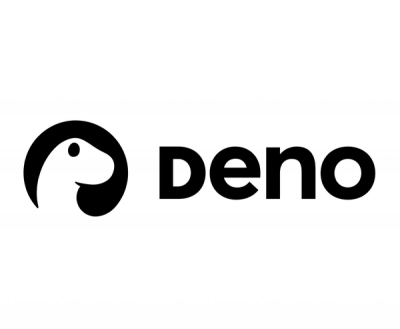
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
@edirect/frontend-foundations
Advanced tools
On this documentation you will find the following topics:
The foundations are a set of components provided for developers to easily replicate the base used by the Designers to place, align, style and structure your pages.
It contains a strict series of components that should always be used in your development, as it follows the Bolttech Guidelines for designing pages.
Install the frontend-foundations
package on your project, with the following command:
npm i @edirect/frontend-foundations@version
For development environments:
git clone git@bitbucket.org:gofrank/frontend-foundations.git
cd frontend-foundations
npm install
npm run storybook
Before you start, you should be aware of two points that are the base of this project. Those points were defined in conjunction with the Design Team to create a basic structure that should be followed everytime designing and developing new components and pages. Those points are:
All of the components inside the foundations follow our breakpoint rules. Those rules are also used later for you to provide specific sizes for some components depending on which screen breakpoint the screen currently is. Those breakpoints are:
Breakpoint | Screen Size |
---|---|
XS | <= 413px |
SM | 414px - 767px |
MD | 768px - 1439px |
LG | >= 1440px |
With that said, you should import our media-queries
based on those breakpoints when developing pages so you can better follow the grid guidelines.
import styled from 'styled-components';
import { MediaQueries } from '@edirect/frontend-foundations';
export const MyComponent = styled.div`
display: flex;
flex-direction: row;
background-color: red;
@media ${MediaQueries.LG} {
background-color: green;
}
@media ${MediaQueries.SM} {
flex-direction:column
}
`
The foundations comes with a preset of colors, paddings, spacings, typography and tokens, these presets are the default theme
or bolttech theme
.
Here is the structure of theme:
type bolttechTheme = {
colors: ColorsType;
spacing: SpacingType;
typography: TypographyType;
effects: EffectType;
components: Record<string, unknown>;
tokens?: Record<string, unknown>;
};
The BolttechThemeProvider
component is a extension of the ThemeProvider
from styled-components. It allows you to inject a theme into all styled-components bellow it in the component tree.
Our ThemeProvider
differs from the styled-components one because we expect a theme with a defined structure, and also, have a default theme with Bolttech styles.
If you want, you can import our default theme and the theme type and use it as you wish.
import { BolttechThemeProvider, bolttechTheme } from '@edirect/frontend-foundations';
const MyApp = () => (
<BolttechThemeProvider theme={bolttechTheme}>
<App />
</BolttechThemeProvider>
);
export default MyApp;
Important: If you plan to use any of the components bellow, you need to wrap your application with the BolttechThemeProvider
as our components use design tokens with a pre-defined structure, so you need to provide a theme with the type BolttechThemeType
.
The Row
component is basicly a div
that wraps the components as a flex container, applying the base styles of our Design Grid System.
It has a maximum width of 1440px, a gap between columns and paddings on both sides of the row. The value of the gap and paddings are based on the Breakpoints of our grid system.
Breakpoint | Gap | Padding |
---|---|---|
XS | flexbox.XS | flexbox.S |
SM | flexbox.S | flexbox.L |
MD | flexbox.M | flexbox['2XL'] |
LG | flexbox.M | flexbox['3XL'] |
Prop | Description | Type | Required | Default |
---|---|---|---|---|
fullWidth | remove row paddings | boolean | false | false |
fullHeight | add 100% height | boolean | false | false |
center | add margin 0 auto | boolean | false | false |
className | extra css classes | string | false | undefined |
children | children component | ReactElement or ReactElement[] | false | undefined |
import { Row } from '@edirect/frontend-foundations';
<Row fullWidth={true} fullHeight={true} center={false} className="mb-xs">
<ChildComponent />
</Row>;
The Column
component is a component which uses percentage of width to define the size of the container. Designers and devs need to be aware that each screen breakpoint have a size to be filled. The possibilities of each breakpoint will be available below:
Breakpoint | Allowed Sizes |
---|---|
XS | 100%, 75%, 66.666%, 50%, 33.333%, 25%, 0% |
SM | 100%, 75%, 66.666%, 50%, 33.333%, 25%, 20%, 16.666%, 0% |
MD and LG | 100%, 75%, 66.666%, 50%, 33.333%, 25%, 20%, 16.666%, 0% |
Prop | Description | Type | Required | Default |
---|---|---|---|---|
children | children component | ReactElement or ReactElement[] | false | undefined |
className | string containing a list of classes that need to be applied into the Column | string | false | undefined |
size | object containing the size of the column for each breakpoint | Object | true |
import { Column, Row } from '@edirect/frontend-foundations';
const MyComponent = () => (
<Row>
<Column size={{ xs: '100%', sm: '33.333%', md: '50%', ld: '50%' }}>Hello</Column>
<Column size={{ xs: '100%', sm: '66.666%', md: '50%', lg: '50%' }}>World</Column>
</Row>
);
The Center
component is a basic component that wraps it's children component and centers it.
import { Center, Column, Row } from '@edirect/frontend-foundations';
const MyComponent = () => (
<Row>
<Center>
<Column size={{ xs: '50%', sm: '50%', md: '50%', lg: '50%' }}>World</Column>
</Center>
</Row>
);
The Typography
is a text component that can assume a lot of sizes determined by the design team based on the tokens inside the typography file under the theme folder. Please provide a valid combination of variant
and type
based on this file.
Prop | Description | Type | Required | Default |
---|---|---|---|---|
type | type of the typography | string | true | body |
variant | style variant | string | true | primary |
text | if you need to provide your text as prop | boolean | false | undefined |
className | extra css classes | string | false | undefined |
children | children component | ReactElement or ReactElement[] | false | undefined |
Note: Depending on what type
and variant
you provide, the Typography
component will render a different HTML Element.
Type | Variant | Element |
---|---|---|
headings | h1 , h1Light | h1 |
headings | h2 | h2 |
headings | h3 | h3 |
headings | h4 | h4 |
body | primary , secondary , tertiary | p |
label | primary , secondary , tertiary | label |
import { Typography } from '@edirect/frontend-foundations';
const MyComponent = () => (
<Typography variant="primary" type="body">
Welcome to bolttech!
</Typography>
);
To help the development and styling of your pages, we decided to add helper classes for colors, paddings and margins as we know that creating styled components just to add those properties is not a fun task.
All of those classes are created based on the colors, spacing and flexbox properties of the tokens provided by your theme configuration
With that said, we have a basic set of classes that we provide:
Based on the structure of the theme, we create classes with the prefixes of text-
, bg-
and fill-
and append the colors provided by your theme. So for example, if you have a theme with the following colors:
colors: {
content: {
accent: tokens.cyan['700'],
base: tokens.navy['700'],
subtle: tokens.navy['500'],
weak: tokens.navy['400'],
// ...
}
}
We will inject the following classes on your global styles:
.text-content-accent {
color: #00bac7;
}
.bg-content-accent {
background-color: #00bac7;
}
.fill-content-accent {
fill: #00bac7;
}
.text-content-base {
color: #170f4f;
}
.bg-content-base {
background-color: #170f4f;
}
.fill-content-base {
fill: #170f4f;
}
/* ... */
Based on the structure of the theme, we create classes with the prefixes of mt-
, mb-
, ml-
, mr-
,mh-
,mv-
, and m-
, and append the margins provided by your theme. So for example, if you have a theme with the following margins:
spacing: {
flexbox: {
XXS: '8px',
XS: '16px',
S: '24px',
M: '32px',
// ...
},
}
We will inject the following classes on your global styles:
.mt-xxs: {
margin-top: 8px;
}
.mb-xxs {
margin-bottom: 8px;
}
.ml-xxs {
margin-left: 8px;
}
.mr-xxs {
margin-right: 8px;
}
.mh-xxs {
margin-left: 8px;
margin-right: 8px;
}
.mv-xxs {
margin-top: 8px;
margin-bottom: 8px;
}
.m-xxs {
margin: 8px;
}
Based on the structure of the theme, we create classes with the prefixes of pt-
, pb-
, pl-
, pr-
,ph-
,pv-
, and p-
, and append the paddings provided by your theme. So for example, if you have a theme with the following paddings:
spacing: {
padding: {
NONE: '0px',
XXXS: '2px',
XXS: '3px',
XS: '4px',
S: '8px',
// ...
},
}
We will inject the following classes on your global styles:
.pt-xxs: {
padding-top: 8px;
}
.pb-xxs {
padding-bottom: 8px;
}
.pl-xxs {
padding-left: 8px;
}
.pr-xxs {
padding-right: 8px;
}
.ph-xxs {
padding-left: 8px;
padding-right: 8px;
}
.pv-xxs {
padding-top: 8px;
padding-bottom: 8px;
}
.p-xxs {
padding: 8px;
}
/* ... */
We also wanted to provide an easy way for people to position elements on the page without having to create custom styled components just for positioning. So we also inject those classes on your global styles:
.flex {
display: flex;
}
.flex-grow {
flex: 1;
}
.align-start {
align-items: flex-start;
}
.align-center {
align-items: center;
}
.align-right {
align-items: flex-end;
}
.justify-left {
justify-content: flex-start;
}
.justify-center {
justify-content: center;
}
.justify-right {
justify-content: flex-end;
}
.justify-between {
justify-content: space-between;
}
.justify-evenly {
justify-content: space-evenly;
}
.justify-arround {
justify-content: space-arround;
}
/* ... */
In some cases you will need to create a theme for a specific partner and/or flow. Based on that, we created a tool that can do this task for you.
Requirements: node 16 and npx
.
How to use: First, you need to install the @edirect/frontend-foundations
package globaly, you can do this by running the following command:
npm i -g @edirect/frontend-foundations
After installing the package, you can run this command with the following parameters to generate your theme:
npx @edirect/frontend-foundations generate-theme ${path-to-tokens-file} ${path-to-save-theme}
Example:
npx @edirect/frontend-foundations generate-theme /home/dev/Downloads/token.json ./src/style/themes/fwdgi
Note: the tokens file has a specific structure that it has to follow for this command to work, so be sure that the you are using a base file provided by the Design team.
Runing this command will generate the following structure (the files created by this command are the ones marked with the + symbol):
project
│---README.md
└───node_modules
└─── ...
└─── src
└─── ...
└─── style
└─── themes
└─── ...
└─── + fwdgi
└─── + theme
│--- + colors.ts
│--- + components.ts
│--- + effects.ts
│--- + index.ts
│--- + spacing.ts
│--- + typography.ts
└─── + tokens
│--- + color.ts
│--- + effect.ts
│--- + index.ts
│--- + spacing.ts
│--- + typography.ts
FAQs
Frontend Foundations
The npm package @edirect/frontend-foundations receives a total of 248 weekly downloads. As such, @edirect/frontend-foundations popularity was classified as not popular.
We found that @edirect/frontend-foundations demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 25 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.