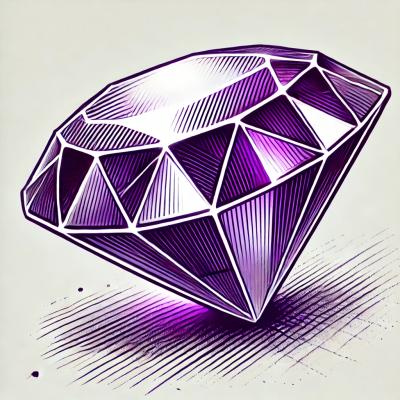
Security News
RubyGems.org Adds New Maintainer Role
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
@effect/data
Advanced tools
@effect/data is a functional programming library for JavaScript and TypeScript that provides a collection of data structures and utilities to work with immutable data, functional effects, and more. It aims to help developers write more predictable and maintainable code by leveraging functional programming principles.
Immutable Data Structures
Provides immutable data structures like List, Map, and Set. These structures help in maintaining immutability in your code, making it easier to reason about state changes.
const { List } = require('@effect/data');
const list = List.of(1, 2, 3);
const newList = list.append(4);
console.log(newList.toArray()); // [1, 2, 3, 4]
Functional Effects
Allows you to work with functional effects, which are computations that can produce side effects. This helps in managing side effects in a controlled manner.
const { Effect } = require('@effect/data');
const effect = Effect.of(() => console.log('Hello, World!'));
effect.run();
Option and Either
Provides Option and Either types for handling optional values and computations that can fail, respectively. These types help in avoiding null or undefined values and managing errors more effectively.
const { Option, Either } = require('@effect/data');
const someValue = Option.some(42);
const noneValue = Option.none();
const rightValue = Either.right('Success');
const leftValue = Either.left('Error');
console.log(someValue.isSome()); // true
console.log(noneValue.isNone()); // true
console.log(rightValue.isRight()); // true
console.log(leftValue.isLeft()); // true
Pattern Matching
Supports pattern matching, which allows you to match values against patterns and execute code based on the matched pattern. This can make your code more expressive and concise.
const { match } = require('@effect/data');
const value = 2;
const result = match(value)
.case(1, () => 'one')
.case(2, () => 'two')
.case(3, () => 'three')
.default(() => 'unknown');
console.log(result); // 'two'
The 'immutable' package provides persistent immutable data structures for JavaScript, such as List, Map, and Set. It is similar to @effect/data in terms of offering immutable data structures but does not include functional effects or pattern matching.
Folktale is a suite of libraries for generic functional programming in JavaScript. It includes data structures like Maybe and Either, similar to Option and Either in @effect/data. However, Folktale also provides utilities for asynchronous programming and algebraic data types.
Ramda is a practical functional library for JavaScript programmers. It focuses on providing utility functions for functional programming, such as currying, composition, and immutability. While it does not provide data structures like @effect/data, it complements it by offering a wide range of functional utilities.
To install the alpha version:
npm install @effect/data
Warning. This package is primarily published to receive early feedback and for contributors, during this development phase we cannot guarantee the stability of the APIs, consider each release to contain breaking changes.
strict
flag enabled in your tsconfig.json
file{
// ...
"compilerOptions": {
// ...
"strict": true,
}
}
The MIT License (MIT)
FAQs
Functional programming in TypeScript
We found that @effect/data demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.
Security News
Research
Socket's threat research team has detected five malicious npm packages targeting Roblox developers, deploying malware to steal credentials and personal data.