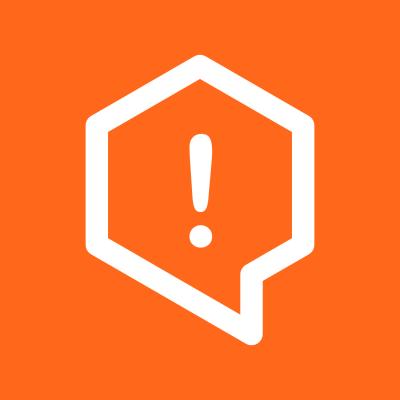
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
@ekreative/react-native-braintree
Advanced tools
A react native interface for integrating payments using Braintree
Add this to your build.gradle
allprojects {
repositories {
maven {
// Braintree 3D Secure
// https://developer.paypal.com/braintree/docs/guides/3d-secure/client-side/android/v4#generate-a-client-token
url "https://cardinalcommerceprod.jfrog.io/artifactory/android"
credentials {
username 'braintree_team_sdk'
password 'AKCp8jQcoDy2hxSWhDAUQKXLDPDx6NYRkqrgFLRc3qDrayg6rrCbJpsKKyMwaykVL8FWusJpp'
}
}
}
}
In Your AndroidManifest.xml
, android:allowBackup="false"
can be replaced android:allowBackup="true"
, it is responsible for app backup.
Also, add this intent-filter to your main activity in AndroidManifest.xml
<activity>
...
<intent-filter>
<action android:name="android.intent.action.VIEW" />
<category android:name="android.intent.category.DEFAULT" />
<category android:name="android.intent.category.BROWSABLE" />
<data android:scheme="${applicationId}.braintree" />
</intent-filter>
</activity>
NOTE: Card payments do not work on rooted devices and Android Emulators
If your project uses Progurad, add the following lines into proguard-rules.pro
file
-keep class com.cardinalcommerce.dependencies.internal.bouncycastle.**
-keep class com.cardinalcommerce.dependencies.internal.nimbusds.**
-keep class com.cardinalcommerce.shared.**
cd ios
pod install
Add a bundle url scheme {BUNDLE_IDENTIFIER}.payments in your app Info via XCode or manually in the Info.plist. In your Info.plist, you should have something like:
<key>CFBundleURLTypes</key>
<array>
<dict>
<key>CFBundleTypeRole</key>
<string>Editor</string>
<key>CFBundleURLName</key>
<string>com.myapp</string>
<key>CFBundleURLSchemes</key>
<array>
<string>com.myapp.payments</string>
</array>
</dict>
</array>
In your AppDelegate.m
:
#import "BraintreeCore.h"
...
- (BOOL)application:(UIApplication *)application didFinishLaunchingWithOptions:(NSDictionary *)launchOptions
{
...
[BTAppContextSwitcher setReturnURLScheme:self.paymentsURLScheme];
}
- (BOOL)application:(UIApplication *)application
openURL:(NSURL *)url
options:(NSDictionary<UIApplicationOpenURLOptionsKey,id> *)options {
if ([url.scheme localizedCaseInsensitiveCompare:self.paymentsURLScheme] == NSOrderedSame) {
return [BTAppContextSwitcher handleOpenURL:url];
}
return [RCTLinkingManager application:application openURL:url options:options];
}
- (NSString *)paymentsURLScheme {
NSString *bundleIdentifier = [[NSBundle mainBundle] bundleIdentifier];
return [NSString stringWithFormat:@"%@.%@", bundleIdentifier, @"payments"];
}
import RNBraintree from '@ekreative/react-native-braintree';
RNBraintree.showPayPalModule({
clientToken: 'CLIENT_TOKEN_GENERATED_ON_SERVER_SIDE',
amount: '1.0',
currencyCode: 'EUR',
// Change button text to “Complete Purchase", optional
userAction: 'commit',
})
.then(result => console.log(result))
.catch((error) => console.log(error));
import RNBraintree from '@ekreative/react-native-braintree';
RNBraintree.tokenizeCard({
clientToken: 'CLIENT_TOKEN_GENERATED_ON_SERVER_SIDE',
number: '1111222233334444',
expirationMonth: '11',
expirationYear: '24',
cvv: '123',
postalCode: '',
})
.then(result => console.log(result))
.catch((error) => console.log(error));
import RNBraintree from '@ekreative/react-native-braintree';
RNBraintree.run3DSecureCheck({
// Optional if you ran `tokenizeCard()` or other Braintree methods before
clientToken: 'CLIENT_TOKEN_GENERATED_ON_SERVER_SIDE',
nonce: 'CARD_NONCE',
amount: '122.00',
// Pass as many of the following fields as possible, but they're optional
email: 'email@mail.com',
firstname: '',
lastname: '',
phoneNumber: '',
streetAddress: '',
streetAddress2: '',
city: '',
region: '',
postalCode: '',
countryCode: ''
})
.then(result => console.log(result))
.catch((error) => console.log(error));
import RNBraintree from '@ekreative/react-native-braintree';
RNBraintree.requestPayPalBillingAgreement({
clientToken: 'CLIENT_TOKEN_GENERATED_ON_SERVER_SIDE',
description: 'BILLING_AGRREEMENT_DESCRIPTION',
localeCode: 'LOCALE_CODE'
})
.then(result => console.log(result))
.catch((error) => console.log(error));
import RNBraintree from '@ekreative/react-native-braintree';
console.log(RNBraintree.isApplePayAvailable())
import RNBraintree from '@ekreative/react-native-braintree';
RNBraintree.runApplePay({
clientToken: 'CLIENT_TOKEN_GENERATED_ON_SERVER_SIDE',
companyName: 'Company',
amount: '100.0',
currencyCode: 'EUR'
})
.then(result => console.log(result))
.catch((error) => console.log(error));
import RNBraintree from '@ekreative/react-native-braintree';
RNBraintree.runGooglePay({
clientToken: 'CLIENT_TOKEN_GENERATED_ON_SERVER_SIDE',
amount: '100.0',
currencyCode: 'EUR'
})
.then(result => console.log(result))
.catch((error) => console.log(error));
getDeviceData
method to call new DataCollector(mBraintreeClient).collectDeviceData()
only once (it seems like it's currently may be called a second time from the setup
method) https://github.com/ekreative/react-native-braintree/pull/37#issuecomment-1752470507getDeviceData
method in other methods, such as tokenizeCard
, showPayPalModule
https://github.com/ekreative/react-native-braintree/pull/37#issuecomment-1752470507If you want to read further you can follow these links
FAQs
A react native interface for integrating payments using Braintree
We found that @ekreative/react-native-braintree demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.