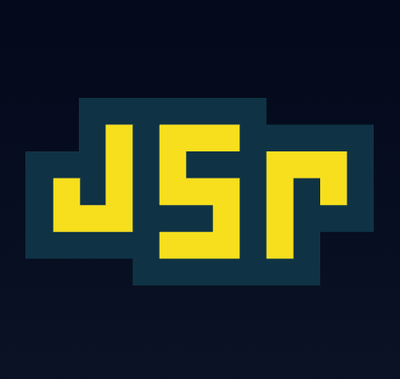
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
@emotion/css
Advanced tools
The @emotion/css package is a powerful library for writing CSS styles in JavaScript. It allows developers to style their applications efficiently with JavaScript and leverage the full power of CSS in their applications. It supports dynamic styling, which is particularly useful for theming, adjusting styles based on props, and more.
Basic Styling
This feature allows you to create basic CSS styles using template literals. The styles are then compiled into class names that can be applied to your components.
import { css } from '@emotion/css';
const className = css`
color: hotpink;
font-size: 20px;
`;
Dynamic Styling
Dynamic styling enables the creation of styles that can change based on inputs, such as props in a component. This is useful for theming or any situation where styles need to change based on user input or application state.
import { css } from '@emotion/css';
const dynamicStyle = (color) => css`
color: ${color};
font-size: 20px;
`;
Composition
Composition allows you to build complex styles by combining multiple simple ones. This promotes reusability and modularity in your style definitions.
import { css } from '@emotion/css';
const base = css`color: darkgray;`;
const override = css`
${base};
font-size: 16px;
`;
Styled-components is a library for React and React Native that allows you to use component-level styles in your application. It uses tagged template literals to style your components. It's similar to @emotion/css in providing a CSS-in-JS solution but differs in its API and the way it integrates with components.
JSS (JavaScript Style Sheets) is a CSS-in-JS library that allows you to write CSS in JavaScript. It supports plugins to extend its capabilities and can be used with or without React. Compared to @emotion/css, JSS offers a more extensive plugin system but has a different syntax and setup process.
Linaria is a zero-runtime CSS-in-JS library that extracts CSS to separate files at build time, resulting in smaller bundle sizes. Unlike @emotion/css, which injects styles at runtime, Linaria's approach to extracting static CSS files can lead to better performance for static sites or server-rendered applications.
The @emotion/css package is framework agnostic and the simplest way to use Emotion.
Get up and running with a single import.
npm install --save @emotion/css
import { css } from '@emotion/css'
const app = document.getElementById('root')
const myStyle = css`
color: rebeccapurple;
`
app.classList.add(myStyle)
The css
function accepts styles as a template literal, object, or array of objects and returns a class name. It is the foundation of emotion.
// @live
import { css } from '@emotion/css'
const color = 'darkgreen'
render(
<div
className={css`
background-color: hotpink;
&:hover {
color: ${color};
}
`}
>
This has a hotpink background.
</div>
)
// @live
import { css } from '@emotion/css'
const color = 'darkgreen'
render(
<div
className={css({
backgroundColor: 'hotpink',
'&:hover': {
color
}
})}
>
This has a hotpink background.
</div>
)
// @live
import { css } from '@emotion/css'
const color = 'darkgreen'
const isDanger = true
render(
<div
className={css([
{
backgroundColor: 'hotpink',
'&:hover': {
color
}
},
isDanger && {
color: 'red'
}
])}
>
This has a hotpink background.
</div>
)
injectGlobal
injects styles into the global scope and is useful for applications such as css resets or font faces.
import { injectGlobal } from '@emotion/css'
injectGlobal`
* {
box-sizing: border-box;
}
@font-face {
font-family: 'Patrick Hand SC';
font-style: normal;
font-weight: 400;
src: local('Patrick Hand SC'),
local('PatrickHandSC-Regular'),
url(https://fonts.gstatic.com/s/patrickhandsc/v4/OYFWCgfCR-7uHIovjUZXsZ71Uis0Qeb9Gqo8IZV7ckE.woff2)
format('woff2');
unicode-range: U+0100-024f, U+1-1eff,
U+20a0-20ab, U+20ad-20cf, U+2c60-2c7f,
U+A720-A7FF;
}
`
keyframes
generates a unique animation name that can be used to animate elements with CSS animations.
String Styles
// @live
import { css, keyframes } from '@emotion/css'
const bounce = keyframes`
from, 20%, 53%, 80%, to {
transform: translate3d(0,0,0);
}
40%, 43% {
transform: translate3d(0, -30px, 0);
}
70% {
transform: translate3d(0, -15px, 0);
}
90% {
transform: translate3d(0,-4px,0);
}
`
render(
<img
className={css`
width: 96px;
height: 96px;
border-radius: 50%;
animation: ${bounce} 1s ease infinite;
transform-origin: center bottom;
`}
src={logoUrl}
/>
)
Object Styles
// @live
import { css, keyframes } from '@emotion/css'
const bounce = keyframes({
'from, 20%, 53%, 80%, to': {
transform: 'translate3d(0,0,0)'
},
'40%, 43%': {
transform: 'translate3d(0, -30px, 0)'
},
'70%': {
transform: 'translate3d(0, -15px, 0)'
},
'90%': {
transform: 'translate3d(0, -4px, 0)'
}
})
render(
<img
src={logoUrl}
className={css({
width: 96,
height: 96,
borderRadius: '50%',
animation: `${bounce} 1s ease infinite`,
transformOrigin: 'center bottom'
})}
/>
)
cx
is emotion's version of the popular classnames
library. The key advantage of cx
is that it detects emotion generated class names ensuring styles are overwritten in the correct order. Emotion generated styles are applied from left to right. Subsequent styles overwrite property values of previous styles.
Combining class names
import { cx, css } from '@emotion/css'
const cls1 = css`
font-size: 20px;
background: green;
`
const cls2 = css`
font-size: 20px;
background: blue;
`
<div className={cx(cls1, cls2)} />
Conditional class names
const cls1 = css`
font-size: 20px;
background: green;
`
const cls2 = css`
font-size: 20px;
background: blue;
`
const foo = true
const bar = false
<div
className={cx(
{ [cls1]: foo },
{ [cls2]: bar }
)}
/>
Using class names from other sources
const cls1 = css`
font-size: 20px;
background: green;
`
<div
className={cx(cls1, 'profile')}
/>
With @emotion/css/create-instance
, you can provide custom options to Emotion's cache.
The main @emotion/css
entrypoint can be thought of as a call to @emotion/css/create-instance
with sensible defaults for most applications.
import createEmotion from '@emotion/css/create-instance'
export const {
flush,
hydrate,
cx,
merge,
getRegisteredStyles,
injectGlobal,
keyframes,
css,
sheet,
cache
} = createEmotion()
Calling it directly will allow for some low level customization.
Create custom names for emotion APIs to help with migration from other, similar libraries.
Could set custom key
to something other than css
Introduces some amount of complexity to your application that can vary depending on developer experience.
Required to keep up with changes in the repo and API at a lower level than if using @emotion/css
directly
Using emotion in embedded contexts such as an <iframe/>
Setting a nonce on any <style/>
tag emotion creates for security purposes
Use emotion with a container different than document.head
for style elements
Using emotion with custom stylis plugins
import createEmotion from '@emotion/css/create-instance'
export const {
flush,
hydrate,
cx,
merge,
getRegisteredStyles,
injectGlobal,
keyframes,
css,
sheet,
cache
} = createEmotion({
// The key option is required when there will be multiple instances in a single app
key: 'some-key'
})
createEmotion
accepts the same options as createCache from @emotion/cache
.
FAQs
The Next Generation of CSS-in-JS.
The npm package @emotion/css receives a total of 2,331,169 weekly downloads. As such, @emotion/css popularity was classified as popular.
We found that @emotion/css demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.