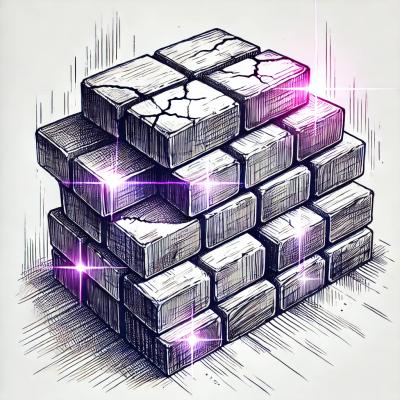
Security News
Input Validation Vulnerabilities Dominate MITRE's 2024 CWE Top 25 List
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
@etomon/encode-tools
Advanced tools
This package aggregates different libraries for encoding, serializing, generating ids and hashing things, exposing a common interface.
This package has been deprecated, see @znetstar/encode-tools-native for a drop-in replacement.
This package aggregates different libraries for encoding, serializing, compressing, generating ids and hashing things, exposing a common interface.
Many other packages serve the same purpose, but our objective is to ensure a consistent experience in both node.js and the browser and standardize the api so functions work the same way across different underlying libraries.
Etomon Encode Tools also has a command line wrapper encode-cli
.
Encoding a Buffer as base64url
let enc = new EncodeTools();
let buf = Buffer.from('hello world', 'utf8');
let newBuf = enc.encodeBuffer(buf, BinaryEncoding.base64url);
console.log(newBuf.toString('utf8'));
Hashing an object wth xxhash
let enc = new EncodeTools();
let obj = { foo: 'bar' };
let newBuf = await enc.hashObject(obj, HashAlgorithm.xxhash64);
console.log(newBuf.toString('utf8'));
Serializing an object wth msgpack
let enc = new EncodeTools();
let obj = { foo: 'bar' };
let newBuf = await enc.serializeObject(obj, SerializationFormat.msgpack);
console.log(newBuf.toString('base64'));
Generating a base64-encoded UUID v4
let enc = new EncodeTools();
let newBuf = await enc.uniqueId(IDFormat.uuidv4);
console.log(newBuf.toString('base64'));
Compressing a buffer with lzma
let enc = new EncodeTools();
let newBuf = await enc.compress(Buffer.from('hi', 'utf8'), CompressionFormat.lzma);
console.log(newBuf.toString('base64'));
Resizing a png image
let enc = new EncodeTools();
let imageBuf = await (await new Promise((resolve, reject) => {
new (Jimp)(500, 500, '#FFFFFF', (err: unknown, image: any) => {
if (err) reject(err);
else resolve(image);
});
})).getBufferAsync('image/png');
let myResizedPng = await enc.resizeImage(imageBuf, { width: 250 }, ImageFormat.png);
Below are a list of supported algorithms, their backing library, and their support in the browser.
Name | Browser? | Underlying Package |
---|---|---|
nodeBuffer | ✓ | buffer/(built-in) |
base64 | ✓ | (built-in) |
base64url | ✓ | (built-in) |
hex | ✓ | (built-in) |
base32 | ✓ | base32.js |
hashids | ✓ | hashids |
arrayBuffer | ✓ | (built-in) |
base85 (ascii85) | ✓ | base85 |
ascii85 | ✓ | base85 |
Name | Browser? | Underlying Package |
---|---|---|
crc32 | ✓ | hash-wasm |
xxhash3 | xxhash-addon | |
xxhash64 | ✓ | xxhash-addon/hash-wasm |
xxhash32 | ✓ | xxhash-addon/hash-wasm |
md5 | ✓ | hash-wasm |
sha1 | ✓ | hash-wasm |
sha2 | ✓ | hash-wasm |
sha3 | ✓ | hash-wasm |
bcrypt | ✓ | hash-wasm |
Name | Browser? | Underlying Package |
---|---|---|
uuidv4 | ✓ | uuid |
uuidv2 | ✓ | uuid |
uuidv4string | ✓ | uuid |
uuidv2string | ✓ | uuid |
objectId | ✓ | bson-ext/bson |
nanoid | ✓ | nanoid |
timestamp | ✓ | (built in) |
Name | Browser? | Underlying Package |
---|---|---|
json | ✓ | (built in) |
cbor | ✓ | cbor/cbor-web |
msgpack | ✓ | @msgpack/msgpack |
bson | ✓ | bson-ext/bson |
Name | Browser? | Underlying Package |
---|---|---|
zstd | ✓ | zstd-codec |
lzma | ✓ | lzma/lzma-native |
Name | Browser? | Underlying Package |
---|---|---|
png | ✓ | jimp/sharp |
jpeg | ✓ | jimp/sharp |
webp | sharp | |
avif | sharp | |
tiff | sharp | |
gif* | sharp |
Etomon Encode Tools runs in the browser and in node.js, with a few exceptions. The bson-ext
, lzma-native
, xxhash-addon
and cbor-extract
packages have native bindings, and so cannot run in the browser. For browser compatibility, the EncodeTools
class uses the pure javascript bson
, lzma
, hash-wsam
, and cbor-x
packages, respectively, to provide equivalent support albeit at the cost of performance. Additionally, hash-wsam
lacks support for xxhash3.
The EncodeToolsAuto
class will use the native packages bson-ext
, lzma-native
and xxhash-addon
(and any future native packages). bson-ext
, lzma-native
and xxhash-addon
are listed as optional dependencies, and NPM will attempt to install them automatically.
The constructor of EncodeToolsAuto
takes a second set of default EncodingOptions
to use as a fallback if it cannot
find the needed module.
const enc = new EncodeToolsAuto({ hashAlgorithm: HashAlgorithm.xxhash3 }, { hashAlgorithm: HashAlgorithm.xxhash64 });
if (enc.availableNativeModules.xxhashAddon)
console.log('should be xxhash3', await enc.hashString('Test'));
else
console.log('should be xxhash64', await enc.hashString('Test'));
The gif
image format in EncodeToolsAuto
requires libvips
compiled with ImageMagick support (as described here). I haven't had time to re-build libvips on my machine, so there are no mocha tests for the gif
format.
Please see the documentation located at https://etomonusa.github.io/encode-tools/
For issues with Webpack, try adding all the native dependencies to the externals
section.
{
externals: {
'xxhash-addon': 'commonjs xxhash-addon',
'bson-ext': 'commonjs bson-ext',
'shelljs': 'commonjs shelljs',
'lzma-native': 'commonjs lzma-native',
'sharp': 'commonjs sharp'
},
// For Webpack 5+ only, add `node-polyfill-webpack-plugin`
plugins: [
new (require('node-polyfill-webpack-plugin'))()
]
}
For Next.js, you can insert into next.config.js
{
webpack: (config, { isServer }) => {
if (!isServer) {
config.resolve.fallback = {
fs: false
}
config.externals = {
...config.externals,
'xxhash-addon': 'commonjs xxhash-addon',
'bson-ext': 'commonjs bson-ext',
'shelljs': 'commonjs shelljs',
'lzma-native': 'commonjs lzma-native',
'sharp': 'commonjs sharp'
}
config.plugins = [
...config.plugins,
new (require('node-polyfill-webpack-plugin'))()
]
}
return config;
}
}
Tests are written in Mocha, to run use npm test
.
Etomon Encode Tools is licensed under the GNU LGPL-3.0, a copy of which can be found at https://www.gnu.org/licenses/.
FAQs
This package aggregates different libraries for encoding, serializing, generating ids and hashing things, exposing a common interface.
The npm package @etomon/encode-tools receives a total of 37 weekly downloads. As such, @etomon/encode-tools popularity was classified as not popular.
We found that @etomon/encode-tools demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.