@equinor/echo-base
Advanced tools
Comparing version 0.6.0 to 0.6.1
@@ -17,15 +17,40 @@ import { BaseErrorArgs } from '../types/error'; | ||
errorTraceId: string; | ||
private fixMissingStackTraceId; | ||
constructor({ name, message, innerError }: BaseErrorArgs); | ||
private fixMissingErrorTraceId; | ||
/** | ||
* Recursively converts all properties (included nested innerErrors) to type of Record<string, unknown> | ||
* @returns The converted properties as Record<string, unknown>, or an empty object | ||
*/ | ||
getProperties(): Record<string, unknown>; | ||
/** | ||
* Returns the innerError or undefined | ||
* @returns innerError or undefined | ||
*/ | ||
getInnerError(): Record<string, unknown> | Error | undefined; | ||
/** | ||
* Recursively converts all properties of innerError to type of Record<string, unknown> | ||
* @returns The converted properties of innerError as Record<string, unknown>, or an empty object | ||
*/ | ||
getInnerErrorProperties(): Record<string, unknown>; | ||
getProperties(): Record<string, unknown>; | ||
tryToFindPropertyByName(propertyName: string): unknown | undefined; | ||
/** | ||
* Find a property with the specified propertyName. | ||
* @param propertyName the name of the property to find | ||
* @param deepSearch default true, also search nested objects | ||
* @returns the value of the property found or undefined | ||
*/ | ||
findPropertyByName(propertyName: string, deepSearch?: boolean): unknown | undefined; | ||
} | ||
export declare function tryToFindPropertyByName(object: Record<string, unknown> | Error, propertyName: string): unknown | undefined; | ||
/** | ||
* Find a property with the specified propertyName on the object | ||
* @param object the object to search | ||
* @param propertyName the name of the property to find | ||
* @param deepSearch default true, also search nested objects | ||
* @returns the value of the property found or undefined | ||
*/ | ||
export declare function findPropertyByName(object: Record<string, unknown> | Error, propertyName: string, deepSearch?: boolean): unknown | undefined; | ||
/** | ||
* Converts an object to a Record<string, unknown>. | ||
* Useful for logging errors to AppInsight, and preserve all properties of an Error. | ||
* Supports custom Error which has public or private fields. Will ignore any functions on the Error object. | ||
* It does not support cycling error references. | ||
* It does not support cycling references (A -> B -> A). | ||
* @param objectWithProperties an object containing properties | ||
@@ -32,0 +57,0 @@ * @returns a record containing all properties of the object |
import { __extends } from "tslib"; | ||
import { randomId } from './randomHelper'; | ||
/** | ||
@@ -31,3 +32,3 @@ * Base Error class is intended to be used as a base class for every type of Error generated | ||
_this.innerError = innerError; | ||
_this.fixMissingStackTraceId(innerError); | ||
_this.fixMissingErrorTraceId(innerError); | ||
var fallBackNameWillBeObfuscatedInProduction = _this.constructor.name; | ||
@@ -40,30 +41,51 @@ _this.name = name !== null && name !== void 0 ? name : fallBackNameWillBeObfuscatedInProduction; | ||
} | ||
BaseError.prototype.fixMissingStackTraceId = function (exceptionWithErrorTraceId) { | ||
BaseError.prototype.fixMissingErrorTraceId = function (innerError) { | ||
if (this.errorTraceId) | ||
return; | ||
this.errorTraceId = tryToFindPropertyByName(exceptionWithErrorTraceId, 'errorTraceId'); | ||
if (!this.errorTraceId) { | ||
this.errorTraceId = "frontEnd_".concat(randomId()); | ||
} | ||
var maybeErrorTraceIdFromBackend = findPropertyByName(innerError, 'errorTraceId'); | ||
this.errorTraceId = maybeErrorTraceIdFromBackend !== null && maybeErrorTraceIdFromBackend !== void 0 ? maybeErrorTraceIdFromBackend : "frontEnd_".concat(randomId()); | ||
}; | ||
/** | ||
* Recursively converts all properties (included nested innerErrors) to type of Record<string, unknown> | ||
* @returns The converted properties as Record<string, unknown>, or an empty object | ||
*/ | ||
BaseError.prototype.getProperties = function () { | ||
return getAllProperties(this); | ||
}; | ||
/** | ||
* Returns the innerError or undefined | ||
* @returns innerError or undefined | ||
*/ | ||
BaseError.prototype.getInnerError = function () { | ||
return this.innerError; | ||
}; | ||
/** | ||
* Recursively converts all properties of innerError to type of Record<string, unknown> | ||
* @returns The converted properties of innerError as Record<string, unknown>, or an empty object | ||
*/ | ||
BaseError.prototype.getInnerErrorProperties = function () { | ||
return getAllProperties(this.innerError); | ||
}; | ||
BaseError.prototype.getProperties = function () { | ||
return getAllProperties(this); | ||
/** | ||
* Find a property with the specified propertyName. | ||
* @param propertyName the name of the property to find | ||
* @param deepSearch default true, also search nested objects | ||
* @returns the value of the property found or undefined | ||
*/ | ||
BaseError.prototype.findPropertyByName = function (propertyName, deepSearch) { | ||
if (deepSearch === void 0) { deepSearch = true; } | ||
return findPropertyByName(this, propertyName, deepSearch); | ||
}; | ||
BaseError.prototype.tryToFindPropertyByName = function (propertyName) { | ||
return tryToFindPropertyByName(this, propertyName); | ||
}; | ||
return BaseError; | ||
}(Error)); | ||
export { BaseError }; | ||
var randomId = function () { | ||
return Math.random().toString(36).substring(2, 11); | ||
}; | ||
//TODO check if we have unitTest for missing property should return undefined | ||
export function tryToFindPropertyByName(object, propertyName) { | ||
/** | ||
* Find a property with the specified propertyName on the object | ||
* @param object the object to search | ||
* @param propertyName the name of the property to find | ||
* @param deepSearch default true, also search nested objects | ||
* @returns the value of the property found or undefined | ||
*/ | ||
export function findPropertyByName(object, propertyName, deepSearch) { | ||
if (deepSearch === void 0) { deepSearch = true; } | ||
var properties = getAllProperties(object); | ||
@@ -74,6 +96,14 @@ var value = properties[propertyName]; | ||
} | ||
var innerProperties = properties['innerError']; //TODO check that this is working after prod build obfuscation | ||
if (innerProperties) { | ||
return tryToFindPropertyByName(innerProperties, propertyName); | ||
var maybeFound = undefined; | ||
if (deepSearch) { | ||
var propertyNames = properties ? Object.getOwnPropertyNames(properties) : []; | ||
propertyNames.forEach(function (name) { | ||
var innerProperties = object[name]; | ||
var valueType = typeof innerProperties; | ||
if (!maybeFound && valueType === 'object') { | ||
maybeFound = findPropertyByName(innerProperties, propertyName); | ||
} | ||
}); | ||
} | ||
return maybeFound; | ||
} | ||
@@ -84,3 +114,3 @@ /** | ||
* Supports custom Error which has public or private fields. Will ignore any functions on the Error object. | ||
* It does not support cycling error references. | ||
* It does not support cycling references (A -> B -> A). | ||
* @param objectWithProperties an object containing properties | ||
@@ -87,0 +117,0 @@ * @returns a record containing all properties of the object |
@@ -13,7 +13,7 @@ import { BaseError } from './BaseError'; | ||
* Fall backs to ErrorType with @type {NetworkError} as base, if none of the above constraints are fulfilled | ||
* @param args Arguments to initialize error with. Add custom properties in args.exception as desired, | ||
* like: .exception = { aCustomProperty: 'test custom property' }; | ||
* access with: tryToFindPropertyByName('aCustomProperty') or .getInnerErrorProperties()['aCustomProperty'] | ||
* @param args Arguments to initialize error with. Add InnerError or custom properties in args.innerError as desired, | ||
* like: innerError = { new NetworkError(...) } or innerError = { aCustomProperty: 'test custom property' }; | ||
* access with: findPropertyByName('aCustomProperty') or .getInnerErrorProperties()['aCustomProperty'] | ||
* @returns Specific error type with base as type | ||
*/ | ||
export declare function initializeNetworkError(args: NetworkErrorArgs): BaseError; |
@@ -12,5 +12,5 @@ import { BackendError, BadRequestError, ForbiddenError, NetworkError, NotFoundError, UnauthorizedError, ValidationError } from './NetworkError'; | ||
* Fall backs to ErrorType with @type {NetworkError} as base, if none of the above constraints are fulfilled | ||
* @param args Arguments to initialize error with. Add custom properties in args.exception as desired, | ||
* like: .exception = { aCustomProperty: 'test custom property' }; | ||
* access with: tryToFindPropertyByName('aCustomProperty') or .getInnerErrorProperties()['aCustomProperty'] | ||
* @param args Arguments to initialize error with. Add InnerError or custom properties in args.innerError as desired, | ||
* like: innerError = { new NetworkError(...) } or innerError = { aCustomProperty: 'test custom property' }; | ||
* access with: findPropertyByName('aCustomProperty') or .getInnerErrorProperties()['aCustomProperty'] | ||
* @returns Specific error type with base as type | ||
@@ -17,0 +17,0 @@ */ |
export * from './errors'; | ||
export * from './module'; | ||
export * from './types'; | ||
export { EchoEvents } from './types/event'; | ||
export * from './utils'; |
export * from './errors'; | ||
export * from './module'; | ||
export * from './types'; | ||
export { EchoEvents } from './types/event'; | ||
export * from './utils'; | ||
//# sourceMappingURL=index.js.map |
@@ -9,10 +9,5 @@ /** | ||
subscribe<T>(key: string, handler: (payload: T) => void): UnsubscribeFunction; | ||
subscribeMany<T>(keys: Array<string | EchoEvents>, handler: (payload: T) => void): UnsubscribeFunction; | ||
subscribeMany<T>(keys: Array<string>, handler: (payload: T) => void): UnsubscribeFunction; | ||
} | ||
export declare type UnsubscribeFunction = () => void; | ||
export declare enum EchoEvents { | ||
PlantChanged = "plantChanged", | ||
ProcosysProjectChanged = "procosysProjectChanged", | ||
Toaster = "toaster" | ||
} | ||
export interface EventMap { | ||
@@ -19,0 +14,0 @@ [custom: string]: unknown; |
@@ -1,7 +0,2 @@ | ||
export var EchoEvents; | ||
(function (EchoEvents) { | ||
EchoEvents["PlantChanged"] = "plantChanged"; | ||
EchoEvents["ProcosysProjectChanged"] = "procosysProjectChanged"; | ||
EchoEvents["Toaster"] = "toaster"; | ||
})(EchoEvents || (EchoEvents = {})); | ||
export {}; | ||
//# sourceMappingURL=event.js.map |
@@ -1,2 +0,2 @@ | ||
import { EchoEventHub, EchoEvents, UnsubscribeFunction } from '../types/event'; | ||
import { EchoEventHub, UnsubscribeFunction } from '../types/event'; | ||
/** | ||
@@ -12,7 +12,7 @@ * Class for creating an eventHub to be used for emitting and subscribing to either | ||
* @template T | ||
* @param {(string | EchoEvents)} key EchoEvent identifier | ||
* @param {(string)} key EchoEvent identifier | ||
* @param {T} payload EchoEvent payload - either message string, an object with more details | ||
* @memberof EventHub | ||
*/ | ||
emit<T>(key: string | EchoEvents, payload: T): void; | ||
emit<T>(key: string, payload: T): void; | ||
/** | ||
@@ -22,3 +22,3 @@ * Function for subscribing to an event. | ||
* @template T | ||
* @param {(string | EchoEvents)} key EchoEvent identifier | ||
* @param {(string)} key EchoEvent identifier | ||
* @param {(payload: T) => void} handler A function to handle a raised event | ||
@@ -28,3 +28,3 @@ * @return {*} Returns a cleanup function for unsubscribing to the event {() => void} | ||
*/ | ||
subscribe<T>(key: string | EchoEvents, handler: (payload: T) => void): UnsubscribeFunction; | ||
subscribe<T>(key: string, handler: (payload: T) => void): UnsubscribeFunction; | ||
/** | ||
@@ -34,3 +34,3 @@ * Function for subscribing to an array of events. | ||
* @template T | ||
* @param {(Array<string | EchoEvents>)} keys An Array of EchoEvent identifiers | ||
* @param {(Array<string>)} keys An Array of EchoEvent identifiers | ||
* @param {(payload: T) => void} handler A function to handle one of the raised events | ||
@@ -40,5 +40,5 @@ * @return {*} Returns cleanup function for unsubscribing from the subscribed events {() => void} | ||
*/ | ||
subscribeMany<T>(keys: Array<string | EchoEvents>, handler: (payload: T) => void): UnsubscribeFunction; | ||
subscribeMany<T>(keys: Array<string>, handler: (payload: T) => void): UnsubscribeFunction; | ||
} | ||
export declare const eventHub: EventHub; | ||
export {}; |
@@ -13,3 +13,3 @@ /** | ||
* @template T | ||
* @param {(string | EchoEvents)} key EchoEvent identifier | ||
* @param {(string)} key EchoEvent identifier | ||
* @param {T} payload EchoEvent payload - either message string, an object with more details | ||
@@ -26,3 +26,3 @@ * @memberof EventHub | ||
* @template T | ||
* @param {(string | EchoEvents)} key EchoEvent identifier | ||
* @param {(string)} key EchoEvent identifier | ||
* @param {(payload: T) => void} handler A function to handle a raised event | ||
@@ -45,3 +45,3 @@ * @return {*} Returns a cleanup function for unsubscribing to the event {() => void} | ||
* @template T | ||
* @param {(Array<string | EchoEvents>)} keys An Array of EchoEvent identifiers | ||
* @param {(Array<string>)} keys An Array of EchoEvent identifiers | ||
* @param {(payload: T) => void} handler A function to handle one of the raised events | ||
@@ -48,0 +48,0 @@ * @return {*} Returns cleanup function for unsubscribing from the subscribed events {() => void} |
@@ -17,15 +17,40 @@ import { BaseErrorArgs } from '../types/error'; | ||
errorTraceId: string; | ||
private fixMissingStackTraceId; | ||
constructor({ name, message, innerError }: BaseErrorArgs); | ||
private fixMissingErrorTraceId; | ||
/** | ||
* Recursively converts all properties (included nested innerErrors) to type of Record<string, unknown> | ||
* @returns The converted properties as Record<string, unknown>, or an empty object | ||
*/ | ||
getProperties(): Record<string, unknown>; | ||
/** | ||
* Returns the innerError or undefined | ||
* @returns innerError or undefined | ||
*/ | ||
getInnerError(): Record<string, unknown> | Error | undefined; | ||
/** | ||
* Recursively converts all properties of innerError to type of Record<string, unknown> | ||
* @returns The converted properties of innerError as Record<string, unknown>, or an empty object | ||
*/ | ||
getInnerErrorProperties(): Record<string, unknown>; | ||
getProperties(): Record<string, unknown>; | ||
tryToFindPropertyByName(propertyName: string): unknown | undefined; | ||
/** | ||
* Find a property with the specified propertyName. | ||
* @param propertyName the name of the property to find | ||
* @param deepSearch default true, also search nested objects | ||
* @returns the value of the property found or undefined | ||
*/ | ||
findPropertyByName(propertyName: string, deepSearch?: boolean): unknown | undefined; | ||
} | ||
export declare function tryToFindPropertyByName(object: Record<string, unknown> | Error, propertyName: string): unknown | undefined; | ||
/** | ||
* Find a property with the specified propertyName on the object | ||
* @param object the object to search | ||
* @param propertyName the name of the property to find | ||
* @param deepSearch default true, also search nested objects | ||
* @returns the value of the property found or undefined | ||
*/ | ||
export declare function findPropertyByName(object: Record<string, unknown> | Error, propertyName: string, deepSearch?: boolean): unknown | undefined; | ||
/** | ||
* Converts an object to a Record<string, unknown>. | ||
* Useful for logging errors to AppInsight, and preserve all properties of an Error. | ||
* Supports custom Error which has public or private fields. Will ignore any functions on the Error object. | ||
* It does not support cycling error references. | ||
* It does not support cycling references (A -> B -> A). | ||
* @param objectWithProperties an object containing properties | ||
@@ -32,0 +57,0 @@ * @returns a record containing all properties of the object |
"use strict"; | ||
Object.defineProperty(exports, "__esModule", { value: true }); | ||
exports.getAllProperties = exports.tryToFindPropertyByName = exports.BaseError = void 0; | ||
exports.getAllProperties = exports.findPropertyByName = exports.BaseError = void 0; | ||
var tslib_1 = require("tslib"); | ||
var randomHelper_1 = require("./randomHelper"); | ||
/** | ||
@@ -34,3 +35,3 @@ * Base Error class is intended to be used as a base class for every type of Error generated | ||
_this.innerError = innerError; | ||
_this.fixMissingStackTraceId(innerError); | ||
_this.fixMissingErrorTraceId(innerError); | ||
var fallBackNameWillBeObfuscatedInProduction = _this.constructor.name; | ||
@@ -43,30 +44,51 @@ _this.name = name !== null && name !== void 0 ? name : fallBackNameWillBeObfuscatedInProduction; | ||
} | ||
BaseError.prototype.fixMissingStackTraceId = function (exceptionWithErrorTraceId) { | ||
BaseError.prototype.fixMissingErrorTraceId = function (innerError) { | ||
if (this.errorTraceId) | ||
return; | ||
this.errorTraceId = tryToFindPropertyByName(exceptionWithErrorTraceId, 'errorTraceId'); | ||
if (!this.errorTraceId) { | ||
this.errorTraceId = "frontEnd_".concat(randomId()); | ||
} | ||
var maybeErrorTraceIdFromBackend = findPropertyByName(innerError, 'errorTraceId'); | ||
this.errorTraceId = maybeErrorTraceIdFromBackend !== null && maybeErrorTraceIdFromBackend !== void 0 ? maybeErrorTraceIdFromBackend : "frontEnd_".concat((0, randomHelper_1.randomId)()); | ||
}; | ||
/** | ||
* Recursively converts all properties (included nested innerErrors) to type of Record<string, unknown> | ||
* @returns The converted properties as Record<string, unknown>, or an empty object | ||
*/ | ||
BaseError.prototype.getProperties = function () { | ||
return getAllProperties(this); | ||
}; | ||
/** | ||
* Returns the innerError or undefined | ||
* @returns innerError or undefined | ||
*/ | ||
BaseError.prototype.getInnerError = function () { | ||
return this.innerError; | ||
}; | ||
/** | ||
* Recursively converts all properties of innerError to type of Record<string, unknown> | ||
* @returns The converted properties of innerError as Record<string, unknown>, or an empty object | ||
*/ | ||
BaseError.prototype.getInnerErrorProperties = function () { | ||
return getAllProperties(this.innerError); | ||
}; | ||
BaseError.prototype.getProperties = function () { | ||
return getAllProperties(this); | ||
/** | ||
* Find a property with the specified propertyName. | ||
* @param propertyName the name of the property to find | ||
* @param deepSearch default true, also search nested objects | ||
* @returns the value of the property found or undefined | ||
*/ | ||
BaseError.prototype.findPropertyByName = function (propertyName, deepSearch) { | ||
if (deepSearch === void 0) { deepSearch = true; } | ||
return findPropertyByName(this, propertyName, deepSearch); | ||
}; | ||
BaseError.prototype.tryToFindPropertyByName = function (propertyName) { | ||
return tryToFindPropertyByName(this, propertyName); | ||
}; | ||
return BaseError; | ||
}(Error)); | ||
exports.BaseError = BaseError; | ||
var randomId = function () { | ||
return Math.random().toString(36).substring(2, 11); | ||
}; | ||
//TODO check if we have unitTest for missing property should return undefined | ||
function tryToFindPropertyByName(object, propertyName) { | ||
/** | ||
* Find a property with the specified propertyName on the object | ||
* @param object the object to search | ||
* @param propertyName the name of the property to find | ||
* @param deepSearch default true, also search nested objects | ||
* @returns the value of the property found or undefined | ||
*/ | ||
function findPropertyByName(object, propertyName, deepSearch) { | ||
if (deepSearch === void 0) { deepSearch = true; } | ||
var properties = getAllProperties(object); | ||
@@ -77,8 +99,16 @@ var value = properties[propertyName]; | ||
} | ||
var innerProperties = properties['innerError']; //TODO check that this is working after prod build obfuscation | ||
if (innerProperties) { | ||
return tryToFindPropertyByName(innerProperties, propertyName); | ||
var maybeFound = undefined; | ||
if (deepSearch) { | ||
var propertyNames = properties ? Object.getOwnPropertyNames(properties) : []; | ||
propertyNames.forEach(function (name) { | ||
var innerProperties = object[name]; | ||
var valueType = typeof innerProperties; | ||
if (!maybeFound && valueType === 'object') { | ||
maybeFound = findPropertyByName(innerProperties, propertyName); | ||
} | ||
}); | ||
} | ||
return maybeFound; | ||
} | ||
exports.tryToFindPropertyByName = tryToFindPropertyByName; | ||
exports.findPropertyByName = findPropertyByName; | ||
/** | ||
@@ -88,3 +118,3 @@ * Converts an object to a Record<string, unknown>. | ||
* Supports custom Error which has public or private fields. Will ignore any functions on the Error object. | ||
* It does not support cycling error references. | ||
* It does not support cycling references (A -> B -> A). | ||
* @param objectWithProperties an object containing properties | ||
@@ -91,0 +121,0 @@ * @returns a record containing all properties of the object |
@@ -13,7 +13,7 @@ import { BaseError } from './BaseError'; | ||
* Fall backs to ErrorType with @type {NetworkError} as base, if none of the above constraints are fulfilled | ||
* @param args Arguments to initialize error with. Add custom properties in args.exception as desired, | ||
* like: .exception = { aCustomProperty: 'test custom property' }; | ||
* access with: tryToFindPropertyByName('aCustomProperty') or .getInnerErrorProperties()['aCustomProperty'] | ||
* @param args Arguments to initialize error with. Add InnerError or custom properties in args.innerError as desired, | ||
* like: innerError = { new NetworkError(...) } or innerError = { aCustomProperty: 'test custom property' }; | ||
* access with: findPropertyByName('aCustomProperty') or .getInnerErrorProperties()['aCustomProperty'] | ||
* @returns Specific error type with base as type | ||
*/ | ||
export declare function initializeNetworkError(args: NetworkErrorArgs): BaseError; |
@@ -15,5 +15,5 @@ "use strict"; | ||
* Fall backs to ErrorType with @type {NetworkError} as base, if none of the above constraints are fulfilled | ||
* @param args Arguments to initialize error with. Add custom properties in args.exception as desired, | ||
* like: .exception = { aCustomProperty: 'test custom property' }; | ||
* access with: tryToFindPropertyByName('aCustomProperty') or .getInnerErrorProperties()['aCustomProperty'] | ||
* @param args Arguments to initialize error with. Add InnerError or custom properties in args.innerError as desired, | ||
* like: innerError = { new NetworkError(...) } or innerError = { aCustomProperty: 'test custom property' }; | ||
* access with: findPropertyByName('aCustomProperty') or .getInnerErrorProperties()['aCustomProperty'] | ||
* @returns Specific error type with base as type | ||
@@ -20,0 +20,0 @@ */ |
export * from './errors'; | ||
export * from './module'; | ||
export * from './types'; | ||
export { EchoEvents } from './types/event'; | ||
export * from './utils'; |
"use strict"; | ||
Object.defineProperty(exports, "__esModule", { value: true }); | ||
exports.EchoEvents = void 0; | ||
var tslib_1 = require("tslib"); | ||
@@ -8,5 +7,3 @@ (0, tslib_1.__exportStar)(require("./errors"), exports); | ||
(0, tslib_1.__exportStar)(require("./types"), exports); | ||
var event_1 = require("./types/event"); | ||
Object.defineProperty(exports, "EchoEvents", { enumerable: true, get: function () { return event_1.EchoEvents; } }); | ||
(0, tslib_1.__exportStar)(require("./utils"), exports); | ||
//# sourceMappingURL=index.js.map |
@@ -9,10 +9,5 @@ /** | ||
subscribe<T>(key: string, handler: (payload: T) => void): UnsubscribeFunction; | ||
subscribeMany<T>(keys: Array<string | EchoEvents>, handler: (payload: T) => void): UnsubscribeFunction; | ||
subscribeMany<T>(keys: Array<string>, handler: (payload: T) => void): UnsubscribeFunction; | ||
} | ||
export declare type UnsubscribeFunction = () => void; | ||
export declare enum EchoEvents { | ||
PlantChanged = "plantChanged", | ||
ProcosysProjectChanged = "procosysProjectChanged", | ||
Toaster = "toaster" | ||
} | ||
export interface EventMap { | ||
@@ -19,0 +14,0 @@ [custom: string]: unknown; |
"use strict"; | ||
Object.defineProperty(exports, "__esModule", { value: true }); | ||
exports.EchoEvents = void 0; | ||
var EchoEvents; | ||
(function (EchoEvents) { | ||
EchoEvents["PlantChanged"] = "plantChanged"; | ||
EchoEvents["ProcosysProjectChanged"] = "procosysProjectChanged"; | ||
EchoEvents["Toaster"] = "toaster"; | ||
})(EchoEvents = exports.EchoEvents || (exports.EchoEvents = {})); | ||
//# sourceMappingURL=event.js.map |
@@ -1,2 +0,2 @@ | ||
import { EchoEventHub, EchoEvents, UnsubscribeFunction } from '../types/event'; | ||
import { EchoEventHub, UnsubscribeFunction } from '../types/event'; | ||
/** | ||
@@ -12,7 +12,7 @@ * Class for creating an eventHub to be used for emitting and subscribing to either | ||
* @template T | ||
* @param {(string | EchoEvents)} key EchoEvent identifier | ||
* @param {(string)} key EchoEvent identifier | ||
* @param {T} payload EchoEvent payload - either message string, an object with more details | ||
* @memberof EventHub | ||
*/ | ||
emit<T>(key: string | EchoEvents, payload: T): void; | ||
emit<T>(key: string, payload: T): void; | ||
/** | ||
@@ -22,3 +22,3 @@ * Function for subscribing to an event. | ||
* @template T | ||
* @param {(string | EchoEvents)} key EchoEvent identifier | ||
* @param {(string)} key EchoEvent identifier | ||
* @param {(payload: T) => void} handler A function to handle a raised event | ||
@@ -28,3 +28,3 @@ * @return {*} Returns a cleanup function for unsubscribing to the event {() => void} | ||
*/ | ||
subscribe<T>(key: string | EchoEvents, handler: (payload: T) => void): UnsubscribeFunction; | ||
subscribe<T>(key: string, handler: (payload: T) => void): UnsubscribeFunction; | ||
/** | ||
@@ -34,3 +34,3 @@ * Function for subscribing to an array of events. | ||
* @template T | ||
* @param {(Array<string | EchoEvents>)} keys An Array of EchoEvent identifiers | ||
* @param {(Array<string>)} keys An Array of EchoEvent identifiers | ||
* @param {(payload: T) => void} handler A function to handle one of the raised events | ||
@@ -40,5 +40,5 @@ * @return {*} Returns cleanup function for unsubscribing from the subscribed events {() => void} | ||
*/ | ||
subscribeMany<T>(keys: Array<string | EchoEvents>, handler: (payload: T) => void): UnsubscribeFunction; | ||
subscribeMany<T>(keys: Array<string>, handler: (payload: T) => void): UnsubscribeFunction; | ||
} | ||
export declare const eventHub: EventHub; | ||
export {}; |
@@ -16,3 +16,3 @@ "use strict"; | ||
* @template T | ||
* @param {(string | EchoEvents)} key EchoEvent identifier | ||
* @param {(string)} key EchoEvent identifier | ||
* @param {T} payload EchoEvent payload - either message string, an object with more details | ||
@@ -29,3 +29,3 @@ * @memberof EventHub | ||
* @template T | ||
* @param {(string | EchoEvents)} key EchoEvent identifier | ||
* @param {(string)} key EchoEvent identifier | ||
* @param {(payload: T) => void} handler A function to handle a raised event | ||
@@ -48,3 +48,3 @@ * @return {*} Returns a cleanup function for unsubscribing to the event {() => void} | ||
* @template T | ||
* @param {(Array<string | EchoEvents>)} keys An Array of EchoEvent identifiers | ||
* @param {(Array<string>)} keys An Array of EchoEvent identifiers | ||
* @param {(payload: T) => void} handler A function to handle one of the raised events | ||
@@ -51,0 +51,0 @@ * @return {*} Returns cleanup function for unsubscribing from the subscribed events {() => void} |
{ | ||
"name": "@equinor/echo-base", | ||
"version": "0.6.0", | ||
"version": "0.6.1", | ||
"module": "esm/index.js", | ||
@@ -5,0 +5,0 @@ "main": "lib/index.js", |
@@ -34,2 +34,4 @@ 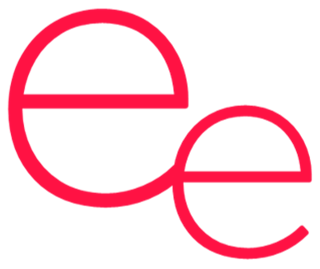 | ||
`exception` argument renamed to `innerError`, of type `Record<string, unknown> | Error` | ||
- Moved `EchoEvents` enum to `EchoCore`. | ||
- Changed types for `EventHub` event keys in all functions from `string | EchoEvents` to `string` only. | ||
@@ -36,0 +38,0 @@ v0.5.0: |
@@ -37,3 +37,3 @@ import { initializeNetworkError } from '../../errors/errorHandlers'; | ||
expect(result.message).toEqual('ValidationError 400 http://localhost:3000'); | ||
expect(result.tryToFindPropertyByName('dummyProperty')).not.toBe(true); | ||
expect(result.findPropertyByName('dummyProperty')).not.toBe(true); | ||
}); | ||
@@ -45,3 +45,3 @@ | ||
expect(result.message).toEqual('BackendError 400 http://localhost:3000'); | ||
const actualDummyProperty = result.tryToFindPropertyByName('dummyProperty'); | ||
const actualDummyProperty = result.findPropertyByName('dummyProperty'); | ||
expect(actualDummyProperty).toBe(true); | ||
@@ -117,3 +117,3 @@ }); | ||
expect(result.message).toEqual('BackendError 500 http://localhost:3000'); | ||
expect(result.tryToFindPropertyByName('dummyProperty')).toBe(true); | ||
expect(result.findPropertyByName('dummyProperty')).toBe(true); | ||
}); | ||
@@ -126,3 +126,3 @@ | ||
expect(result.message).toEqual('NetworkError 500 http://localhost:3000'); | ||
expect(result.tryToFindPropertyByName('dummyProperty')).toBe(undefined); | ||
expect(result.findPropertyByName('dummyProperty')).toBe(undefined); | ||
}); | ||
@@ -141,3 +141,3 @@ | ||
const result = initializeNetworkError(withExceptionCustomProperty); | ||
expect(result.tryToFindPropertyByName('aCustomProperty')).toBe( | ||
expect(result.findPropertyByName('aCustomProperty')).toBe( | ||
withExceptionCustomProperty.exception?.aCustomProperty | ||
@@ -144,0 +144,0 @@ ); |
@@ -38,3 +38,3 @@ import { BaseError, getAllProperties } from '../../errors'; | ||
stack: 'ignore', | ||
errorTraceId: 'frontEnd_ignore' | ||
errorTraceId: 'frontEnd_mocked-static-id-9999' | ||
}; | ||
@@ -75,3 +75,3 @@ | ||
stack: 'ignore', | ||
errorTraceId: 'frontEnd_ignore' | ||
errorTraceId: 'frontEnd_mocked-static-id-9999' | ||
}; | ||
@@ -78,0 +78,0 @@ |
@@ -1,2 +0,2 @@ | ||
import { BaseError, getAllProperties, tryToFindPropertyByName } from './BaseError'; | ||
import { BaseError, findPropertyByName, getAllProperties } from './BaseError'; | ||
@@ -36,3 +36,3 @@ describe('BaseError', () => { | ||
expect(actualError.errorTraceId).toBe('frontEnd_ignore'); | ||
expect(actualError.errorTraceId).toBe('frontEnd_mocked-static-id-9999'); | ||
}); | ||
@@ -74,3 +74,3 @@ | ||
describe('tryToFindPropertyByName', () => { | ||
describe('findPropertyByName', () => { | ||
const message = 'This is a custom error message for testing'; | ||
@@ -80,3 +80,3 @@ | ||
const error = new BaseError({ name: 'BaseError', message }); | ||
const actualPropertyMessage = tryToFindPropertyByName(error, 'message'); | ||
const actualPropertyMessage = findPropertyByName(error, 'message'); | ||
expect(actualPropertyMessage).toBe(message); | ||
@@ -91,3 +91,3 @@ }); | ||
}); | ||
const actualPropertyMessage = tryToFindPropertyByName(error, 'message'); | ||
const actualPropertyMessage = findPropertyByName(error, 'message'); | ||
expect(actualPropertyMessage).toBe(message); | ||
@@ -101,3 +101,3 @@ }); | ||
}); | ||
const actualPropertyMessage = tryToFindPropertyByName(error, 'message2'); | ||
const actualPropertyMessage = findPropertyByName(error, 'message2'); | ||
expect(actualPropertyMessage).toBe(undefined); | ||
@@ -112,6 +112,17 @@ }); | ||
}); | ||
const actualPropertyMessage = tryToFindPropertyByName(error, 'innerProperty'); | ||
const actualPropertyMessage = findPropertyByName(error, 'innerProperty'); | ||
expect(actualPropertyMessage).toBe(1); | ||
}); | ||
it('should not find property on nested innerError if deepSearch is false', () => { | ||
const error = new BaseError({ | ||
name: 'BaseError', | ||
message, | ||
innerError: { errors: { innerProperty: 1 } } | ||
}); | ||
const deepSearch = false; | ||
const actualPropertyMessage = findPropertyByName(error, 'innerProperty', deepSearch); | ||
expect(actualPropertyMessage).toBe(undefined); | ||
}); | ||
it('should find property on nested innerError of type customError', () => { | ||
@@ -123,3 +134,3 @@ const error = new BaseError({ | ||
}); | ||
const actualPropertyMessage = tryToFindPropertyByName(error, 'anotherProperty'); | ||
const actualPropertyMessage = findPropertyByName(error, 'anotherProperty'); | ||
expect(actualPropertyMessage).toBe('another value'); | ||
@@ -126,0 +137,0 @@ }); |
@@ -49,3 +49,3 @@ import { BaseErrorArgs } from '../types/error'; | ||
const maybeErrorTraceIdFromBackend = tryToFindPropertyByName(innerError, 'errorTraceId') as string; | ||
const maybeErrorTraceIdFromBackend = findPropertyByName(innerError, 'errorTraceId') as string; | ||
this.errorTraceId = maybeErrorTraceIdFromBackend ?? `frontEnd_${randomId()}`; | ||
@@ -79,8 +79,9 @@ } | ||
/** | ||
* Tries to find a property with the specified propertyName. | ||
* Find a property with the specified propertyName. | ||
* @param propertyName the name of the property to find | ||
* @param deepSearch default true, also search nested objects | ||
* @returns the value of the property found or undefined | ||
*/ | ||
tryToFindPropertyByName(propertyName: string): unknown | undefined { | ||
return tryToFindPropertyByName(this, propertyName); | ||
findPropertyByName(propertyName: string, deepSearch = true): unknown | undefined { | ||
return findPropertyByName(this, propertyName, deepSearch); | ||
} | ||
@@ -90,10 +91,12 @@ } | ||
/** | ||
* Tries to find a property with the specified propertyName on the object | ||
* Find a property with the specified propertyName on the object | ||
* @param object the object to search | ||
* @param propertyName the name of the property to find | ||
* @param deepSearch default true, also search nested objects | ||
* @returns the value of the property found or undefined | ||
*/ | ||
export function tryToFindPropertyByName( | ||
export function findPropertyByName( | ||
object: Record<string, unknown> | Error, | ||
propertyName: string | ||
propertyName: string, | ||
deepSearch = true | ||
): unknown | undefined { | ||
@@ -106,11 +109,14 @@ const properties = getAllProperties(object); | ||
const propertyNames = properties ? Object.getOwnPropertyNames(properties) : []; | ||
let maybeFound: unknown = undefined; | ||
propertyNames.forEach((name) => { | ||
const innerProperties = object[name]; | ||
const valueType = typeof innerProperties; | ||
if (!maybeFound && valueType === 'object') { | ||
maybeFound = tryToFindPropertyByName(innerProperties, propertyName); | ||
} | ||
}); | ||
if (deepSearch) { | ||
const propertyNames = properties ? Object.getOwnPropertyNames(properties) : []; | ||
propertyNames.forEach((name) => { | ||
const innerProperties = object[name]; | ||
const valueType = typeof innerProperties; | ||
if (!maybeFound && valueType === 'object') { | ||
maybeFound = findPropertyByName(innerProperties, propertyName); | ||
} | ||
}); | ||
} | ||
return maybeFound; | ||
@@ -117,0 +123,0 @@ } |
@@ -25,3 +25,3 @@ import { BaseError } from './BaseError'; | ||
* like: innerError = { new NetworkError(...) } or innerError = { aCustomProperty: 'test custom property' }; | ||
* access with: tryToFindPropertyByName('aCustomProperty') or .getInnerErrorProperties()['aCustomProperty'] | ||
* access with: findPropertyByName('aCustomProperty') or .getInnerErrorProperties()['aCustomProperty'] | ||
* @returns Specific error type with base as type | ||
@@ -28,0 +28,0 @@ */ |
export * from './errors'; | ||
export * from './module'; | ||
export * from './types'; | ||
export { EchoEvents } from './types/event'; | ||
export * from './utils'; |
@@ -9,3 +9,3 @@ /** | ||
subscribe<T>(key: string, handler: (payload: T) => void): UnsubscribeFunction; | ||
subscribeMany<T>(keys: Array<string | EchoEvents>, handler: (payload: T) => void): UnsubscribeFunction; | ||
subscribeMany<T>(keys: Array<string>, handler: (payload: T) => void): UnsubscribeFunction; | ||
} | ||
@@ -15,8 +15,2 @@ | ||
export enum EchoEvents { | ||
PlantChanged = 'plantChanged', | ||
ProcosysProjectChanged = 'procosysProjectChanged', | ||
Toaster = 'toaster' | ||
} | ||
export interface EventMap { | ||
@@ -23,0 +17,0 @@ [custom: string]: unknown; |
@@ -1,2 +0,2 @@ | ||
import { EchoEventHub, EchoEvents, UnsubscribeFunction } from '../types/event'; | ||
import { EchoEventHub, UnsubscribeFunction } from '../types/event'; | ||
@@ -13,7 +13,7 @@ /** | ||
* @template T | ||
* @param {(string | EchoEvents)} key EchoEvent identifier | ||
* @param {(string)} key EchoEvent identifier | ||
* @param {T} payload EchoEvent payload - either message string, an object with more details | ||
* @memberof EventHub | ||
*/ | ||
emit<T>(key: string | EchoEvents, payload: T): void { | ||
emit<T>(key: string, payload: T): void { | ||
const event = new CustomEvent(key, { detail: payload }); | ||
@@ -27,3 +27,3 @@ window.dispatchEvent(event); | ||
* @template T | ||
* @param {(string | EchoEvents)} key EchoEvent identifier | ||
* @param {(string)} key EchoEvent identifier | ||
* @param {(payload: T) => void} handler A function to handle a raised event | ||
@@ -33,3 +33,3 @@ * @return {*} Returns a cleanup function for unsubscribing to the event {() => void} | ||
*/ | ||
subscribe<T>(key: string | EchoEvents, handler: (payload: T) => void): UnsubscribeFunction { | ||
subscribe<T>(key: string, handler: (payload: T) => void): UnsubscribeFunction { | ||
const eventHandler = (e: Event): void => { | ||
@@ -48,3 +48,3 @@ const customEvent = e as CustomEvent; | ||
* @template T | ||
* @param {(Array<string | EchoEvents>)} keys An Array of EchoEvent identifiers | ||
* @param {(Array<string>)} keys An Array of EchoEvent identifiers | ||
* @param {(payload: T) => void} handler A function to handle one of the raised events | ||
@@ -54,3 +54,3 @@ * @return {*} Returns cleanup function for unsubscribing from the subscribed events {() => void} | ||
*/ | ||
subscribeMany<T>(keys: Array<string | EchoEvents>, handler: (payload: T) => void): UnsubscribeFunction { | ||
subscribeMany<T>(keys: Array<string>, handler: (payload: T) => void): UnsubscribeFunction { | ||
const unsubscribeFunctions: Array<() => void> = keys.map((key) => this.subscribe(key, handler)); | ||
@@ -57,0 +57,0 @@ return (): void => { |
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
New author
Supply chain riskA new npm collaborator published a version of the package for the first time. New collaborators are usually benign additions to a project, but do indicate a change to the security surface area of a package.
Found 1 instance in 1 package
269148
253
5458
50
0