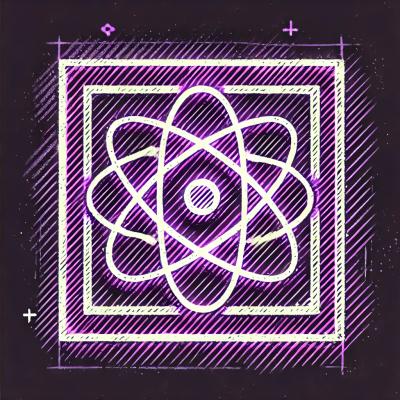
Security News
Create React App Officially Deprecated Amid React 19 Compatibility Issues
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
@drivekyte/request
Advanced tools
A helper library used in Kyte frontend packages to perform HTTP requests. Written in TypeScript, uses axios in the background.
Run
yarn add @drivekyte/request
In the root of your project, import configureRequest
and execute it to provide configuration to subsequent requests performed using the library:
import { configureRequest } from '@drivekyte/request';
configureRequest({ baseURL: API_BASE_URL, timeout: REQUEST_TIMEOUT });
Perform an actual HTTP request anywhere in the code:
import request from '@drivekyte/request';
const response = await request({ method: 'GET', url: 'example' });
Behind the scenes, request
passes the arguments as is to axios. See more about possible options on https://github.com/axios/axios.
It's possible to perform HTTP requests directly in React components or create custom React hooks using the useRequest
hook provided by the library:
import { useRequest } from '@drivekyte/request';
type DataType = { id: number; value: string }[];
type MetaType = { example: string };
const useRequestExample = () => {
return useRequest<DataType, MetaType>({
method: 'GET',
url: '/api/users/me',
});
};
export default useRequestExample;
The useRequest
function can also accept a second argument, an object that defines request options:
{
// prop to indicate if we should avoid to persist data
// default: false
avoidPersistData: boolean,
// prop to indicate an initial state, avoiding therefore an initial request
// default: undefined
initialData: any,
// prop to indicate if the request should start straight away
// default: true
requestIf: boolean,
// get data directly from the json response instead from data
// default: undefined
retrieveDataFromResponse: boolean
}
We export a serverRequest
function to be used in the server side. It will use the same configuration that you used when configuring the request.
export async function getServerSideProps() {
const {
data: quotes,
errorMessage,
response,
statusCode,
} = await serverRequest<Quotes>({
method: 'GET',
url: 'v2/rentals/quotes',
params: {
end_address: 'San Francisco',
end_date: 1617264000000,
has_damage_coverage: false,
has_liability_coverage: false,
start_address: 'San Francisco',
start_date: 1614589200000,
},
});
return {
props: {
errorMessage,
data: {
quotes,
},
},
};
}
Use createMock
and mockResponse
import request, { createMock, mockResponse } from '@drivekyte/request';
import { render } from '@testing-library/react';
const mockAdapter = createMock(request);
const exampleMock = mockResponse({ method: 'GET', url: /example/ });
describe('<Example/>', () => {
afterEach(() => {
mockAdapter.reset();
});
it('should render with text "example"', async () => {
exampleMock.reply(200, { data: [{ id: 1, text: 'example' }] });
const { findByText } = render(<Example />);
expect(await findByText('example')).toBeInTheDocument();
});
});
The axios-mock-adapter
with a modified API is used to mock HTTP requests. For more info, check https://github.com/ctimmerm/axios-mock-adapter.
Contributions to this project are welcome. After cloning the repo, use the following instructions:
yarn install
yarn dev
yarn test:watch
We've a CI/CD for publishing automatically to NPM once a branch is merged into master. The step to publish to NPM should be manually triggered. Make sure to bump the package version before.
FAQs
Utilities for doing HTTP requests
The npm package @drivekyte/request receives a total of 2 weekly downloads. As such, @drivekyte/request popularity was classified as not popular.
We found that @drivekyte/request demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
Security News
Oracle seeks to dismiss fraud claims in the JavaScript trademark dispute, delaying the case and avoiding questions about its right to the name.
Security News
The Linux Foundation is warning open source developers that compliance with global sanctions is mandatory, highlighting legal risks and restrictions on contributions.