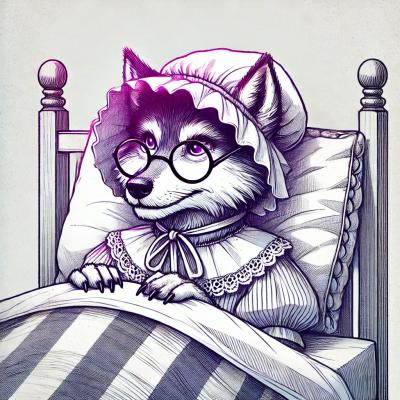
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
@entity-factory/core
Advanced tools
Entity Factory is a library used for quickly creating fixture data from plain objects or classes using faker. Inspired by laravel's factories for generating test data. Currently the library supports plain JS objects and Typeorm entities.
npm install --save @entity-factory/core
Try it on Runkit
const { EntityFactory, ObjectBlueprint } = require('@entity-factory/core');
export const entityFactory = new EntityFactory();
entityFactory.register(bp => {
// Set the key used for identifying an object in the factory.
bp.type('user');
bp.define(async faker => ({
username: faker.internet.userName(),
email: faker.internet.email(),
active: faker.random.boolean(),
}));
// define a state transform to return a user with embedded posts
bp.state('with-posts', async faker => ({
posts: async factory => factory.for('post').create(2),
}));
});
entityFactory.register(bp => {
bp.type('post');
bp.define(async faker => ({
title: faker.company.bsBuzz(),
body: faker.lorem.sentences(2),
}));
});
// Generate entities
entityFactory
.for('user') // get builder instance for 'user'
.state('with-posts') // get users with posts
.create(3) // generate 3 users with incrementing id's
.then(users => console.log(users));
If you wish to retain more control over the structure of your code blueprints can be defined in classes.
const entityFactory = new EntityFactory({
adapter: new OjectAdapter()
blueprints: [
'**/*.blueprint.js', // glob pattern
new UserBlueprint(), // blueprint instance
PostBlueprint, // blueprint reference
],
})
Create a new entity factory instance. Opts is optional and can contain the following attributes.
new ObjectAdapter()
. The adapter is
used when making and creating objects. Available adapters
_ ObjectAdapter: Creates standard javascript objects with incrementing
id values.
_ TypeormAdapter: Makes and persists TyprORM entities.[]
. The profiles array accepts an
array of glob patterns, Profile instances or Profile classes.
_ glob patterns: ex: ’src/\*\*/_.profile.js'
. If a glob pattern is passed EntityFactory will attempt to load all profiles found in the path and register them. * Class Reference: exUserProfile
. If a class reference is passed Entity factory will create a new instance and register it. * Profile Instance: Exnew UserProfile()
. If a profile instance is
provided EntityFactory will register it.class UserProfile extends ObjectBlueprint {}
// Accepts a reference or an instance to a blueprint
factory.register(UserBlueprint);
factory.register(new PostBlueprint());
// or a callback
factory.register(bp => {
bp.type('user');
bp.define(/*...*/);
});
Registers a profile with the factory
const users = await factory.for('user').create(3);
const users = await factory.for(User).create(3);
type(key)
in a blueprint and used to retrieve the profile from the factory
and supply it to the ProfileBuilder
.Definging blueprint can be done either via callback to EntityFactory.register()
or as a separate Blueprint class.
Class based blueprint must extend a blueprint class. Each adapter has it's own blueprint class which provides different available options specific to the adapter. By default the Object adapter can be used with Entity Factory. For more information on the options available please refer to the docs for each adapter blueprint.
// class based Blueprint definition
export class UserBlueprint extends ObjectBlueprint {
constructor() {
super();
this.type('user');
this.define(async faker => {
return {
// user attributes
};
});
}
}
// class based blueprints are passed to entity factory via the constructor
const factory = new EntityFactory({
blueprints: [UserBlueprint],
});
Registering a blueprint via a callback is an alternative to using a blueprint class. It does however have it's drawbacks. Using this method will always return a basic Blueprint class that is not specific to the adapter being used. As a result any additional functionality provided by the adapters blueprint will not be available with this method.
const factory = new EntityFactory();
factory.register(bp => {
bp.type('user');
bp.define(async faker => {
return {
// user attributes
};
});
});
Type is used as an identifier within entity factory and can be either a string
or a class reference that is passed. An important thing to note is that the
value passed to type()
will effect the value returned. Types passed as string
will be created as plain javascript objects. If however a class reference is
provided then Entity factory will attempt to return an instantiated instance of
that class.
This method must be called
class User {}
blueprint.type(User);
// or
blueprint.type('user');
Each adapter blueprint can specify different available options. The options are passed to the adapter at runtime. For adapter blueprint specific options please refer to their documentation:
blueprint.options({
idAttribute: '_id',
});
Used to create the primary factory method for an entity. The callback method receives a single argument which is a faker instance.
Property values can also an async callback to the factory which will allow data to be resolved from related factories.
This method must be called
blueprint.define(faker => {
return {
// faker usage
username: faker.internet.userName(),
// fixed values
active: true,
// async facory callback
posts: async factory => await factory.for('post').create(2),
};
});
Used to override portions of the primary factory method and/or to add additional properties.
Property values can also an async callback to the factory which will allow data to be resolved from related factories.
blueprint.state('inactive', faker => {
return {
active: false,
};
});
blueprint.state('with-posts', faker => {
return {
// factory callbacks can contain nested factory calls to resolve other
// blueprint instances from the factory.
posts: async factory => factory.for('posts').make(2),
};
});
Fired after a call to make()
on the builder instance. The entity received will
have been resolved to it's base type but not persisted. The callback will
receive the current entity as well as a context object.
callback
// called after calling builder.make()
blueprint.afterMaking(async (user, { faker, factory, adapter }) => {
// manipulate entity
});
// fired after calling builder.make() with an 'active' state transform
blueprint.afterMakingState(
'active',
async (user, { faker, factory, adapter }) => {
// manipulate entity
},
);
Fired after a call to create()
on the builder instance. The entity received
will have been resolved and persisted. The callback will receive the current
entity as well as a context object.
callback
// called after calling builder.create()
blueprint.afterCreating(async (user, { faker, factory, adapter }) => {
// manipulate entity
});
// fired after calling builder.create() with an 'active' state transform
blueprint.afterCreatingState(
'active',
async (user, { faker, factory, adapter }) => {
// manipulate entity
},
);
The builder is accessed via an entity factory instance by calling the for()
method.
factory
.for('user')
.state('active')
.with({
email: 'test@test.com',
})
.create();
Used to apply defined state transforms to a factory. The states are applied the order they are provided to the function.
factory.for(User).state('active', 'with-posts');
Used for overriding properties on a created entity. This is particularly useful when testing for a specific value.
factory.for(User).with({
name: 'john',
});
Resolves the partials generated by the factory methods and converts them into
plain objects or class instances. Unlike create()
the entity will not be
persisted
factory.for(User).make();
factory.for(User).make(1);
factory.for(User).make(2);
Resolves and persists the partials generated by the factory methods and converts them into plain objects and class instances. Depending on the adapter this can mean that they simple have an id generated for them or they are saved to teh database.
factory.for(User).create();
factory.for(User).create(1);
factory.for(User).create(2);
Entity Factory has the ability to use adapters to persist data in various formats. The ObjectAdapter is included in Entity Factory by default.
Available Adapters
The ObjectAdapter is used for creating mock data as plain javascript objects. It will generate sequential id's for objects to simulate data coming from a database.
const objectAdapter = new new ObjectAdapter()
// or
const objectAdapter = new ObjectAdaper({
generateId: true;
defaultIdAttribute: 'id';
});
const factory = new EntityFactory({
adapter: objectAdapter,
})
Available Options
export class WidgetBlueprint extends ObjectBlueprint {
constructor() {
super();
this.type(Widget);
this.options({
generateId: true
idAttribute: '_id';
})
}
}
FAQs
Create entities on the fly for mocking and testing
The npm package @entity-factory/core receives a total of 17 weekly downloads. As such, @entity-factory/core popularity was classified as not popular.
We found that @entity-factory/core demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.