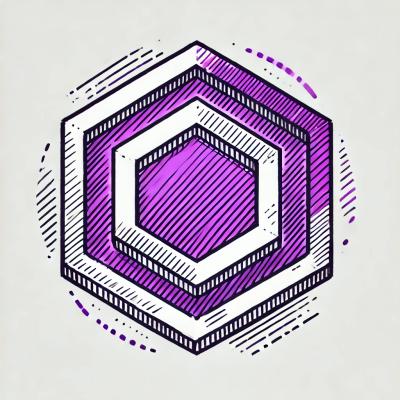
Security News
ESLint is Now Language-Agnostic: Linting JSON, Markdown, and Beyond
ESLint has added JSON and Markdown linting support with new officially-supported plugins, expanding its versatility beyond JavaScript.
@elrondnetwork/attest.web
Advanced tools
The Browser implementation of the __Elrond Attest__ service, built using [Node.js](https://nodejs.org/en/) and [Typescript](https://www.typescriptlang.org/).
The Browser implementation of the Elrond Attest service, built using Node.js and Typescript.
// Creating a hash
import { hash } from '@elrondnetwork/attest.web'
// (file: File) => Promise<HashResult>
const hashResult = await hash(file) // regular Web API file,
// see https://developer.mozilla.org/en-US/docs/Web/API/File
// Creating the attest client
import { attest } from '@elrondnetwork/attest.web'
// Only one of the following fields should be used at once
const authorization = {
token: 'sampleToken',
}
const client = attest({ authorization })
// The client can then be used to access the attestation functions
// Fetching an user's account
// () => Promise<Result>
const account = await client.account()
// Fetching an user's attestations
// Both of the following fields are nullable
const parameter = {
sha256: 'some hash', // Last hash after which to fetch attestations
timeStamp: 'some timestamp' // Last timestamp after which to fetch attestations
}
// (input: AttestationParameter) => Promise<Result>
const attestations = await client.attestations(parameter)
// Creating an attestation
const input = {
file,
options: {
shouldStoreObject: true // Flag to store generated object alongside attestation
}
}
// (input: { file: File, onProgress?: onProgress, options?: Options }) => Promise<Result>
const createResponse = await client.create(input)
// Verifying if a file is already attested
const input = "some existing hash" // Or use the reader if you don't already have a hash
const verifyResponse = await client.verify(input)
TokenAuthorization: { baseUrl: string, token: string }
Type to be used for authorizing the client by access token
AttestWeb: { attestations, account, create, verify }
Type of the attest client
FAQs
The Browser implementation of the __Elrond Attest__ service, built using [Node.js](https://nodejs.org/en/) and [Typescript](https://www.typescriptlang.org/).
We found that @elrondnetwork/attest.web demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 12 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
ESLint has added JSON and Markdown linting support with new officially-supported plugins, expanding its versatility beyond JavaScript.
Security News
Members Hub is conducting large-scale campaigns to artificially boost Discord server metrics, undermining community trust and platform integrity.
Security News
NIST has failed to meet its self-imposed deadline of clearing the NVD's backlog by the end of the fiscal year. Meanwhile, CVE's awaiting analysis have increased by 33% since June.