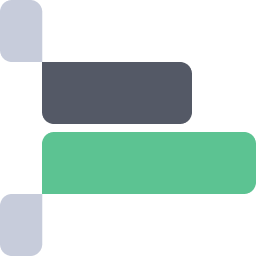
Rekey
A simple and performant zero dependency library that allows you to modify your object structure using references.
Installation
npm i @eramux/rekey
Usage
First we will need to create an object which will be passed to the rekey funcitons
let data = {
key1: "some string",
settings: {
name: "John",
active: true
},
users: [
{
name: "Marie"
},
{
name: "Steven",
type: "Human"
}
],
items: [
{
name: "abs"
},
{
name: "pvc",
supported: false
}
]
}
Rename key:
import { renameKey } from "@eramux/rekey"
renameKey(data, "settings.name", "username")
renameKey(data, "items.name", "material")
renameKey(data, "users.type", "species")
Rename key:
deleteKey(data, "users.name")
Since rekey does not copy the object, you won't have any unexpected memory spikes. It works by only modifying the reference recursively.
Contributions
Any contributions are welcome! Please make sure to first file an issue so we can discuss the problem at hand and you don't create a PR that doesn't get pulled.