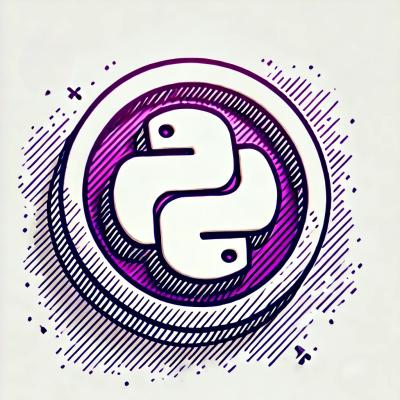
Security News
Introducing the Socket Python SDK
The initial version of the Socket Python SDK is now on PyPI, enabling developers to more easily interact with the Socket REST API in Python projects.
@eslint/plugin-kit
Advanced tools
A collection of utilities to help build ESLint plugins.
For Node.js and compatible runtimes:
npm install @eslint/plugin-kit
# or
yarn add @eslint/plugin-kit
# or
pnpm install @eslint/plugin-kit
# or
bun install @eslint/plugin-kit
For Deno:
deno add @eslint/plugin-kit
This package exports the following utilities:
ConfigCommentParser
- used to parse ESLint configuration comments (i.e., /* eslint-disable rule */
)VisitNodeStep
and CallMethodStep
- used to help implement SourceCode#traverse()
TextSourceCodeBase
- base class to help implement the SourceCode
interfaceConfigCommentParser
To use the ConfigCommentParser
class, import it from the package and create a new instance, such as:
import { ConfigCommentParser } from "@eslint/plugin-kit";
// create a new instance
const commentParser = new ConfigCommentParser();
// pass in a comment string without the comment delimiters
const directive = commentParser.parseDirective(
"eslint-disable prefer-const, semi -- I don't want to use these.",
);
// will be undefined when a directive can't be parsed
if (directive) {
console.log(directive.label); // "eslint-disable"
console.log(directive.value); // "prefer-const, semi"
console.log(directive.justification); // "I don't want to use these"
}
There are different styles of directive values that you'll need to parse separately to get the correct format:
import { ConfigCommentParser } from "@eslint/plugin-kit";
// create a new instance
const commentParser = new ConfigCommentParser();
// list format
const list = commentParser.parseListConfig("prefer-const, semi");
console.log(Object.entries(list)); // [["prefer-const", true], ["semi", true]]
// string format
const strings = commentParser.parseStringConfig("foo:off, bar");
console.log(Object.entries(strings)); // [["foo", "off"], ["bar", null]]
// JSON-like config format
const jsonLike = commentParser.parseJSONLikeConfig(
"semi:[error, never], prefer-const: warn",
);
console.log(Object.entries(jsonLike.config)); // [["semi", ["error", "never"]], ["prefer-const", "warn"]]
VisitNodeStep
and CallMethodStep
The VisitNodeStep
and CallMethodStep
classes represent steps in the traversal of source code. They implement the correct interfaces to return from the SourceCode#traverse()
method.
The VisitNodeStep
class is the more common of the two, where you are describing a visit to a particular node during the traversal. The constructor accepts three arguments:
target
- the node being visited. This is used to determine the method to call inside of a rule. For instance, if the node's type is Literal
then ESLint will call a method named Literal()
on the rule (if present).phase
- either 1 for enter or 2 for exit.args
- an array of arguments to pass into the visitor method of a rule.For example:
import { VisitNodeStep } from "@eslint/plugin-kit";
class MySourceCode {
traverse() {
const steps = [];
for (const { node, parent, phase } of iterator(this.ast)) {
steps.push(
new VisitNodeStep({
target: node,
phase: phase === "enter" ? 1 : 2,
args: [node, parent],
}),
);
}
return steps;
}
}
The CallMethodStep
class is less common and is used to tell ESLint to call a specific method on the rule. The constructor accepts two arguments:
target
- the name of the method to call, frequently beginning with "on"
such as "onCodePathStart"
.args
- an array of arguments to pass to the method.For example:
import { VisitNodeStep, CallMethodStep } from "@eslint/plugin-kit";
class MySourceCode {
traverse() {
const steps = [];
for (const { node, parent, phase } of iterator(this.ast)) {
steps.push(
new VisitNodeStep({
target: node,
phase: phase === "enter" ? 1 : 2,
args: [node, parent],
}),
);
// call a method indicating how many times we've been through the loop
steps.push(
new CallMethodStep({
target: "onIteration",
args: [steps.length]
});
)
}
return steps;
}
}
TextSourceCodeBase
The TextSourceCodeBase
class is intended to be a base class that has several of the common members found in SourceCode
objects already implemented. Those members are:
lines
- an array of text lines that is created automatically when the constructor is called.getLoc(node)
- gets the location of a node. Works for nodes that have the ESLint-style loc
property and nodes that have the Unist-style position
property. If you're using an AST with a different location format, you'll still need to implement this method yourself.getRange(node)
- gets the range of a node within the source text. Works for nodes that have the ESLint-style range
property and nodes that have the Unist-style position
property. If you're using an AST with a different range format, you'll still need to implement this method yourself.getText(nodeOrToken, charsBefore, charsAfter)
- gets the source text for the given node or token that has range information attached. Optionally, can return additional characters before and after the given node or token. As long as getRange()
is properly implemented, this method will just work.getAncestors(node)
- returns the ancestry of the node. In order for this to work, you must implement the getParent()
method yourself.Here's an example:
import { TextSourceCodeBase } from "@eslint/plugin-kit";
export class MySourceCode extends TextSourceCodeBase {
#parents = new Map();
constructor({ ast, text }) {
super({ ast, text });
}
getParent(node) {
return this.#parents.get(node);
}
traverse() {
const steps = [];
for (const { node, parent, phase } of iterator(this.ast)) {
//save the parent information
this.#parent.set(node, parent);
steps.push(
new VisitNodeStep({
target: node,
phase: phase === "enter" ? 1 : 2,
args: [node, parent],
}),
);
}
return steps;
}
}
In general, it's safe to collect the parent information during the traverse()
method as getParent()
and getAncestor()
will only be called from rules once the AST has been traversed at least once.
Apache 2.0
The following companies, organizations, and individuals support ESLint's ongoing maintenance and development. Become a Sponsor to get your logo on our README and website.
FAQs
Utilities for building ESLint plugins.
The npm package @eslint/plugin-kit receives a total of 1,270,562 weekly downloads. As such, @eslint/plugin-kit popularity was classified as popular.
We found that @eslint/plugin-kit demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The initial version of the Socket Python SDK is now on PyPI, enabling developers to more easily interact with the Socket REST API in Python projects.
Security News
Floating dependency ranges in npm can introduce instability and security risks into your project by allowing unverified or incompatible versions to be installed automatically, leading to unpredictable behavior and potential conflicts.
Security News
A new Rust RFC proposes "Trusted Publishing" for Crates.io, introducing short-lived access tokens via OIDC to improve security and reduce risks associated with long-lived API tokens.