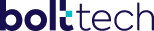
Foundations
The foundations of the design system

Features
- New components: Row, Column, Typography and Icons;
- Patterns with regards to spacing, colours and shadows;
- It is ready to be used in any frontend application that uses React.
- All of the components are styled-components based, so you can easily extend them and apply some customization.
Installation
Frontend Foundations requires Node.js v14+ to run.
Install into your project:
npm i @edirect/frontend-foundations
For development environments:
git clone git@bitbucket.org:gofrank/frontend-foundations.git
cd frontend-foundations
npm install
npm run storybook
Plugins
Frontend Foundations is currently extended with the following plugins.
Instructions on how to use them in your own application are linked below.
Screen breakpoints:
These foundations have some screen breakpoints and they are categorized by the min and max screen size of the user agent.
Breakpoint | Screen Size |
---|
XS | <= 412px |
SM | 412px - 1430px |
MD | 1440px - 1559px |
LG | >= 1600px |
How to use the components:
First of all you need to wrap your frontend application with bolttech theme provider. This theme provider receives a theme
the schema that can be imported to be used by default or be extended to be used by other partners and/or flows.
Example:
import { BolttechThemeProvider, bolttechTheme } from '@edirect/frontend-foundations';
const MyApp = () => (
<BolttechThemeProvider theme={bolttechTheme}>
<App />
</BolttechThemeProvider>
);
export default MyApp;
After wrapping your app with the bolttech theme provider, you will be able to use all of these components below.
- Row
The Row component has some left and right margin-based in the size of the screen. Using the breakpoints we have these left/right margins:
Breakpoint | Margin |
---|
XS | spacingXS (16px) |
SM | spacingS (24px) |
MD or LG | spacingM (32px) |
If you want to remove these margins, you just need to set true to the prop fullWidth. Example:
import { Row } from '@edirect/frontend-foundations';
<Row fullWidth={true}><ChildComponent /></Row>
- Column
Column component is a component who uses FlexBox to define the size of the container. Designers and devs need to be aware that each screen breakpoint have a size to be filled. The possibilities of each breakpoint will be available below:
Breakpoint | Margin |
---|
XS | 100%, 50%, 33% and 25% |
SM | 100%, 50%, 33%, 25%, 20% and 16% |
MD and LG | 100%, 50%, 33%, 25%, 20%, 16% and 8,3% |
Note: Devs, please respect these sizes above to maintain consistency. | |
Props
Prop | Type | Description | Example |
---|
children | ReactElement or ReactElement[] | - | - |
className | String | String containing a list of classes that need to be applied into the Column. | "bg-secondary-base" |
size | Object | Object containing the size of the column for each breakpoint. | { xs: '25%', sm: '50%', md: '100%', lg: '50%' } |
Example:
import { Column, Row } from '@edirect/frontend-foundations';
const MyComponent = () => (
<Row>
<Column size={{ xs: '50%', sm: '50%', md: '50%', ld: '50%' }}>Hello</Column>
<Column className="bg-primary-base" size={{ xs: '50%', sm: '50%', md: '50%', ld: '50%' }}>World</Column>
</Row>
);
Typography
Typography is a text component that can assume a lot of sizes determined by the design team. Is easy to use and has a lot of props that can have the best fit for your component.
Props
Prop | Type | Description | Example |
---|
children | ReactElement, ReactElement[], string[], string, number[], number, boolean | - | - |
className | String | String containing a list of classes that need to be applied into the Typography. | "text-secondary-base" |
element | String | Sets the HTML element to be used on this Typography component. | h1, span, p and etc... |
type | String | Sets the font size, weight, and height based on the foundations to be used on this Typography component. | displayL, bodyM, labelS and etc... |
align | String | Sets the alignment of the text. | left, center or right |
Example:
import { Typography } from '@edirect/frontend-foundations';
const MyComponent = () => (
<Typography element="h5" type="displayBoldS" align="center">Welcome to bolttech!</Typography>
);
Icon
Using the same approach of the Column component, Icon has the size prop that uses the breakpoints of the foundations.
Prop | Type | Description | Example |
---|
colorName | String | String containing a name of the color that will to be applied into the Icon. You need to follow the format "{colorCategory}-{colorName}" | "secondary-base" |
iconName | String | Sets the SVG file correlated with the icon name. Click here to access the icon list. | content/call |
size | Object | Object containing the size of the icon for each breakpoint. | { xs: '24px', sm: '32px', md: '32px', lg: '48px' } |
Example:
import { Icon } from '@edirect/frontend-foundations';
const MyComponent = () => (
<Icon
iconName="action/policy"
colorName="primary-base"
size={{ xs: '12px', sm: '24px', md: '32px', ld: '32px' }}
/>
);
Helper classes
To help the development, we decided to add helper classes to be added to text, backgrounds and fill SVGs. Each colour of the theme has 3 classes to change the colours of the background, text and SVGs.
Example:
Color | Text Class | Background Class | SVG Class |
---|
Cyan -- primary-base (#00BAC7) | text-primary-base | bg-primary-base | fill-primary-base |
Navy -- secondary-base (#170F4F) | text-secondary-base | bg-secondary-base | fill-secondary-base |
Yellow (600) -- accent-600 (#E9E133) | text-accent-600 | bg-accent-600 | fill-accent-600 |
Default Theme - bolttech
The foundations came with a preset of colors, paddings, spacings, typography and tokens, these presets are the default
theme or bolttech theme.
Here is the structure of theme:
type bolttechTheme = {
colors: Record<colors, Record<string, string>>;
paddingScale: Record<paddingScaleType, string>;
spacingScale: Record<spacingScaleType, string>;
typography: Record<typographyBreakPoints, TypographyProps>;
effects: EffectType;
tokens?: Record<string, unknown>;
}
In some cases you will need to write a theme for a specific partner and/or flow.
To do that you just need to override the theme object with the properties that you want.
Example: Override the base primary color.
import { bolttechTheme } from '@edirect/frontend-foundations';
const customTheme = {
...bolttechTheme,
colors: {
...bolttechTheme.colors,
primary: {
...bolttechTheme.colors.primary,
base: '#f3f3f3',
}
}
}