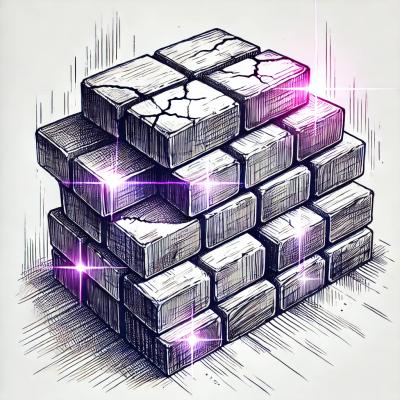
Security News
Input Validation Vulnerabilities Dominate MITRE's 2024 CWE Top 25 List
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
@discordjs/collection
Advanced tools
@discordjs/collection is a powerful utility for managing collections of data. It extends JavaScript's native Map class and provides additional methods that are useful for handling and manipulating collections, especially in the context of Discord bots.
Basic Collection Operations
This feature allows you to perform basic operations such as setting, getting, and checking the existence of elements in the collection.
const { Collection } = require('@discordjs/collection');
const collection = new Collection();
collection.set('key1', 'value1');
collection.set('key2', 'value2');
console.log(collection.get('key1')); // Output: value1
console.log(collection.has('key2')); // Output: true
console.log(collection.size); // Output: 2
Filtering and Mapping
This feature allows you to filter and map the elements in the collection, providing a way to transform and query the data.
const { Collection } = require('@discordjs/collection');
const collection = new Collection();
collection.set('key1', 10);
collection.set('key2', 20);
collection.set('key3', 30);
const filtered = collection.filter(value => value > 15);
console.log(filtered); // Output: Collection { 'key2' => 20, 'key3' => 30 }
const mapped = collection.map(value => value * 2);
console.log(mapped); // Output: [ 20, 40, 60 ]
Reducing and Finding
This feature allows you to reduce the collection to a single value and find specific elements based on a condition.
const { Collection } = require('@discordjs/collection');
const collection = new Collection();
collection.set('key1', 1);
collection.set('key2', 2);
collection.set('key3', 3);
const sum = collection.reduce((acc, value) => acc + value, 0);
console.log(sum); // Output: 6
const found = collection.find(value => value === 2);
console.log(found); // Output: 2
Lodash is a popular utility library that provides a wide range of functions for manipulating arrays, objects, and other data structures. It offers similar functionalities to @discordjs/collection, such as filtering, mapping, and reducing collections, but it is more general-purpose and not specifically tailored for Discord bots.
Immutable.js provides immutable data structures, which can be useful for managing collections of data in a way that prevents accidental mutations. While it offers similar functionalities like filtering and mapping, it focuses on immutability, which is a different approach compared to @discordjs/collection's mutable collections.
Collect.js is a library that provides a fluent and convenient way to work with arrays and objects in JavaScript. It offers chainable methods for manipulating collections, similar to @discordjs/collection, but it is designed to be more general-purpose and not specifically for Discord bots.
Utility data structure used in Discord.js.
FAQs
Utility data structure used in discord.js
The npm package @discordjs/collection receives a total of 335,694 weekly downloads. As such, @discordjs/collection popularity was classified as popular.
We found that @discordjs/collection demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.