folder.
Clone the repository and run:
yarn
To open the example page while development you can run:
yarn dev
Linting should run automatically when pushing. To lint your code manually run:
yarn lint
Allowed to be used by people at diesdas and its creator.
Login as diesdas using the credentials provided in 1Password and run:
yarn publish . --access=public
FAQs
This is a JS library to create canvas based applications using the Elm Architecture.
The npm package @diesdasdigital/js-canvas-library receives a total of 4 weekly downloads. As such, @diesdasdigital/js-canvas-library popularity was classified as not popular.
We found that @diesdasdigital/js-canvas-library demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
require(esm) backported to Node.js 20, easing the transition to ESM-only packages and reducing complexity for developers as Node 18 nears end-of-life.
Security News
PyPI now supports iOS and Android wheels, making it easier for Python developers to distribute mobile packages.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.