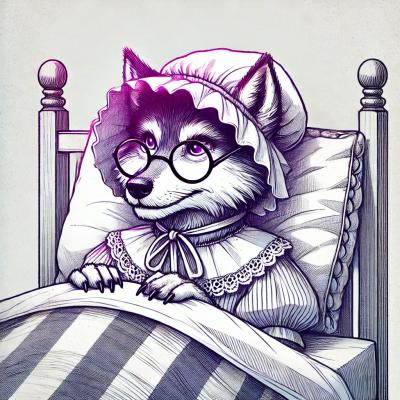
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
@electron/get
Advanced tools
@electron/get is an npm package designed to facilitate the downloading of Electron binaries. It provides a simple API to fetch Electron versions, which can be useful for setting up Electron-based projects or managing different versions of Electron.
Download a specific version of Electron
This feature allows you to download a specific version of Electron. The code sample demonstrates how to download Electron version 13.1.7 and log the path where it was downloaded.
const { download } = require('@electron/get');
(async () => {
const zipPath = await download('v13.1.7');
console.log(`Downloaded Electron v13.1.7 to ${zipPath}`);
})();
Specify a mirror for downloading
This feature allows you to specify a mirror URL for downloading Electron binaries. The code sample shows how to download Electron version 13.1.7 from a custom mirror.
const { download } = require('@electron/get');
(async () => {
const zipPath = await download('v13.1.7', {
mirrorOptions: {
mirror: 'https://mirror.example.com/electron/'
}
});
console.log(`Downloaded Electron v13.1.7 from mirror to ${zipPath}`);
})();
Get the URL for a specific Electron version
This feature allows you to get the download URL for a specific version of Electron without actually downloading it. The code sample demonstrates how to get the URL for Electron version 13.1.7.
const { getDownloadUrl } = require('@electron/get');
(async () => {
const url = await getDownloadUrl('v13.1.7');
console.log(`Download URL for Electron v13.1.7: ${url}`);
})();
electron-download is another package that helps in downloading Electron binaries. It is often used internally by other Electron-related tools. Compared to @electron/get, it offers similar functionalities but may not be as straightforward to use for some specific tasks.
electron-builder is a complete solution to package and build Electron applications. While its primary focus is on building and packaging, it also includes functionality to download Electron binaries. It is more feature-rich compared to @electron/get but may be overkill if you only need to download Electron binaries.
electron-prebuilt-compile is a package that provides prebuilt Electron binaries with support for custom compilation. It is useful for developers who need to compile Electron with specific settings. Compared to @electron/get, it offers more flexibility but requires more setup.
Download Electron release artifacts
import { download } from '@electron/get';
// NB: Use this syntax within an async function, Node does not have support for
// top-level await as of Node 12.
const zipFilePath = await download('4.0.4');
import { downloadArtifact } from '@electron/get';
// NB: Use this syntax within an async function, Node does not have support for
// top-level await as of Node 12.
const zipFilePath = await downloadArtifact({
version: '4.0.4',
platform: 'darwin',
artifactName: 'electron',
artifactSuffix: 'symbols',
arch: 'x64',
});
Anatomy of a download URL, in terms of mirrorOptions
:
https://github.com/electron/electron/releases/download/v4.0.4/electron-v4.0.4-linux-x64.zip
| | | |
------------------------------------------------------- -----------------------------
| |
mirror / nightly_mirror | | customFilename
------
||
customDir
Example:
import { download } from '@electron/get';
const zipFilePath = await download('4.0.4', {
mirrorOptions: {
mirror: 'https://mirror.example.com/electron/',
customDir: 'custom',
customFilename: 'unofficial-electron-linux.zip'
}
});
// Will download from https://mirror.example.com/electron/custom/unofficial-electron-linux.zip
const nightlyZipFilePath = await download('8.0.0-nightly.20190901', {
mirrorOptions: {
nightly_mirror: 'https://nightly.example.com/',
customDir: 'nightlies',
customFilename: 'nightly-linux.zip'
}
});
// Will download from https://nightly.example.com/nightlies/nightly-linux.zip
This module downloads Electron to a known place on your system and caches it so that future requests for that asset can be returned instantly. The cache locations are:
$XDG_CACHE_HOME
or ~/.cache/electron/
~/Library/Caches/electron/
%LOCALAPPDATA%/electron/Cache
or ~/AppData/Local/electron/Cache/
By default, the module uses got
as the
downloader. As a result, you can use the same options
via downloadOptions
.
Downstream packages should utilize the initializeProxy
function to add HTTP(S) proxy support. If
the environment variable ELECTRON_GET_USE_PROXY
is set, it is called automatically. A different
proxy module is used, depending on the version of Node in use, and as such, there are slightly
different ways to set the proxy environment variables. For Node 10 and above,
global-agent
is used. Otherwise,
global-tunnel-ng
is used. Refer to the
appropriate linked module to determine how to configure proxy support.
FAQs
Utility for downloading artifacts from different versions of Electron
The npm package @electron/get receives a total of 0 weekly downloads. As such, @electron/get popularity was classified as not popular.
We found that @electron/get demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.