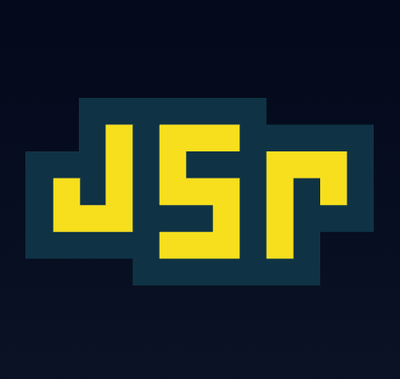
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
@ethereumjs/util
Advanced tools
@ethereumjs/util is a utility library for Ethereum-related operations. It provides a wide range of utility functions for handling Ethereum addresses, private keys, public keys, signatures, and other cryptographic operations. This package is essential for developers working on Ethereum-based applications, as it simplifies many common tasks.
Address Utilities
This feature allows you to convert an Ethereum address to its checksummed version, which includes mixed-case letters to help prevent errors.
const { toChecksumAddress } = require('@ethereumjs/util');
const address = '0x32Be343B94f860124dC4fEe278FDCBD38C102D88';
const checksumAddress = toChecksumAddress(address);
console.log(checksumAddress); // '0x32Be343B94f860124dC4fEe278FDCBD38C102D88'
Private Key Utilities
This feature allows you to derive an Ethereum address from a given private key.
const { privateToAddress } = require('@ethereumjs/util');
const privateKey = Buffer.from('c87509a1c067bbde78beb793e6fa1b2b4a5c4c9c3c8e3b3a3b3a3b3a3b3a3b3a', 'hex');
const address = privateToAddress(privateKey);
console.log(address.toString('hex')); // '0x32Be343B94f860124dC4fEe278FDCBD38C102D88'
Signature Utilities
This feature allows you to sign a message hash with a private key and convert the signature to RPC format.
const { ecsign, toRpcSig } = require('@ethereumjs/util');
const msgHash = Buffer.from('5c6ffbdd40d9556b73a21e63c3e0e904e4e1a8a0', 'hex');
const privateKey = Buffer.from('c87509a1c067bbde78beb793e6fa1b2b4a5c4c9c3c8e3b3a3b3a3b3a3b3a3b3a', 'hex');
const sig = ecsign(msgHash, privateKey);
const rpcSig = toRpcSig(sig.v, sig.r, sig.s);
console.log(rpcSig); // '0x...' (signature in RPC format)
ethereumjs-wallet is a library for creating and managing Ethereum wallets. It provides functionalities for generating private keys, addresses, and signing transactions. Compared to @ethereumjs/util, it is more focused on wallet management rather than general utility functions.
web3-utils is a utility library that is part of the Web3.js suite. It provides a wide range of utility functions for Ethereum-related operations, including encoding/decoding, hashing, and address validation. It overlaps with @ethereumjs/util in many areas but is part of a larger framework for interacting with the Ethereum blockchain.
ethers is a complete Ethereum library that includes utilities for interacting with the Ethereum blockchain, managing wallets, and performing cryptographic operations. It offers similar functionalities to @ethereumjs/util but is more comprehensive, providing a full suite of tools for Ethereum development.
Note: this README has been updated containing the changes from our next breaking release round [UNRELEASED] targeted for Summer 2023. See the README files from the maintenance-v6 branch for documentation matching our latest releases.
A collection of utility functions for Ethereum. |
---|
To obtain the latest version, simply require the project using npm
:
npm install @ethereumjs/util
import { hexToBytes, isValidChecksumAddress } from '@ethereumjs/util'
isValidChecksumAddress('0x2F015C60E0be116B1f0CD534704Db9c92118FB6A') // true
hexToBytes('0x342770c0')
With the breaking release round in Summer 2023 we have added hybrid ESM/CJS builds for all our libraries (see section below) and have eliminated many of the caveats which had previously prevented a frictionless browser usage.
It is now easily possible to run a browser build of one of the EthereumJS libraries within a modern browser using the provided ESM build. For a setup example see ./examples/browser.html.
Read the API docs.
KECCAK256_NULL_S
for string representation of Keccak-256 hash of null)DB
interfaceDepending on the extend of Buffer
usage within your own libraries and other planning considerations, there are the two upgrade options to do the switch to Uint8Array
yourself or keep Buffer
and do transitions for input and output values.
We have updated the @ethereumjs/util
bytes
module with helpers for the most common conversions:
Buffer.alloc(97) // Allocate a Buffer with length 97
new Uint8Array(97) // Allocate a Uint8Array with length 97
Buffer.from('342770c0', 'hex') // Convert a hex string to a Buffer
hexToBytes('0x342770c0') // Convert a prefixed hex string to a Uint8Array, Util.hexToBytes()
`0x${myBuffer.toString('hex')}` // Convert a Buffer to a prefixed hex string
bytesToHex(myUint8Array) // Convert a Uint8Array to a prefixed hex string
intToBuffer(9) // Convert an integer to a Buffer, old (removed)
intToBytes(9) // Convert an integer to a Uint8Array, Util.intToBytes()
bytesToInt(myUint8Array) // Convert a Uint8Array to an integer, Util.bytesToInt()
bigIntToBytes(myBigInt) // Convert a BigInt to a Uint8Array, Util.bigIntToBytes()
bytesToBigInt(myUint8Array) // Convert a Uint8Array to a BigInt, Util.bytesToInt()
utf8ToBytes(myUtf8String) // Converts a UTF-8 string to a Uint8Array, Util.utf8ToBytes()
bytesToUtf8(myUint8Array) // Converts a Uint8Array to a UTF-8 string, Util.bytesToUtf8()
toBuffer(v: ToBufferInputTypes) // Converts various byte compatible types to Buffer, old (removed)
toBytes(v: ToBytesInputTypes) // Converts various byte compatible types to Uint8Array, Util.toBytes()
Helper methods can be imported like this:
import { hexToBytes } from '@ethereumjs/util'
With the breaking releases from Summer 2023 we have started to ship our libraries with both CommonJS (cjs
folder) and ESM builds (esm
folder), see package.json
for the detailed setup.
If you use an ES6-style import
in your code files from the ESM build will be used:
import { EthereumJSClass } from '@ethereumjs/[PACKAGE_NAME]'
If you use Node.js specific require
the CJS build will be used:
const { EthereumJSClass } = require('@ethereumjs/[PACKAGE_NAME]')
Using ESM will give you additional advantages over CJS beyond browser usage like static code analysis / Tree Shaking which CJS can not provide.
With the breaking releases from Summer 2023 we have removed all Node.js specific Buffer
usages from our libraries and replace these with Uint8Array representations, which are available both in Node.js and the browser (Buffer
is a subclass of Uint8Array
).
We have converted existing Buffer conversion methods to Uint8Array conversion methods in the @ethereumjs/util bytes
module, see the respective README section for guidance.
Starting with Util v8 the usage of BN.js for big numbers has been removed from the library and replaced with the usage of the native JS BigInt data type (introduced in ES2020
).
Please note that number-related API signatures have changed along with this version update and the minimal build target has been updated to ES2020
.
The following methods are available by an internalized version of the ethjs-util package (MIT
license), see internal.ts. The original package is not maintained any more and the original functionality will be replaced by own implementations over time (starting with the v7.1.3
release, October 2021).
They can be imported by name:
import { stripHexPrefix } from '@ethereumjs/util'
See our organizational documentation for an introduction to EthereumJS
as well as information on current standards and best practices. If you want to join for work or carry out improvements on the libraries, please review our contribution guidelines first.
FAQs
A collection of utility functions for Ethereum
The npm package @ethereumjs/util receives a total of 549,481 weekly downloads. As such, @ethereumjs/util popularity was classified as popular.
We found that @ethereumjs/util demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.